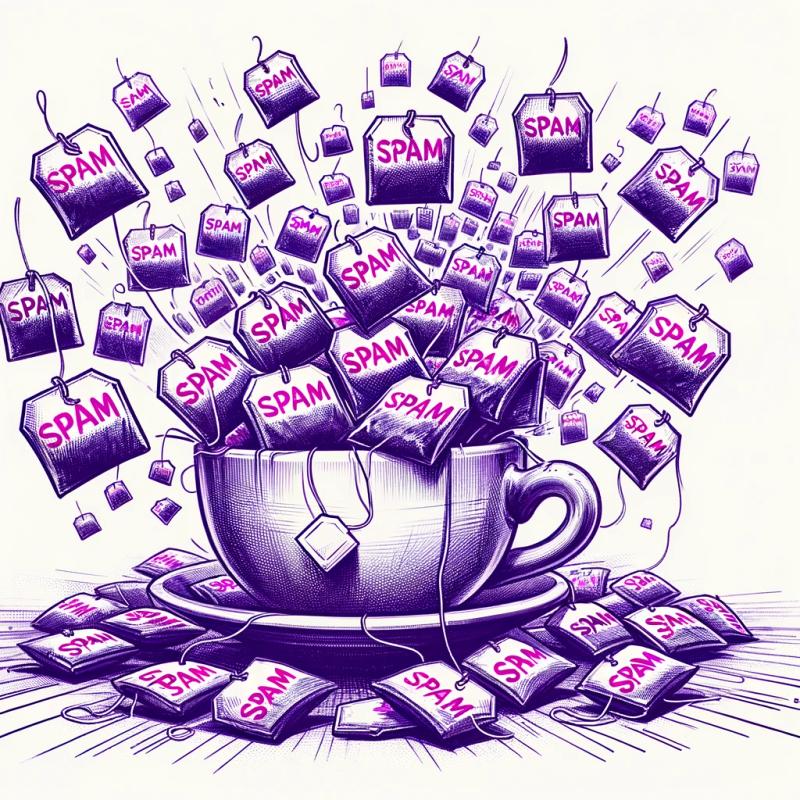
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
material-react-table
Advanced tools
Readme
View Documentation
Want to use Mantine instead of Material UI? Check out Mantine React Table
View additional storybook examples
All features can easily be enabled/disabled
Fully Fleshed out Docs are available for all features
View the full Installation Docs
Ensure that you have React 18 or later installed
Install Peer Dependencies (Material UI V5)
npm install @mui/material @mui/x-date-pickers @mui/icons-material @emotion/react @emotion/styled
npm install material-react-table
@tanstack/react-table
,@tanstack/react-virtual
, and@tanstack/match-sorter-utils
are internal dependencies, so you do NOT need to install them yourself.
Read the full usage docs here
import { useMemo, useState, useEffect } from 'react';
import {
MaterialReactTable,
useMaterialReactTable,
} from 'material-react-table';
//data must be stable reference (useState, useMemo, useQuery, defined outside of component, etc.)
const data = [
{
name: 'John',
age: 30,
},
{
name: 'Sara',
age: 25,
},
];
export default function App() {
const columns = useMemo(
() => [
{
accessorKey: 'name', //simple recommended way to define a column
header: 'Name',
muiTableHeadCellProps: { sx: { color: 'green' } }, //optional custom props
Cell: ({ cell }) => <span>{cell.getValue()}</span>, //optional custom cell render
},
{
accessorFn: (row) => row.age, //alternate way
id: 'age', //id required if you use accessorFn instead of accessorKey
header: 'Age',
Header: () => <i>Age</i>, //optional custom header render
},
],
[],
);
//optionally, you can manage any/all of the table state yourself
const [rowSelection, setRowSelection] = useState({});
useEffect(() => {
//do something when the row selection changes
}, [rowSelection]);
const table = useMaterialReactTable({
columns,
data,
enableColumnOrdering: true, //enable some features
enableRowSelection: true,
enablePagination: false, //disable a default feature
onRowSelectionChange: setRowSelection, //hoist internal state to your own state (optional)
state: { rowSelection }, //manage your own state, pass it back to the table (optional)
});
const someEventHandler = () => {
//read the table state during an event from the table instance
console.log(table.getState().sorting);
};
return (
<MaterialReactTable table={table} /> //other more lightweight MRT sub components also available
);
}
Open in Code Sandbox
PRs are Welcome, but please discuss in GitHub Discussions or the Discord Server first if it is a large change!
Read the Contributing Guide to learn how to run this project locally.
FAQs
A fully featured Material UI V5 implementation of TanStack React Table V8, written from the ground up in TypeScript.
The npm package material-react-table receives a total of 73,026 weekly downloads. As such, material-react-table popularity was classified as popular.
We found that material-react-table demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.