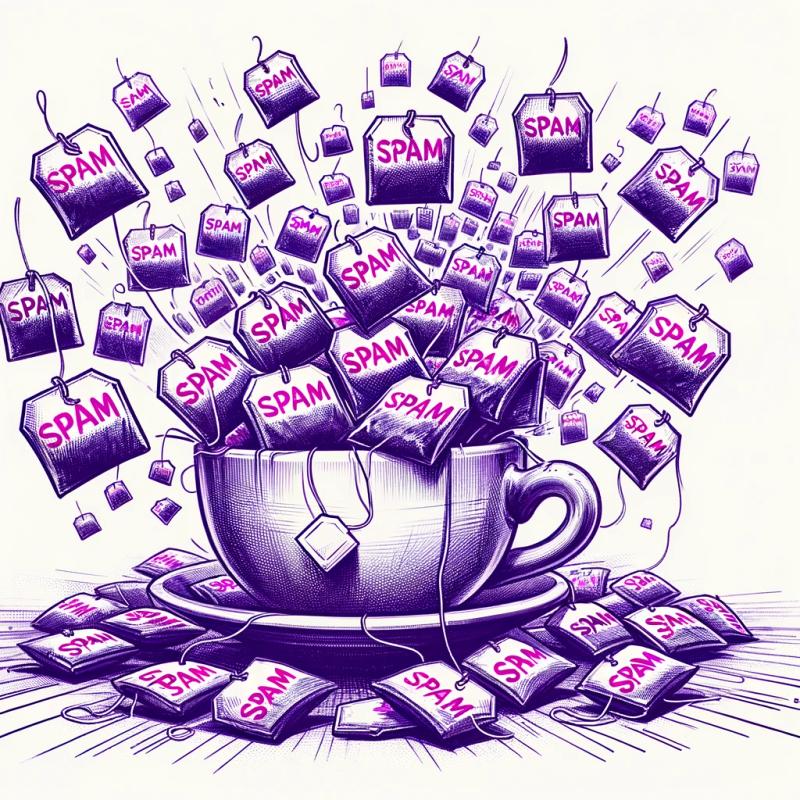
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
jsx-to-styled
Advanced tools
Readme
jsx-to-styled adds styled props to your React components
Inspired by xstyled and styled-system, jsx-to-styled adds styled props to your React styled-components. The main idea of this library is to stay really simple and performant.
All styled props injected by jsx-to-styled are prefixed by
$
symbol to prevent forwarding props to html element, check the styled-components transiant props section for more informations.
You must have react
and styled-components
already installed
npm i jsx-to-styled
# or
yarn add jsx-to-styled
You can use jsx-to-styled without theme (codesandbox example)
import styled from 'styled-components'
import system from 'jsx-to-styled'
// create your styled component with system props
const Box = styled.div(system)
// now you can use all jsx-to-styled props
return (
<Box $color="white" $backgroundColor="tomato" $p="30px" $borderRadius="50%">
Hello World!
</Box>
)
Or use with a theme to use custom values (codesandbox example)
import styled, { ThemeProvider } from 'styled-components'
import system from 'jsx-to-styled'
// create your styled component with system props
const Box = styled.div(system)
// create your theme
const theme = {
colors: {
primary: 'white',
secondary: 'tomato',
},
spaces: {
xl: '30px',
},
radii: {
half: '50%',
},
}
// now you can use all jsx-to-styled props with theme values
return (
<ThemeProvider theme={theme}>
<Box $color="primary" $backgroundColor="secondary" $p="xl" $borderRadius="half">
Hello World!
</Box>
</ThemeProvider>
)
Thanks to csstype, jsx-to-styled is fully typed. You will have autocomplete for all possible css values.
