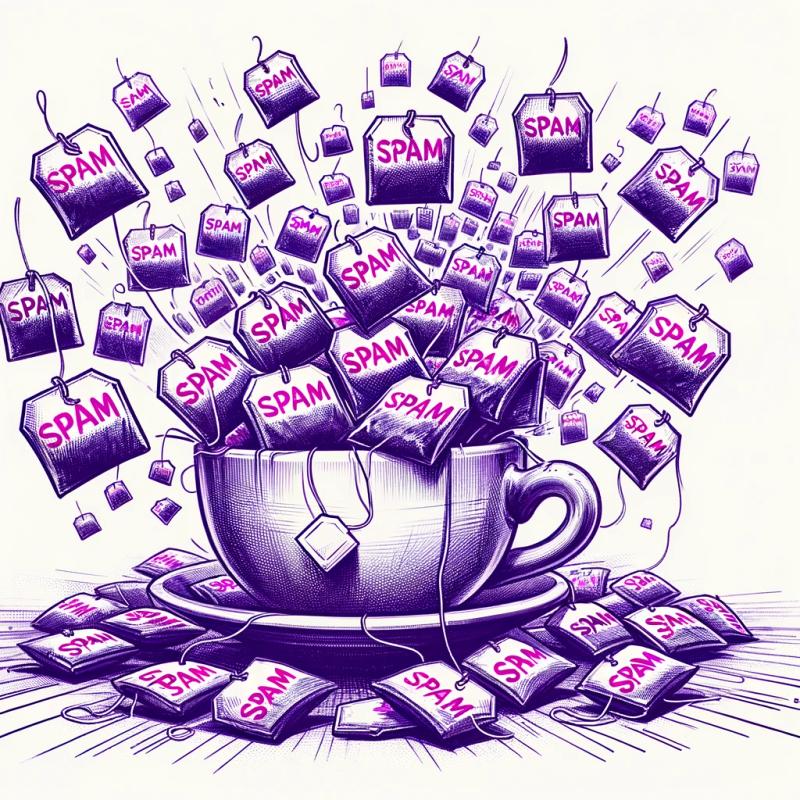
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ngx-modal-ease
Advanced tools
Readme
ngx-modal-ease is a versatile Angular library providing a lightweight, simple, and performant modal. This library supports data communication between components, opening of multiple modals, custom animations, and a range of customisable options.
Support Angular version starts at v16 (see changelog).
Live demonstration of the ngx-modal-ease library here.
Version | Command | Description |
---|---|---|
V16 | npm i ngx-modal-ease@0.0.6 | Install the V16 compatible version. |
V17 | npm i ngx-modal-ease | Install the V17 compatible version. |
The modal supports a range of customisable options:
Options {
modal?: {
enter?: string;
leave?: string;
top?: string;
left?: string;
};
overlay?: {
enter?: string;
leave?: string;
backgroundColor?: string;
};
size?: {
height?: string;
maxHeight?: string;
width?: string;
maxWidth?: string;
padding?: string;
};
actions?: {
escape?: boolean;
click?: boolean;
};
data?: {
[key: string]: unknown;
};
}
Name | Default | Description |
---|---|---|
enter | Define the enter animation for the modal or the overlay respecting the shorthand animation property. | |
leave | Define the leave animation for the modal or the overlay respecting the shorthand animation property. | |
top | 50 | Top position of the modal in percent. Can be defined in any measure unit. |
left | 50 | Left position of the modal in percent. Can be defined in any measure unit. |
backgroundColor | rgba(0, 0, 0, 0.4); | Background color of the overlay. Can be defined in any color notation (rgba, hex, hsl, ...). |
minHeight | Minimum height of the modal. Can be defined in any measure unit. | |
height | Height of the modal. Can be defined in any measure unit. | |
width | Width of the modal. Can be defined in any measure unit. | |
maxWidth | 100 | Max width of the modal in percent. Can be defined in any measure unit. |
padding | 0.5 | Padding to be applied on the modal in rem. Can be defined in any measure unit. |
escape | true | Enable escape to close the current modal. |
click | true | Enable click outside of the modal to close the current modal. |
data | Data communication between components under the form of key-value pairs. Any type of data is supported. |
Inject the ModalService through regular dependency injection in your component.
In the following example, ModalContentComponent
is the content of the modal and should be provided as first parameter. As second parameter, provide an object respecting the Options
interface (see above).
this.modalService.open(ModalContentComponent, {
modal: {
<!-- animation -->
enter: 'enter-scale-down 0.1s ease-out',
},
overlay: {
<!-- animation -->
leave: 'fade-out 0.3s',
},
size: {
<!-- modal configuration -->
width: '400px',
},
data: {
<!-- data to ModalContentComponent -->
type: 'Angular modal library',
},
})
.subscribe((dataFromModalContentComponent) => {
...
