mercadopago
Advanced tools
Comparing version 1.5.17 to 2.0.0
{ | ||
"name": "mercadopago", | ||
"version": "1.5.17", | ||
"version": "2.0.0", | ||
"description": "Mercadopago SDK for Node.js", | ||
"main": "index.js", | ||
"files": [ | ||
"lib/" | ||
], | ||
"main": "dist/index.js", | ||
"types": "dist/index.d.ts", | ||
"scripts": { | ||
"doc": "jsdoc -c jsdoc.json", | ||
"lint": "NODE_ENV=test eslint lib/*.js", | ||
"coverage": "NODE_ENV=test nyc ./node_modules/mocha/bin/_mocha ./test/*.*", | ||
"coveralls": "npm run coverage -- --report lcovonly && cat ./coverage/lcov.info | coveralls", | ||
"test": "NODE_ENV=test mocha ./test/*.*" | ||
"build": "rm -rf dist && tsc --build tsconfig.production.json && tsc-alias -p tsconfig.production.json", | ||
"test": "jest", | ||
"test:e2e": "jest --config e2e/jest.config.ts", | ||
"lint": "eslint 'src/**/*.{ts,tsx}' 'src/examples/**/*.{ts,tsx}' --cache", | ||
"lint:fix": "eslint --fix --ext .ts src src/examples", | ||
"exec": "node_modules/.bin/ts-node -r tsconfig-paths/register", | ||
"prepare": "husky install" | ||
}, | ||
@@ -29,3 +29,3 @@ "repository": { | ||
], | ||
"author": "Ariel Rey <ariel.rey@mercadolibre.com>", | ||
"author": "Mercado Pago (https://www.mercadopago.com/developers/en)", | ||
"bugs": { | ||
@@ -36,22 +36,24 @@ "url": "https://github.com/mercadopago/sdk-nodejs/issues" | ||
"devDependencies": { | ||
"chai": "3.5.0", | ||
"chai-as-promised": "6.0.0", | ||
"coveralls": "^3.1.0", | ||
"eslint": ">=4.18.2", | ||
"eslint-config-airbnb-base": "11.0.1", | ||
"eslint-plugin-import": "2.2.0", | ||
"jsdoc": "^4.0.2", | ||
"mocha": "^9.1.3", | ||
"mocha-lcov-reporter": "1.2.0", | ||
"nyc": "^15.1.0", | ||
"sinon": "1.17.7" | ||
"@types/jest": "^29.5.3", | ||
"@types/node": "^20.4.8", | ||
"@types/node-fetch": "^2.6.4", | ||
"@types/uuid": "^9.0.3", | ||
"@typescript-eslint/eslint-plugin": "^6.3.0", | ||
"@typescript-eslint/parser": "^6.3.0", | ||
"eslint": "^8.46.0", | ||
"husky": "^8.0.0", | ||
"jest": "^29.6.2", | ||
"ts-jest": "^29.1.1", | ||
"ts-node": "^10.9.1", | ||
"tsc-alias": "^1.8.7", | ||
"tsconfig-paths": "^4.2.0", | ||
"typescript": "^5.1.6" | ||
}, | ||
"files": [ | ||
"dist" | ||
], | ||
"dependencies": { | ||
"ajv": "^6.12.3", | ||
"bluebird": "3.4.7", | ||
"dayjs": "^1.11.7", | ||
"request": "^2.88.0", | ||
"request-etag": "2.0.3", | ||
"uuid": "3.0.1" | ||
"node-fetch": "^2.6.12", | ||
"uuid": "^9.0.0" | ||
} | ||
} |
110
README.md
@@ -0,1 +1,4 @@ | ||
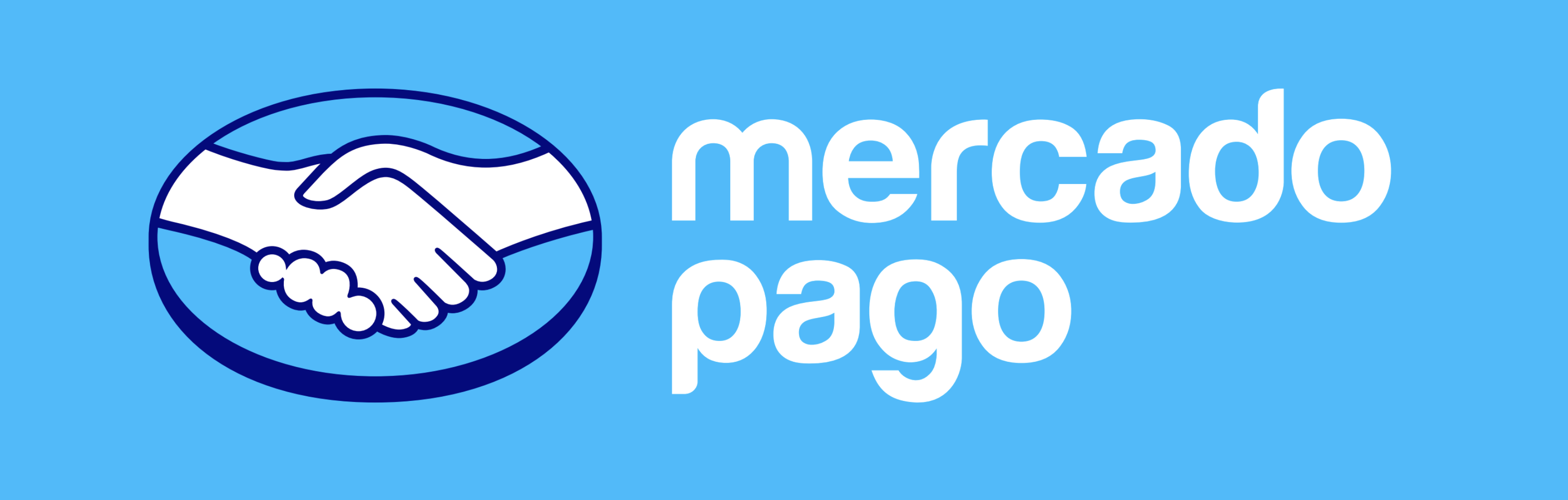 | ||
# Mercado Pago SDK for NodeJS | ||
@@ -11,3 +14,3 @@ | ||
The SDK Supports Node.js v10 or higher. | ||
