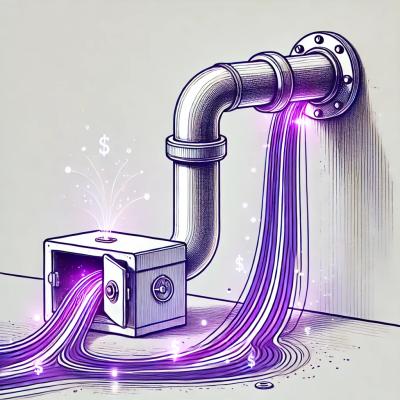
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
memory-cache-node
Advanced tools
Fast, modern and event loop non-blocking memory cache for Node.js and browse
A fast and type safe memory cache for Node.js and browser. memory-cache-node
use Javascript Map as cache implementation which
is faster than using Javascript object that some other similar libraries use. memory-cache-node
also uses implementation that does
not block the event loop for a long time, if the cache is very large (hundreds of thousands or millions of entries).
npm install --save memory-cache-node
Below example creates a memory cache for items which has string keys and number values. Memory cache checks expiring items every 600 seconds (i.e. every 10 minutes) The maximum number of items in the cache is 1 million.
import { MemoryCache } from 'memory-cache-node';
const itemsExpirationCheckIntervalInSecs = 10 * 60;
const maxItemCount = 1000000;
const memoryCache = new MemoryCache<string, number>(itemsExpirationCheckIntervalInSecs, maxItemCount);
Below example stores a permanent item in the memory cache with key key1
and stores an expiring item with key
key2
. The latter item expires earliest after 30 minutes.
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
memoryCache.storePermanentItem('key1', 1);
const timeToLiveInSecs = 30 * 60;
memoryCache.storeExpiringItem('key2', 2, timeToLiveInSecs);
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
memoryCache.storePermanentItem('key1', 1);
const timeToLiveInSecs = 30 * 60;
memoryCache.storeExpiringItem('key2', 2, timeToLiveInSecs);
console.log(memoryCache.getItemCount()); // Logs to console: 2
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
memoryCache.storePermanentItem('key1', 1);
console.log(memoryCache.hasItem('key1')); // Logs to console: true
console.log(memoryCache.hasItem('notFound')); // Logs to console: false
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
memoryCache.storePermanentItem('key1', 1);
console.log(memoryCache.retrieveItemValue('key1')); // Logs to console: 1
console.log(memoryCache.retrieveItemValue('notFound')); // Logs to console: undefined
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
memoryCache.storePermanentItem('key1', 1);
console.log(memoryCache.getItemExpirationTimestampInMillisSinceEpoch('key1')); // Logs some large number to console
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
memoryCache.storePermanentItem('key1', 1);
console.log(memoryCache.hasItem('key1')); // Logs to console: true
memoryCache.removeItem('key1');
console.log(memoryCache.hasItem('key1')); // Logs to console: false
Below examples removes all items from the memory cache
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
// Use cache here
// ...
memoryCache.clear()
Below example destroys the memory cache and it should not be used after that. It clears the memory cache and also removes the timer for checking expired items. NOTE! You should NEVER use a destroyed cache again! If you try to use a destroyed memory cache, an exception will be thrown. You should destroy your memory cache if it is not used in your application anymore.
import { MemoryCache } from 'memory-cache-node';
const memoryCache = new MemoryCache<string, number>(600, 1000000);
// Use cache here
// ...
memoryCache.destroy()
`K` is the type of the item key.
`V` is the type of the item value.
class MemoryCache<K, V> {
constructor(itemsExpirationCheckIntervalInSecs: number, maxItemCount: number);
storePermanentItem(itemKey: K, itemValue: V): void;
storeExpiringItem(itemKey: K, itemValue: V, timeToLiveInSecs: number): void;
getItemCount(): number;
hasItem(itemKey: K): boolean;
getValues(): V[];
getItems(): [K, V][];
retrieveItemValue(itemKey: K): V | undefined;
getItemExpirationTimestampInMillisSinceEpoch(itemKey: K): number | undefined;
removeItem(itemKey: K): void;
exportItemsToJson(): string;
// Use below function only with JSON output from exportItemsToJson method
importItemsFrom(json: string): void; // Can throw if JSON is invalid
clear(): void;
destroy(): void;
}
FAQs
Fast, modern and event loop non-blocking memory cache for Node.js and browse
The npm package memory-cache-node receives a total of 4,866 weekly downloads. As such, memory-cache-node popularity was classified as popular.
We found that memory-cache-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.