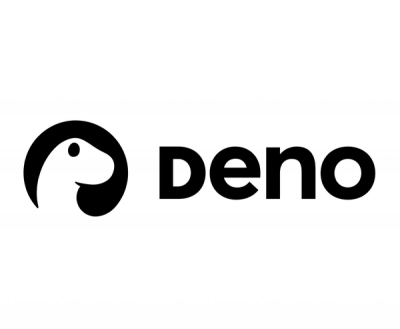
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
A simple framework for MVC web applications and RESTful APIs.
mkdir test-app
cd test-app
npm init
npm install express@5 --save
npm install mvc-webapp --save
mkdir -p application/models
mkdir -p application/controllers
mkdir -p application/views
mkdir -p application/adapters
mkdir -p application/public
At some point this will be automated by a script, for now, it will involve some keystrokes.
#!/usr/bin/env node
const webapp = require('mvc-webapp')
webapp.run({
applicationRoot: process.env.PWD,
listenPort: process.env.PORT || '3000',
sessionRedisUrl: process.env.REDISCLOUD_URL || undefined,
sessionSecret: process.env.SESSION_SECRET || undefined,
redirectSecure: true,
allowCORS: false,
viewEngine: 'ejs', // Optional: Pug, Handlebars, EJS, etc
loggerFormat: 'common', // Morgan formats
trustProxy: true,
notfoundMiddleware: (request, response, next) => {
response.status(404).json({
code: 404,
message: 'Sorry! File Not Found'
})
},
errorMiddleware: (error, request, response, _) => {
if (request.xhr) {
response.status(500).json({
code: 500,
message: (error.message)? error.message : error,
stack: request.app.get('env') === 'development' ? error.stack : ''
})
} else {
response.render('error', {
pageTitle: 'Oops!',
status: 500,
message: (error.message)? error.message : error,
stack: request.app.get('env') === 'development' ? error.stack : '',
})
}
},
})
This is the minimal amount of options you can give, sensible and secure default values are given for everything else:
#!/usr/bin/env node
const webapp = require('mvc-webapp')
webapp.run({
// Mandatory
applicationRoot: process.env.PWD,
listenPort: process.env.PORT || '3000',
// Optional Redis Session Management
// sessionRedisUrl: undefined,
// sessionSecret: undefined,
// Optional Security Related
// redirectSecure: false,
// allowCORS: false,
// trustProxy: false,
// Optional Framework
// viewEngine: undefined, // Pug, Handlebars, EJS, etc
// loggerFormat: 'common', // Morgan formats
// Optional Error Handling
// notfoundMiddleware: undefined,
// errorMiddleware: undefined,
})
The error handling can be customized to return plain JSON, HTTP codes or an EJS rendered page, your choice.
exports.actions = controller => {
controller.get('/', (request, response, _) => {
response.json({
status: 'Sample status...',
data: null,
})
})
controller.get('/async', async (request, response) => {
const hi = await Promise.resolve('Hi!')
response.send(hi)
})
controller.get('/fail', async (request, response) => {
await Promise.reject('REJECTED!')
})
controller.get('/denied', async (request, response) => {
response.status(403).send('Not here')
})
return controller
}
This should be familiar to any Express user. A special exception is made for the index.js controller file, this is mapped to the root / folder. Additionally, any routes inside that controller, get appended as a method.
In order to render a view, invoke the view (file)name in the res.render call:
response.render('index', {
title: 'Homepage',
user: 'octopie'
})
Add the following file to the root folder and docker build:
FROM node:latest
WORKDIR /app
ADD . /app
RUN npm install
CMD ["npm","start"]
FAQs
A simple framework for MVC web applications and RESTful APIs.
The npm package mvc-webapp receives a total of 4 weekly downloads. As such, mvc-webapp popularity was classified as not popular.
We found that mvc-webapp demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.