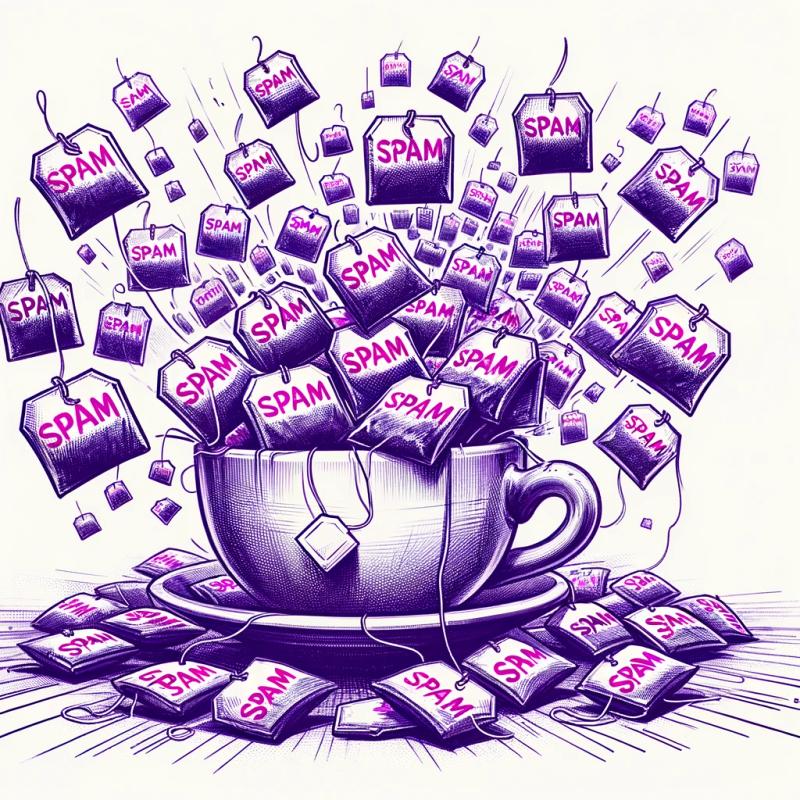
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
node-mysql-basemodel
Advanced tools
Node-mysql-basemodel is an easy to use model from which other models can be extended from. It is designed to provide basic out-of-the-box functionality without making assumptions or providing a complicated API. The focus is very much on development spee
Readme
Node-mysql-basemodel is an easy to use model from which other models can be extended from. It is designed to provide basic out-of-the-box functionality without making assumptions or providing a complicated API. The focus is very much on development speed and providing an easy to reason about way to structure your application's models. It is intended to be used with node-mysql. It requires IO.js or Node 4.* for es6 support however porting it over to es5 shouldn't be too much trouble.
##Installation
npm install node-mysql-basemodel
##Usage
This module comes with many built on methods for simple and regularly used queries. All methods will return promises. All methods ultimately flow into query(sql). When extending if you have more complicated SQL simply write out whatever SQL you need and pass it into query.
'use strict';
var Base = require('node-mysql-basemodel'),
pool = require('path/to/dbpool');
class UsersModel extends Base {
someNonBasicQuery(options) {
return this.query(`
...
`);
}
}
module.exports = new UsersModel({
name: 'users',
pool: pool
});
##Methods
###model.query(sql)
####Description
Model.query() is the basic method all other methods ultimately call. It takes a single argument which is a sql string it uses to query the database. This is the method you will want to use to make your own custom queries.
####Options
####Returns
Returns an array containing the results of your query.
####Example
UsersModel.query('SELECT * FROM users u JOIN pets p ON u.id=p.owner_id WHERE p.type = "dog"')
.then((results) => {
...
})
.catch((err) => {
...
})
###model.all()
####Description
Model.all() returns all records in a table.
####Returns
Returns an array containing all the records in the associated table.
####Example
UsersModel.all()
.then((results) => {
...
})
.catch((err) => {
...
})
###model.insert(options)
####Description
Model.insert() is used to insert a single record into the model's associated table.
####options
####Returns
Returns an array containing the mysql response with the insertId.
####Example
UsersModel.insert({
fields: {
name : 'Bob',
occupation: 'Plumber',
active : 0
}
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.insertMulti(options)
####Description
Inserts multiple records into the model's associated table.
####Options
####Returns
Returns an array containing information relating to the bulk insert.
