nf-photo-collage
Advanced tools
Comparing version 1.1.13 to 1.2.0
export type CollageOptions = { | ||
sources: string[]; | ||
sources: string|Buffer[]; | ||
width: number; | ||
@@ -4,0 +4,0 @@ height: number; |
46
index.js
@@ -37,24 +37,2 @@ "use strict"; | ||
function wrapText(context, text, x, y, maxWidth, lineHeight) { | ||
var words = text.split(' '); | ||
var line = ''; | ||
let initialY = y; | ||
for(var n = 0; n < words.length; n++) { | ||
var testLine = line + words[n] + ' '; | ||
var metrics = context.measureText(testLine); | ||
var testWidth = metrics.width; | ||
if (testWidth > maxWidth && n > 0) { | ||
context.fillText(line, x, y); | ||
line = words[n] + ' '; | ||
y += lineHeight; | ||
} | ||
else { | ||
line = testLine; | ||
} | ||
} | ||
context.fillText(line, x, y); | ||
return y - initialY + lineHeight; // height used | ||
} | ||
const PARAMS = [ | ||
@@ -68,5 +46,3 @@ {field: "sources", required: true}, | ||
{field: "backgroundColor", default: "#eeeeee"}, | ||
{field: "backgroundImage", default: ""}, | ||
{field: "lines", default: []}, | ||
{field: "textStyle", default: {}}, | ||
{field: "backgroundImage", default: ""} | ||
]; | ||
@@ -89,6 +65,4 @@ | ||
const headerHeight = (options.header || {}).height || 0; | ||
const canvasWidth = options.width * options.imageWidth + (options.width - 1) * (options.spacing); | ||
const canvasHeight = headerHeight + options.height * options.imageHeight + (options.height - 1) * (options.spacing); | ||
console.log("Created canvas" + " - W:" + canvasWidth + " H:" + canvasHeight) | ||
const canvasHeight = options.height * options.imageHeight + (options.height - 1) * (options.spacing); | ||
const canvas = createCanvas(canvasWidth, canvasHeight); | ||
@@ -136,18 +110,4 @@ | ||
}) | ||
.then(() => { | ||
// if (options.text) { | ||
// ctx.font = (options.textStyle.fontSize || "20") + "px " + (options.textStyle.font || "Helvetica"); | ||
// wrapText(ctx, options.text, 10, canvasHeight - (options.textStyle.height || 200) + 50, canvasWidth - 10, (options.textStyle.fontSize || 20) * 1.2); | ||
// } | ||
// else { | ||
// let curHeight = 150; | ||
// options.lines.map((line) => { | ||
// ctx.font = line.font || "20px Helvetica"; | ||
// ctx.fillStyle = line.color || "#333333"; | ||
// const heightUsed = wrapText(ctx, line.text, 10, canvasHeight - curHeight, canvasWidth - 10, (parseInt(line.font) || 20) * 1.2); | ||
// curHeight -= heightUsed; | ||
// }); | ||
// } | ||
}) | ||
.then(() => {}) | ||
.return(canvas); | ||
}; |
{ | ||
"name": "nf-photo-collage", | ||
"version": "1.1.13", | ||
"version": "1.2.0", | ||
"publishConfig": { | ||
@@ -5,0 +5,0 @@ "access": "public" |
@@ -1,27 +0,21 @@ | ||
# photo-collage | ||
# nf-photo-collage | ||
Combines several images into a photo collage. | ||
