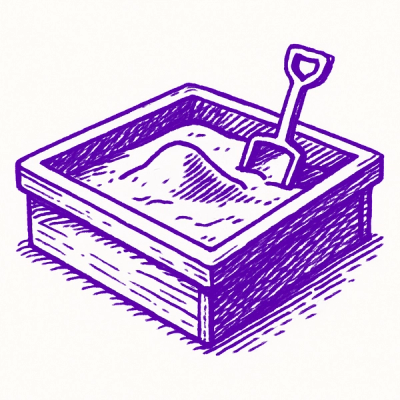
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Redis storage for Koa session middleware/cache with Sentinel and Cluster support
Redis storage for Koa session middleware/cache with Sentinel and Cluster support
v4.0.0+ now uses ioredis
and has support for Sentinel and Cluster!
npm:
npm install koa-redis
yarn:
yarn add koa-redis
koa-redis
works with koa-generic-session (a generic session middleware for koa).
For more examples, please see the examples folder of koa-generic-session
.
const session = require('koa-generic-session');
const redisStore = require('koa-redis');
const koa = require('koa');
const app = koa();
app.keys = ['keys', 'keykeys'];
app.use(session({
store: redisStore({
// Options specified here
})
}));
app.use(function *() {
switch (this.path) {
case '/get':
get.call(this);
break;
case '/remove':
remove.call(this);
break;
case '/regenerate':
yield regenerate.call(this);
break;
}
});
function get() {
const session = this.session;
session.count = session.count || 0;
session.count++;
this.body = session.count;
}
function remove() {
this.session = null;
this.body = 0;
}
function *regenerate() {
get.call(this);
yield this.regenerateSession();
get.call(this);
}
app.listen(8080);
const session = require('koa-generic-session');
const redisStore = require('koa-redis');
const koa = require('koa');
const app = koa();
app.keys = ['keys', 'keykeys'];
app.use(session({
store: redisStore({
// Options specified here
// <https://github.com/luin/ioredis#sentinel>
sentinels: [
{ host: 'localhost', port: 26379 },
{ host: 'localhost', port: 26380 }
// ...
],
name: 'mymaster'
})
}));
// ...
const session = require('koa-generic-session');
const redisStore = require('koa-redis');
const koa = require('koa');
const app = koa();
app.keys = ['keys', 'keykeys'];
app.use(session({
store: redisStore({
// Options specified here
// <https://github.com/luin/ioredis#cluster>
isRedisCluster: true,
nodes: [
{
port: 6380,
host: '127.0.0.1'
},
{
port: 6381,
host: '127.0.0.1'
}
// ...
],
// <https://github.com/luin/ioredis/blob/master/API.md#new-clusterstartupnodes-options>
clusterOptions: {
// ...
redisOptions: {
// ...
}
}
})
}));
// ...
ioredis
options - Useful things include url
, host
, port
, and path
to the server. Defaults to 127.0.0.1:6379
db
(number) - will run client.select(db)
after connectionclient
(object) - supply your own client, all other options are ignored unless duplicate
is also suppliedduplicate
(boolean) - When true, it will run client.duplicate()
on the supplied client
and use all other options supplied. This is useful if you want to select a different DB for sessions but also want to base from the same client object.serialize
- Used to serialize the data that is saved into the store.unserialize
- Used to unserialize the data that is fetched from the store.isRedisCluster
(boolean) - Used for creating a Redis cluster instance per ioredis
Cluster options, if set to true
, then a new Redis cluster will be instantiated with new Redis.Cluster(options.nodes, options.clusterOptions)
(see Cluster docs for more info).nodes
(array) - Conditionally used for creating a Redis cluster instance when isRedisCluster
option is true
, this is the first argument passed to new Redis.Cluster
and contains a list of all the nodes of the cluster ou want to connect to (see Cluster docs for more info).clusterOptions
(object) - Conditionally used for created a Redi cluster instance when isRedisCluster
option is true
, this is the second argument passed to new Redis.Cluster
and contains options, such as redisOptions
(see Cluster docs for more info).auth_pass
and pass
have been replaced with password
, and socket
has been replaced with path
, however all of these options are backwards compatible.See the ioredis
docs for more info.
Note that as of v4.0.0 the disconnect
and warning
events are removed as ioredis
does not support them. The disconnect
event is deprecated, although it is still emitted when end
events are emitted.
These are some the functions that koa-generic-session
uses that you can use manually. You will need to initialize differently than the example above:
const session = require('koa-generic-session');
const redisStore = require('koa-redis')({
// Options specified here
});
const app = require('koa')();
app.keys = ['keys', 'keykeys'];
app.use(session({
store: redisStore
}));
Initialize the Redis connection with the optionally provided options (see above). The variable session
below references this.
Generator that gets a session by ID. Returns parsed JSON is exists, null
if it does not exist, and nothing upon error.
Generator that sets a JSON session by ID with an optional time-to-live (ttl) in milliseconds. Yields ioredis
's client.set()
or client.setex()
.
Generator that destroys a session (removes it from Redis) by ID. Tields ioredis
's client.del()
.
Generator that stops a Redis session after everything in the queue has completed. Yields ioredis
's client.quit()
.
Alias to session.quit()
. It is not safe to use the real end function, as it cuts off the queue.
String giving the connection status updated using client.status
.
Boolean giving the connection status updated using client.status
after any of the events above is fired.
Direct access to the ioredis
client object.
Server | Transaction rate | Response time |
---|---|---|
connect without session | 6763.56 trans/sec | 0.01 secs |
koa without session | 5684.75 trans/sec | 0.01 secs |
connect with session | 2759.70 trans/sec | 0.02 secs |
koa with session | 2355.38 trans/sec | 0.02 secs |
Detailed benchmark report here
localhost:6379
. You can use redis-windows
if you are on Windows or just want a quick VM-based server.npm i
in it (Windows should work fine).DEBUG
flag.npm test
to run the tests and generate coverage. To run the tests without generating coverage, run npm run-script test-only
.MIT © dead_horse
Name | Website |
---|---|
dead_horse | |
Nick Baugh | http://niftylettuce.com/ |
FAQs
Redis storage for Koa session middleware/cache with Sentinel and Cluster support
The npm package koa-redis receives a total of 10,201 weekly downloads. As such, koa-redis popularity was classified as popular.
We found that koa-redis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.