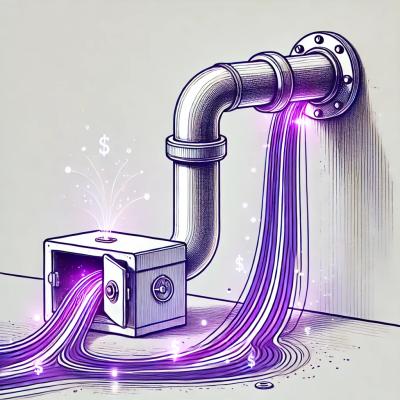
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
mock-socket
Advanced tools
The mock-socket npm package is designed to mock WebSocket and Server-Sent Events (SSE) in JavaScript applications. It is particularly useful for testing and development purposes, allowing developers to simulate WebSocket connections and SSE without needing a real server.
Mock WebSocket
This feature allows you to create a mock WebSocket server and client. The server listens for connections and messages, and the client can send and receive messages. This is useful for testing WebSocket interactions without needing a real server.
const { WebSocket, Server } = require('mock-socket');
const mockServer = new Server('ws://localhost:8080');
mockServer.on('connection', socket => {
socket.on('message', data => {
console.log('Received message from client:', data);
socket.send('Hello from server');
});
});
const client = new WebSocket('ws://localhost:8080');
client.onopen = () => {
client.send('Hello from client');
};
client.onmessage = event => {
console.log('Received message from server:', event.data);
};
Mock Server-Sent Events (SSE)
This feature allows you to create a mock Server-Sent Events (SSE) server and client. The server can send messages to the client, which can listen for these messages. This is useful for testing SSE interactions without needing a real server.
const { EventSource, Server } = require('mock-socket');
const mockServer = new Server('http://localhost:8080');
mockServer.on('connection', socket => {
socket.send('Hello from server');
});
const eventSource = new EventSource('http://localhost:8080');
eventSource.onmessage = event => {
console.log('Received message from server:', event.data);
};
The ws package is a simple to use, blazing fast, and thoroughly tested WebSocket client and server for Node.js. Unlike mock-socket, ws is designed for production use and does not provide mocking capabilities. It is more suitable for actual WebSocket implementations rather than testing.
Socket.IO is a library that enables real-time, bidirectional, and event-based communication between web clients and servers. It provides both client and server components. While it is more feature-rich and supports more use cases than mock-socket, it is not specifically designed for mocking WebSocket or SSE connections.
Nock is an HTTP mocking and expectations library for Node.js. While it does not specifically mock WebSocket or SSE, it is widely used for mocking HTTP requests in tests. It is similar to mock-socket in that it is used for testing purposes, but it focuses on HTTP rather than WebSocket or SSE.
Javascript mocking library for websockets and socket.io
npm install mock-socket
import { WebSocket, Server } from 'mock-socket';
import test from 'ava';
import { Server } from 'mock-socket';
class ChatApp {
constructor(url) {
this.messages = [];
this.connection = new WebSocket(url);
this.connection.onmessage = event => {
this.messages.push(event.data);
};
}
sendMessage(message) {
this.connection.send(message);
}
}
test.cb('that chat app can be mocked', t => {
const fakeURL = 'ws://localhost:8080';
const mockServer = new Server(fakeURL);
mockServer.on('connection', socket => {
socket.on('message', data => {
t.is(data, 'test message from app', 'we have intercepted the message and can assert on it');
socket.send('test message from mock server');
});
});
const app = new ChatApp(fakeURL);
app.sendMessage('test message from app'); // NOTE: this line creates a micro task
// NOTE: this timeout is for creating another micro task that will happen after the above one
setTimeout(() => {
t.is(app.messages.length, 1);
t.is(app.messages[0], 'test message from mock server', 'we have stubbed our websocket backend');
mockServer.stop(t.done);
}, 100);
});
import { WebSocket, Server } from 'mock-socket';
/*
* By default the global WebSocket object is stubbed out when
* a new Server instance is created and is restored when you stop
* the server.
* However, you can disable this behavior by passing `mock: false`
* to the options and manually mock the socket when you need it.
*/
const server = new Server('ws://localhost:8080', { mock: false });
/*
* If you need to stub something else out you can like so:
*/
window.WebSocket = WebSocket; // Here we stub out the window object
const mockServer = new Server('ws://localhost:8080');
mockServer.on('connection', socket => {
socket.on('message', () => {});
socket.on('close', () => {});
socket.on('error', () => {});
socket.send('message');
socket.close();
});
mockServer.clients(); // array of all connected clients
mockServer.emit('room', 'message');
mockServer.stop(optionalCallback);
A declaration file is included by default. If you notice any issues with the types please create an issue or a PR!
Socket.IO has limited support. Below is a similar example to the one above but modified to show off socket.io support.
import test from 'ava';
import { SocketIO, Server } from 'mock-socket';
class ChatApp {
constructor(url) {
this.messages = [];
this.connection = new io(url);
this.connection.on('chat-message', data => {
this.messages.push(event.data);
});
}
sendMessage(message) {
this.connection.emit('chat-message', message);
}
}
test.cb('that socket.io works', t => {
const fakeURL = 'ws://localhost:8080';
const mockServer = new Server(fakeURL);
window.io = SocketIO;
mockServer.on('connection', socket => {
socket.on('chat-message', data => {
t.is(data, 'test message from app', 'we have intercepted the message and can assert on it');
socket.emit('chat-message', 'test message from mock server');
});
});
const app = new ChatApp(fakeURL);
app.sendMessage('test message from app');
setTimeout(() => {
t.is(app.messages.length, 1);
t.is(app.messages[0], 'test message from mock server', 'we have subbed our websocket backend');
mockServer.stop(t.done);
}, 100);
});
The easiest way to work on the project is to clone the repo down via:
git clone git@github.com:thoov/mock-socket.git
cd mock-socket
yarn install
Then to create a local build via:
yarn build
Then create a local npm link via:
yarn link
At this point you can create other projects / apps locally and reference this local build via:
yarn link mock-socket
from within your other projects folder. Make sure that after any changes you run yarn build
!
This project uses ava.js as its test framework. Tests are located in /tests. To run tests:
yarn test
This project uses eslint and a rules set from airbnb's javascript style guides. To run linting:
yarn lint
This project uses prettier. To run the formatting:
yarn format
Code coverage reports are created in /coverage after all of the tests have successfully passed. To run the coverage:
yarn test:coverage
If you have any feedback, encounter any bugs, or just have a question, please feel free to create a github issue or send me a tweet at @thoov.
v9.3.1 (Sep 11th, 2023)
FAQs
Javascript mocking library for websockets and socket.io
We found that mock-socket demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.