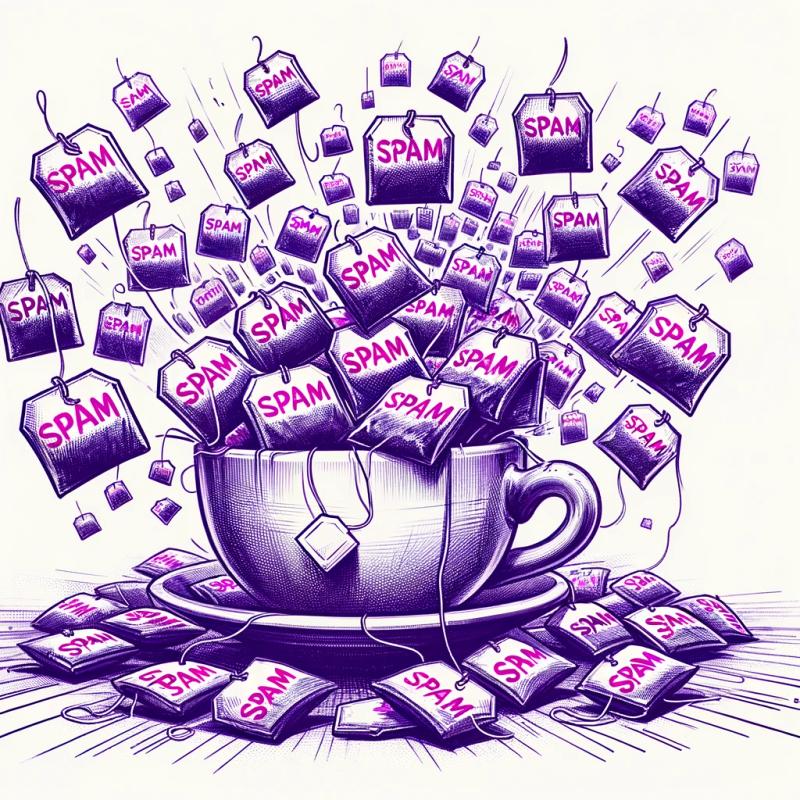
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ng-authentication-service
Advanced tools
Readme
An authentication and authorization helper service for Angular client applications.
Add this module to your app as a dependency:
var app = angular.module('yourApp', ['authentication.service']);
Inject $authentication as a parameter in declarations that require it:
app.controller('yourController', function($scope, $authentication){ ... });
To override the default configuration options, configure the module with an options argument during application configuration and provide overrides for any of the following options.
app.config(['$authenticationProvider', function ($authenticationProvider) {
$authenticationProvider.configure({
authCookieKey: undefined,
storageService: undefined,
profileStorageKey: '$authentication.user.profile',
lastAttemptedUrlStorageKey: '$authentication.last-attempted-url',
onLoginRedirectUrl: '/',
onLogoutRedirectUrl: '/',
notAuthorizedRedirectUrl: '/',
notAuthenticatedRedirectUrl: '/',
trackLastAttemptedUrl: true,
userRolesProperty: 'roles',
extensions: undefined,
expirationProperty: undefined,
events: {
loginConfirmed: 'event:auth-loginConfirmed',
loginRequired: 'event:auth-loginRequired',
logoutConfirmed: 'event:auth-logoutConfirmed',
notAuthenticated: 'event:auth-notAuthenticated',
notAuthorized: 'event:auth-notAuthorized'
},
rolesFunction: function (userProfile) {
if (_.has(userProfile, this.userRolesProperty)) {
var roles = userProfile[this.userRolesProperty];
return _.isArray(roles) ? roles : [roles];
}
return [];
},
validationFunction: function (userRoles, allowedRoles) {
return !_.isEmpty(userRoles) && !_.isEmpty(allowedRoles) &&
(_.find(allowedRoles, function (role) { return _.includes(userRoles, role); }) !== undefined);
},
reauthentication: {
fn: function () {},
timeout: 1200000,
timer: undefined
}
});
}]);
All properties (own and inherited) of the extensions object will be available as native to the $authentication service API. The extensions object is applied using the _.defaults(...) method and cannot overwrite any of the existing API properties. This is intended to provide implementors with a way to add objects or functions that are application specific and should fall within the context of the authentication service to expose, e.g., functions to check if a profile has specific roles.
If you do not provide a storage service then a simple, in-memory dictionary will be used.
You can provide any storage service or object that supports the following API:
any get(key)
boolean has(key)
void remove(key)
void set(key, value)
To configure a storage service for the authentication provider you provide the service name:
app.config(['$authenticationProvider', function ($authenticationProvider) {
$authenticationProvider.configure({
storageService: '$store'
});
}]);
or an object that provides the expected functionality:
app.config(['$authenticationProvider', function ($authenticationProvider) {
$authenticationProvider.configure({
storageService: new CustomStorageService()
});
}]);
The ng-authentication-service was designed in tandem with the ng-local-storage-service.
