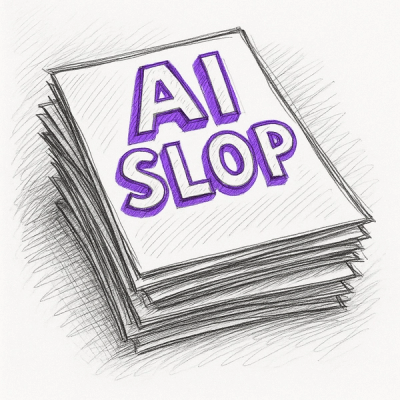
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
lambda-stream
Advanced tools
This library adds types and local support for AWS Lambda Response Streaming. Locally, it'll buffer and return the entire response.
In AWS Lambda, it'll simply use the global awslambda.streamifyResponse
.
This library exposes a ResponseStream
class, the streamifyResponse
method, and isInAWS
method. Types are also included.
Works like this:
import { APIGatewayProxyEvent } from "aws-lambda";
import { streamifyResponse, ResponseStream } from 'lambda-stream'
export const handler = streamifyResponse(myHandler)
async function myHandler (event: APIGatewayProxyEvent, responseStream: ResponseStream): Promise<void> {
console.log('Handler got event:', event)
responseStream.setContentType("text/plain");
responseStream.write("Hello, world!");
responseStream.end();
}
Or in commonjs:
'use strict';
const { streamifyResponse } = require('lambda-stream')
module.exports.hello = streamifyResponse(
async (event, responseStream, context) => {
responseStream.setContentType("text/plain");
responseStream.write("Hello, world!");
responseStream.end();
}
);
Pipelining is also supported:
const pipeline = require("util").promisify(require("stream").pipeline);
const { Readable } = require('stream');
const { streamifyResponse } = require('lambda-stream')
const handler = async (event, responseStream, _context) => {
// As an example, convert event to a readable stream.
requestStream = Readable.from(Buffer.from(JSON.stringify({hello: 'world'})));
await pipeline(requestStream, zlib.createGzip(), responseStream);
}
module.exports.gzip = streamifyResponse(handler)
FAQs
awslambda.streamifyResponse, but locally and with typescript
The npm package lambda-stream receives a total of 30,971 weekly downloads. As such, lambda-stream popularity was classified as popular.
We found that lambda-stream demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.