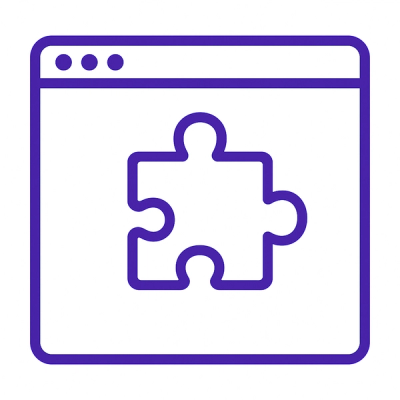
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
nc-one-react-helpers
Advanced tools
Developments that will simplify the life of a React developer
npm i nc-one-react-helpers
or yarn add nc-one-react-helpers
Import:
import { DateTimePicker } from 'nc-one-react-helpers'
Example of use:
<DateTimePicker
date={new Date()}
onDateTimeChange={(date) => console.log(date)}
getDateTimeString={(date) => console.log(date)}
format='MMMM DD, YYYY - hh:mm'
/>
Interface:
interface DateTimePickerProps extends ITextFieldProps {
date?: Date
stringDate?: string
format?: string
withIcon?: boolean
onDateTimeChange?: (date?: Date) => void
getDateTimeString?: (date?: string) => void
CalendarStrings?: ICalendarStrings
}
Name | Type | Default value | Description |
---|---|---|---|
date | date | undefined | date and time to be recorded and selected automatically |
stringDate | string | undefined | date and time which will be written and selected automatically as a string (string must be in the same format as format) |
format | string | "MM.DD.YYYY, hh:mma" | format of the string in which the date and time will be displayed and output more here |
withIcon | boolean | true | whether the time date icon will be shown |
onDateTimeChange | (date?: Date) => void | undefined | Do something with the date time every time it changes |
getDateTimeString | ICalendarStrings | initialCalendarStrings | the way the calendar lines will be displayed |
DateTimePickerProps
is inherited from ITextFieldProps
, so all TextField
props will work fine!
Import:.
import { useMediaQuery } from 'nc-one-react-helpers'
Example of use:
const matches = useMediaQuery('(min-width: 800px)')
if (matches) return <>Screen width greater than 800px</>
else return <>Screen width 800px or less</>
Type:
type useMediaQuery = (query: string) => boolean
Takes the string as in css @usemedia
and returns true
if the screen width satisfies the condition or false
otherwise.
Import:.
import { UTCHoursPlus } from 'nc-one-react-helpers'
Example usage:
export const Time: React.FC = () => {
const [time, setTime] = useState('')
setTimeout(() => setTime(`${UTCHoursPlus(1)}:${new Date().getUTCMinutes()}:${new Date().getUTCSeconds()}`), 1000)
return <>Storage time: {time} (UTC+01:00)</>
}
type:
type UTCHoursPlus = (plus: number) => number
Accepts how much to increase the current time and returns UTC clock+input parameter
