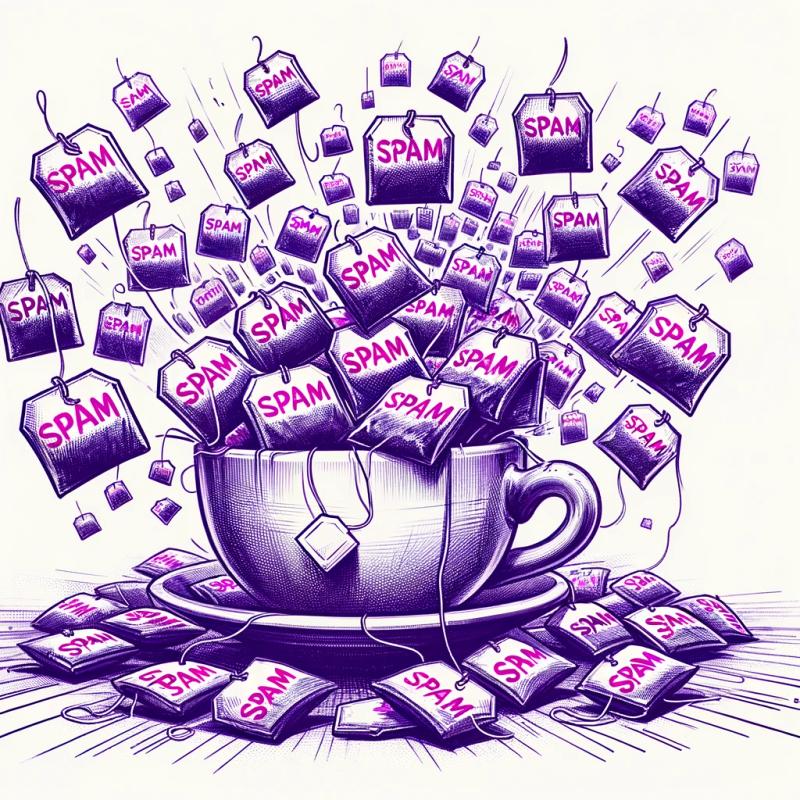
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
koa-mount
Advanced tools
Readme
Mount other Koa applications as middleware. The path
passed to mount()
is stripped
from the URL temporarily until the stack unwinds. This is useful for creating entire
apps or middleware that will function correctly regardless of which path segment(s)
they should operate on.
$ npm install koa-mount
View the ./examples directory for working examples.
Entire applications mounted at specific paths. For example you could mount a blog application at "/blog", with a router that matches paths such as "GET /", "GET /posts", and will behave properly for "GET /blog/posts" etc when mounted.
const mount = require('koa-mount');
const Koa = require('koa');
// hello
const a = new Koa();
a.use(async function (ctx, next){
await next();
ctx.body = 'Hello';
});
// world
const b = new Koa();
b.use(async function (ctx, next){
await next();
ctx.body = 'World';
});
// app
const app = new Koa();
app.use(mount('/hello', a));
app.use(mount('/world', b));
app.listen(3000);
console.log('listening on port 3000');
Try the following requests:
$ GET /
Not Found
$ GET /hello
Hello
$ GET /world
World
Mount middleware at specific paths, allowing them to operate independently of the prefix, as they're not aware of it.
const mount = require('koa-mount');
const Koa = require('koa');
async function hello(ctx, next){
await next();
ctx.body = 'Hello';
}
async function world(ctx, next){
await next();
ctx.body = 'World';
}
const app = new Koa();
app.use(mount('/hello', hello));
app.use(mount('/world', world));
app.listen(3000);
console.log('listening on port 3000');
The path argument is optional, defaulting to "/":
app.use(mount(a));
app.use(mount(b));
Use the DEBUG environement variable to whitelist koa-mount debug output:
$ DEBUG=koa-mount node myapp.js &
$ GET /foo/bar/baz
koa-mount enter /foo/bar/baz -> /bar/baz +2s
koa-mount enter /bar/baz -> /baz +0ms
koa-mount enter /baz -> / +0ms
koa-mount leave /baz -> / +1ms
koa-mount leave /bar/baz -> /baz +0ms
koa-mount leave /foo/bar/baz -> /bar/baz +0ms
MIT
FAQs
Mounting middleware for koa
The npm package koa-mount receives a total of 144,554 weekly downloads. As such, koa-mount popularity was classified as popular.
We found that koa-mount demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.