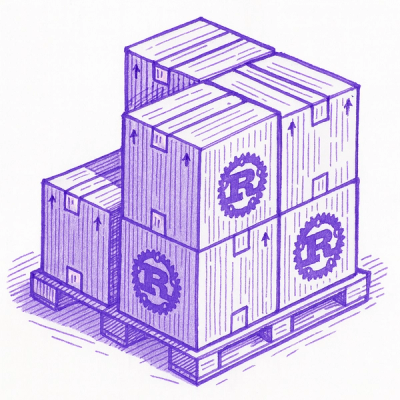
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
The neo-async package is a utility module which provides straight-forward, powerful functions for working with asynchronous JavaScript. It is similar to the async package but with some performance improvements.
Control Flow
Execute a series of functions in sequential order. Each function is passed a callback it must call on completion.
async.series([
function(callback) {
// do some stuff ...
callback(null, 'one');
},
function(callback) {
// do some more stuff ...
callback(null, 'two');
}
],
function(err, results) {
// results is now equal to ['one', 'two']
});
Collections
Apply a function to each item in a collection and collect the results.
async.map(['file1','file2','file3'], fs.stat, function(err, results) {
// results is now an array of stats for each file
});
Utilities
Call a function a certain number of times and collect the results.
async.times(5, function(n, next) {
createUser(n, function(err, user) {
next(err, user);
});
}, function(err, users) {
// we should now have 5 users
});
The original async package offers a wide array of functions for working with asynchronous code. Neo-async claims to offer similar functionality with improved performance.
Bluebird is a full-featured promise library with a focus on innovative features and performance. It can be used as an alternative to neo-async for handling asynchronous operations using promises instead of callbacks.
Q is a tool for making and composing asynchronous promises in JavaScript. It's an older promise library that can serve similar purposes to neo-async but with a different style of handling async operations.
Neo-Async is faster than Async.js and has more feature.
$ npm install neo-async
var _ = require('lodash');
var async = require('async');
var neo_async = require('neo-async');
var start = function() {
return process.hrtime();
};
var getDiff = function(start) {
var diff = process.hrtime(start);
return diff[0] * 1e9 + diff[1];
};
var sample = 1000;
var time = {
async: 0,
neo_async: 0
};
var tasks = _.map(_.times(sample), function(item) {
return function(callback) {
callback(null, item);
};
});
var timer = start();
async.series(tasks, function(err, res1) {
time.async = getDiff(timer);
timer = start();
neo_async.series(tasks, function(err, res2) {
time.neo_async = getDiff(timer);
var check = _.every(res1, function(item, index) {
return res2[index] === item;
});
console.log('check', check);
console.log('**** time ****');
console.log(time.async - time.neo_async);
});
});
var tasks = _.map(_.times(sample), function(item) {
return function(callback) {
callback(null, item);
};
});
var timer = start();
neo_async.parallel(tasks, function(err, res1) {
time.neo_async = getDiff(timer);
timer = start();
async.parallel(tasks, function(err, res2) {
time.async = getDiff(timer);
var check = _.every(res1, function(item, index) {
return res2[index] === item;
});
console.log('check', check);
console.log('**** time ****');
console.log(time.async - time.neo_async);
});
});
var tasks = (function createSimpleTasks(num) {
var first = true;
var tasks = _.transform(_.times(num), function(memo, num, key) {
if (first) {
first = false;
memo[key] = function(done) {
done(null, num);
};
} else {
memo[key] = function(sum, done) {
done(null, sum + num);
};
}
});
return tasks;
})(sample);
var timer = start();
async.waterfall(tasks, function(err, res1) {
time.neo_async = getDiff(timer);
timer = start();
async.waterfall(tasks, function(err, res2) {
time.async = getDiff(timer);
console.log('check', res1 === res2);
console.log('**** time ****');
console.log(time.async - time.neo_async);
});
});
FAQs
Neo-Async is a drop-in replacement for Async, it almost fully covers its functionality and runs faster
The npm package neo-async receives a total of 31,314,824 weekly downloads. As such, neo-async popularity was classified as popular.
We found that neo-async demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.