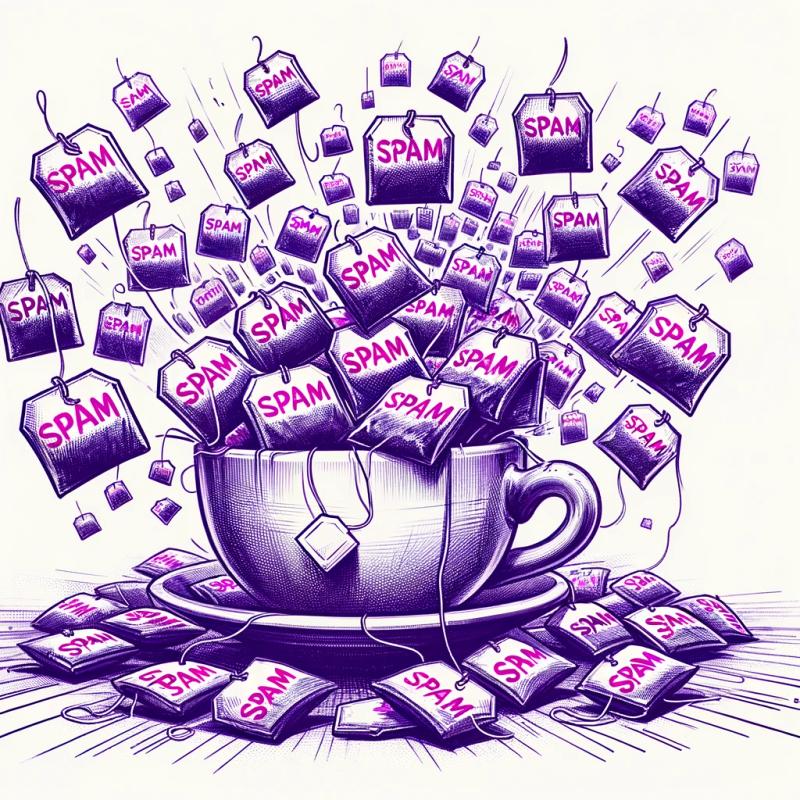
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
MFdb is a portable P2P database backed by the IPFS Mutable File System.
Create large searchable datasets and easily share them with others. Works in Node and the browser.
Create a database locally and store it in IPFS. Then share the hash with others. They load it and can instantly search it after downloading. Since each commit is written to MFS you can also load a database at each intermediate state if you have the CID. Broadcast these changes and mirrored copies can stay up to date in real time. All merging happens at the Mutable File System level.
Query data by primary and non-primary indexes. Each table has a schema and when records are inserted we build btree indexes. B-trees are loaded into memory when fields are searched.
The entire database is stored in an IPFS Mutable File System folder and can be exchanged with a single hash. Broadcast the hash via IPNS and any peers can sync up to the latest version. The sync speed depends on many different factors but should be faster the more peers you have.
npm install ipfs mfdb
First initialize MFdb with IPFS.
import assert from 'assert'
import { MFdb } from "mfdb"
const ipfs = await IPFS.create()
const db = new MFdb(ipfs)
Create a database definition and use it to create the database. In this example we're saving baseball players.
We'll add a single table with 4 different indexed fields. Indexes can be unique. A table must have exactly 1 primary key field defined.
let definition: DatabaseDefinition = {
name: "test",
tables: [
{
name: "player",
columns: [
{ name: "id", unique: true, primary: true },
{ name: "name", unique: false },
{ name: "currentTeam", unique: false },
{ name: "battingHand", unique: false },
{ name: "throwingHand", unique: false }
]
}
]
}
await db.createDatabase(definition)
Use the database
await db.useDatabase("my-database")
Add a record and retreive it by the primary key. Note: The primary key must be a string.
//Start transaction
db.startTransaction()
//Save it
db.insert("player", {
id: '101',
name: "Andrew McCutchen",
currentTeam: "PIT",
battingHand: "R",
throwingHand: "R"
})
//Commit changes
await db.commit()
//Retrieve it
let player = await store.get("player", '101')
Now we're going to add a few more players
//Start transaction
db.startTransaction()
store.insert("player", {
id: '102',
name: "Pedro Alvarez",
currentTeam: "BAL",
battingHand: "R",
throwingHand: "R"
})
store.insert("player", {
id: '103',
name: "Jordy Mercer",
currentTeam: "PIT",
battingHand: "L",
throwingHand: "R"
})
store.insert("player", {
id: '104',
name: "Doug Drabek",
currentTeam: "BAL",
battingHand: "L",
throwingHand: "R"
})
//Commit changes
await db.commit()
Now retreive the values by the secondary indexes.
//Get players who play for PIT
let teamPIT = await store.searchIndex({
table: "players",
index: "currentTeam",
value: "PIT",
sortDirection: "desc",
offset: 0,
limit: 100
})
//Get players who who bat right handed.
let battingR = await store.searchIndex({
table: "players",
index: "battingHand",
value: "R",
sortDirection: "desc",
offset: 0,
limit: 100
})
Update a player with new info
db.startTransaction()
db.update("test-table", {
id: '101',
name: "Andrew McCutchen",
currentTeam: "PIT",
battingHand: "L", //was R
throwingHand: "R"
})
await db.commit()
Delete a row
db.startTransaction()
db.delete("test-table", '103')
await db.commit()
Get the CID of the database.
let cid = await db.getDatabaseCid('players')
Load database from CID.
await db.updateFromCid("test", "QmSRtaj7Vu8Q4YhcU32xaiHvmKR4KD5h7WR27azzeECCki")
Examples can be found in the tests.
MFdb exposes the following functions:
Search request is an object with the following fields:
interface IndexSearch {
table: string,
index:string,
value?:any,
sortDirection:SortDirection
offset: number
limit: number
}
//Example:
//Get players who who bat right handed.
let battingR = await store.searchIndex({
table: "players",
index: "battingHand",
value: "R",
sortDirection: "desc",
offset: 0,
limit: 100
})
interface DatabaseDefinition {
name:string
tables:TableDefinition[]
}
interface TableDefinition {
name: string
columns: ColumnDefinition[]
}
interface ColumnDefinition {
name: string
primary?: boolean
unique: boolean
}
FAQs
MFdb is a portable P2P database backed by the IPFS Mutable File System.
The npm package mfdb receives a total of 2 weekly downloads. As such, mfdb popularity was classified as not popular.
We found that mfdb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.