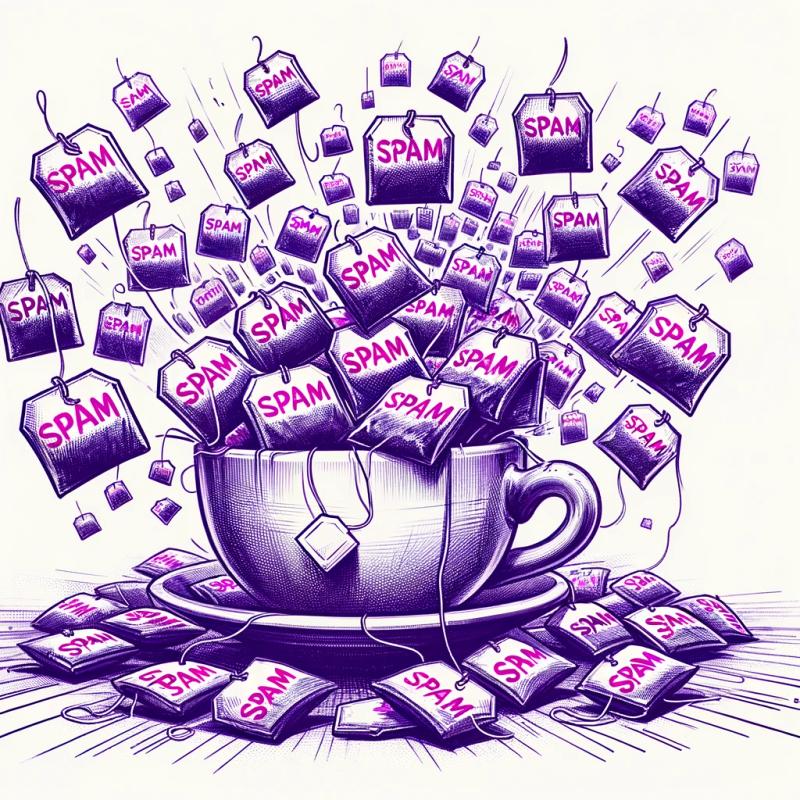
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
node-sms-active
Advanced tools
Readme
This library provides the following features
npm i node-sms-active
const smsActive = require("node-sms-active");
smsActive.buyNumber({
country: 6, // See the bottom of the documentation for the country list.
service: 'dr', // See the bottom of the documentation for the service list.
operator: 'any',
apiKey: '',
verification: false
})
.then(response=>{
console.log(response)
})
.catch(error =>{
console.log(error)
})
country: The number of sms active in the country where the number will be received. To find the country you are looking for, see the country codes at the bottom of the page.
service: The sms active short code of the service to receive the number. To find the service you are looking for, see the service codes at the bottom of the page.
operator: You can use it if you want to get a number from a private operator in your country. It is not mandatory to send it. Default any
apiKey: https://sms-activate.org/en/api2 You can create a key by saying create api key from this link.
verification: Default false. Sending is not mandatory. If true, a number that supports the call will be received.
{
status: true,
data: {
activationId: '1779177117',
phoneNumber: '628973921615',
activationCost: '4.50',
countryCode: '6',
canGetAnotherSms: true,
activationTime: '2023-09-30 02:36:18',
activationOperator: 'three'
}
}
{
status: false,
data: 'WRONG_COUNTRY'
}
const smsActive = require("node-sms-active");
smsActive.getCode({
activationId: '',
apiKey: '',
})
.then(response=>{
console.log(response)
})
.catch(error =>{
console.log(error)
})
activationId: When you buy the number, you need to send the value returned to you in json.
apiKey: https://sms-activate.org/en/api2 You can create a key by saying create api key from this link.
{
status: true,
data: '396989'
}
{
status: false,
data: 'STATUS_WAIT_CODE'
}
const smsActive = require("node-sms-active");
smsActive.waitForBuyNumber({
country: 6, // See the bottom of the documentation for the country list.
service: 'dr', // See the bottom of the documentation for the service list.
operator: 'any',
apiKey: '',
verification: false,
timeOut: 0
})
.then(response=>{
console.log(response)
})
.catch(error =>{
console.log(error)
})
country: The number of sms active in the country where the number will be received. To find the country you are looking for, see the country codes at the bottom of the page.
service: The sms active short code of the service to receive the number. To find the service you are looking for, see the service codes at the bottom of the page.
operator: You can use it if you want to get a number from a private operator in your country. It is not mandatory to send it. Default any
apiKey: https://sms-activate.org/en/api2 You can create a key by saying create api key from this link.
