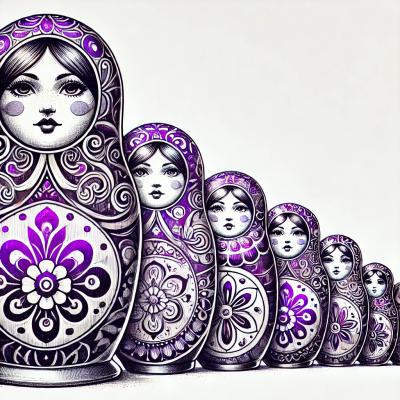
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
monaco-editor
Advanced tools
The monaco-editor npm package provides the code editor that powers VS Code, offering rich IntelliSense, validation for a variety of languages, and advanced editing features. It can be integrated into web applications to provide a full-fledged code editing experience.
Syntax highlighting and IntelliSense
This code initializes the Monaco Editor with JavaScript syntax highlighting and IntelliSense support.
var editor = monaco.editor.create(document.getElementById('container'), {
value: 'function x() {\n console.log("Hello world!");\n}',
language: 'javascript'
});
Code validation and linting
This code adds a marker to the editor model, indicating an error at the specified position with a message.
monaco.editor.setModelMarkers(editor.getModel(), 'owner', [
{ startLineNumber: 1, startColumn: 1, endLineNumber: 1, endColumn: 1, message: 'Error message', severity: monaco.MarkerSeverity.Error }
]);
Custom themes
This code defines a custom theme for the editor and applies it.
monaco.editor.defineTheme('myTheme', {
base: 'vs',
inherit: true,
rules: [{ background: 'EDF9FA' }],
colors: { 'editor.foreground': '#000000' }
});
monaco.editor.setTheme('myTheme');
Keybindings and editor actions
This code adds a custom action to the editor that can be triggered with a keyboard shortcut.
editor.addAction({
id: 'my-unique-id',
label: 'My Label',
keybindings: [monaco.KeyMod.CtrlCmd | monaco.KeyCode.KEY_S],
run: function(ed) {
alert('Action triggered!');
}
});
Ace is a standalone code editor written in JavaScript. It is similar to monaco-editor but with a different API and less out-of-the-box language support. Ace is lightweight and can be easier to integrate into existing projects.
CodeMirror is another browser-based code editor with features like syntax highlighting, a rich API, and various language modes. It is less resource-intensive than monaco-editor and is often used in scenarios where performance is critical.
Brace is a fork of Ace that packages the editor for use with browserify, which can make it easier to use with npm and Node.js-based build systems. It offers similar functionality to Ace.
The Monaco Editor is the code editor that powers VS Code, a good page describing the code editor's features is here.
See the editor in action here.
Learn how to extend the editor and try out your own customizations in the playground.
Browse the latest editor API at monaco.d.ts
.
Please mention the version of the editor when creating issues and the browser you're having trouble in. Create issues in this repository.
In IE, the editor must be completely surrounded in the body element, otherwise the hit testing we do for mouse operations does not work. You can inspect this using F12 and clicking on the body element and confirm that visually it surrounds the editor.
npm install monaco-editor
You will get:
dev
: bundled, not minifiedmin
: bundled, and minifiedmin-maps
: source maps for min
monaco.d.ts
: this specifies the API of the editor (this is what is actually versioned, everything else is considered private and might break with any release).It is recommended to develop against the dev
version, and in production to use the min
version.
Here is the most basic HTML page that embeds the editor. More samples are available at monaco-editor-samples.
