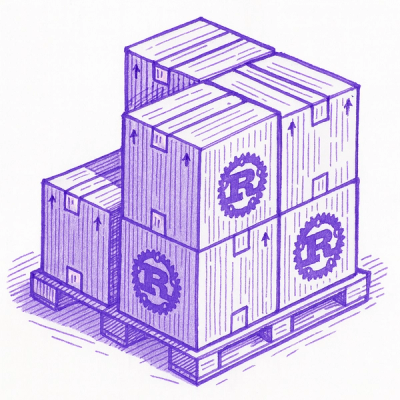
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
newscatcherapi-typescript-sdk
Advanced tools
Visit our website https://newscatcherapi.com
newscatcher.authors.get
newscatcher.authors.post
newscatcher.latestHeadlines.get
newscatcher.latestHeadlines.post
newscatcher.search.get
newscatcher.search.post
newscatcher.searchLink.get
newscatcher.searchLink.post
newscatcher.searchSimilar.get
newscatcher.searchSimilar.post
newscatcher.sources.get
newscatcher.sources.post
newscatcher.subscription.get
newscatcher.subscription.post
npm | pnpm | yarn |
---|---|---|
|
|
|
import { Newscatcher } from "newscatcherapi-typescript-sdk";
const newscatcher = new Newscatcher({
// Defining the base path is optional and defaults to https://v3-api.newscatcherapi.com
// basePath: "https://v3-api.newscatcherapi.com",
apiKey: "API_KEY",
});
const getResponse = await newscatcher.authors.get({
authorName: "authorName_example",
byParseDate: false,
sortBy: "relevancy",
page: 1,
pageSize: 100,
});
console.log(getResponse);
newscatcher.authors.get
This endpoint allows you to search for articles by author. You need to specify the author name. You can also filter by language, country, source, and more.
const getResponse = await newscatcher.authors.get({
authorName: "authorName_example",
byParseDate: false,
sortBy: "relevancy",
page: 1,
pageSize: 100,
});
string
string
any
any
any
any
any
any
any
From
To
string
boolean
string
RankedOnly
number
number
boolean
boolean
boolean
any
any
any
number
number
number
number
boolean
boolean
string
string
number
number
number
number
any
any
any
any
/api/authors
GET
newscatcher.authors.post
This endpoint allows you to search for articles by author. You need to specify the author name. You can also filter by language, country, source, and more.
const postResponse = await newscatcher.authors.post({
author_name: "author_name_example",
by_parse_date: false,
sort_by: "relevancy",
page: 1,
page_size: 100,
});
string
string
any
any
any
any
any
any
any
From
To
string
boolean
string
RankedOnly
number
number
boolean
boolean
boolean
any
any
any
number
number
number
number
boolean
boolean
string
string
number
number
number
number
any
any
any
any
/api/authors
POST
newscatcher.latestHeadlines.get
This endpoint allows you to get latest headlines. You need to specify since when you want to get the latest headlines. You can also filter by language, country, source, and more.
const getResponse = await newscatcher.latestHeadlines.get({
when: "7d",
byParseDate: false,
sortBy: "relevancy",
page: 1,
pageSize: 100,
});
string
boolean
string
any
any
any
any
any
any
any
any
RankedOnly
boolean
boolean
boolean
any
any
any
number
number
number
number
string
boolean
number
boolean
boolean
string
string
string
string
string
string
number
number
number
number
any
any
any
any
/api/latest_headlines
GET
newscatcher.latestHeadlines.post
This endpoint allows you to get latest headlines. You need to specify since when you want to get the latest headlines. You can also filter by language, country, source, and more.
const postResponse = await newscatcher.latestHeadlines.post({
when: "7d",
by_parse_date: false,
sort_by: "relevancy",
page: 1,
page_size: 100,
});
string
boolean
string
any
any
any
any
any
any
any
any
RankedOnly
boolean
boolean
boolean
any
any
any
number
number
number
number
string
boolean
number
boolean
boolean
string
string
string
string
string
string
number
number
number
number
any
any
any
any
/api/latest_headlines
POST
newscatcher.search.get
This endpoint allows you to search for articles. You can search for articles by keyword, language, country, source, and more.
