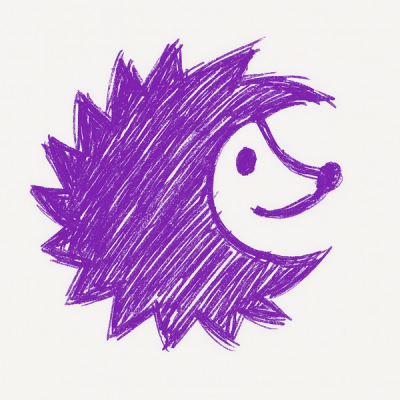
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
mailparser
Advanced tools
The mailparser npm package is a powerful tool for parsing email messages. It can handle various email formats and extract useful information such as headers, attachments, and the email body. This package is particularly useful for applications that need to process and analyze email content programmatically.
Parsing Email from a String
This feature allows you to parse an email from a raw string. The `simpleParser` function takes the raw email string and a callback function, and it returns a parsed email object containing various parts of the email such as headers, text, and attachments.
const { simpleParser } = require('mailparser');
const email = `From: sender@example.com
To: receiver@example.com
Subject: Test email
This is a test email.`;
simpleParser(email, (err, parsed) => {
if (err) {
console.error(err);
} else {
console.log(parsed);
}
});
Parsing Email from a Stream
This feature allows you to parse an email from a stream, such as a file stream. The `simpleParser` function can take a stream as input and parse the email content, making it useful for processing large email files.
const { simpleParser } = require('mailparser');
const fs = require('fs');
const emailStream = fs.createReadStream('path/to/email.eml');
simpleParser(emailStream, (err, parsed) => {
if (err) {
console.error(err);
} else {
console.log(parsed);
}
});
Extracting Attachments
This feature allows you to extract attachments from an email. The parsed email object contains an `attachments` array, where each attachment object includes properties like `filename` and `content`.
const { simpleParser } = require('mailparser');
const email = `From: sender@example.com
To: receiver@example.com
Subject: Test email
This is a test email with an attachment.`;
simpleParser(email, (err, parsed) => {
if (err) {
console.error(err);
} else {
parsed.attachments.forEach(attachment => {
console.log(attachment.filename);
console.log(attachment.content);
});
}
});
Nodemailer is a module for Node.js applications to allow easy email sending. While it focuses on sending emails rather than parsing them, it can be used in conjunction with mailparser for a complete email handling solution.
The imap package is used to connect to and interact with IMAP email servers. It allows you to fetch emails from an IMAP server, which can then be parsed using mailparser. It is more focused on email retrieval rather than parsing.
Mail-listener2 is a library for listening to incoming emails using IMAP. It can be used to monitor an email account and retrieve new emails, which can then be parsed using mailparser. It is useful for real-time email processing.
Advanced email parser for Node.js. Everything is handled as a stream which should make it able to parse even very large messages (100MB+) with relatively low overhead.
mailparser is Node.js only library, so you can't use it reliably in the front-end or bundle with WebPack. If you do need a solution to parse emails in the front-end then use PostalMime.
First install the module from npm:
$ npm install mailparser
next import the mailparser
object into your script:
const mailparser = require('mailparser');
See mailparser homepage for documentation and terms.
Licensed under MIT
FAQs
Parse e-mails
The npm package mailparser receives a total of 1,058,401 weekly downloads. As such, mailparser popularity was classified as popular.
We found that mailparser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.