Import:
import { sleep } from 'nc-one-react-helpers'
Example of use:
onSubmit={async (values) => {
setProgressIndicator(true); // show spinner
try {
sleep(500) //wait 500 milliseconds
// mimic a request to the server
} catch (e) {
console.log(e)
} finally {
setProgressIndicator(false) // hide the spinner
}
}}
Type:
type sleep = (ms: number) => Promise<unknown>
Wait for the entered number of milliseconds, and then execute the code below
Import:
import { required, invalidEmail, invalidPassword, matchPassword, positiveNumber } from 'nc-one-react-helpers'
Type:
type required = (value: string, text?: string | undefined) => string | undefined
type invalidEmail = (value: string, text?: string | undefined) => string | undefined
type invalidPassword = (value: string, text?: string | undefined) => string | undefined
type matchPassword = (password: string, rePassword: string, text?: string | undefined) => string | undefined
type positiveNumber = (value: number, text?: string | undefined) => string | undefined
Example of use:
validate={({ email, position, password, repassword }) => {
if (
!required(email) &&
!invalidEmail(email) &&
!required(position) &&
!positiveNumber(position) &&
!required(password) &&
!invalidPassword(password) &&
!& required(repassword) &&
!!invalidPassword(repassword) &&
!!matchPassword(password, repassword)
) return {};
return {
email: required(email) || invalidEmail(email),
position: required(position) || positiveNumber(position),
password: required(password) || invalidPassword(password),
repassword: required(repassword) || invalidPassword(repassword) || matchPassword(password, repassword)
};
}}
required
- Accepts value
and returns error text if value
is empty or undefined
otherwise.invalidEmail
- Accepts value
and returns error text if value
is not a valid email address or undefined
otherwise.invalidPassword
- Accepts value
and returns error text if value
fails validation check (too simple) or undefined
otherwise.password validation: minimum 8 characters, minimum 1 Latin letter A-Za-z, minimum 1 digit 0-9
matchPassword
- Accepts password
and rePassword
and returns error text if passwords do not match or undefined
otherwise.positiveNumber
- Accepts a number and returns an error text if it is negative or undefined
otherwise.text
- Optional parameter for all validations. It determines what the error message will be. If it is not specified, the default (in Polish) message will be displayed
