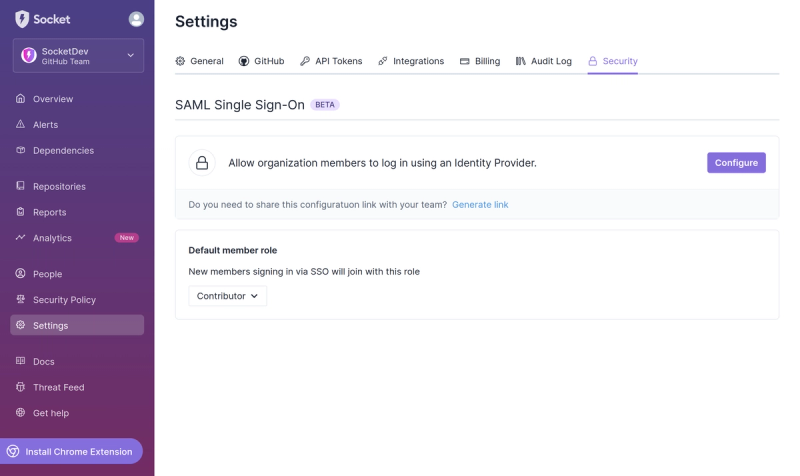
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
kentico-cloud-delivery-typescript-sdk
Advanced tools
Readme
A client library for retrieving content from Kentico Cloud that supports JavaScript and TypeScript.
Visit GitHub repository to see how you can use this SDK in Node.js environment.
npm i kentico-cloud-delivery-typescript-sdk --save
import { ContentItem, Fields,TypeResolver,DeliveryClient,DeliveryClientConfig } from 'kentico-cloud-delivery-typescript-sdk';
/**
* This is optional, but it is considered a best practice to define your models
* so you can leverage intellisense and so that you can extend your models with
* additional properties / methods.
*/
export class Movie extends ContentItem {
public title: Fields.TextField;
}
/**
* Type resolvers make sure instance of proper class is created for your content types.
* If you don't use any custom models, return an empty array.
*/
let typeResolvers: TypeResolver[] = [
new TypeResolver('movie', () => new Movie()),
];
/**
* Create new instance of Delivery Client
*/
var deliveryClient = new DeliveryClient(
new DeliveryClientConfig('projectId', typeResolvers)
);
/**
* Get typed data from Cloud (note that the 'Movie' has to be registered in your type resolvers)
*/
deliveryClient.items<Movie>()
.type('movie')
.get()
.subscribe(response => {
console.log(response);
// you can access strongly types properties
console.log(response.items[0].title.text);
});
/**
* Get data without having custom models
*/
deliveryClient.items<ContentItem>()
.type('movie')
.get()
.subscribe(response => {
console.log(response);
// you can access properties same way as with strongly typed models, but note
// that you don't get any intellisense and the underlying object
// instance is of 'ContentItem' type
console.log(response.items[0].title.text);
});
var KenticoCloud = require('kentico-cloud-delivery-typescript-sdk');
/**
* This is optional, but it is considered a best practice to define your models
* so that you can leverage intellisense and extend your models with
* additional methods.
*/
class Movie extends KenticoCloud.ContentItem {
constructor() {
super();
}
}
/**
* Type resolvers make sure instance of proper class is created for your content types.
* If you don't use any custom classes, return an empty array.
*/
var typeResolvers = [
new KenticoCloud.TypeResolver('movie', () => new Movie()),
];
/**
* Delivery client configuration object
*/
var config = new KenticoCloud.DeliveryClientConfig(projectId, typeResolvers);
/**
* Create new instance of Delivery Client
*/
var deliveryClient = new KenticoCloud.DeliveryClient(config);
/**
* Fetch all items of 'movie' type and given parameters from Kentico Cloud.
* Important note: SDK will convert items to your type if you registered it. For example,
* in this case the objects will be of 'Movie' type we defined above.
* If you don't use custom models, 'ContentItem' object instances will be returned.
*/
deliveryClient.items()
.type('movie')
.get()
.subscribe(response => console.log(response));
Note: You need to have Firefox
installed in order to run tests via Karma.
npm test
to run all tests.npm run dev-test
to run developer tests created in dev-test
folder. Use this for your testing purposes.In order to publish SDK first run one of following tasks to increase version & update sdk info file:
npm run new-patch
npm run new-minor
npm run new-major
And then run (note that tests and necessary scripts are automatically executed using the prepublishOnly
script):
npm run publish
Feedback & Contributions are welcomed. Feel free to take/start an issue & submit PR.
FAQs
Official Kentico Cloud Delivery SDK
The npm package kentico-cloud-delivery-typescript-sdk receives a total of 55 weekly downloads. As such, kentico-cloud-delivery-typescript-sdk popularity was classified as not popular.
We found that kentico-cloud-delivery-typescript-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.