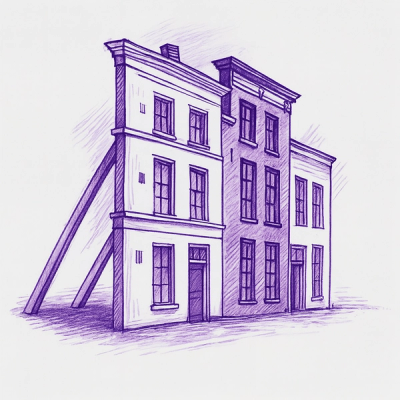
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
kik-node-api
Advanced tools
A chatting API for kik built with Node.js, based on https://github.com/tomer8007/kik-bot-api-unofficial
THIS IS NOT AN OFFICIAL API
Join the group chat on kik #kiknodeapi
NPM:
npm i kik-node-api
You can use the API by creating an instance of KikClient
, you'll use it to listen
to events and send requests to kik
const KikClient = require("kik-node-api");
Kik = new KikClient({
username: "username",
password: "1234",
promptCaptchas: true,
trackUserInfo: true,
trackFriendInfo: true
});
Kik.connect()
username
: your kik account's username
password
: your kik account's password
promptCaptchas
: prompt in the console to solve captchas. If not you must handle it yourself using the event
trackUserInfo
: track users and return their usernames and display names in the events when possible
trackFriendInfo
: track friends and return their usernames and display names in the events when possible
All users are represented in a js object that looks like this:
user: {
jid: "kikteam@talk.kik.com",
username: "kikteam",
displayName: "Kik Team",
pic: "http://profilepics.cf.kik.com/luN9IXX3a4sks-RzyiC7xlK-HdE"
}
groups are represented in the following js object:
group: {
jid: "1100221067977_g@groups.kik.com",
code: "#kikbotapi",
name: "Kik Bot API Unofficial",
users: [
{jid: "jid1", isOwner: true, isAdmin: true},
{jid: "jid2", isAdmin: true},
{jid: "jid3"}
]
}
private groups have a code of null
KikClient
uses Node's Event Emitter class
to handle events, all events are attached in the following way:
Kik.on(eventname, (param1, param2) => {
//do stuff with params here
})
Below are the details of all events emitted by the KikClient
class
Kik.on("authenticated", () => {
console.log("Authenticated")
})
Kik.on("receivedroster", (groups, friends) => {
console.log(groups);
console.log(friends)
})
groups
: an array of group
objects representing the groups you are in
friends
: an array of user
objects, each representing a friend
Kik.on("receivedcaptcha", (captchaUrl) => {
console.log("Please solve captcha" + captchaUrl)
})
captchaUrl
: url to the captcha page
Kik.on("receivedjidinfo", (users) => {
console.log("We got peer info:");
console.log(users)
})
users
: an array of user
objects returned as a result of requesting jids
Kik.on("receivedgroupmsg", (group, sender, msg) => {
console.log(`Received message from ${sender.jid} in group ${group.jid}`)
})
group
: a group
object representing the group where the message was sent
sender
: a user
object representing the message sender
msg
: the received message
Kik.on("receivedgroupimg", (group, sender, img) => {
console.log(`Received image from ${sender.jid} in group ${group.jid}`)
})
group
: a group
object representing the group where the message was sent
sender
: a user
object representing the message sender
img
: a buffer
object representing the image
Kik.on("grouptyping", (group, sender, isTyping) => {
if(isTyping){
console.log(`${sender.jid} is typing in ${group.jid}`)
}else{
console.log(`${sender.jid} stopped typing in ${group.jid}`)
}
})
group
: a group
object representing the group where the message was sent
sender
: a user
object representing the message sender
isTyping
: true if the user is typing, false if he stopped
Kik.on("userleftgroup", (group, user, kickedBy) => {
console.log(`${user.jid} left the group: ${group.jid}`)
})
group
: a group
object representing the group
user
: WIP
kickedBy
: WIP
Kik.on("userjoinedgroup", (group, user, invitedBy) => {
console.log(`${user.jid} joined the group: ${group.jid}`)
})
group
: a group
object representing the group
user
: WIP
invitedBy
: WIP
Kik.on("receivedprivatemsg", (sender, msg) => {
console.log(`Received message from ${sender.jid}`)
})
sender
: a user
object representing the message sender
msg
: the received message
Kik.on("receivedprivateimg", (sender, img) => {
console.log(`Received image from ${sender.jid}`)
})
sender
: a user
object representing the message sender
img
: a buffer
object representing the image
Kik.on("privatetyping", (sender, isTyping) => {
if(isTyping){
console.log(`${sender.jid} is typing`)
}else{
console.log(`${sender.jid} stopped typing`)
}
})
sender
: a user
object representing the message sender
isTyping
: true if the user is typing, false if he stopped
Note that all callback functions can be excluded
Kik.getRoster((groups, friends) => {
});
This function can be used to search users by username
Kik.getUserInfo(usernamesOrJids, useXiphias, (users) => {
});
usernamesOrJids
: a single username or a single jid string.
Also accepts an array of jid strings or username strings
useXiphias
: if true will use the xiphias endpoint.
This endpoint accepts jids only and returns different data
useXiphias = true | useXiphias = false | |
---|---|---|
username | ❌ | ✔️ |
displayName | ✔️ | ✔️ |
profilePic | ❌ | ✔️ |
backgroundPic | ✔️ | ❌ |
registrationTimestamp | ✔️ | ❌ |
kinId | ✔️ | ❌ |
note that some data will only be returned if you're chatting with a user
returns an array of user
objects,
or an empty array if no results are found
You can provide a group's or a user's jid, they will automatically use the appropriate format
Kik.sendMessage(jid, msg, (delivered, read) => {
if(delivered){
console.log("Delivered")
}else if(read){
console.log("Read")
}
})
You can provide a group's or a user's jid, they will automatically use the appropriate format
Kik.sendImage(jid, imgPath, allowForwarding, allowSaving)
allowForwarding
: boolean, if false this image will not give the
receiver a forwarding option. true by default
allowSaving
: boolean, if false this image will not give the
receiver a download option. true by default
returns a promise, make sure to use this inside an async function with the await keyword
Kik.addFriend(jid)
Kik.removeFriend(jid)
Kik.searchGroups(searchQuery, (groups) => {
})
groups
: an array of group
objects representing the search results,
the group objects here have a special joinToken
variable used for
joining the group
Kik.joinGroup(groupJid, groupCode, joinToken)
Kik.leaveGroup(groupJid)
Kik.setGroupMember(groupJid, userJid, bool)
Kik.setAdmin(groupJid, userJid, bool)
Kik.setBanned(groupJid, userJid, bool)
Kik.setGroupName(groupJid, name)
Kik.setProfileName(firstName, lastName)
Kik.setEmail(newEmail, password)
Kik.setPassword(newPassword, oldPassword)
FAQs
An API for creating kik bots (and other stuff)
The npm package kik-node-api receives a total of 40 weekly downloads. As such, kik-node-api popularity was classified as not popular.
We found that kik-node-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.