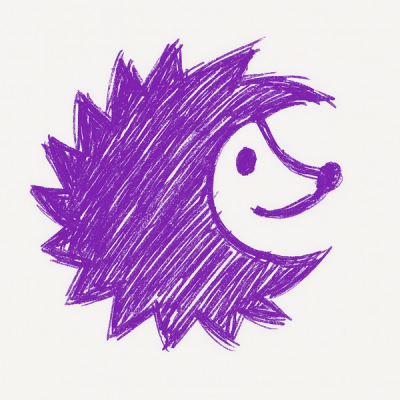
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
markdown-toc
Advanced tools
The markdown-toc package is a tool for generating a table of contents (TOC) for markdown files. It can automatically create a TOC based on the headings in a markdown document, making it easier to navigate and organize content.
Generate TOC from Markdown
This feature allows you to generate a table of contents from a markdown string. The code sample demonstrates how to use the markdown-toc package to create a TOC from a markdown string containing headings.
const toc = require('markdown-toc');
const markdown = '# Title\n## Subtitle\n### Sub-subtitle';
const result = toc(markdown).content;
console.log(result);
Insert TOC into Markdown
This feature allows you to insert a generated TOC directly into a markdown document. The code sample shows how to use the insert method to add a TOC to the markdown content.
const toc = require('markdown-toc');
const markdown = '# Title\n## Subtitle\n### Sub-subtitle';
const result = toc.insert(markdown);
console.log(result);
Customizing TOC
This feature provides options to customize the TOC, such as setting the maximum depth of headings to include. The code sample demonstrates how to generate a TOC with a maximum depth of 2.
const toc = require('markdown-toc');
const markdown = '# Title\n## Subtitle\n### Sub-subtitle';
const result = toc(markdown, { maxdepth: 2 }).content;
console.log(result);
doctoc is a command-line tool that automatically generates a table of contents for markdown files. It is similar to markdown-toc but is more focused on being used as a CLI tool, making it suitable for batch processing of files.
markdown-it-toc-done-right is a plugin for the markdown-it parser that generates a TOC. It is similar to markdown-toc but is designed to work within the markdown-it ecosystem, providing seamless integration with markdown-it's parsing capabilities.
markdown-toc-generator is another package for generating TOCs in markdown files. It offers similar functionality to markdown-toc but with a focus on simplicity and ease of use, making it a good choice for users who need basic TOC generation without additional features.
Generate a markdown TOC (table of contents) with Remarkable.
(TOC generated by verb using markdown-toc)
Install with npm:
$ npm install --save markdown-toc
Usage: markdown-toc [options] <input>
input: The Markdown file to parse for table of contents,
or "-" to read from stdin.
-i: Edit the <input> file directly, injecting the TOC at <!-- toc -->
(Without this flag, the default is to print the TOC to stdout.)
--json: Print the TOC in JSON format
--append: Append a string to the end of the TOC
--bullets: Bullets to use for items in the generated TOC
(Supports multiple bullets: --bullets "*" --bullets "-" --bullets "+")
(Default is "*".)
--maxdepth: Use headings whose depth is at most maxdepth
(Default is 6.)
--no-firsth1: Include the first h1-level heading in a file
--no-stripHeadingTags: Do not strip extraneous HTML tags from heading
text before slugifying
Features
content
), as well as a json
property with the raw TOC object, so you can generate your own TOC using templates or however you wantSafe!
#
)var toc = require('markdown-toc');
toc('# One\n\n# Two').content;
// Results in:
// - [One](#one)
// - [Two](#two)
To allow customization of the output, an object is returned with the following properties:
content
{String}: The generated table of contents. Unless you want to customize rendering, this is all you need.highest
{Number}: The highest level heading found. This is used to adjust indentation.tokens
{Array}: Headings tokens that can be used for custom renderingUse as a remarkable plugin.
var Remarkable = require('remarkable');
var toc = require('markdown-toc');
function render(str, options) {
return new Remarkable()
.use(toc.plugin(options)) // <= register the plugin
.render(str);
}
Usage example
var results = render('# AAA\n# BBB\n# CCC\nfoo\nbar\nbaz');
Results in:
- [AAA](#aaa)
- [BBB](#bbb)
- [CCC](#ccc)
Object for creating a custom TOC.
toc('# AAA\n## BBB\n### CCC\nfoo').json;
// results in
[ { content: 'AAA', slug: 'aaa', lvl: 1 },
{ content: 'BBB', slug: 'bbb', lvl: 2 },
{ content: 'CCC', slug: 'ccc', lvl: 3 } ]
Insert a table of contents immediately after an opening <!-- toc -->
code comment, or replace an existing TOC if both an opening comment and a closing comment (<!-- tocstop -->
) are found.
(This strategy works well since code comments in markdown are hidden when viewed as HTML, like when viewing a README on GitHub README for example).
Example
<!-- toc -->
- old toc 1
- old toc 2
- old toc 3
<!-- tocstop -->
## abc
This is a b c.
## xyz
This is x y z.
Would result in something like:
<!-- toc -->
- [abc](#abc)
- [xyz](#xyz)
<!-- tocstop -->
## abc
This is a b c.
## xyz
This is x y z.
As a convenience to folks who wants to create a custom TOC, markdown-toc's internal utility methods are exposed:
var toc = require('markdown-toc');
toc.bullets()
: render a bullet list from an array of tokenstoc.linkify()
: linking a heading content
stringtoc.slugify()
: slugify a heading content
stringtoc.strip()
: strip words or characters from a heading content
stringExample
var result = toc('# AAA\n## BBB\n### CCC\nfoo');
var str = '';
result.json.forEach(function(heading) {
str += toc.linkify(heading.content);
});
Append a string to the end of the TOC.
toc(str, {append: '\n_(TOC generated by Verb)_'});
Type: Function
Default: undefined
Params:
str
{String} the actual heading stringele
{Objecct} object of heading tokensarr
{Array} all of the headings objectsExample
From time to time, we might get junk like this in our TOC.
[.aaa([foo], ...) another bad heading](#-aaa--foo--------another-bad-heading)
Unless you like that kind of thing, you might want to filter these bad headings out.
function removeJunk(str, ele, arr) {
return str.indexOf('...') === -1;
}
var result = toc(str, {filter: removeJunk});
//=> beautiful TOC
Type: Function
Default: Basic non-word character replacement.
Example
var str = toc('# Some Article', {slugify: require('uslug')});
Type: String|Array
Default: *
The bullet to use for each item in the generated TOC. If passed as an array (['*', '-', '+']
), the bullet point strings will be used based on the header depth.
Type: Number
Default: 6
Use headings whose depth is at most maxdepth.
Type: Boolean
Default: true
Exclude the first h1-level heading in a file. For example, this prevents the first heading in a README from showing up in the TOC.
Type: Boolean
Default: true
Strip extraneous HTML tags from heading text before slugifying. This is similar to GitHub markdown behavior.
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb#dev verb-generate-readme && verb
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
Jon Schlinkert
Copyright © 2017, Jon Schlinkert. Released under the MIT License.
This file was generated by verb-generate-readme, v0.6.0, on September 19, 2017.
FAQs
Generate a markdown TOC (table of contents) with Remarkable.
The npm package markdown-toc receives a total of 184,417 weekly downloads. As such, markdown-toc popularity was classified as popular.
We found that markdown-toc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.