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" >
</head>
<body>
<div id="container" style="width:800px;height:600px;border:1px solid grey"></div>
<script src="monaco-editor/min/vs/loader.js"></script>
<script>
require.config({ paths: { 'vs': 'monaco-editor/min/vs' }});
require(['vs/editor/editor.main'], function() {
var editor = monaco.editor.create(document.getElementById('container'), {
value: [
'function x() {',
'\tconsole.log("Hello world!");',
'}'
].join('\n'),
language: 'javascript'
});
});
</script>
</body>
</html>
If you are hosting your .js
on a different domain (e.g. on a CDN) than the HTML, you should know that the Monaco Editor creates web workers for smart language features. Cross-domain web workers are not allowed, but here is how you can proxy their loading and get them to work:
<!--
Assuming the HTML lives on www.mydomain.com and that the editor is hosted on www.mycdn.com
-->
<script type="text/javascript" src="http://www.mycdn.com/monaco-editor/min/vs/loader.js"></script>
<script>
require.config({ paths: { 'vs': 'http://www.mycdn.com/monaco-editor/min/vs' }});
// Before loading vs/editor/editor.main, define a global MonacoEnvironment that overwrites
// the default worker url location (used when creating WebWorkers). The problem here is that
// HTML5 does not allow cross-domain web workers, so we need to proxy the instantion of
// a web worker through a same-domain script
window.MonacoEnvironment = {
getWorkerUrl: function(workerId, label) {
return 'monaco-editor-worker-loader-proxy.js';
}
};
require(["vs/editor/editor.main"], function () {
// ...
});
</script>
<!--
Create http://www.mydomain.com/monaco-editor-worker-loader-proxy.js with the following content:
self.MonacoEnvironment = {
baseUrl: 'http://www.mycdn.com/monaco-editor/min/'
};
importScripts('www.mycdn.com/monaco-editor/min/vs/base/worker/workerMain.js');
That's it. You're good to go! :)
-->
Find full HTML samples here.
Create a Monarch tokenizer here.
Q: What is the relationship between VS Code and the Monaco Editor?
A: The Monaco Editor is generated straight from VS Code's sources with some shims around services the code needs to make it run in a web browser outside of its home.
Q: What is the relationship between VS Code's version and the Monaco Editor's version?
A: None. The Monaco Editor is a library and it reflects directly the source code.
Q: I've written an extension for VS Code, will it work on the Monaco Editor in a browser?
A: No.
Q: Why all these web workers and why should I care?
A: Language services create web workers to compute heavy stuff outside the UI thread. They cost hardly anything in terms of resource overhead and you shouldn't worry too much about them, as long as you get them to work (see above the cross-domain case).
Q: What is this
loader.js
? Can I userequire.js
?
A: It is an AMD loader that we use in VS Code. Yes.
Q: I see the warning "Could not create web worker". What should I do?
A: HTML5 does not allow pages loaded onfile://
to create web workers. Please load the editor with a web server onhttp://
orhttps://
schemes. Please also see the cross domain case above.
Q: Is the editor supported in mobile browsers or mobile web app frameworks?
A: No.
npm run simpleserver
, open http://localhost:8080/monaco-editor/website/npm run release
npm run website
$/src/vscode/
(next to this repo)$/src/vscode> gulp watch
$/src/monaco-editor> npm run simpleserver
$/src/monaco-typescript
(next to this repo)$/src/monaco-typescript> npm run watch
$/src/monaco-editor> npm run simpleserver
monaco-editor
versionmonaco-editor-core
version (if necessary)$/src/vscode> gulp editor-distro
$/src/vscode/out-monaco-editor-core> npm publish
monaco-editor-core
in plugins (if necessary)monaco-editor-core
$/src/monaco-editor/package.json
and update the version for (as necessary):monaco-editor-core
monaco-typescript
monaco-css
monaco-json
monaco-languages
$/src/monaco-editor/package.json
monaco-editor-core
, maybe just vary the patch version.$/src/monaco-editor> npm install .
monaco-editor
$/src/monaco-editor> npm run release
$/src/monaco-editor/release> npm publish
$/src/monaco-editor> npm run release
$/src/monaco-editor> npm run website
$/src/monaco-editor-website> git push origin gh-pages --force
This project has adopted the Microsoft Open Source Code of Conduct. For more information see the Code of Conduct FAQ or contact opencode@microsoft.com with any additional questions or comments.
[0.6.1] (06.09.2016)
editor.addCommand
was no longer working.FAQs
A browser based code editor
The npm package monaco-editor receives a total of 1,160,159 weekly downloads. As such, monaco-editor popularity was classified as popular.
We found that monaco-editor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.