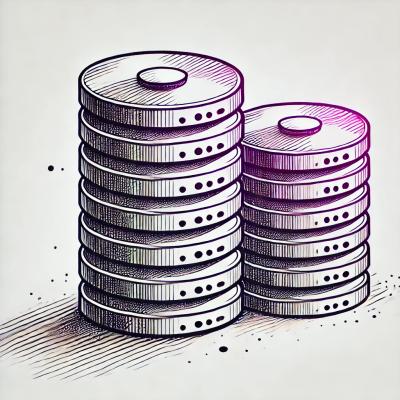
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
nestjs-graphql-connection
Advanced tools
Relay-style pagination for NestJS GraphQL server.
npm i nestjs-graphql-connection
Extend a class from createEdgeType
function, passing it the class of objects to be represented by the edge's node
.
For correct typings also indicate that your class implements EdgeInterface
, as shown:
import { ObjectType } from '@nestjs/graphql';
import { createEdgeType, EdgeInterface } from 'nestjs-graphql-connection';
import { Person } from './entities';
@ObjectType()
export class PersonEdge extends createEdgeType(Person) implements EdgeInterface<Person> {}
Extend a class from createConnectionType
function, passing it the class of objects to be represented by the
connection's edges
:
import { ObjectType } from '@nestjs/graphql';
import { createConnectionType } from 'nestjs-graphql-connection';
@ObjectType()
export class PersonConnection extends createConnectionType(PersonEdge) {}
Extend a class from ConnectionArgs
class to have pagination arguments pre-defined for you. You can additionally
define your own arguments for filtering, etc.
import { ArgsType, Field, ID } from '@nestjs/graphql';
import { ConnectionArgs } from 'nestjs-graphql-connection';
@ArgsType()
export class PersonConnectionArgs extends ConnectionArgs {
/*
* PersonConnectionArgs will inherit `first`, `last`, `before`, `after`, and `page` fields from ConnectionArgs
*/
// Optional: example of a custom argument for filtering
@Field(type => ID, { nullable: true })
public personId?: string;
}
Your resolvers can return a Connection
as an object type. Use a Paginator
class to help you determine which page
of results to fetch and to create PageInfo
and cursors in the result.
With offset pagination, cursor values are an encoded representation of the row offset. It is possible for clients to
paginate by specifying either an after
argument with the cursor of the last row on the previous page, or to pass a
page
argument with an explicit page number (based on the rows per page set by the first
argument).
import { Query, Resolver } from '@nestjs/graphql';
import { OffsetCursorPaginator } from 'nestjs-graphql-connection';
@Resolver()
export class PersonQueryResolver {
@Query(returns => PersonConnection)
public async persons(@Args() connectionArgs: PersonConnectionArgs): Promise<PersonConnection> {
const { personId } = connectionArgs;
// Example: Count the total number of matching persons (ignoring pagination)
const totalPersons = await countPersons({ where: { personId } });
// Create paginator instance
const paginator = OffsetCursorPaginator.createFromConnectionArgs(connectionArgs, totalPersons);
// Example: Do whatever you need to do to fetch the current page of persons
const persons = await fetchPersons({
where: { personId },
take: paginator.take, // how many rows to fetch
skip: paginator.skip, // row offset to fetch from
});
// Return resolved PersonConnection with edges and pageInfo
return new PersonConnection({
pageInfo: paginator.createPageInfo(persons.length),
edges: persons.map((node, index) => {
return new PersonEdge({
node,
cursor: paginator.createCursor(index).encode(),
});
}),
});
}
}
🚧 WIP 🚧
Cursors are ready but there is no Paginator class implementation yet.
FAQs
Relay-style pagination for NestJS GraphQL server.
The npm package nestjs-graphql-connection receives a total of 8,866 weekly downloads. As such, nestjs-graphql-connection popularity was classified as popular.
We found that nestjs-graphql-connection demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.