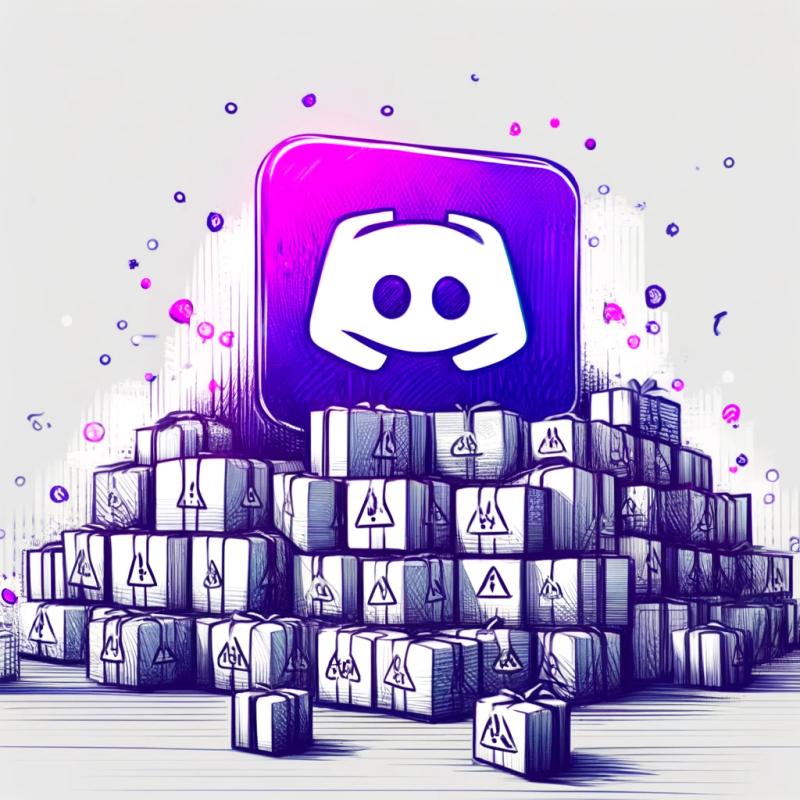
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
new-github-issue-url
Advanced tools
Readme
Generate a URL for opening a new GitHub issue with prefilled title, body, and other fields
GitHub supports prefilling a new issue by setting certain search parameters. This package simplifies generating such URL.
npm install new-github-issue-url
import newGithubIssueUrl from 'new-github-issue-url';
import open from 'open';
const url = newGithubIssueUrl({
user: 'sindresorhus',
repo: 'new-github-issue-url',
body: '\n\n\n---\nI\'m a human. Please be nice.'
});
//=> 'https://github.com/sindresorhus/new-github-issue-url/issues/new?body=%0A%0A%0A---%0AI%27m+a+human.+Please+be+nice.'
// Then open it
await open(url);
Try the URL
(Don't click the "Submit new issue" button!)
If you use Electron, check out electron-util
's openNewGitHubIssue()
method.
Returns a URL string.
Type: object
You are required to either specify the repoUrl
option or both the user
and repo
options.
Type: string
The full URL to the repo.
Type: string
GitHub username or organization.
Type: string
GitHub repo.
Type: string
The issue body.
Type: string
The issue title.
Type: string
Use an issue template.
For example, if you want to use a template at ISSUE_TEMPLATE/unicorn.md
, you would specify unicorn.md
here.
Type: string[]
The labels for the issue.
Requires the user to have the permission to add labels.
Type: string
The milestone for the issue.
Requires the user to have the permission to add milestone.
Type: string
The user to assign to the issue.
Requires the user to have the permission to add assignee.
Type: string[]
The projects to add the issue to.
The project reference format is user/repo/<project-number>
, for example, if the URL to the project is https://github.com/sindresorhus/some-repo/projects/3
, the project reference would be sindresorhus/some-repo/3
.
Requires the user to have the permission to add projects.
FAQs
Generate a URL for opening a new GitHub issue with prefilled title, body, and other fields
We found that new-github-issue-url demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.