npm i nc-one-react-helpers
ΠΈΠ»ΠΈ yarn add nc-one-react-helpers
ΠΠΌΠΏΠΎΡΡ:
import { DateTimePicker } from 'nc-one-react-helpers'
ΠΡΠΈΠΌΠ΅Ρ ΠΈΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°Π½ΠΈΡ:
<DateTimePicker
date={new Date()}
onDateTimeChange={(date) => console.log(date)}
getDateTimeString={(date) => console.log(date)}
format='MMMM DD, YYYY - hh:mm'
/>
ΠΠ½ΡΠ΅ΡΡΠ΅ΠΉΡ:
interface DateTimePickerProps extends ITextFieldProps {
date?: Date
stringDate?: string
format?: string
withIcon?: boolean
onDateTimeChange?: (date?: Date) => void
getDateTimeString?: (date?: string) => void
CalendarStrings?: ICalendarStrings
}
ΠΠ°Π·Π²Π°Π½ΠΈΠ΅ | Π’ΠΈΠΏ | ΠΠ΅ΡΠΎΠ»ΡΠ½ΠΎΠ΅ Π·Π½Π°ΡΠ΅Π½ΠΈΠ΅ | ΠΠΏΠΈΡΠ°Π½ΠΈΠ΅ |
---|---|---|---|
date | Date | undefined | ΠΠ°ΡΠ° ΠΈ Π²ΡΠ΅ΠΌΡ ΠΊΠΎΡΠΎΡΡΠ΅ Π±ΡΠ΄ΡΡ Π·Π°ΠΏΠΈΡΠ°Π½Ρ ΠΈ Π²ΡΠ±ΡΠ°Π½Ρ Π°Π²ΡΠΎΠΌΠ°ΡΠΈΡΠ΅ΡΠΊΠΈ |
stringDate | string | undefined | ΠΠ°ΡΠ° ΠΈ Π²ΡΠ΅ΠΌΡ ΠΊΠΎΡΠΎΡΡΠ΅ Π±ΡΠ΄ΡΡ Π·Π°ΠΏΠΈΡΠ°Π½Ρ ΠΈ Π²ΡΠ±ΡΠ°Π½Ρ Π°Π²ΡΠΎΠΌΠ°ΡΠΈΡΠ΅ΡΠΊΠΈ Π² Π²ΠΈΠ΄Π΅ ΡΡΡΠΎΠΊΠΈ (ΡΡΡΠΎΠΊΠ° Π΄ΠΎΠ»ΠΆΠ½Π° Π±ΡΡΡ Π² ΡΠ°ΠΊΠΎΠΌ ΠΆΠ΅ ΡΠΎΡΠΌΠ°ΡΠ΅ ΡΡΠΎ ΠΈ format) |
format | string | "MM.DD.YYYY, hh:mma" | Π€ΠΎΡΠΌΠ°Ρ ΡΡΡΠΎΠΊΠΈ Π² ΠΊΠΎΡΠΎΡΠΎΠΌ Π±ΡΠ΄Π΅Ρ ΠΎΡΠΎΠ±ΡΠ°ΠΆΠ°ΡΡΡΡ ΠΈ Π²ΡΠ²ΠΎΠ΄ΠΈΡΡΡΡ Π΄Π°ΡΠ° Π²ΡΠ΅ΠΌΡ ΠΏΠΎΠ΄ΡΠΎΠ±Π½Π΅Π΅ Π·Π΄Π΅ΡΡ |
withIcon | boolean | true | ΠΡΠ΄Π΅Ρ Π»ΠΈ ΠΏΠΎΠΊΠ°Π·ΡΠ²Π°ΡΡΡΡ ΠΈΠΊΠΎΠ½ΠΊΠ° Π΄Π°ΡΡ Π²ΡΠ΅ΠΌΠ΅Π½ΠΈ |
onDateTimeChange | (date?: Date) => void | undefined | ΠΠ΅Π»Π°ΡΡ ΡΡΠΎ-ΡΠΎ Ρ Π΄Π°ΡΠΎΠΉ Π²ΡΠ΅ΠΌΠ΅Π½Π΅ΠΌ ΠΏΡΠΈ ΠΊΠ°ΠΆΠ΄ΠΎΠΌ ΠΈΠ·ΠΌΠ΅Π½Π΅Π½ΠΈΠΈ |
getDateTimeString | ICalendarStrings | initialCalendarStrings | Π’ΠΎ ΠΊΠ°ΠΊ Π±ΡΠ΄ΡΡ ΠΎΡΠΎΠ±ΡΠ°ΠΆΠ°ΡΡΡΡ ΡΡΡΠΎΠΊΠΈ ΠΊΠ°Π»Π΅Π½Π΄Π°ΡΡ |
DateTimePickerProps
Π½Π°ΡΠ»Π΅Π΄ΡΠ΅ΡΡΡ ΠΎΡ ITextFieldProps
, ΠΏΠΎΡΡΠΎΠΌΡ Π²ΡΠ΅ ΠΏΡΠΎΠΏΡΡ TextField
Π±ΡΠ΄ΡΡ ΠΏΡΠ΅ΠΊΡΠ°ΡΠ½ΠΎ ΡΠ°Π±ΠΎΡΠ°ΡΡ!
ΠΠΌΠΏΠΎΡΡ:
import { useMediaQuery } from 'nc-one-react-helpers'
ΠΡΠΈΠΌΠ΅Ρ ΠΈΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°Π½ΠΈΡ:
const matches = useMediaQuery('(min-width: 800px)')
if (matches) return <>Π¨ΠΈΡΠΈΠ½Π° ΡΠΊΡΠ°Π½Π° Π±ΠΎΠ»ΡΡΠ΅ 800px</>
else return <>Π¨ΠΈΡΠΈΠ½Π° ΡΠΊΡΠ°Π½Π° 800px ΠΈΠ»ΠΈ ΠΌΠ΅Π½ΡΡΠ΅</>
Π’ΠΈΠΏ:
type useMediaQuery = (query: string) => boolean
ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ ΡΡΡΠΎΠΊΡ ΠΊΠ°ΠΊ Π² css @usemedia
ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ true
Π² ΡΠ»ΡΡΠ°Π΅ Π΅ΡΠ»ΠΈ ΡΠΈΡΠΈΠ½Π° ΡΠΊΡΠ°Π½Π° ΡΠ΄ΠΎΠ²Π»Π΅ΡΠ²ΠΎΡΡΠ΅Ρ ΡΡΠ»ΠΎΠ²ΠΈΠ΅ ΠΈΠ»ΠΈ false
Π² ΠΏΡΠΎΡΠΈΠ²Π½ΠΎΠΌ ΡΠ»ΡΡΠ°Π΅.
