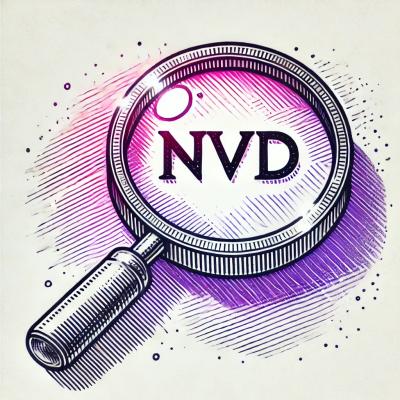
Security News
NIST Under Federal Audit for NVD Processing Backlog and Delays
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
node-box-api
Advanced tools
Currently supporting basic Box Content API
Working towards full feature support of Box Content API & Box View API
https://box-content.readme.io/reference
https://box-view.readme.io/reference
npm install node-box-api
var Box = require('node-box-api');
var box = new Box({
client_id: 'APPLICATION_CLIENT_ID',
client_secret: 'APPLICATION_CLIENT_SECRET',
access_token: 'USER_ACCESS_TOKEN',
refresh_token: 'USER_REFRESH_TOKEN'
});
After instantiating the Box class you can call API
resources by calling box.folders
box.folders.root(function(err, res) {
console.log(res);
});
box.folders.info('FOLDER_ID', function(err, res) {
console.log(res);
});
var params = {
limit: 100,
offset: 0,
fields: 'name,etc'
};
box.folders.items('FOLDER_ID', params, function(err, res) {
console.log(res);
});
The params
argument is optional
box.folders.items('FOLDER_ID', function(err, res) {
console.log(res);
});
After instantiating the Box class you can call API
resources by calling box.files
box.files.info('FILE_ID', function(err, res) {
console.log(res);
});
box.files.download('FILE_ID', function(err, res) {
console.log(res);
});
The download function does accept params
, however it is
not currently supported
var params = {
min_height: 32,
min_width: 32,
max_height: 256,
max_width: 256,
extension: 'jpg'
};
box.files.thumbnail('FILE_ID', params, function(err, res) {
console.log(res);
});
The params
argument is optional
box.files.thumbnail('FILE_ID', function(err, res) {
console.log(res);
});
FAQs
Box API for Nodejs
The npm package node-box-api receives a total of 0 weekly downloads. As such, node-box-api popularity was classified as not popular.
We found that node-box-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.
Security News
TypeScript Native Previews offers a 10x faster Go-based compiler, now available on npm for public testing with early editor and language support.