// Returns true if there is a user profile loaded in local storage, false otherwise.
$authentication.isAuthenticated();
// Returns true if the authCookieKey is defined and no auth cookie is present, false otherwise.
$authentication.isAuthCookieMissing();
// Returns true if there is a user profile and it has an expiration value in the past, false otherwise.
$authentication.isProfileExpired();
// Store the profile (data) in local storage, notify all listeners of login, and redirect to:
// 1. lastAttemptedUrl if defined and trackLastAttemptedUrl is true
// 2. onLoginRedirectUrl if defined
// 3. do not redirect
$authentication.loginConfirmed({ ... });
Broadcast via: event:auth-loginConfirmed
with the data
parameter as an argument.
// Check whether a user is logged in and broadcast the auth-loginConfirmed event, if so.
$authentication.checkAndBroadcastLoginConfirmed();
// Notify all listeners that authentication is required.
$authentication.loginRequired();
Broadcast via: event:auth-loginRequired
.
// Remove any existing profile from local storage, notify all listeners of logout, and redirect to:
// 1. do not redirect if @doNotRedirect === true
// 2. lastAttemptedUrl if defined and trackLastAttemptedUrl is true
// 3. onLogoutRedirectUrl if defined
// 4. do not redirect
$authentication.logoutConfirmed();
Broadcast via: event:auth-logoutConfirmed
.
// Return true if the user is unauthenticated, false otherwise.
$authentication.allowed('anonymous');
// Return true if the user is authenticated, false otherwise.
$authenticated.allowed('all');
// Return true if the configured validationFunction returns true, false otherwise.
$authenticated.allowed('role1', 'role2', ...);
// will flatten provided arrays that are any depth in the arguments list
$authentication.allowed('X', ['Y', 'Z'], [['A']]) === $authentication.allowed('X', 'Y', 'Z', 'A')
// Get or set the current user profile from local storage.
// If data is provided then it overwrites the existing user profile before returning it.
$authentication.profile(data);
// Return the current collection of roles of the user profile from local storage if it exists.
$authentication.roles();
// Return true if the current user profile is in all of the specified roles, false otherwise.
$authentication.isInAllRoles('role1', 'role2', ...);
// will flatten provided arrays that are any depth in the arguments list
$authentication.isInAllRoles('X', ['Y', 'Z'], [['A']]) === $authentication.isInAllRoles('X', 'Y', 'Z', 'A')
// Return true if the current user profile is in at least one of the specified roles, false otherwise.
$authentication.isInAnyRoles('role1', 'role2', ...);
// will flatten provided arrays that are any depth in the arguments list
$authentication.isInAnyRoles('X', ['Y', 'Z'], [['A']]) === $authentication.isInAnyRoles('X', 'Y', 'Z', 'A')
// Determine if the current user profile is allowed and redirect to either notAuthorizedRedirectUrl or notAuthenticatedRedirectUrl if not.
$authentication.permit('role1', 'role2', ...);
// will flatten provided arrays that are any depth in the arguments list
$authentication.permit('X', ['Y', 'Z'], [['A']]) === $authentication.permit('X', 'Y', 'Z', 'A')
// Return the configuration object.
$authentication.getConfiguration();
// Return the last attempted url value, or fallback if value is undefined or tracking is disabled,.
$authentication.getLastAttemptedUrl();
// Set and return the last attempted url value.
$authentication.setLastAttemptedUrl();
// Returns the configured storage service.
$authentication.store();
// Enable re-authentication via the configured reauthentication.fn at reauthentication.timeout intervals.
$authentication.reauthenticate();
// Sets the provided function handler as a listener to the event: 'event:auth-loginConfirmed'.
// The event data is the data provided to the loginConfirmed call that triggered this event.
$authentication.$onLoginConfirmed(function (event, data) { ... });
// Sets the provided function handler as a listener to the event: 'event:auth-loginRequired'.
$authentication.$onLoginRequired(function (event) { ... });
// Sets the provided function handler as a listener to the event: 'event:auth-logoutConfirmed'.
$authentication.$onLogoutConfirmed(function (event) { ... });
// Sets the provided function handler as a listener to the event: 'event:auth-notAuthenticated'.
// The event data is the array of arguments provided to the permit call that triggered this event.
$authentication.$onNotAuthenticated(function (event, data) { ... });
// Sets the provided function handler as a listener to the event: 'event:auth-notAuthorized'.
// The event data is the array of arguments provided to the permit call that triggered this event.
$authentication.$onNotAuthorized(function (event, data) { ... });
After forking you should only have to run npm install
from a command line to get your environment setup.
After install you have two gulp commands available to you:
gulp js:lint
gulp js:test
FAQs
[](https://github.com/justinsa/angular-authentication-service) [](https://www.npmjs.com/package/ng-aut
The npm package ng-authentication-service receives a total of 4 weekly downloads. As such, ng-authentication-service popularity was classified as not popular.
We found that ng-authentication-service demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.