The SDK Supports NodeJS version 12 or higher. | ||
@@ -23,3 +26,3 @@ ## π² Installation | ||
2. Copy the access_token in the [credentials](https://www.mercadopago.com/mlb/account/credentials) section of the page and replace YOUR_ACCESS_TOKEN with it. | ||
2. Copy the access_token in the [credentials](https://www.mercadopago.com/developers/en/docs/your-integrations/credentials) section of the page and replace YOUR_ACCESS_TOKEN with it. | ||
@@ -33,21 +36,78 @@ That's it! Mercado Pago SDK has been successfully installed. | ||
```javascript | ||
var mercadopago = require('mercadopago'); | ||
mercadopago.configure({ | ||
access_token: 'YOUR_ACCESS_TOKEN' | ||
}); | ||
// Step 1: Import the parts of the module you want to use | ||
import MercadoPago, { Payment } from 'mercadopago'; | ||
var preference = { | ||
items: [ | ||
{ | ||
title: 'Test', | ||
quantity: 1, | ||
currency_id: 'ARS', | ||
unit_price: 10.5 | ||
} | ||
] | ||
// Step 2: Initialize the client object | ||
const client = new MercadoPago({ accessToken: 'access_token', options: { timeout: 5000, idempotencyKey: 'abc' } }); | ||
// Step 3: Initialize the API object | ||
const payment = new Payment(client); | ||
// Step 4: Create the request object | ||
const body = { | ||
transaction_amount: 12.34, | ||
description: '<DESCRIPTION>', | ||
payment_method_id: '<PAYMENT_METHOD_ID>', | ||
payer: { | ||
email: '<EMAIL>' | ||
}, | ||
}; | ||
mercadopago.preferences.create(preference) | ||
// Step 5: Make the request | ||
payment.create({ body }).then(console.log).catch(console.log); | ||
``` | ||
### Step 1: Import the parts of the module you want to use | ||
Import `MercadoPago` and API objects from the MercadoPago module. | ||
``` javascript | ||
import MercadoPago, { Payment } from 'mercadopago'; | ||
``` | ||
### Step 2: Initialize the client object | ||
Initialize the client object, passing the following: | ||
- `accessToken`: Application's private key. | ||
- `options`: These are optional fields, | ||
- `timeout`: Are the timeout of requests | ||
- `idempotencyKey`: [Idempotency](https://en.wikipedia.org/wiki/Idempotence) Is for retrying requests without accidentally performing the same operation twice | ||
For example: | ||
``` javascript | ||
const client = new MercadoPago({ accessToken: 'access_token', options: { timeout: 5000, idempotencyKey: 'abc' } }); | ||
``` | ||
### Step 3: Initialize the API object | ||
Initialize the API object you want to use, passing the `client` object from the previous step. | ||
``` javascript | ||
const payment = new Payment(client); | ||
``` | ||
