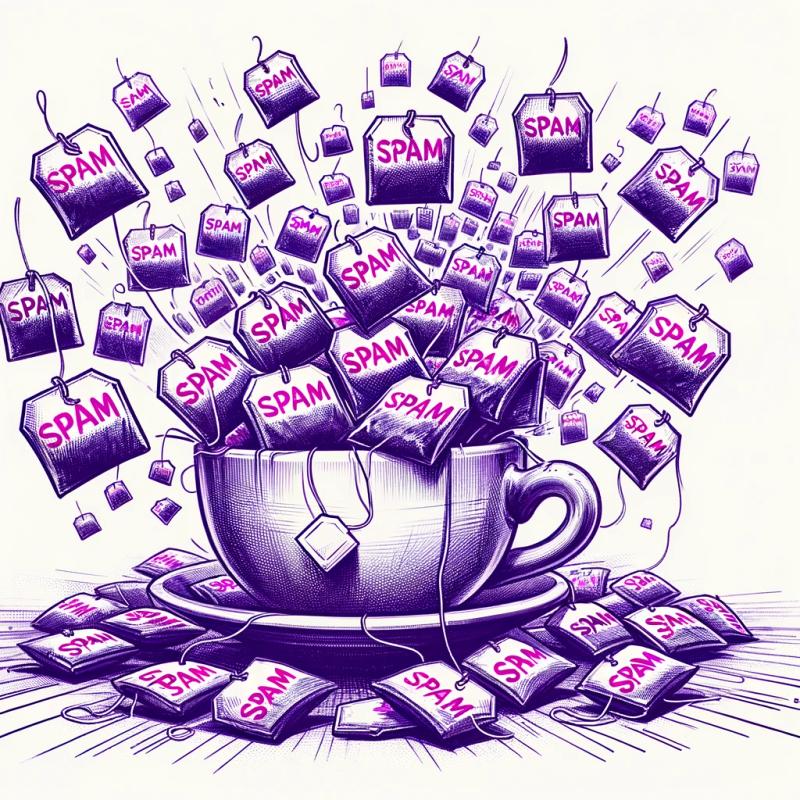
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
modern-error
Advanced tools
Readme
A modern take on JavaScript errors
modern-error
is a drop-in replacement for the native Error class, with slightly more modern features:
message
property to JSON by default, without the need for a JSON replacer function or subclassnpm install modern-error
// Modules
import ModernError from 'modern-error';
// CommonJS
const ModernError = require('modern-error');
Create an error with a message
throw new ModernError('An error occurred');
Create an error with a message and additional properties
throw new ModernError('An error occurred', { code: 'D12' });
Create an error with an object
throw new ModernError({
message: 'An error occurred',
code: 'D12',
test: true
});
Create an error by wrapping a native Error object
const nativeError = new Error('An error occurred');
nativeError.code = 'D12';
throw new ModernError(nativeError);
// The 'message' and 'code' properties are inherited from the native error object
modern-error
is designed to be easily extensible.
class CustomError extends ModernError {}
const error = new CustomError();
console.log(error instanceof CustomError); // true
Subclasses may also define custom properties by overriding the static 'defaults' getter
class ErrorWithDefaults extends ModernError() {
static get defaults() {
return {
test: false,
code: null,
details: 'No further details'
};
}
}
const error = new ErrorWithDefaults('Test', { code: 'D12' });
// error's properties are:
// message: 'Test'
// test: false
// code: 'D12'
// details: 'No further details'
Subclasses may alternatively be created by invoking the static subclass
function.
const HTTPError = ModernError.subclass({
name: 'HTTPError',
defaults: { status: 500 },
serialize: ['message', 'stack']
});
const error = new HTTPError('Error occurred');
// error's properties are:
// message: Error occurred
// status: 500
Defaults properties for instances of ModernError
may be defined by setting the class defaults
property:
ModernError.defaults = {
status: 500,
test: false
};
Or overriding the defaults
static function of a subclass:
class ErrorWithDefaults extends ModernError() {
static get defaults() {
return {
status: 500,
test: false
};
}
}
Or, finally, by declaring defaults in a quick subclass:
const ErrorWithDefaults = ModernError.subclass({
defaults: {
status: 500,
test: false
}
});
modern-error
serializes its non-enumerable message
property to JSON by default. You may also specify which other non-enumerable properties to serialize without subclassing by setting the class property serialize
.
ModernError.serialize = ['message', 'stack'];
// From now on, all JSON representations of a ModernError will include the `stack` property
Or overriding the getter in a subclass.
class StackError extends ModernError {
static get serialize() {
return ['message', 'stack'];
}
}
const error = new StackError('An error has occurred');
console.log(JSON.stringify(error));
// JSON representation will include the `stack` property
All property keys are sorted alphabetically before serialization.
MIT © Jesse Youngblood
FAQs
A modern take on JavaScript errors
The npm package modern-error receives a total of 3 weekly downloads. As such, modern-error popularity was classified as not popular.
We found that modern-error demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.