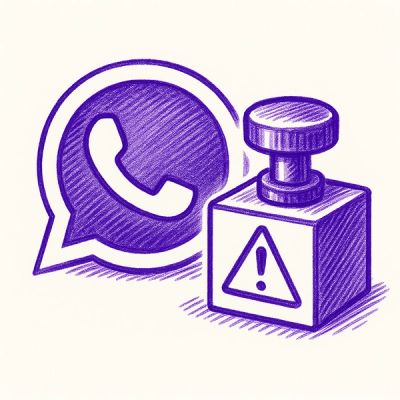
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
mysql-simple-query
Advanced tools
Simple query wrapper for mysql-promise to make querying, inserting, updating, and deleting easier for developers.
Simple mysql query builder to make querying, inserting, updating, and deleting easier for developers. This library can be used with any mysql library.
This library takes the annoyance out of writing raw MySQL queries in Javascript. The queries are easy to read in your code and easy to build complex queries.
npm install --save mysql-simple-query
All calls need to be have a reference to the mysql-simple-query class.
const mysqlQuery = require("mysql-simple-query");
const db = new mysqlQuery();
db.select('id, name');
db.from('users');
db.where('name', 'foo');
const results = db.query();
results.then(function(result) {
console.log(result);
});
If Select *
is needed you can leave off the db.select and it will be defaulted.
db.select('id, name');
db.join('table', 'abc = def');
db.from('users');
db.where('name', 'foo');
const results = db.query();
results.then(function(result) {
console.log(result);
});
The above example will produce an INNER JOIN. There can be multiple db.join and db.where to chain them together.
db.select('id, name');
db.join('table', 'abc = def');
db.join('table2', 'abc = def');
db.from('users');
db.where('id', '123');
db.where('name', 'foo');
const results = db.query();
results.then(function(result) {
console.log(result);
});
db.groupBy('item');
db.orderBy('item', 'ACS');
db.limit(1);
/**
* @param {string} table Name of the database table to insert into
* @param {object} data data to insert into the database
* @returns {promise}
*/
const results = db.insert('users_table', {
'name': 'foo bar',
'department': 'engineering',
'datetime': '2019-08-27 03:11:06'
});
results.then(function(result) {
console.log(result);
});
/**
* @param {string} table Name of the database table to update
* @param {object} data data to update into the database
* @returns {promise}
*/
const results = db.update('users_table', {
'name': 'foo bar',
'department': 'engineering',
'datetime': '2019-08-27 03:11:06'
});
results.then(function(result) {
console.log(result);
});
db.where('name', 'foo');
db.delete('users');
If you need to pass in your own custom query into mysql-promise you can do so by calling the following.
const results = db.queryRaw('SELECT * FROM TABLE...');
/**
* @param {string} select string Comma seperated list
* @returns {promise}
*/
db.select('id','name','department');
/**
* @param {string} table_name
* @returns {promise}
*/
db.from('users');
These calls can be chained together to form multiple where statements.
/**
* @param {string} key
* @param {string} value
* @returns {promise}
*/
db.where('name','foo');
db.where('deparment','engineering');
This will produce an INNER JOIN. These calls can be chained together to form multiple join statements.
/**
* @param {string} key
* @param {string} value
* @returns {promise}
*/
db.join('table', 'item = item2');
/**
* @param {string} key
* @returns {promise}
*/
db.groupBy('key');
/**
* @param {string} key
* @param {ENUM} order ASC or DESC
* @returns {promise}
*/
db.orderBy('key', 'ASC');
/**
* @param {int} number to limit by
* @returns {promise}
*/
db.limit(1);
This project is licensed under the MIT License
FAQs
Simple query wrapper for mysql-promise to make querying, inserting, updating, and deleting easier for developers.
The npm package mysql-simple-query receives a total of 1 weekly downloads. As such, mysql-simple-query popularity was classified as not popular.
We found that mysql-simple-query demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.