### Step 4: Create the request object | ||
Create a the request object. For example, for a request to the `/v1/payments` endpoint: | ||
``` javascript | ||
const body = { | ||
transaction_amount: 12.34, | ||
description: '<DESCRIPTION>', | ||
payment_method_id: '<PAYMENT_METHOD_ID>', | ||
payer: { | ||
email: '<EMAIL>' | ||
}, | ||
}; | ||
``` | ||
### Step 5: Make the request | ||
Use the API object's method to make the request. For example, to make a request to the `/v1/payments` endpoint using the `payment` object: | ||
``` | ||
payment.create({ body }).then(console.log).catch(console.log); | ||
``` | ||
## π Documentation | ||
@@ -58,9 +118,21 @@ | ||
- Mercado Pago checkout: [Spanish](https://www.mercadopago.com.ar/developers/es/guides/payments/web-payment-checkout/introduction/) / [Portuguese](https://www.mercadopago.com.br/developers/pt/guides/payments/web-payment-checkout/introduction/) | ||
- Web Tokenize checkout: [Spanish](https://www.mercadopago.com.ar/developers/es/guides/payments/web-tokenize-checkout/introduction/) / [Portuguese](https://www.mercadopago.com.br/developers/pt/guides/payments/web-tokenize-checkout/introduction/) | ||
Check our [official code reference](https://mercadopago.github.io/sdk-nodejs/) to explore all available functionalities. | ||
## π€ Contributing | ||
All contributions are welcome, ranging from people wanting to triage issues, others wanting to write documentation, to people wanting to contribute with code. | ||
Please read and follow our [contribution guidelines](CONTRIBUTING.md). Contributions not following these guidelines will be disregarded. The guidelines are in place to make all of our lives easier and make contribution a consistent process for everyone. | ||
### Patches to version 1.x.x | ||
Since the release of version 2.0.0, version 1 is deprecated and will not be receiving new features, only bug fixes. If you need to submit PRs for that version, please do so by using develop-v1 as your base branch. | ||
## β€οΈ Support | ||
If you require technical support, please contact our support team at [developers.mercadopago.com](https://developers.mercadopago.com) | ||
If you require technical support, please contact our support team at our developers | ||
site: [English](https://www.mercadopago.com/developers/en/support/center/contact) | ||
/ [Portuguese](https://www.mercadopago.com/developers/pt/support/center/contact) | ||
/ [Spanish](https://www.mercadopago.com/developers/es/support/center/contact) | ||
@@ -70,4 +142,4 @@ ## π» License | ||
``` | ||
MIT license. Copyright (c) 2021 - Mercado Pago / Mercado Libre | ||
