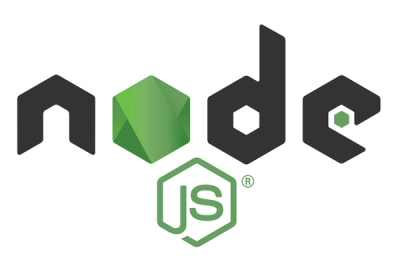
Security News
Node.js Homepage Adds Paid Support Link, Prompting Contributor Pushback
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
mol_data_all
Advanced tools
Defines static typed DTO with strict runtime validation and user friendly error messages like:
Defines static typed DTO with strict runtime validation and user friendly error messages like:
["friends"][0]["phone"] undefined is not any of variants
undefined is not a string
undefined is not a number
// Base Type
const PersonDTO = $mol_data_record({
name : $mol_data_string ,
age : $mol_data_optional( $mol_data_integer ) ,
birthday : $mol_data_pipe( $mol_data_string , $mol_time_moment ) ,
})
// Derived Type
const UserDTO = $mol_data_record({
... PersonDTO.config,
phone: $mol_data_variant( $mol_data_string , $mol_data_integer ),
mail: $mol_data_email,
})
// Ensure this is a User
const jin = UserDTO({
name : 'Jin' ,
age : 33 ,
birthday : '1984-08-04T12:00:00Z' ,
phone : 791234567890,
mail : 'foo@example.org' ,
})
// typeof jin === {
// readonly name: string;
// readonly age?: number | undefined;
// readonly birthday: $mol_time_moment;
// readonly phone: string | number;
// readonly mail: string;
// }
// Allow only Users
function printName( user : typeof UserDTO.Value ) {
console.log( user.name )
}
printName( jin )
// Wrong json from server
const json = {
name : 'Jin' ,
age : 33 ,
birthday : '1984-08-04T12:00:00Z' ,
phone : 791234567890,
mail : '</script>' , // !
} as any
// Runtime error: ["mail"] </script> is not a /.+@.+/
printName( UserDTO( json ) )
const { Weight, Length } = $mol_data_tagged({
Weight: $mol_data_integer,
Length: $mol_data_integer,
})
Length( 20 ) // Validate
let len = Length( 10 ) // Inferred type
let kg: typeof Weight.Value = Weight( 1000 ) // Explicit type
len = 20 // Implicit Cast
let num: number = len // Implicit Cast
len = Length( Weight( 20 ) ) // Explicit Cast
len = Weight( 20 ) // Compile time error
len = Length( 20.1 ) // Run time error
const Duration = $mol_data_pipe(
$mol_data_variant(
$mol_data_string ,
$mol_data_integer ,
) ,
$mol_time_duration ,
)
JSON.stringify( Duration( 'P1D' ) ) // "P1DT"
JSON.stringify( Duration( 1000 ) ) // "PT1S"
const Password = $mol_data_setup( ( val: string ) => {
const str = $mol_data_string( val )
if( str.length >= 8 ) return str
return $mol_fail(
new $mol_data_error( `${ val } have length less than 8` )
)
} )
Password( 123 ) // ❌ 123 is not a string
Password( 'qwerty' ) // ❌ qwerty have length less than 8
Password( 'qwertyuiop' ) // ✅
const MinLength = ( minLength = 8 )=> $mol_data_setup( ( val: string ) => {
const str = $mol_data_string( val )
if( str.length >= minLength ) return str
return $mol_fail(
new $mol_data_error( `${ val } have length less than ${minLength}` )
)
}, minLength )
const Password = MinLength(8)
Password( 123 ) // ❌ 123 is not a string
Password( 'qwerty' ) // ❌ qwerty have length less than 8
Password( 'qwertyuiop' ) // ✅
npm install mol_data_all
import {
$mol_data_tagged as Tagged,
$mol_data_integer as Integer,
} from "mol_data_all"
const { Age } = Tagged({ Age: Integer })
let age = Age( 17 )
age = Age( age + 1 )
FAQs
Defines static typed DTO with strict runtime validation and user friendly error messages like:
The npm package mol_data_all receives a total of 756 weekly downloads. As such, mol_data_all popularity was classified as not popular.
We found that mol_data_all demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.
Research
Security News
The Socket Research Team investigates a malicious Python typosquat of a popular password library that forces Windows shutdowns when input is incorrect.