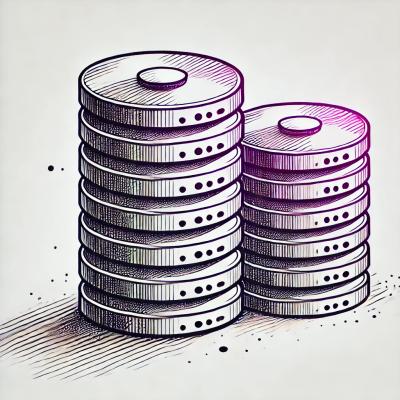
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
memorystream
Advanced tools
The memorystream npm package is a simple stream implementation that can be used to store data in memory. It is useful for testing or for small data manipulation tasks where you want to use streams but don't need to persist the data to disk or send it over a network.
Writable and Readable Stream
This feature allows the memorystream to act both as a writable and readable stream. Data can be written to the stream and then piped elsewhere, such as to stdout, or read directly.
const MemoryStream = require('memorystream');
const memStream = new MemoryStream();
memStream.write('Data to store in memory stream');
memStream.pipe(process.stdout);
Data Capture for Testing
Memorystream can be used to capture output in testing environments. By writing to the stream and then converting it to a string, you can easily assert the output of the stream.
const MemoryStream = require('memorystream');
const memStream = new MemoryStream();
memStream.write('Test data');
memStream.end();
console.log(memStream.toString());
The 'stream-buffers' npm package offers similar functionality to 'memorystream' by providing in-memory storage for streams. It differs by offering more detailed control over the size and growth of the internal buffer.
Similar to 'memorystream', 'memory-streams' provides classes for readable and writable memory streams. It is often used for testing purposes like 'memorystream', but it provides additional features such as high-water mark management.
node-memorystream - this module allow create streams in memory. It can be used for emulating file streams, filtering/mutating data between one stream and another, buffering incoming data, being the gap between two data/network streams of variable rates, etc. MemoryStream support read/write states or only read state or only write state. The API is meant to follow node's Stream implementation. Module supports streams for node > 0.10 now.
Original module is here git://github.com/ollym/memstream.git was remade and improved.
If you have npm installed, you can simply type:
npm install memorystream
Or you can clone this repository using the git command:
git clone git://github.com/JSBizon/node-memorystream.git
Some examples how to use memorystream module.
In this example I illustrate the basic I/O operations of the memory stream.
var MemoryStream = require('memorystream');
var memStream = new MemoryStream(['Hello',' ']);
var data = '';
memStream.on('data', function(chunk) {
data += chunk.toString();
});
memStream.write('World');
memStream.on('end', function() {
// outputs 'Hello World!'
console.log(data);
});
memStream.end('!');
In this example I'm piping all data from the memory stream to the process's stdout stream.
var MemoryStream = require('memorystream');
var memStream = new MemoryStream();
memStream.pipe(process.stdout, { end: false });
memStream.write('Hello World!');
In this example I'm piping all data from the response stream to the memory stream.
var http = require('http'),
MemoryStream = require('memorystream');
var options = {
host: 'google.com'
};
var memStream = new MemoryStream(null, {
readable : false
});
var req = http.get(options, function(res) {
res.pipe(memStream);
res.on('end', function() {
console.log(memStream.toString());
});
});
In the example below, we first pause the stream before writing the data to it. The stream is then resumed after 1 second, and the data is written to the console.
var MemoryStream = require('memorystream');
var memStream = new MemoryStream('Hello');
var data = '';
memStream.on('data', function(chunk) {
data += chunk;
});
memStream.pause();
memStream.write('World!');
setTimeout(function() {
memStream.resume();
}, 1000);
The memory stream adopts all the same methods and events as node's Stream implementation. Documentation is available here.
FAQs
This is lightweight memory stream module for node.js.
The npm package memorystream receives a total of 3,512,470 weekly downloads. As such, memorystream popularity was classified as popular.
We found that memorystream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.