verification: Default false. Sending is not mandatory. If true, a number that supports the call will be received.
timeOut: 0 Wait indefinitely if sent. If sent in milliseconds, it will return the status when the time expires. 1 Second = 1000 Milliseconds
{
status: true,
data: {
activationId: '1779177117',
phoneNumber: '628973921615',
activationCost: '4.50',
countryCode: '6',
canGetAnotherSms: true,
activationTime: '2023-09-30 02:36:18',
activationOperator: 'three'
}
}
{
status: false,
data: 'WRONG_COUNTRY'
}
const smsActive = require("node-sms-active");
smsActive.waitForCode({
activationId: '',
apiKey: '',
timeOut: 0
})
.then(response=>{
console.log(response)
})
.catch(error =>{
console.log(error)
})
activationId: When you buy the number, you need to send the value returned to you in json.
apiKey: https://sms-activate.org/en/api2 You can create a key by saying create api key from this link.
timeOut: 0 Wait indefinitely if sent. If sent in milliseconds, it will return the status when the time expires. 1 Second = 1000 Milliseconds
{
status: true,
data: '396989'
}
{
status: false,
data: 'STATUS_WAIT_CODE'
}
const smsActive = require("node-sms-active");
smsActive.setStatusActivation({
activationId: '',
apiKey: '',
status: 8
})
.then(response=>{
console.log(response)
})
.catch(error =>{
console.log(error)
})
activationId: When you buy the number, you need to send the value returned to you in json.
apiKey: https://sms-activate.org/en/api2 You can create a key by saying create api key from this link.
status: Can only take values 1, 3, 6, 8
1 inform about the readiness of the number (SMS sent to the number)
3 request another code (free)
6 complete activation *
8 inform that the number has been used and cancel the activation
{
status: true,
data: 'ACCESS_CANCEL'
}
{
status: false,
data: 'EARLY_CANCEL_DENIED - You can‘t cancel the number within the first 2 minutes'
}
const smsActive = require("node-sms-active");
smsActive.getBallance({
apiKey: '',
})
.then(response=>{
console.log(response)
})
.catch(error =>{
console.log(error)
})
apiKey: https://sms-activate.org/en/api2 You can create a key by saying create api key from this link.
{
status: true,
data: 498.28
}
{
status: false,
data: 'ERROR_SQL'
}
Number | Country | Title |
---|---|---|
0 | Russia | ![]() |
1 | Ukraine | ![]() |
2 | Kazakhstan | ![]() |
3 | China | ![]() |
4 | Philippines | ![]() |
5 | Myanmar | ![]() |
6 | Indonesia | ![]() |
7 | Malaysia | ![]() |
8 | Kenya | ![]() |
9 | Tanzania | ![]() |
10 | Vietnam | ![]() |
11 | Kyrgyzstan | ![]() |
12 | USA (virtual) | ![]() |
13 | Israel | ![]() |
14 | HongKong | ![]() |
15 | Poland | ![]() |
16 | England | ![]() |
17 | Madagascar | ![]() |
18 | DCongo | ![]() |
19 | Nigeria | ![]() |
20 | Macao | ![]() |
21 | Egypt | ![]() |
22 | India | ![]() |
23 | Ireland | ![]() |
24 | Cambodia | ![]() |
25 | Laos | ![]() |
26 | Haiti | ![]() |
27 | Ivory | ![]() |
28 | Gambia | ![]() |
29 | Serbia | ![]() |
30 | Yemen | ![]() |
31 | Southafrica | ![]() |
32 | Romania | ![]() |
33 | Colombia | ![]() |
34 | Estonia | ![]() |
35 | Azerbaijan | ![]() |
36 | Canada | ![]() |
37 | Morocco | ![]() |
38 | Ghana | ![]() |