ΠΠΌΠΏΠΎΡΡ:
import { UTCHoursPlus } from 'nc-one-react-helpers'
ΠΡΠΈΠΌΠ΅Ρ ΠΈΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°Π½ΠΈΡ:
export const Time: React.FC = () => {
const [time, setTime] = useState('')
setTimeout(() => setTime(`${UTCHoursPlus(1)}:${new Date().getUTCMinutes()}:${new Date().getUTCSeconds()}`), 1000)
return <>Storage time: {time} (UTC+01:00)</>
}
Π’ΠΈΠΏ:
type UTCHoursPlus = (plus: number) => number
ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ ΡΠΎ Π½Π°ΡΠΊΠΎΠ»ΡΠΊΠΎ ΡΠ²Π΅Π»ΠΈΡΠΈΡΡ ΡΠ΅ΠΊΡΡΠ΅Π΅ Π²ΡΠ΅ΠΌΡ ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ ΡΠ°ΡΡ UTC+Π²Π²ΡΠ΄ΡΠΉ ΠΏΠ°ΡΠ°ΠΌΠ΅ΡΡ
ΠΠΌΠΏΠΎΡΡ:
import { sleep } from 'nc-one-react-helpers'
ΠΡΠΈΠΌΠ΅Ρ ΠΈΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°Π½ΠΈΡ:
onSubmit={async (values) => {
setProgressIndicator(true); // ΠΏΠΎΠΊΠ°Π·Π°ΡΡ ΡΠΏΠΈΠ½Π½Π΅Ρ
try {
sleep(500) // ΠΏΠΎΠ΄ΠΎΠΆΠ΄Π°ΡΡ 500 ΠΌΠΈΠ»Π»ΠΈΡΠ΅ΠΊΡΠ½Π΄
// ΡΠ΄Π΅Π»Π°ΡΡ ΠΈΠΌΠΈΡΠ°ΡΠΈΡ Π·Π°ΠΏΡΠΎΡΠ° Π½Π° ΡΠ΅ΡΠ²Π΅Ρ
} catch (e) {
console.log(e)
} finally {
setProgressIndicator(false) // ΡΠΊΡΡΡΡ ΡΠΏΠΈΠ½Π½Π΅Ρ
}
}}
Π’ΠΈΠΏ:
type sleep = (ms: number) => Promise<unknown>
ΠΠΎΠ΄ΠΎΠΆΠ΄Π°ΡΡ Π²Π²Π΅Π΄ΡΠ½Π½ΠΎΠ΅ ΠΊΠΎΠ»ΠΈΡΠ΅ΡΡΠ²ΠΎ ΠΌΠΈΠ»ΠΈΡΠ΅ΠΊΡΠ½Π΄, ΠΈ ΡΠΎΠ»ΡΠΊΠΎ ΠΏΠΎΡΠΎΠΌ Π²ΡΠΏΠΎΠ»Π½ΠΈΡΡ ΠΊΠΎΠ΄ Π½ΠΈΠΆΠ΅
ΠΠΌΠΏΠΎΡΡ:
import { required, invalidEmail, invalidPassword, matchPassword, positiveNumber } from 'nc-one-react-helpers'
Π’ΠΈΠΏΡ:
type required = (value: string, text?: string | undefined) => string | undefined
type invalidEmail = (value: string, text?: string | undefined) => string | undefined
type invalidPassword = (value: string, text?: string | undefined) => string | undefined
type matchPassword = (password: string, rePassword: string, text?: string | undefined) => string | undefined
type positiveNumber = (value: number, text?: string | undefined) => string | undefined
ΠΡΠΈΠΌΠ΅Ρ ΠΈΡΠΏΠΎΠ»ΡΠ·ΠΎΠ²Π°Π½ΠΈΡ:
validate={({ email, position, password, repassword }) => {
if (
!required(email) &&
!invalidEmail(email) &&
!required(position) &&
!positiveNumber(position) &&
!required(password) &&
!invalidPassword(password) &&
!required(repassword) &&
!invalidPassword(repassword) &&
!matchPassword(password, repassword)
) return {};
return {
email: required(email) || invalidEmail(email),