import system, { SystemProps } from 'jsx-to-styled'
// don't forget to add SystemProps type to your styled-component definition
const Box = styled.div<SystemProps>(system)
If you want to access to your theme values, you have to redefine "Theme" interface with your custom theme like that:
// theme.d.ts
import 'jsx-to-styled'
import { theme } from './theme'
type MyTheme = typeof theme
declare module 'jsx-to-styled' {
export interface Theme extends MyTheme {}
}
You can use breakpoints in your styled props (codesandbox example)
// add "breakpoints" key in your theme
const theme = {
colors: {
primary: 'white',
secondary: 'tomato',
},
breakpoints: {
sm: '600px',
},
}
// flexDirection will be "column" by default and "row" on screens > 600px
return (
<Box $display="flex" $flexDirection={{ _: 'column', sm: 'row' }}>
<Box>A</Box>
<Box>B</Box>
</Box>
)
You can use states in your styled props (codesandbox example)
// add "states" key in your theme
const theme = {
colors: {
primary: 'white',
secondary: 'tomato',
},
states: {
hover: '&:hover',
},
}
// color will be "white" by default and "tomato" on hover
return <Box $color={{ _: 'primary', hover: 'secondary' }}>Hello World!</Box>
System is composed by all of props below
JSX Property | CSS property | Theme key |
---|---|---|
$background | background | |
$backgroundImage | background-image | |
$backgroundSize | background-size | |
$backgroundPosition | background-position | |
$backgroundRepeat | background-repeat |
JSX Property | CSS property | Theme key |
---|---|---|
$border | border | |
$borderWidth | border-width | borderWidths |
$borderStyle | border-style | |
$borderColor | border-color | colors |
$borderRadius | border-radius | radii |
$borderTop | border-top | |
$borderTopWidth | border-top-width | borderWidths |
$borderTopStyle | border-top-style | |
$borderTopColor | border-top-color | colors |
$borderTopLeftRadius | border-top-left-radius | radii |
$borderTopRightRadius | border-top-right-radius | radii |
$borderRight | border-right | |
$borderRightWidth | border-right-width | borderWidths |
$borderRightStyle | border-right-style | |
$borderRightColor | border-right-color | colors |
$borderBottom | border-bottom | |
$borderBottomWidth | border-bottom-width | borderWidths |
$borderBottomStyle | border-bottom-style | |
$borderBottomColor | border-bottom-color | colors |
$borderBottomLeftRadius | border-bottom-left-radius | radii |
$borderBottomRightRadius | border-bottom-right-radius | radii |
$borderLeft | border-left | |
$borderLeftWidth | border-left-width | borderWidths |
$borderLeftStyle | border-left-style | |
$borderLeftColor | border-left-color | colors |
JSX Property | CSS property | Theme key |
---|---|---|
$backgroundColor | background-color | colors |
$color | color | colors |
$opacity | opacity |
JSX Property | CSS property | Theme key |
---|---|---|
$flex | flex | |
$alignContent | align-content | |
$alignItems | align-items | |
$alignSelf | align-self | |
$justifyContent | justify-content | |
$justifyItems | justify-items | |
$justifySelf | justify-self | |
$flexBasis | flex-basis | |
$flexDirection | flex-direction | |
$flexGrow | flex-grow | |
$flexShrink | flex-shrink | |
$flexWrap | flex-wrap | |
$order | order |
JSX Property | CSS property | Theme key |
---|---|---|
$gap | gap | spaces |
$columnGap | column-gap | spaces |
$rowGap | row-gap | spaces |
$gridColumn | grid-column | |
$gridRow | grid-row | |
$gridArea | grid-area | |
$gridAutoFlow | grid-auto-flow | |
$gridAutoColumns | grid-auto-columns | |
$gridAutoRows | grid-auto-rows | |
$gridTemplateColumns | grid-template-columns | |
$gridTemplateRows | grid-template-rows | |
$gridTemplateAreas | grid-template-areas |
JSX Property | CSS property | Theme key |
---|---|---|
$w | width | sizes |
$h | height | sizes |
$minW | min-width | sizes |
$maxW | max-width | sizes |
$minH | min-height | sizes |
$maxH | max-height | sizes |
$display | display | |
$verticalAlign | vertical-align | |
$overflow | overflow | |
$overflowX | overflow-x | |
$overflowY | overflow-y |
JSX Property | CSS property | Theme key |
---|---|---|
$position | position | |
$zIndex | z-index | |
$top | op | spaces |
$right | right | spaces |
$bottom | bottom | spaces |
$left | left | spaces |
JSX Property | CSS property | Theme key |
---|---|---|
$m | margin | spaces |
$mt | margin-top | spaces |
$mr | margin-right | spaces |
$mb | margin-bottom | spaces |
$ml | margin-left | spaces |
$my | margin-top, margin-bottom | spaces |
$mx | margin-right, margin-left | spaces |
$p | padding | spaces |
$pt | padding-top | spaces |
$pr | padding-right | spaces |
$pb | padding-bottom | spaces |
$pl | padding-left | spaces |
$py | padding-top, padding-bottom | spaces |
$px | padding-right, padding-left | spaces |
JSX Property | CSS property | Theme key |
---|---|---|
$fontFamily | font-family | fonts |
$fontSize | font-size | fontSizes |
$fontWeight | font-weight | fontWeights |
$lineHeight | line-height | lineHeights |
$letterSpacing | line-spacing | letterSpacings |
$textAlign | text-align | |
$fontStyle | font-style | |
$textDecoration | text-decoration | |
$textTransform | text-transform |
JSX Property | CSS property | Theme key |
---|---|---|
$cusor | cursor | |
$float | float | |
$objectFit | object-fit | |
$objectPosition | object-position | |
$transform | transform | |
$visibility | visibility |
FAQs
jsx-to-styled adds styled props to your React components
The npm package jsx-to-styled receives a total of 16 weekly downloads. As such, jsx-to-styled popularity was classified as not popular.
We found that jsx-to-styled demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.