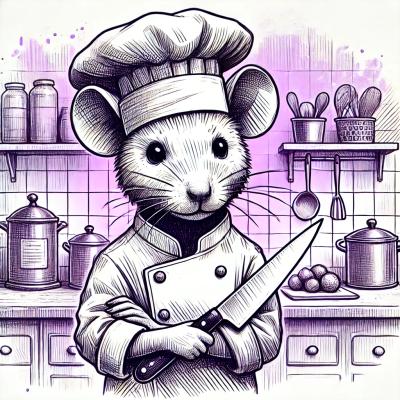
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
kentico-cloud-delivery
Advanced tools
A client library for retrieving content from Kentico Cloud written in TypeScript and published in following formats: UMD
, ES2015
and CommonJs
. Works both in browser & node.
Resources |
---|
Full Documentation |
Example apps |
API Reference |
Upgrade guide |
You can install this library using npm
or you can use global CDNs such jsdelivr
directly. In both cases, you will also need to include rxjs
as its listed as peer dependency.
npm i rxjs --save
npm i kentico-cloud-delivery --save
When using UMD bundle and including this library in script
tag on your html
page, you can find it under the kenticoCloudDelivery
global variable.
Bundles are distributed in _bundles
folder and there are several options that you can choose from.
kentico-cloud-delivery-sdk.browser.legacy.umd.min
if you need to support legacy browsers (IE9, IE10, IE11)kentico-cloud-delivery-sdk.browser.umd.min
if you intend to use SDK only in browsers (strips code specific to Node.js = smaller bundle)kentico-cloud-delivery-sdk.umd.min
if you need to use it in Node.jshttps://cdn.jsdelivr.net/npm/kentico-cloud-delivery/_bundles/kentico-cloud-delivery-sdk.browser.legacy.umd.min.js
https://cdn.jsdelivr.net/npm/kentico-cloud-delivery/_bundles/kentico-cloud-delivery-sdk.browser.umd.min.js
https://cdn.jsdelivr.net/npm/kentico-cloud-delivery/_bundles/kentico-cloud-delivery-sdk.umd.min.js
import {
ContentItem,
Elements,
TypeResolver,
DeliveryClient
} from 'kentico-cloud-delivery';
/**
* This is optional, but it is considered a best practice to define your models
* so you can leverage intellisense and so that you can extend your models with
* additional properties / methods.
*/
export class Movie extends ContentItem {
public title: Elements.TextElement;
}
const deliveryClient = new DeliveryClient({
projectId: 'xxx',
typeResolvers: [
new TypeResolver('movie', () => new Movie()),
]
});
/** Getting items from Kentico Cloud as Promise */
deliveryClient.items<Movie>()
.type('movie')
.toPromise()
.then(response => {
const movieText = response.items[0].title.value;
)
});
/** Getting items from Kentico Cloud as Observable */
deliveryClient.items<Movie>()
.type('movie')
.toObservable()
.subscribe(response => {
const movieText = response.items[0].title.value;
)
});
/**
* Get data without having custom models
*/
deliveryClient.items<ContentItem>()
.type('movie')
.get()
.subscribe(response => {
// you can access properties same way as with strongly typed models, but note
// that you don't get any intellisense and the underlying object
// instance is of 'ContentItem' type
console.log(response.items[0].title.value);
});
const KenticoCloud = require('kentico-cloud-delivery');
class Movie extends KenticoCloud.ContentItem {
constructor() {
super();
}
}
const deliveryClient = new KenticoCloud.DeliveryClient({
projectId: 'xxx',
typeResolvers: [
new KenticoCloud.TypeResolver('movie', () => new Movie()),
]
});
/** Getting items from Kentico Cloud as Promise */
deliveryClient.items()
.type('movie')
.toPromise()
.then(response => {
const movieText = response.items[0].title.value;
)
});
/** Getting items from Kentico Cloud as Observable */
const subscription = deliveryClient.items()
.type('movie')
.toObservable()
.subscribe(response => {
const movieText = response.items[0].title.value;
});
/*
Don't forget to unsubscribe from your Observables. You can use 'takeUntil' or 'unsubscribe' method for this purpose. Unsubsription is usually done when you no longer need to process the result of Observable. (Example: 'ngOnDestroy' event in Angular app)
*/
subscription.unsubscribe();
/**
* Fetch all items of 'movie' type and given parameters from Kentico Cloud.
* Important note: SDK will convert items to your type if you registered it. For example,
* in this case the objects will be of 'Movie' type we defined above.
* If you don't use custom models, 'ContentItem' object instances will be returned.
*/
deliveryClient.items()
.type('movie')
.toObservable()
.subscribe(response => console.log(response));
Bundles are distributed in _bundles
folder and there are several options that you can choose from.
kentico-cloud-delivery-sdk.browser.legacy.umd.min
if you need to support legacy browsers (IE9, IE10, IE11)kentico-cloud-delivery-sdk.browser.umd.min
if you intend to use SDK only in browsers (strips code specific to Node.js = smaller bundle)kentico-cloud-delivery-sdk.umd.min
if you need to use it in Node.js<!DOCTYPE html>
<html>
<head>
<title>Kentico Cloud SDK - Html sample</title>
<script type="text/javascript" src="https://unpkg.com/rxjs@6.4.0/bundles/rxjs.umd.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/kentico-cloud-delivery/_bundles/kentico-cloud-delivery-sdk.browser.umd.min.js"></script>
</head>
<body>
<script type="text/javascript">
var Kc = window['kenticoCloudDelivery'];
var deliveryClient = new Kc.DeliveryClient({
projectId: 'da5abe9f-fdad-4168-97cd-b3464be2ccb9'
});
deliveryClient.items()
.type('movie')
.toPromise()
.then(response => console.log(response));
</script>
<h1>See console</h1>
</body>
</html>
Note: You need to have Chrome
installed in order to run tests via Karma.
npm run test:browser
Runs tests in Chromenpm run test:node
Runs tests in node.jsnpm run test:dev
Runs developer tests (useful for testing functionality)npm run test:travis
Runs browser tests that are executed by travisIf you want to mock http responses, it is possible to use external implementation of configurable Http Service as a part of the delivery client configuration.
Feedback & Contributions are welcomed. Feel free to take/start an issue & submit PR.
FAQs
Official Kentico Cloud Delivery SDK
The npm package kentico-cloud-delivery receives a total of 80 weekly downloads. As such, kentico-cloud-delivery popularity was classified as not popular.
We found that kentico-cloud-delivery demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.