39 | Argentina | ![]() |
40 | Uzbekistan | ![]() |
41 | Cameroon | ![]() |
42 | Chad | ![]() |
43 | Germany | ![]() |
44 | Lithuania | ![]() |
45 | Croatia | ![]() |
46 | Sweden | ![]() |
47 | Iraq | ![]() |
48 | Netherlands | ![]() |
49 | Latvia | ![]() |
50 | Austria | ![]() |
51 | Belarus | ![]() |
52 | Thailand | ![]() |
53 | Saudiarabia | ![]() |
54 | Mexico | ![]() |
55 | Taiwan | ![]() |
56 | Spain | ![]() |
57 | Iran | ![]() |
58 | Algeria | ![]() |
59 | Slovenia | ![]() |
60 | Bangladesh | ![]() |
61 | Senegal | ![]() |
62 | Turkey | ![]() |
63 | Czech | ![]() |
64 | Srilanka | ![]() |
65 | Peru | ![]() |
66 | Pakistan | ![]() |
67 | Newzealand | ![]() |
68 | Guinea | ![]() |
69 | Mali | ![]() |
70 | Venezuela | ![]() |
71 | Ethiopia | ![]() |
72 | Mongolia | ![]() |
73 | Brazil | ![]() |
74 | Afghanistan | ![]() |
75 | Uganda | ![]() |
76 | Angola | ![]() |
77 | Cyprus | ![]() |
78 | France | ![]() |
79 | Papua | ![]() |
80 | Mozambique | ![]() |
81 | Nepal | ![]() |
82 | Belgium | ![]() |
83 | Bulgaria | ![]() |
84 | Hungary | ![]() |
85 | Moldova | ![]() |
86 | Italy | ![]() |
87 | Paraguay | ![]() |
88 | Honduras | ![]() |
89 | Tunisia | ![]() |
90 | Nicaragua | ![]() |
91 | Timorleste | ![]() |
92 | Bolivia | ![]() |
93 | Costarica | ![]() |
94 | Guatemala | ![]() |
95 | Uae | ![]() |
96 | Zimbabwe | ![]() |
97 | Puertorico | ![]() |
98 | Sudan | ![]() |
99 | Togo | ![]() |
100 | Kuwait | ![]() |
101 | Salvador | ![]() |
102 | Libyan | ![]() |
103 | Jamaica | ![]() |
104 | Trinidad | ![]() |
105 | Ecuador | ![]() |
106 | Swaziland | ![]() |
107 | Oman | ![]() |
108 | Bosnia | ![]() |
109 | Dominican | ![]() |
110 | Syrian | ![]() |
111 | Qatar | ![]() |
112 | Panama | ![]() |
113 | Cuba | ![]() |
114 | Mauritania | ![]() |
115 | Sierraleone | ![]() |
116 | Jordan | ![]() |
117 | Portugal | ![]() |
118 | Barbados | ![]() |
119 | Burundi | ![]() |
120 | Benin | ![]() |
121 | Brunei | ![]() |
122 | Bahamas | ![]() |
123 | Botswana | ![]() |
124 | Belize | ![]() |
125 | Caf | ![]() |
126 | Dominica | ![]() |
127 | Grenada | ![]() |
128 | Georgia | ![]() |
129 | Greece | ![]() |
130 | Guineabissau | ![]() |
131 | Guyana | ![]() |
132 | Iceland | ![]() |
133 | Comoros | ![]() |
134 | Saintkitts | ![]() |
135 | Liberia | ![]() |
136 | Lesotho | ![]() |
137 | Malawi | ![]() |
138 | Namibia | ![]() |
139 | Niger | ![]() |
140 | Rwanda | ![]() |
141 | Slovakia | ![]() |
142 | Suriname | ![]() |
143 | Tajikistan | ![]() |
144 | Monaco | ![]() |
145 | Bahrain | ![]() |
146 | Reunion | ![]() |
147 | Zambia | ![]() |
148 | Armenia | ![]() |
149 | Somalia | ![]() |
150 | Congo | ![]() |
151 | Chile | ![]() |
152 | Burkinafaso | ![]() |
153 | Lebanon | ![]() |
154 | Gabon | ![]() |
155 | Albania | ![]() |
156 | Uruguay | ![]() |
157 | Mauritius | ![]() |
158 | Bhutan | ![]() |
159 | Maldives | ![]() |
160 | Guadeloupe | ![]() |
161 | Turkmenistan | ![]() |
162 | Frenchguiana | ![]() |
163 | Finland | ![]() |
164 | Saintlucia | ![]() |
165 | Luxembourg | ![]() |
166 | Saintvincentgrenadines | ![]() |
167 | Equatorialguinea | ![]() |
168 | Djibouti | ![]() |
169 | Antiguabarbuda | ![]() |
170 | Caymanislands | ![]() |