position: required(position) || positiveNumber(position),
password: required(password) || invalidPassword(password),
repassword: required(repassword) || invalidPassword(repassword) || matchPassword(password, repassword)
};
}}
required
- ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ value
ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ ΡΠ΅ΠΊΡΡ ΠΎΡΠΈΠ±ΠΊΠΈ Π² ΡΠ»ΡΡΠ°Π΅ Π΅ΡΠ»ΠΈ value
ΠΏΡΡΡΠΎΠΉ ΠΈΠ»ΠΈ undefined
Π² ΠΏΡΠΎΡΠΈΠ²Π½ΠΎΠΌ ΡΠ»ΡΡΠ°Π΅.invalidEmail
- ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ value
ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ ΡΠ΅ΠΊΡΡ ΠΎΡΠΈΠ±ΠΊΠΈ Π² ΡΠ»ΡΡΠ°Π΅ Π΅ΡΠ»ΠΈ value
Π½Π΅ ΡΠ²Π»ΡΠ΅ΡΡΡ Π²Π°Π»ΠΈΠ΄Π½ΡΠΌ ΠΏΠΎΡΡΠΎΠ²ΡΠΌ Π°Π΄ΡΠ΅ΡΠΎΠΌ (email) ΠΈΠ»ΠΈ undefined
Π² ΠΏΡΠΎΡΠΈΠ²Π½ΠΎΠΌ ΡΠ»ΡΡΠ°Π΅.invalidPassword
- ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ value
ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ ΡΠ΅ΠΊΡΡ ΠΎΡΠΈΠ±ΠΊΠΈ Π² ΡΠ»ΡΡΠ°Π΅ Π΅ΡΠ»ΠΈ value
Π½Π΅ ΠΏΡΠΎΡ
ΠΎΠ΄ΠΈΡ ΠΏΡΠΎΠ²Π΅ΡΠΊΡ Π²Π°Π»ΠΈΠ΄Π°ΡΠΈΠΈ (ΡΠ»ΠΈΡΠΊΠΎΠΌ ΠΏΡΠΎΡΡΠΎΠΉ) ΠΈΠ»ΠΈ undefined
Π² ΠΏΡΠΎΡΠΈΠ²Π½ΠΎΠΌ ΡΠ»ΡΡΠ°Π΅.Π²Π°Π»ΠΈΠ΄Π°ΡΠΈΡ ΠΏΠ°ΡΠΎΠ»Ρ: ΠΌΠΈΠ½ΠΈΠΌΡΠΌ 8 ΡΠΈΠΌΠ²ΠΎΠ»ΠΎΠ², ΠΌΠΈΠ½ΠΈΠΌΡΠΌ 1 Π»Π°ΡΠΈΠ½ΡΠΊΠ°Ρ Π±ΡΠΊΠ²Π° A-Za-z, ΠΌΠΈΠ½ΠΈΠΌΡΠΌ 1 ΡΠΈΡΡΠ° 0-9
matchPassword
- ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ password
ΠΈ rePassword
ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ ΡΠ΅ΠΊΡΡ ΠΎΡΠΈΠ±ΠΊΠΈ Π² ΡΠ»ΡΡΠ°Π΅ Π΅ΡΠ»ΠΈ ΠΏΠ°ΡΠΎΠ»ΠΈ Π½Π΅ ΡΠΎΠ²ΠΏΠ°Π΄Π°ΡΡ ΠΈΠ»ΠΈ undefined
Π² ΠΏΡΠΎΡΠΈΠ²Π½ΠΎΠΌ ΡΠ»ΡΡΠ°Π΅.positiveNumber
- ΠΡΠΈΠ½ΠΈΠΌΠ°Π΅Ρ ΡΠΈΡΠ»ΠΎ ΠΈ Π²ΠΎΠ·Π²ΡΠ°ΡΠ°Π΅Ρ ΡΠ΅ΠΊΡΡ ΠΎΡΠΈΠ±ΠΊΠΈ Π² ΡΠ»ΡΡΠ°Π΅ Π΅ΡΠ»ΠΈ ΠΎΠ½ΠΎ ΠΎΡΡΠΈΡΠ°ΡΠ΅Π»ΡΠ½ΠΎΠ΅ ΠΈΠ»ΠΈ undefined
Π² ΠΏΡΠΎΡΠΈΠ²Π½ΠΎΠΌ ΡΠ»ΡΡΠ°Π΅.text
- ΠΠ΅ΠΎΠ±ΡΠ·Π°ΡΠ΅Π»ΡΠ½ΡΠΉ ΠΏΠ°ΡΠ°ΠΌΠ΅ΡΡ Π²ΡΠ΅Ρ
Π²Π°Π»ΠΈΠ΄Π°ΡΠΈΠΉ. ΠΠ½ ΠΎΡΠ²Π΅ΡΠ°Π΅Ρ Π·Π° ΡΠΎ ΠΊΠ°ΠΊΠΈΠΌ Π±ΡΠ΄Π΅Ρ ΡΠΎΠΎΠ±ΡΠ΅Π½ΠΈΠ΅ ΠΎΠ± ΠΎΡΠΈΠ±ΠΊΠ΅. ΠΡΠ»ΠΈ ΠΎΠ½ Π½Π΅ ΡΠΊΠ°Π·Π°Π½ Π±ΡΠ΄Π΅Ρ Π²ΡΠ²Π΅Π΄Π΅Π½ΠΎ ΡΠΎΠΎΠ±ΡΠ΅Π½ΠΈΠ΅ ΠΏΠΎ ΡΠΌΠΎΠ»ΡΠ°Π½ΠΈΡ (Π½Π° ΠΠΎΠ»ΡΡΠΊΠΎΠΌ)
FAQs
Developments that will simplify the life of a React developer
The npm package nc-one-react-helpers receives a total of 2 weekly downloads. As such, nc-one-react-helpers popularity was classified as not popular.
We found that nc-one-react-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.