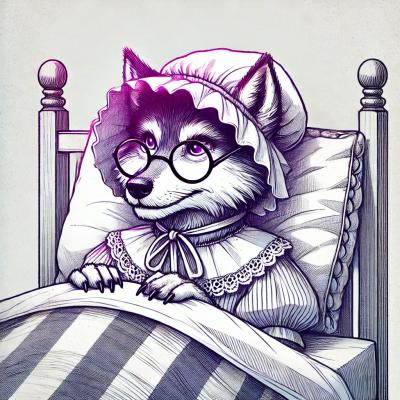
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
napi-thread-safe-callback
Advanced tools
C++ utility class to perform callbacks into JavaScript from any thread
This package contains a header-only C++ helper class to facilitate calling back into JavaScript from threads other than the Node.JS main thread.
void example_async_work(const CallbackInfo& info)
{
// Capture callback in main thread
auto callback = std::make_shared<ThreadSafeCallback>(info[0].As<Function>());
bool fail = info.Length() > 1;
// Pass callback to other thread
std::thread([callback, fail]
{
try
{
// Do some work to get a result
if (fail)
throw std::runtime_error("Failure during async work");
std::string result = "foo";
// Call back with result
callback->call([result](Napi::Env env, std::vector<napi_value>& args)
{
// This will run in main thread and needs to construct the
// arguments for the call
args = { env.Undefined(), Napi::String::New(env, result) };
});
}
catch (std::exception& e)
{
// Call back with error
callback->callError(e.what());
}
}).detach();
}
void example_async_return_value(const CallbackInfo& info)
{
auto callback = std::make_shared<ThreadSafeCallback>(info[0].As<Function>());
std::thread([callback]
{
try
{
int result = 0;
while (true)
{
// Do some work...
try
{
result += 1;
if (result > 20)
throw std::runtime_error("Failure during async work");
}
catch (std::exception &e)
{
// Call back with error
// Note that this will cause the thread to exit and the
// ThreadSafeCallback to be destroyed. But the callback
// will still be called, even afterwards
callback->callError(e.what());
throw;
}
// Call back into Javascript with current result
auto future = callback->call<bool>(
[result](Env env, std::vector<napi_value> &args) {
args = {env.Undefined(), Number::New(env, result)};
},
[](const Value &val) {
return val.As<Boolean>().Value();
});
// This blocks until the JS call has returned a value
// In case the callback throws we just let the exception
// bubble up and end the thread
auto continue_running = future.get();
if (!continue_running)
break;
}
}
catch (...)
{
// Ignore errors
}
}).detach();
}
package.json
: "dependencies": {
"napi-thread-safe-callback": "0.0.1",
}
binding.gyp
: 'include_dirs': ["<!@(node -p \"require('napi-thread-safe-callback').include\")"],
#include "napi-thread-safe-callback.hpp"
Exceptions need to be enabled in the native module. To do this use the following
in binding.gyp
:
'cflags!': [ '-fno-exceptions' ],
'cflags_cc!': [ '-fno-exceptions' ],
'xcode_settings': {
'GCC_ENABLE_CPP_EXCEPTIONS': 'YES',
'CLANG_CXX_LIBRARY': 'libc++',
'MACOSX_DEPLOYMENT_TARGET': '10.7',
},
'msvs_settings': {
'VCCLCompilerTool': { 'ExceptionHandling': 1 },
},
'conditions': [
['OS=="win"', { 'defines': [ '_HAS_EXCEPTIONS=1' ] }]
]
FAQs
C++ utility class to perform callbacks into JavaScript from any thread
We found that napi-thread-safe-callback demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.