## Example | ||
This is slightly restructured version of original [photo-collage](https://github.com/classdojo/photo-collage) repo. It is also using [RectangleEquals fork](https://github.com/RectangleEquals/photo-collage) so it is using Canvas v2. | ||
#### Source files | ||
 | ||
 | ||
 | ||
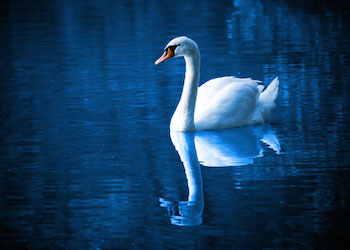 | ||
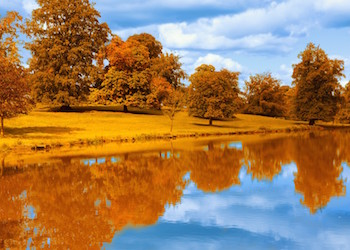 | ||
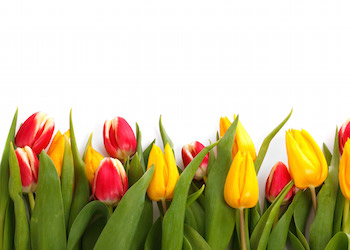 | ||
### Changes | ||
- Removed header and support for adding text | ||
- Add support for adding image as background | ||
- Add typings (`index.d.ts`) | ||
#### Result | ||
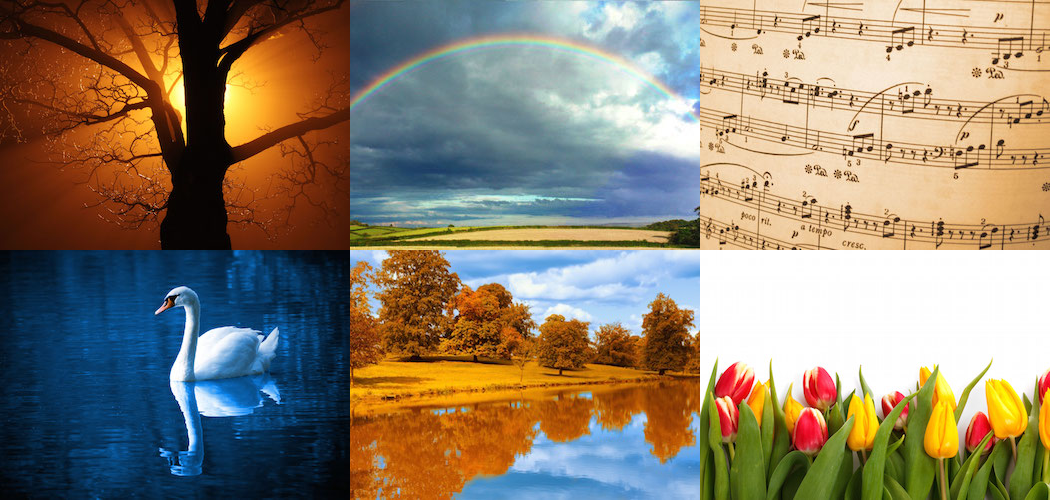 | ||
## Installation | ||
`npm install --save photo-collage` | ||
`yarn add nf-photo-collage` | ||
This library depends on `node-canvas`, which may require additional setup. See [their installation page](https://github.com/Automattic/node-canvas/wiki/_pages) for details. | ||
This library depends on `node-canvas` (v2), which may require additional setup. See [their installation page](https://github.com/Automattic/node-canvas/wiki/_pages) for details. | ||
## Usage | ||
The following example creates a 2x3 collage from a variety of image sources. | ||
```js | ||
const createCollage = require("photo-collage"); | ||
```ts | ||
import createCollage from "nf-photo-collage"; | ||
@@ -42,13 +36,4 @@ const options = { | ||
// backgroundColor: "#cccccc", // optional, defaults to #eeeeee. | ||
backgroundImage: "./localfile.png" // same formats supported as source | ||
spacing: 2, // optional: pixels between each image | ||
lines: [ | ||
{font: "", color: "", text: "Sometimes we want to find out when a single one time event has"}, | ||
{font: "", color: "", text: "Sometimes we want to find out when a single one time event has"}, | ||
{font: "", color: "", text: "Sometimes we want to find out when a single one time event has"}, | ||
{font: "", color: "", text: "Sometimes we want to find out when a single one time event has"}, | ||
{font: "", color: "", text: "Sometimes we want to find out when a single one time event has"}, | ||
], | ||
//text: "Sometimes we want to find out when a single one time event has finished. For example - a stream is done. For this we can use new Promise. Note that this option should be considered only if automatic conversion isn't possible.Note that promises model a single value through time, they only resolve once - so while they're a good fit for a single event, they are not recommended for multiple event APIs." | ||
//textStyle: {color: "#fff", fontSize: 20, font: "Arial", height: 300} | ||
// we can use either lines or text (text will be warped) | ||
}; | ||
@@ -63,1 +48,14 @@ | ||
``` | ||
## Example | ||
#### Source files | ||
 | ||
 | ||
 | ||
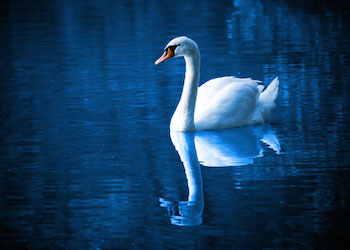 | ||
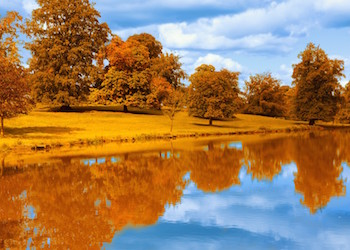 | ||
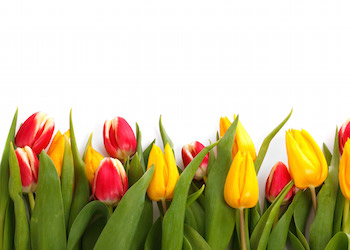 | ||
#### Result | ||
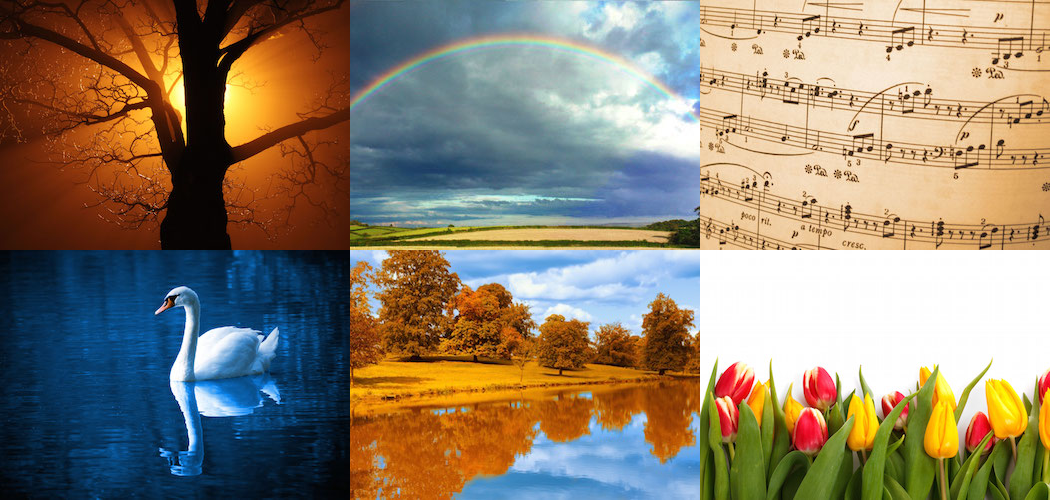 |
10343
155
60