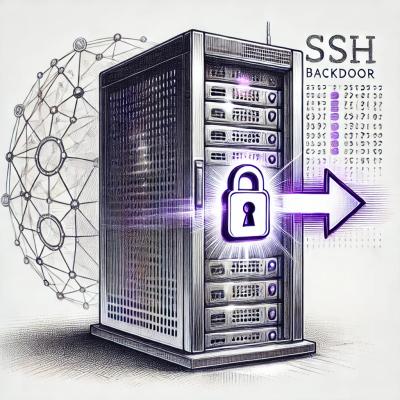
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
meta-whatsapp-cloud-api-sdk
Advanced tools
This is the SDK for simplifying messaging and webhooks for meta whatsapp cloud api.
This Node.js SDK provides functionalities to interact with the WhatsApp Cloud Business API, including sending templates, messages, attachments, uploading media, and verifying webhooks.
npm i meta-whatsapp-cloud-api-sdk
const Whatsapp = require('meta-whatsapp-cloud-api-sdk');
// Provide Phone Number ID, Access Token, Webhook Verify Token, and Meta App Version.
const phoneNumberId = "your_phone_number_id";
const accessToken = "your_access_token";
const appVersion = "your_app_version"; // Optional, Default version is v19.0.
const accountId = "your_account_id"; // Optional, Add this if you wish to use Template Management.
const webhookVerifyToken = "your_webhook_verify_token"; // Optional, Add this if you wish to use Webhook.
const whatsapp = new Whatsapp(phoneNumberId, accessToken, appVersion, accountId, webhookVerifyToken);
// Example header
const header = [
{
type: "image",
image: {
link: "URL"
}
},
{
type: "location",
location: {
latitude: "<LATITUDE>",
longitude: "<LONGITUDE>",
name: "<NAME>",
address: "<ADDRESS>"
}
}
];
// Example body
const body = [
{
type: "text",
text: "TEXT-STRING"
},
{
type: "currency",
currency: {
fallback_value: "VALUE",
code: "USD",
amount_1000: NUMBER
}
},
{
type: "date_time",
date_time: {
fallback_value: "MONTH DAY, YEAR"
}
}
];
// Example buttons
const buttons = [
{
type: "button",
sub_type: "quick_reply",
index: "0",
parameters: [
{
type: "payload",
payload: "PAYLOAD"
}
]
},
{
type: "button",
sub_type: "quick_reply",
index: "1",
parameters: [
{
type: "payload",
payload: "PAYLOAD"
}
]
}
];
// Send a template message (async/await) - header, body and buttons parameters are optional
const response = await whatsapp.sendTemplate(to, templateName, language, header, body, buttons);
Or
// Send a template message (promise) - header, body and buttons parameters are optional
whatsapp.sendTemplate(to, templateName, language, header, body, buttons)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
// Send a text message (async/await)
const response = await whatsapp.sendMessage(to, message);
Or
// Send a text message (promise)
whatsapp.sendMessage(to, message)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
// Upload media (async/await)
const media = await whatsapp.uploadMedia(file);
// Send an image message (async/await) - 2nd Parameter is Media Id or Media Link
const response = await whatsapp.sendImage(to, media.id, caption);
Or
// Upload media (promise)
whatsapp.uploadMedia(file)
.then((media)=>{
// Send an image message (promise) - 2nd Parameter is Media Id or Media Link
whatsapp.sendImage(to, media.id, caption)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
}).catch((error)=>{
console.log(error);
});
// Upload media (async/await)
const media = await whatsapp.uploadMedia(file);
// Send a video message (async/await) - 2nd Parameter is Media Id or Media Link
const response = await whatsapp.sendVideo(to, media.id, caption);
Or
// Upload media (promise)
whatsapp.uploadMedia(file)
.then((media)=>{
// Send a video message (promise) - 2nd Parameter is Media Id or Media Link
whatsapp.sendVideo(to, media.id, caption)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
}).catch((error)=>{
console.log(error);
});
// Upload media (async/await)
const media = await whatsapp.uploadMedia(file);
// Send an audio message (async/await) - 2nd Parameter is Media Id or Media Link
const response = await whatsapp.sendAudio(to, media.id);
Or
// Upload media (promise)
whatsapp.uploadMedia(file)
.then((media)=>{
// Send an audio message (promise) - 2nd Parameter is Media Id or Media Link
whatsapp.sendAudio(to, media.id)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
}).catch((error)=>{
console.log(error);
});
// Upload media (async/await)
const media = await whatsapp.uploadMedia(file);
// Send a document message (async/await) - 2nd Parameter is Media Id or Media Link
const response = await whatsapp.sendDocument(to, media.id, caption, filename);
Or
// Upload media (promise)
whatsapp.uploadMedia(file)
.then((media)=>{
// Send a document message (promise) - 2nd Parameter is Media Id or Media Link
whatsapp.sendDocument(to, media.id, caption, filename)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
}).catch((error)=>{
console.log(error);
});
// Download media (async/await)
const response = await whatsapp.downloadMedia(mediaId);
Or
// Download media (promise)
whatsapp.downloadMedia(mediaId)
.then((response)=>{
console.log(response);
}).catch((error)=>{
console.log(error);
});
// Verify webhook endpoint (Express.js example)
app.get("/webhook", whatsapp.verifyWebhook);
// Register template
const response = await whatsapp.registerTemplate(name, components, category, allowCategoryChange, language);
// Get templates
const response = await whatsapp.getTemplates(query, fields, limit);
// Get template Info
const response = await whatsapp.getTemplateInfo(templateId);
// Update template
const response = await whatsapp.updateTemplate(templateId, category, components);
// Delete template
const response = await whatsapp.deleteTemplate(name, templateId);
// Validate contacts
const response = await whatsapp.validateContacts(contacts, blocking, forceCheck);
FAQs
This is the SDK for simplifying messaging and webhooks for meta whatsapp cloud api.
The npm package meta-whatsapp-cloud-api-sdk receives a total of 0 weekly downloads. As such, meta-whatsapp-cloud-api-sdk popularity was classified as not popular.
We found that meta-whatsapp-cloud-api-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.