const getResponse = await newscatcher.search.get({
q: "q_example",
searchIn: "title_content",
byParseDate: false,
sortBy: "relevancy",
page: 1,
pageSize: 100,
});
string
string
any
any
any
any
any
any
any
any
From
To
string
boolean
string
RankedOnly
number
number
boolean
boolean
boolean
any
any
any
number
number
number
number
string
boolean
number
boolean
boolean
string
string
string
string
string
string
number
number
number
number
any
any
any
any
any
boolean
boolean
boolean
any
any
/api/search
GET
newscatcher.search.post
This endpoint allows you to search for articles. You can search for articles by keyword, language, country, source, and more.
const postResponse = await newscatcher.search.post({
q: "q_example",
search_in: "title_content",
by_parse_date: false,
sort_by: "relevancy",
page: 1,
page_size: 100,
});
string
string
any
any
any
any
any
any
any
any
From
To
string
boolean
string
RankedOnly
number
number
boolean
boolean
boolean
any
any
any
number
number
number
number
string
boolean
number
boolean
boolean
string
string
string
string
string
string
number
number
number
number
any
any
any
any
any
boolean
boolean
boolean
any
any
/api/search
POST
newscatcher.searchLink.get
This endpoint allows you to search for articles. You can search for articles by id(s) or link(s).
const getResponse = await newscatcher.searchLink.get({
page: 1,
pageSize: 100,
});
any
any
From1
To1
number
number
DtoResponsesSearchResponseSearchResponse
/api/search_by_link
GET
newscatcher.searchLink.post
This endpoint allows you to search for articles. You can search for articles by id(s) or link(s).
const postResponse = await newscatcher.searchLink.post({
page: 1,
page_size: 100,
});
any
any
From1
To1
number
number
DtoResponsesSearchResponseSearchResponse
/api/search_by_link
POST
newscatcher.searchSimilar.get
This endpoint returns a list of articles that are similar to the query provided. You also have the option to get similar articles for the results of a search.
const getResponse = await newscatcher.searchSimilar.get({
q: "q_example",
searchIn: "title_content",
includeSimilarDocuments: false,
similarDocumentsNumber: 5,
similarDocumentsFields: "title,content",
byParseDate: false,
sortBy: "relevancy",
page: 1,
pageSize: 100,
});
string
string
boolean
number
string
any
any
any
any
any
any
any
From
To
boolean
string
string
RankedOnly
number
number
boolean
boolean
boolean
any
any
any
number
number
number
number
boolean
boolean
string
string
number
number
number
number
any
any
/api/search_similar
GET
newscatcher.searchSimilar.post
This endpoint returns a list of articles that are similar to the query provided. You also have the option to get similar articles for the results of a search.
const postResponse = await newscatcher.searchSimilar.post({
q: "q_example",
search_in: "title_content",
include_similar_documents: false,
similar_documents_number: 5,
similar_documents_fields: "title,content",
by_parse_date: false,
sort_by: "relevancy",
page: 1,
page_size: 100,
});
string
string
boolean
number
string
any
any
any
any
any
any
any
From
To
boolean
string
string
RankedOnly
number
number
boolean
boolean
boolean
any
any
any
number
number
number
number
boolean
boolean
string
string
number
number
number
number
any
any
/api/search_similar
POST
newscatcher.sources.get
This endpoint allows you to get the list of sources that are available in the database. You can filter the sources by language and country. The maximum number of sources displayed is set according to your plan. You can find the list of plans and their features here: https://newscatcherapi.com/news-api#news-api-pricing
const getResponse = await newscatcher.sources.get({});
any
any
any
boolean
number
number
any
any
boolean
any
any
/api/sources
GET
newscatcher.sources.post
This endpoint allows you to get the list of sources that are available in the database. You can filter the sources by language and country. The maximum number of sources displayed is set according to your plan. You can find the list of plans and their features here: https://newscatcherapi.com/news-api#news-api-pricing
const postResponse = await newscatcher.sources.post({});
any
any
any
boolean
number
number
any
any
boolean
any
any
/api/sources
POST
newscatcher.subscription.get
This endpoint allows you to get info about your subscription plan.
const getResponse = await newscatcher.subscription.get();
/api/subscription
GET
newscatcher.subscription.post
This endpoint allows you to get info about your subscription plan.
const postResponse = await newscatcher.subscription.post();
/api/subscription
POST
This TypeScript package is automatically generated by Konfig
FAQs
Client for NewsCatcher-V3 Production API
The npm package newscatcherapi-typescript-sdk receives a total of 1 weekly downloads. As such, newscatcherapi-typescript-sdk popularity was classified as not popular.
We found that newscatcherapi-typescript-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.