});
Any type of data can be provided between components. Create the corresponding property (here, type
) in your component (here, ModalContentComponent
) and the property will be assigned with the provided value.
In your ModalContentComponent
:
To pass information from the ModalContentComponent
to your current component, inject the ModalService
through regular dependency injection and call the close(data)
method from the service with any data you wish to send back to your component. This method returns an RxJs subject, so subscribe to it as shown in the above example. It is not necessary to unsubscribe from the subscription since it will automatically complete()
in the service.
<!-- Inside ModalContentComponent -->
onClose() {
this.modalService.close(this.dataToSendBack);
}
Publicly available methods have been exhaustively documented and typed, so you should get autocomplete and help from your code editor. Press on CTRL + space
to get help on the available properties in the Options
object.
This library exposes a ModalService
that contains the following API:
<!-- Opens a component inside the modal -->
open<C>(componentToCreate: Type<C>, options?: Options);
<!-- Close a modal with optional data to send back -->
close(data?: unknown);
<!-- Close all opened modals -->
closeAll();
NB: Z-index of overlay and modal start at 1000 and 2000 respectively. In case of multiple modals, z-indexes will be incremented by 1000.
This library comes with predefined and ready-to-use animations keyframes. Just fill in the name
, duration
and easing function
(more info on the animation CSS shorthand
here). Those animations are position agnostic, so if you wish to position your modal at other top
and left
values than default, it will correctly work. Of course, you can create your own keyframes too.
/* Recommended: 0.2s ease-out */
@keyframes enter-going-down {
from {
transform: translate(-50%, -60%);
}
to {
transform: translate(-50%, -50%);
}
}
/* Recommended: 0.2s linear */
@keyframes enter-scaling {
from {
transform: scale(0.8) translate(-50%, -50%);
transform-origin: left;
}
to {
transform: scale(1) translate(-50%, -50%);
transform-origin: left;
}
}
/* Recommended: 0.1s ease-out */
@keyframes enter-scale-down {
from {
transform: scale(1.5) translate(-50%, -60%);
transform-origin: left;
}
to {
transform: scale(1) translate(-50%, -50%);
transform-origin: left;
}
}
/* Recommended: 0.3s linear */
@keyframes fade-in {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
/* Recommended: 0.3s linear */
@keyframes fade-out {
from {
opacity: 1;
}
to {
opacity: 0;
}
}
/* Recommended: 0.7s linear */
@keyframes bounce-in {
0% {
transform: translate(-50%, -85%);
}
18% {
transform: translate(-50%, -50%);
}
60% {
transform: translate(-50%, -65%);
}
80% {
transform: translate(-50%, -50%);
}
90% {
transform: translate(-50%, -53%);
}
100% {
transform: translate(-50%, -50%);
}
}
/* Recommended: 1s linear */
@keyframes scale-rotate {
30% {
transform: scale(1.05) translate(-50%, -50%);
transform-origin: left;
}
40%,
60% {
transform: rotate(-3deg) scale(1.05) translate(-50%, -50%);
transform-origin: left;
}
50% {
transform: rotate(3deg) scale(1.05) translate(-50%, -50%);
transform-origin: left;
}
70% {
transform: rotate(0deg) scale(1.05) translate(-50%, -50%);
transform-origin: left;
}
100% {
transform: scale(1) translate(-50%, -50%);
transform-origin: left;
}
}
If you create your own keyframes, I would recommend to create a new file modal-animations
(.css or preprocessor), and @import it in your styles.css
(or preprocessor) at the root of the application.
This library supports Server Side Rendering (SSR). The modal will not instantiate during server-side execution, as it requires access to the DOM API.
This library has been documented and should provide autocomplete and help from your code editor. Tested on VS Code.
Emphasis has been placed on performance, adopting ChangeDetectionStrategy.OnPush
strategy. This library respects Angular's mindset and use the Angular API to create components. Modal components will be removed from the DOM after closing.
Version 0.0.4: Fixed of a communication bug between components. Every modal has now its own subject to send back data to the calling component.
Version 0.0.5: Fixed a bug between components that prevented the component from closing when applying different animations with multiple stacked modals.
Version 0.0.6: Added V16 backward compatibility support.
Please provide a detailed description of the encountered bug, including your options and the steps/actions that led to the issue. An accurate description will help me to reproduce the issue.
You like this library? Discover the ngx-ease serie here.
FAQs
ngx-modal-ease is a versatile Angular library providing a lightweight, simple, and performant modal. This library supports data communication between components, opening of multiple modals, custom animations, and a range of customisable options.
The npm package ngx-modal-ease receives a total of 31 weekly downloads. As such, ngx-modal-ease popularity was classified as not popular.
We found that ngx-modal-ease demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.