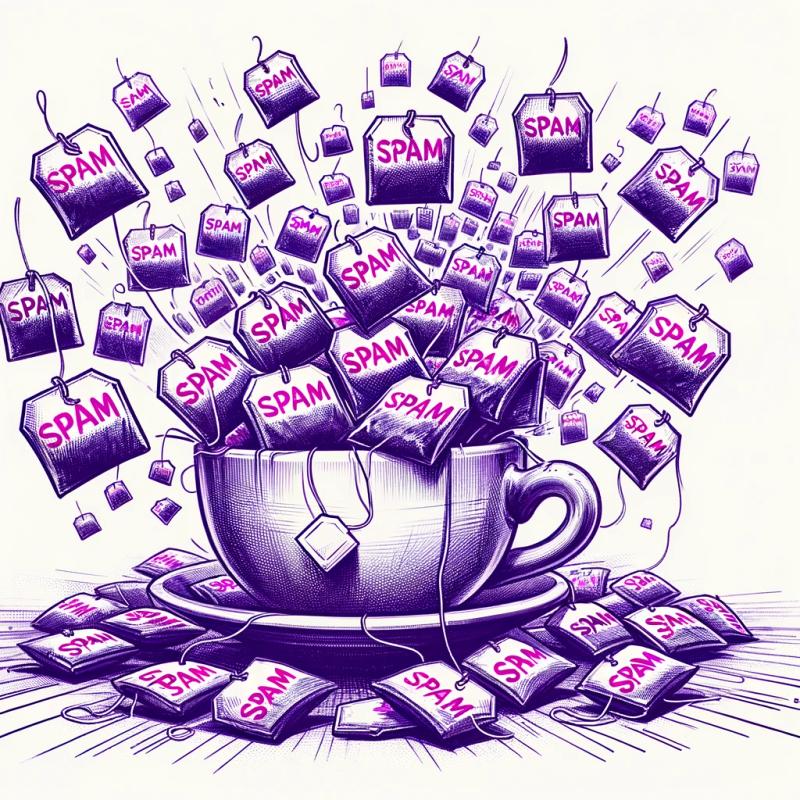
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
mysql-node-client
Advanced tools
Readme
A Node.js mysql client implementation, using the following mysql driver: https://www.npmjs.com/package/mysql
Installation is done using the npm install
command
$ npm install mysql-node-client
In order to create an instance of the mysqlClient, you must do the following:
const createMysqlClient = require('mysql-node-client')
const CONNECTION_DATA = {
host: 'localhost',
port: '3307',
user: 'myUser',
password: 'asd123',
database: 'test_db'
}
const test = async () => {
try {
//create an instance of the client
const mysqlClient = createMysqlClient(CONNECTION_DATA)
//call a method exposed by the client
const data = await mysqlClient.list('test_table')
//use the response data
console.log(data.results)
//destroy the connection pool when it's not needed anymore
mysqlClient.destroyConnectionPool()
} catch (err) {
console.log(err)
}
}
test()
All the connections are pooled in a connection pool. The default connectionLimit is 100 and the default acquireTimeout is 10 seconds. If all the connections in the pool are used, there's a default queue of 100 slots which can hold requests. All the default options can be overriden by passing a second object to the createMysqlClient method.
createDatabase
- The connection must not be using a database nameconst data = await mysqlClient.createDatabase('test_db')
listDatabases
- The connection must not be using a database nameconst data = await mysqlClient.listDatabases()
createTable
const TABLE_SCHEMA = [
{
name: 'id',
type: 'INT AUTO_INCREMENT PRIMARY KEY',
},
{
name: 'name',
type: 'VARCHAR(255) NOT NULL'
}
]
const data = await mysqlClient.createTable('test_table', TABLE_SCHEMA)
listTables
const data = await mysqlClient.listTables()
describeTable
const data = await mysqlClient.describeTable('test_table')
store
const data = await mysqlClient.store(
'test_table', //tableName
{ //record
name: 'test_entry'
}
)
update
const data = await mysqlClient.update(
'test_table', //tableName
{ name: 'test_updated_name' }, //updatedFields
{ placeholders: 'id > ? AND id <= ?', values: [ recordId - 1, recordId ]} //filters
)
destroy
const data = await mysqlClient.destroy(
'test_table', //tableName
{ placeholders: 'id = ?', values: [ recordId ]} //filters
)
list
- Supports columns projectionconst data = await mysqlClient.list(
'test_table', //tableName
[ 'id' ] //projection - only these columns will be returned. if absent, all columns are displayed
)
fetch
- Supports columns projection and filteringconst data = await mysqlClient.fetch(
'test_table', //tableName
{ placeholders: 'id = ?', values: [ recordId ]}, //filters
[ 'id' ] //projection - only these columns will be returned. if absent, all columns are displayed
)
getConnection
- Used for retrieving a connection from the connection pool, or creating a new one. That connection is locked and can be used until it is released.//create an instance of the client
const mysqlClient = createMysqlClient(CONNECTION_DATA)
//retrieve or create a connection to the db
const connection = await mysqlClient.getConnection()
//use any method from the connection object, as provided by the mysql driver
connection.query('SELECT * FROM test_table', (err, results, fields) => {
if (err) throw err
console.log(results)
})
destroyConnectionPool
- Destroy the connection pool when it's not needed anymorequery
- A wrapper over the base query method. Extras: connection management (retrieval and release from/to the connection pool), logging system (connection id and sql query executed).const data = await mysqlClient.query('SELECT count(*) FROM test_table')
For more information, check the mysql driver documentation (https://www.npmjs.com/package/mysql). This implementation should be treated as a wrapper of the base driver.
FAQs
A node client for mysql
The npm package mysql-node-client receives a total of 35 weekly downloads. As such, mysql-node-client popularity was classified as not popular.
We found that mysql-node-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.