####Example
UsersModel.insertMulti({
fields: [{
name : 'Bob',
occupation: 'Plumber',
active : 0
},
{
name : 'Jim',
occupation: 'Coach',
active : 1
}]
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.insertAndReturnData(options)
####Description
Inserts into the model's associated table and retrieves the newly inserted record and returns it.
####Options
####Returns
Returns an array with a single object containing the newly inserted record's data.
####Example
UsersModel.insertAndReturnData({
fields: {
name : 'Bob',
occupation: 'Plumber',
active : 0
}
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.insertMultiAndReturnData(options)
####Description
Inserts multiple records into the model's associated table and returns the newly inserted records.
####Options
####Returns
Returns an array containing the newly inserted records.
####Example
UsersModel.insertMultiAndReturnData({
fields: [{
name : 'Bob',
occupation: 'Plumber',
active : 0
},
{
name : 'Jim',
occupation: 'Coach',
active : 1
}]
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.deleteById(options)
####Description
Deletes a record by id.
####Options
####Returns
Returns the mysql response for the deleted record.
####Example
UsersModel.deleteById({
id: 5
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.delete(options)
####Description
deletes rows based on either the where property and/or the ids property.
####Options
####Returns
Returns the mysql response to the deletion.
####Example
UsersModel.delete({
where: {
name: 'Bob'
},
ids: [3,5]
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.selectById(options)
####Description
Model.selectById() selects a single record by id.
####options
####Returns
Returns an array containing one object, the record of the selected id.
####Example
UsersModel.selectById({
id: 5
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.select(options)
####Description
Model.select() selects one record based on the where property.
####options
####Returns
Returns an array containing the selected record.
####Example
UsersModel.select({
where: {
active: 1,
type: 'member'
}
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.selectAll(options)
####Description
Model.select() selects multiple records based on the where property.
####options
####Returns
Returns an array containing the selected records.
####Example
UsersModel.selectAll({
where: {
active: 1,
type: 'member'
}
})
.then((results) => {
...
})
.catch((err) => {
...
})
###model.update(options)
####Description
Model.update() updates records based on the where and set properties.
####options
####Returns
The mysql response for the update.
####Example
UsersModel.update({
set: {
active: 1
},
where: {
type: 'member'
}
})
.then((results) => {
...
})
.catch((err) => {
...
})
##Adding more complicated queries and structure
Node-mysql-basemodel by design only provides basic functionality. However it's extremely easy to add any query you would like. Within your childModel you can create any number of methods that use this.query() to execute your queries. This provides you with the freedom to not wrestle an api that may or may not execute exactly what you're looking for as well as an easy way to group queries related to a specific table.
For example let's assume we're writing a restaurant app and it will have three tables - users, restaurants, and visits.
Schemas:
The three models might look like this:
'use strict';
var Base = require('node-mysql-basemodel'),
pool = require('path/to/dbpool');
class UsersModel extends Base {
getUsersWithVisits(options){
var ids = options.ids.join(',');
return this.query(`
SELECT u.id, u.name, count(v.id) FROM users u
LEFT JOIN visits v ON u.id=v.user_id
WHERE id IN (${ids})
GROUP BY u.id
`);
}
}
class RestaurantsModel extends Base {
getRestaurantsWithVisits(){
return this.query(`
SELECT r.id, r.name, count(r.id) from restaurants r
LEFT JOIN visits v on r.id=v.restaurant_id
GROUP BY r.id
`);
}
}
class VisitsModel extends Base {
getVisitsForUserForRestaurant(options){
return this.query(`
SELECT count(v.id) FROM visits v
WHERE v.user_id=${options.user_id}
AND v.restaurant_id=${options.restaurant_id}
`);
}
}
var Users = new UsersModel({
name: 'users',
pool: pool
});
var Restaurants = new RestaurantsModel({
name: 'restaurants',
pool: pool
});
var Visits = new VisitsModel({
name: 'visits',
pool: pool
});
Ok so now let's chain together a few of these methods
Users.getUsersWithVisits({
ids: [1]
})
.then((results) => {
return Visits.getVisitsForUserForRestaurant({
user_id: results[0].id,
restaurant_id: 10
});
})
.then((visits) => {
return Restaurants.selectById(visits[0].restaurant_id);
})
.then((restaurant) => {
...
});
FAQs
Node-mysql-basemodel is an easy to use model from which other models can be extended from. It is designed to provide basic out-of-the-box functionality without making assumptions or providing a complicated API. The focus is very much on development spee
The npm package node-mysql-basemodel receives a total of 0 weekly downloads. As such, node-mysql-basemodel popularity was classified as not popular.
We found that node-mysql-basemodel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.