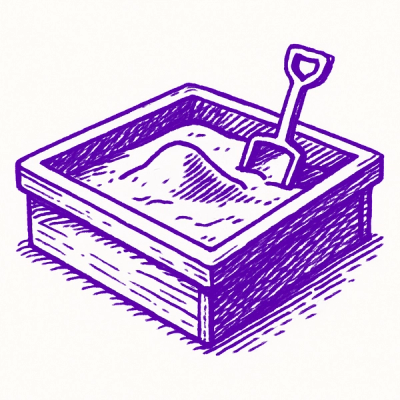
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
nestjs-pgpromise
Advanced tools
pg-promise Module for Nest framework
This's a nest-pgpromise module for Nest. This quickstart guide will show you how to install and execute an example nestjs program..
This document assumes that you have a working nodejs setup in place.
npm install --save nestjs-pgpromise
You need five items in order to connect to the PostgreSQL server.
Params | Description |
---|---|
host | Host IP address or URL. |
port | TCP/IP port number to access the database. |
database | The name of the database to connect to. |
user | The username to access the database. |
password | The username's password to access the database. |
And you can use as well all the other parameters allowed by pg-promise
package. See the documentation.
Provide the credentials for pg-promise module by importing it as :
import { Module } from '@nestjs/common';
import { NestPgpromiseClientController } from './nest-pgpromise-client.controller';
import { NestPgpromiseModule } from 'nestjs-pgpromise';
@Module({
controllers: [NestPgpromiseClientController],
imports: [
NestPgpromiseModule.register({
isGlobal: true,
connection: {
host: 'localhost',
port: 5432,
database: 'cmdbbtbi',
user: 'cmadbbtbi',
password: 'cghQZynG0whwtGki-ci2bpxV5Jw_5k6z',
},
}),
],
})
export class AppModule {}
import { Module } from '@nestjs/common';
import { NestPgpromiseClientController } from './nest-pgpromise-client.controller';
import { NestPgpromiseModule } from 'nestjs-pgpromise';
@Module({
controllers: [NestPgpromiseClientController],
imports: [
NestPgpromiseModule.register(
isGlobal: true,
{
connection: "postgres://YourUserName:YourPassword@YourHost:5432/YourDatabase"
}),
],
})
export class AppModule {}
Then you can use it in the controller or service by injecting it in the controller as:
constructor(@Inject(NEST_PGPROMISE_CONNECTION) private readonly pg: IDatabase<any>) {}
This example program connects to postgres on localhost and executes a simple select
query from table tasks
.
import { Controller, Get, Inject, Logger } from '@nestjs/common';
import { NEST_PGPROMISE_CONNECTION } from 'nestjs-pgpromise';
import { IDatabase } from 'pg-promise';
@Controller()
export class NestPgpromiseClientController {
private logger = new Logger('controller');
constructor(@Inject(NEST_PGPROMISE_CONNECTION) private readonly pg: IDatabase<any>) {}
@Get()
async index() {
this.pg
.any('SELECT * FROM task')
.then(data => {
// success;
this.logger.log(data);
})
.catch(error => {
// error;
this.logger.log(error);
});
}
}
As pg-promise
methods return promises, the new async/await
syntaxis can be used.
import { Controller, Get, Inject, Logger } from '@nestjs/common';
import { NEST_PGPROMISE_CONNECTION } from 'nestjs-pgpromise';
import { IDatabase } from 'pg-promise';
@Controller()
export class NestPgpromiseClientController {
private logger = new Logger('controller');
constructor(@Inject(NEST_PGPROMISE_CONNECTION) private readonly pg: IDatabase<any>) {}
@Get()
async index() {
try {
const data = await this.pg.any('SELECT * FROM task');
// success;
this.logger.log(data);
} catch(e) {
// error;
this.logger.log(error);
}
}
}
You can also pass in initoptions
as supported by pg-promise.
import { Module } from '@nestjs/common';
import { NestPgpromiseClientController } from './nest-pgpromise-client.controller';
import { NestPgpromiseModule } from 'nestjs-pgpromise';
@Module({
controllers: [NestPgpromiseClientController],
imports: [
NestPgpromiseModule.register(
isGlobal: true,
{
connection: {
host: 'localhost',
port: 5432,
database: 'cmdbbtbi',
user: 'cmadbbtbi',
password: 'cghQZynG0whwtGki-ci2bpxV5Jw_5k6z',
},
initOptions:{/* initialization options */};
}),
],
})
export class AppModule {}
Note: You can then access the underlying PGP object through the $config property, for example:
new this.pg.$config.pgp.helpers.ColumnSet(['col1', 'col2']);
You can find the details about them in the pg-promise documentation
Thanks goes to these wonderful people (emoji key):
Vitaly Tomilov 🚇 ⚠️ 💻 | Matthew J. Clemente 🚇 ⚠️ 💻 | Jason Santiago 📖 | Hector 📖 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
pg-promise module for nestjs
The npm package nestjs-pgpromise receives a total of 474 weekly downloads. As such, nestjs-pgpromise popularity was classified as not popular.
We found that nestjs-pgpromise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.