171 | Montenegro | ![]() |
172 | Denmark | ![]() |
173 | Switzerland | ![]() |
174 | Norway | ![]() |
175 | Australia | ![]() |
176 | Eritrea | ![]() |
177 | Southsudan | ![]() |
178 | Saotomeandprincipe | ![]() |
179 | Aruba | ![]() |
180 | Montserrat | ![]() |
181 | Anguilla | ![]() |
183 | Northmacedonia | ![]() |
184 | Seychelles | ![]() |
185 | Newcaledonia | ![]() |
186 | Capeverde | ![]() |
187 | USA | ![]() |
189 | Fiji | ![]() |
196 | Singapore | ![]() |
201 | Gibraltar | ![]() |
Service Code | Name |
---|---|
full | Full Rent |
full | Full rent |
tg | Telegram |
ig | Instagram+Threads |
wa | |
fb | |
go | Google,youtube,Gmail |
tw | |
mm | Microsoft |
hw | Alipay/Alibaba/1688 |
am | Amazon |
oi | Tinder |
ma | Mail.ru |
ds | Discord |
mt | Steam |
ju | Indomaret |
lf | TikTok/Douyin |
me | Line messenger |
ot | Any other call forwarding |
ot | Any other |
of | urent/jet/RuSharing |
da | MTS CashBack |
nv | Naver |
dr | OpenAI |
tn | |
vk | vk.com |
ew | Nike |
mb | Yahoo |
ya | Yandex |
ya | Yandex call forwarding |
dh | eBay |
pm | AOL |
vs | WinzoGame |
ts | PayPal |
dl | Lazada |
ok | ok.ru |
ka | Shopee |
av | avito |
av | avito call forwarding |
nz | Foodpanda |
ub | Uber |
ki | 99app |
uu | Wildberries |
wb | |
yw | Grindr |
acz | Claude |
bw | Signal |
vi | Viber |
xd | Tokopedia |
kt | KakaoTalk |
fw | 99acres |
sn | OLX |
kc | Vinted |
be | СберМегаМаркет |
ft | Букмекерские |
pf | pof.com |
qf | RedBook |
ll | 888casino |
wx | Apple |
yl | Yalla |
mj | Zalo |
fu | Snapchat |
xk | DiDi |
pd | IFood |
ni | Gojek |
xh | OVO |
vm | OkCupid |
fr | Dana |
xj | СберМаркет |
df | Happn |
cy | РСА |
bc | GCash |
jg | Grab |
mv | Fruitz |
tk | МВидео |
im | Imo |
pc | Casino/bet/gambling |
gf | GoogleVoice |
sg | OZON |
act | Maxis |
ly | Olacabs |
vz | Hinge |
nf | Netflix |
cq | Mercado |
mo | Bumble |
yy | Venmo |
bz | Blizzard |
vr | MotorkuX |
uk | Airbnb |
fx | PGbonus call forwarding |
fx | PGbonus |
bv | Metro |
do | Leboncoin |
cb | Bazos |
zp | Pinduoduo |
wh | TanTan |
ac | DoorDash |
ev | Picpay |
ado | SmartyPig |
gx | Hepsiburadacom |
kf | |
tx | Bolt |
acp | BonusLink |
Tencent QQ | |
rr | Wolt |
cp | Uklon |
aav | Alchemy |
yr | Miravia |
ie | bet365 |
acy | Airtime |
fo | MobiKwik |
ep | Temu |
ns | Oldubil |
em | ZéDelivery |
zk | Deliveroo |
dt | Delivery Club |
acb | Spark Driver |
et | Clubhouse |
tu | Lyft |
ah | EscapeFromTarkov |
gp | Ticketmaster |
ad | Iti |
xq | MPL |
abx | Kaching |
abk | GMX |
ze | Shpock |
pu | Justdating |
ada | TRUTH SOCIAL |
ke | Эльдорадо |
zb | FreeNow |
gj | Carousell |
ib | Immowelt |
qv | Badoo |
ls | Careem |
hu | Ukrnet |
fd | Mamba |
zu | BigC |
hs | Asda |
fk | BLIBLI |
aaa | Nubank |
rd | Lenta |
yu | Xiaomi |
ua | BlaBlaCar |
xy | Depop |
ym | youla.ru call forwarding |
ym | youla.ru |
bn | Alfagift |
kj | YAPPY |
nc | Payoneer |
xm | Лэтуаль |
jr | Самокат |
mg | Magnit |
nt | Sravni |
abq | Upwork |
abt | ArenaPlus |
kl | kolesa.kz |
ge | Paytm |
wv | AIS |
aec | JinJiang |
zs | Bilibili |
lx | DewuPoison |
ae | myGLO |
acc | LuckyLand Slots |
za | JDcom |
yk | СпортМастер |
gu | Fora |
adc | PlayOJO |
hx | AliExpress |
