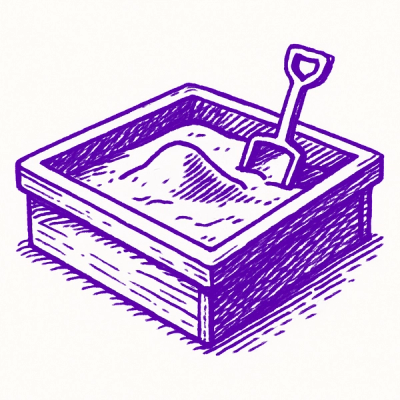
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
lru-memoizer
Advanced tools
The lru-memoizer npm package is a utility for memoizing function results with an LRU (Least Recently Used) cache. This helps in optimizing performance by storing the results of expensive function calls and reusing them when the same inputs occur again, while also managing memory usage by discarding the least recently used items when the cache limit is reached.
Basic Memoization
This feature allows you to memoize a function so that its results are cached. Subsequent calls with the same arguments will return the cached result, improving performance.
const memoizer = require('lru-memoizer');
const slowFunction = (num) => {
// Simulate a slow computation
for (let i = 0; i < 1e6; i++);
return num * 2;
};
const memoizedFunction = memoizer({ load: slowFunction, max: 100 });
console.log(memoizedFunction(5)); // First call, slow
console.log(memoizedFunction(5)); // Second call, fast (cached)
Custom Cache Key
This feature allows you to define a custom cache key for the memoized function. This is useful when the function arguments are complex objects and you want to control how they are hashed for caching.
const memoizer = require('lru-memoizer');
const slowFunction = (obj) => {
// Simulate a slow computation
for (let i = 0; i < 1e6; i++);
return obj.value * 2;
};
const memoizedFunction = memoizer({
load: slowFunction,
max: 100,
hash: (obj) => obj.key // Custom cache key
});
console.log(memoizedFunction({ key: 'a', value: 5 })); // First call, slow
console.log(memoizedFunction({ key: 'a', value: 5 })); // Second call, fast (cached)
Cache Statistics
This feature provides statistics about the cache usage, such as the number of hits, misses, and the current number of keys in the cache. This can be useful for monitoring and debugging.
const memoizer = require('lru-memoizer');
const slowFunction = (num) => {
// Simulate a slow computation
for (let i = 0; i < 1e6; i++);
return num * 2;
};
const memoizedFunction = memoizer({ load: slowFunction, max: 100 });
memoizedFunction(5);
memoizedFunction(5);
console.log(memoizedFunction.cache.stats); // { hits: 1, misses: 1, keys: 1 }
memoizee is a powerful memoization library for JavaScript. It supports multiple cache strategies, including LRU, and offers a wide range of configuration options. Compared to lru-memoizer, memoizee provides more flexibility and features, such as support for asynchronous functions and cache expiration.
lodash.memoize is a memoization function from the popular lodash utility library. It provides basic memoization capabilities with a simple API. While it does not support LRU caching out of the box, it is a lightweight and easy-to-use alternative for basic memoization needs.
fast-memoize is a high-performance memoization library designed for speed. It focuses on providing the fastest possible memoization with a minimal API. Unlike lru-memoizer, it does not support LRU caching, but it is an excellent choice when performance is the primary concern.
Memoize functions results using an lru-cache.
npm i lru-memoizer --save
This module uses an lru-cache internally to cache the results of an async function.
The load
function can have N parameters and the last one must be a callback. The callback should be an errback (first parameter is err
).
The hash
function purpose is generate a custom hash for storing results. It has all the arguments applied to it minus the callback, and must return a synchronous string.
The disable
function allows you to conditionally disable the use of the cache. Useful for test environments.
The freeze
option (defaults to false) allows you to deep-freeze the result of the async function.
The clone
option (defaults to false) allows you to deep-clone the result every time is returned from the cache.
const memoizer = require("lru-memoizer");
const memoizedGet = memoizer({
//defines how to load the resource when
//it is not in the cache.
load: function (options, callback) {
request.get(options, callback);
},
//defines how to create a cache key from the params.
hash: function (options) {
return options.url + qs.stringify(options.qs);
},
//don't cache in test environment
disable: isTestEnv(),
//all other params for the LRU cache.
max: 100,
ttl: 1000 * 60,
});
memoizedGet(
{
url: "https://google.com",
qs: { foo: 123 },
},
function (err, result, body) {
//console.log(body);
}
);
Use memoizer.sync
to cache things that are slow to calculate, methods returning promises, or only if you don't want to use a callback and want it synchronous.
const memoizer = require("lru-memoizer");
const memoizedGet = memoizer.sync({
//defines how to load the resource when
//it is not in the cache.
load: function (params) {
return somethingHardToCompute();
},
//defines how to create a cache key from the params.
hash: function (params) {
return params.foo;
},
//all other params for the LRU cache.
max: 100,
ttl: 1000 * 60,
});
This module is very similar to async-cache(deprecated), the main difference is the hash
function.
MIT 2016 - José F. Romaniello
FAQs
Memoize functions results using an lru-cache.
The npm package lru-memoizer receives a total of 3,593,030 weekly downloads. As such, lru-memoizer popularity was classified as popular.
We found that lru-memoizer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.