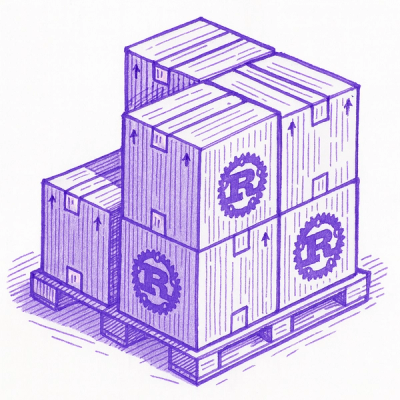
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
lucide-angular
Advanced tools
Lucide Angular package, Lucide is a community-run fork of Feather Icons, open for anyone to contribute icons.
Implementation of the lucide icon library for angular applications.
What is lucide? Read it here.
yarn add lucide-angular
# or
npm install lucide-angular
There are three ways for use this library.
After install lucide-angular
change content of file app.component.html
and app.component.ts
.
<!-- app.component.html -->
<div id="ico"></div>
// app.component.ts
import { Component, OnInit } from '@angular/core';
import { createElement } from 'lucide-angular';
import { Activity } from 'lucide-angular/icons';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
ngOnInit(): void {
const div = document.getElementById('ico');
const elm = createElement(Activity);
elm.setAttribute('color', 'red'); // or set `width`, `height`, `fill`, `stroke-width`, ...
div.appendChild(elm);
}
}
After install lucide-angular
change content of file app.component.html
, app.component.ts
, app.component.css
and app.module.ts
.
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { LucideAngularModule } from 'lucide-angular';
import { AlarmCheck, Edit } from 'lucide-angular/icons'; // or import other icons
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
LucideAngularModule.pick({ AlarmCheck, Edit }) // add all of icons that is imported.
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
/* app.component.css */
.myicon {
/* Be sure to set these values */
width: 48px;
height: 48px;
/* or other properties like `color`, `fill`, `stroke-width` */
}
<!-- app.component.html -->
<lucide-icon name="alarm-check" class="myicon"></lucide-icon>
<lucide-icon name="edit" class="myicon"></lucide-icon>
// app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
}
After install lucide-angular
change content of file app.component.html
, app.component.ts
, app.component.css
and app.module.ts
.
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { LucideAngularModule } from 'lucide-angular';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
LucideAngularModule.pick({ })
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
/* app.component.css */
.myicon {
/* Be sure to set these values */
width: 48px;
height: 48px;
/* or other properties like `color`, `fill`, `stroke-width` */
}
<!-- app.component.html -->
<lucide-icon [img]="ico1" class="myicon"></lucide-icon>
<lucide-icon [img]="ico2" class="myicon"></lucide-icon>
// app.component.ts
import { Component } from '@angular/core';
import { Airplay, Circle } from 'lucide-angular/icons';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
ico1 = Airplay;
ico2 = Circle;
}
In Method 2
: import all icons in app.module.ts
by:
...
import { icons } from 'lucide-angular/icons';
....
LucideAngularModule.pick(icons)
....
You can use the following tags instead of lucide-icon
:
All of the above are the same
FAQs
A Lucide icon library package for Angular applications.
The npm package lucide-angular receives a total of 8,515 weekly downloads. As such, lucide-angular popularity was classified as popular.
We found that lucide-angular demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.