vd | Betfair |
zh | Zoho |
zo | Kaggle |
bd | X5ID |
mx | SoulApp |
ov | Beget |
lj | Santander |
qz | Faceit |
gq | Freelancer |
bl | BIGO LIVE |
bm | MarketGuru |
vg | ShellBox |
fz | KFC |
ff | AVON |
rl | inDriver |
lc | Subito |
bo | Wise |
at | Perfluence |
jx | Swiggy |
abl | gpnbonus |
io | ЗдравСити |
wc | Craigslist |
ue | Onet |
km | Rozetka |
gr | Astropay |
jl | Hopi |
cm | Prom |
ex | Linode |
tl | Truecaller |
ps | Zdorov |
rt | hily |
acn | Radium |
xu | RecargaPay |
jq | Paysafecard |
gk | AptekaRU |
ng | FunPay |
acj | Meituan |
li | Baidu |
mi | Zupee |
rn | neftm |
abc | Taptap Send |
cn | Fiverr |
ta | Wink |
sh | Vkusvill |
sm | YoWin |
qy | Zhihu |
hb | Twitch |
kx | Vivo |
nl | Myntra |
vp | Kwai |
abn | Namars |
dp | ProtonMail |
re | Coinbase |
gi | Hotline |
rc | Skype |
ys | ZCity |
yj | eWallet |
co | Rediffmail |
ye | ZaleyCash |
yx | JTExpress |
th | WestStein |
vy | Meta |
cr | TenChat |
bh | Uteka |
ix | Celcoin |
zm | OfferUp |
hy | Ininal |
ml | ApostaGanha |
mz | Zolushka |
hz | Drom |
po | premium.one |
bb | LazyPay |
tz | Лейка |
hp | Meesho |
aau | RockeTreach |
ip | Burger King |
acw | YouDo |
oj | LoveRu |
aq | Glovo |
wg | Skout |
cj | Dotz |
xt | Flipkart |
rk | Fotka |
fh | Lalamove |
jv | Consultant |
vc | Banqi |
my | CAIXA |
ky | SpatenOktoberfest |
sd | dodopizza |
ln | Grofers |
kh | Bukalapak |
zd | Zilch |
ve | Dream11 |
xz | paycell |
ul | Getir |
aeb | GoPayz |
oz | Poshmark |
ao | UU163 |
wd | CasinoPlus |
js | GolosZa |
aba | Rappi |
kk | Idealista |
rj | Детский мир |
adp | Cabify |
uz | OffGamers |
oe | Codashop |
adr | Boosty |
ck | BeReal |
sr | Starbucks |
il | IQOS |
ady | ТОКИО-CITY |
fj | Potato Chat |
sy | Brahma |
yi | Yemeksepeti |
aby | Couponscom |
ax | CrefisaMais |
dn | Paxful |
no | Virgo |
wr | Walmart |
ko | AdaKami |
acs | Tata CLiQ Palette |
rm | Faberlic |
aaq | Netease |
sz | Pivko24 |
jc | IVI |
fa | XadrezFeliz |
mc | Michat |
ow | RegRu |
an | Adidas |
kq | FotoCasa |
tm | Akulaku |
gw | CallApp |
fl | RummyLoot |
jd | GiraBank |
ld | Cashmine |
adl | EarnEasy |
kb | kufarby |
abo | WEBDE |
dd | CloudChat |
ks | Hirect |
lt | BitClout |
zr | Papara |
je | Nanovest |
rf | Akudo |
cg | Gemgala |
vh | Штолле |
sc | Crypto |
xg | Дзен |
ilr | YANDEX |
uwc | Odobrenie |
lsq | Spoiler |
acf | ShopeeMY |
syg | JustDates |
tc | AmazonML |
twm | Solana |
kd | IviNews |
ktp | Divo |
lp | CryptoMining |
dx | CubeTV |
yuq | CCB |
atq | Авто2Ру |
adq | ACMarket |
ijd | INTENCITY |
lyd | Birge |
adg | иРНД |
iud | AdGuard |
adw | Wolt |
lyw | Трк ГуляйПоле |
fp | Buy+ |
liq | Limpkin |
fpq | YouZik |
adk | Однокласники |
abp | Youzik |
kn | KINOPOISK |
lxq | Lmz |
fwq | Новая почта |
ffq | Тануки |
fq | Розетка |
flq | GuaiGuai |
fn | Shopee |
fzq | СмартьФинанс |
qfq | Амедиа |
fqq | BlockParty |
bf | Olx |
fqq | Firestarter |
FAQs
It performs functions such as number purchase and code waiting using sms active api.
The npm package node-sms-active receives a total of 2 weekly downloads. As such, node-sms-active popularity was classified as not popular.
We found that node-sms-active demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.