MIT license. Copyright (c) 2023 - Mercado Pago / Mercado Libre | ||
For more information, see the LICENSE file. | ||
``` |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
178025
2
274
4383
141
0
14
2
+ Addednode-fetch@^2.6.12
+ Addednode-fetch@2.7.0(transitive)
+ Addedtr46@0.0.3(transitive)
+ Addeduuid@9.0.1(transitive)
+ Addedwebidl-conversions@3.0.1(transitive)
+ Addedwhatwg-url@5.0.0(transitive)
- Removedajv@^6.12.3
- Removedbluebird@3.4.7
- Removeddayjs@^1.11.7
- Removedrequest@^2.88.0
- Removedrequest-etag@2.0.3
- Removedajv@6.12.6(transitive)
- Removedasn1@0.2.6(transitive)
- Removedassert-plus@1.0.0(transitive)
- Removedasynckit@0.4.0(transitive)
- Removedaws-sign2@0.7.0(transitive)
- Removedaws4@1.12.0(transitive)
- Removedbcrypt-pbkdf@1.0.2(transitive)
- Removedbluebird@3.4.7(transitive)
- Removedcaseless@0.12.0(transitive)
- Removedcombined-stream@1.0.8(transitive)
- Removedcore-util-is@1.0.2(transitive)
- Removeddashdash@1.14.1(transitive)
- Removeddayjs@1.11.11(transitive)
- Removeddelayed-stream@1.0.0(transitive)
- Removedecc-jsbn@0.1.2(transitive)
- Removedextend@3.0.2(transitive)
- Removedextsprintf@1.3.0(transitive)
- Removedfast-deep-equal@3.1.3(transitive)
- Removedfast-json-stable-stringify@2.1.0(transitive)
- Removedforever-agent@0.6.1(transitive)
- Removedform-data@2.3.3(transitive)
- Removedgetpass@0.1.7(transitive)
- Removedhar-schema@2.0.0(transitive)
- Removedhar-validator@5.1.5(transitive)
- Removedhttp-signature@1.2.0(transitive)
- Removedis-typedarray@1.0.0(transitive)
- Removedisstream@0.1.2(transitive)
- Removedjsbn@0.1.1(transitive)
- Removedjson-schema@0.4.0(transitive)
- Removedjson-schema-traverse@0.4.1(transitive)
- Removedjson-stringify-safe@5.0.1(transitive)
- Removedjsprim@1.4.2(transitive)
- Removedlodash.assign@4.2.0(transitive)
- Removedlodash.clonedeep@4.5.0(transitive)
- Removedlru-cache@4.1.5(transitive)
- Removedmime-db@1.52.0(transitive)
- Removedmime-types@2.1.35(transitive)
- Removedoauth-sign@0.9.0(transitive)
- Removedperformance-now@2.1.0(transitive)
- Removedpseudomap@1.0.2(transitive)
- Removedpsl@1.9.0(transitive)
- Removedpunycode@2.3.1(transitive)
- Removedqs@6.5.3(transitive)
- Removedrequest@2.88.2(transitive)
- Removedrequest-etag@2.0.3(transitive)
- Removedsafe-buffer@5.2.1(transitive)
- Removedsafer-buffer@2.1.2(transitive)
- Removedsshpk@1.18.0(transitive)
- Removedtough-cookie@2.5.0(transitive)
- Removedtunnel-agent@0.6.0(transitive)
- Removedtweetnacl@0.14.5(transitive)
- Removeduri-js@4.4.1(transitive)
- Removeduuid@3.0.13.4.0(transitive)
- Removedverror@1.10.0(transitive)
- Removedyallist@2.1.2(transitive)
Updateduuid@^9.0.0