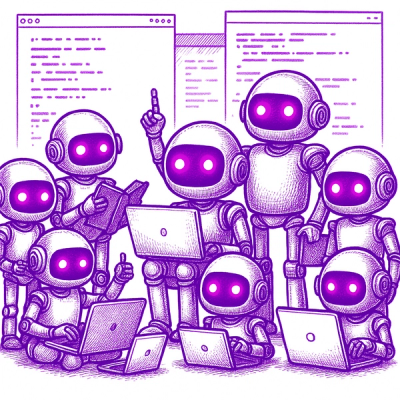
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
level-blobs
Advanced tools
Save binary blobs in level and stream then back. Similar to level-store but streams2 and with support for random access writes and reads
npm install level-blobs
var blobs = require('level-blobs');
var level = require('level');
var db = level('/tmp/my-blobs-db');
var bl = blobs(db);
// create a write stream
var ws = blobs.createWriteStream('my-file.txt');
ws.on('finish', function() {
// lets read the blob and pipe it to stdout
var rs = blobs.createReadStream('my-file.txt');
rs.pipe(process.stdout);
});
ws.write('hello ');
ws.write('world');
ws.end();
blobs(db, opts)
Create a new blobs instance. Options default to
{
blockSize: 65536, // byte size for each block of data stored
batch: 100 // batch at max 100 blocks when writing
}
bl.createReadStream(name, opts)
Create a read stream for name
. Options default to
{
start: 0 // start reading from this byte offset
end: Infinity // end at end-of-file or this offset (inclusive)
}
bl.createWriteStream(name, opts)
Create a write stream to name
. Options default to
{
start: 0 // start writing at this offset
// if append === true start defaults to end-of-file
append: false // set to true if you want to append to the file
// if not true the file will be truncated before writing
}
bl.read(name, opts, cb)
Create a read stream and buffer the stream into a single buffer that is passed to the callback.
Options are passed to createReadStream
.
bl.write(name, data, opts, cb)
Write data
to name
and call the callback when done.
Options are passed to createWriteStream
.
bl.remove(name, cb)
Remove name
from the blob store
MIT
FAQs
Save binary blobs in level and stream then back
The npm package level-blobs receives a total of 139,128 weekly downloads. As such, level-blobs popularity was classified as popular.
We found that level-blobs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.