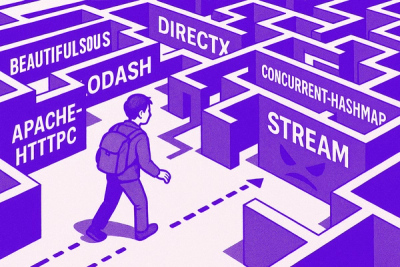
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
next-cookies-universal
Advanced tools
An utility that can help you to handle the Cookies in NextJS App Route with every context (both Server or Client) 🍪🔥
An utility that can help you to handle the Cookies in NextJS App Route with every context (both Server or Client) 🍪🔥
All supported to NextJS App Route
You can see Live Demo here
npm i next-cookies-universal
yarn add next-cookies-universal
import Cookies from 'next-universal-cookies';
const ServerCookies = Cookies('server');
// or
const ClientCookies = Cookies('client');
'use client';
import Cookies from 'next-universal-cookies';
const MyClientComponent = () => {
const cookies = Cookies('client');
const handleClick = () => {
cookies.set('my_token', 'my_token_value');
};
return (
<button onClick={handleClick}>
Click to set cookies
</button>
);
};
import Cookies from 'next-universal-cookies';
const MyServerComponent = async() => {
const cookies = Cookies('server');
const myToken = cookies.get('my_token');
const data = await fetch('http://your.endpoint', {
headers: {
Authentication: `Bearer ${myToken}`
}
}).then(response => response.json());
return (
<div>
<p>Cookies Value: <strong>{myToken}</strong></p>
<code>
{JSON.stringify(data)}
</code>
</div>
);
};
Note: if you want to set cookies in Server, you not to allowed to set it on Server Component, you should do that in Server Actions.
import Cookies from 'next-universal-cookies';
const MyServerComponent = async() => {
const cookies = Cookies('server');
/** you should not to do like this!
* please read Server Actions reference if you want to set the cookies through Server.
*/
cookies.set('my_token', 'my_token_value');
const myToken = cookies.get('my_token');
return (
<div>
<p>Cookies Value: <strong>{myToken}</strong></p>
<code>
{JSON.stringify(data)}
</code>
</div>
);
};
import Cookies from 'next-cookies-universal';
async function setFromAction(formData: FormData) {
'use server';
const cookies = Cookies('server');
cookies.set('my_token', formData.get('cookie-value'));
}
function Form() {
return (
<div>
<form action={setFromAction}>
<input type="text" name="cookie-value" />
<div>
<button type="submit">
Set Your cookies
</button>
</div>
</form>
</div>
);
}
/** action.ts */
'use server';
export async function setFromAction(formData: FormData) {
const cookies = Cookies('server');
cookies.set('my_token', formData.get('cookie-value'));
}
/** Form.tsx */
'use client';
import { setFromAction } from './action.ts';
function Form() {
/** client logic */
return (
<div>
<form action={setFromAction}>
<input type="text" name="cookie-value" />
<div>
<button type="submit">
Set Your cookies
</button>
</div>
</form>
</div>
);
}
/** parameter to initialize the Cookies() */
export type ICookiesContext = 'server'|'client';
/** both Cookies('client') and Cookies('server') implements this interface */
export interface IBaseCookies {
set<T = string>(
key: string,
value: T,
options?: ICookiesOptions
): void;
get<T = string>(key: string): T;
remove(key: string, options?: ICookiesOptions): void;
has(key: string): boolean;
clear(): void;
}
for ICookiesOptions
API, we use CookieSerializeOptions
from DefinetlyTyped
version
in package.json
is changed to newest version. Then run npm install
for synchronize it to package-lock.json
main
, you can publish the packages by creating new Relase here: https://github.com/gadingnst/next-cookies-universal/releases/newtag
, make sure the tag
name is same as the version
in package.json
.Publish Release
button, then wait the package to be published.next-cookies-universal
is freely distributable under the terms of the MIT license.
Feel free to open issues if you found any feedback or issues on next-cookies-universal
. And feel free if you want to contribute too! 😄
Built with ❤️ by Sutan Gading Fadhillah Nasution on 2023
FAQs
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.