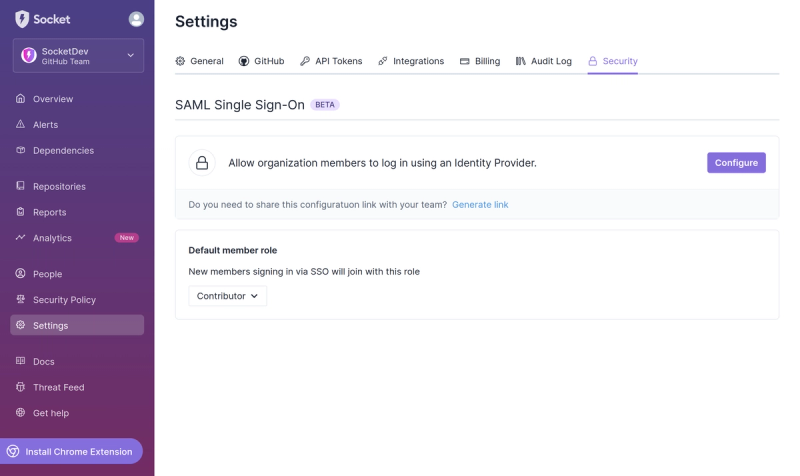
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
node-threads-pool
Advanced tools
Readme
Node-Threads-Pool is a Node.js library that provides a simple and efficient way to execute tasks in child threads. It creates a pool of threads that can be used to parallelize the execution of CPU-intensive tasks, making it possible to take advantage of multi-core processors.
The library is designed to be easy to use and to integrate with existing projects. It provides a simple API that allows you to create a thread pool and run tasks in child threads, and it also supports passing data between threads.
You can install Node-Threads-Pool using NPM:
npm install node-threads-pool
To use Node-Threads-Pool, you need to create an instance of the Eve
class, which represents the thread pool.
// main.js
const { Eve } = require('node-threads-pool');
const tp = new Eve(filename, threadCount);
The Eve
constructor takes two arguments: the path to the worker script and the number of threads to create. The run
method takes one argument, which is the data to be passed to the worker script.
filename
: The path to the worker's main script or module. See the Node.js documentation for more details.threadCount
: The number of threads in the thread pool.To run a task in a worker thread, use the run
method:
// main.js
tp.run(workerData)
.then(result => {
// handle the result
})
.catch(err => {
// handle the error
})
You can create a new thread directly using the Thread
class:
// thread.js
const {Thread} = require('node-threads-pool');
new Thread(async (data) => {
// Do some work with data
const result = await doSomething(data);
return result;
});
The Thread
constructor takes a single argument, which is the function to be executed in the child thread. The function should return a Promise that resolves with the result of the computation.
Here are some examples of how to use Node Threads Pool.
This example shows how to create a pool of 20 worker threads to perform some calculations:
// main.js
const os = require('os');
const { Eve } = require('node-threads-pool');
const tp = new Eve('thread.js', os.cpus().length);
module.exports = async (data) => {
return await tp.run(data);
};
// thread.js
const { Thread } = require('node-threads-pool');
const thread = new Thread(async (data) => {
return await doSomething(data);
});
Alternatively, you can write to the same JS file:
// main.js
const { Eve, Thread, isMainThread } = require('node-threads-pool');
const os = require('os');
if(isMainThread) {
const tp = new Eve('thread.js', os.cpus().length);
module.exports = async (data) => {
return await tp.run(data);
};
} else {
new Thread(async (data) => {
return await doSomething(data);
});
}
This example shows how to use Node Threads Pool to render Pug templates to HTML:
const pug = require('pug');
const os = require('os');
const {Eve, Thread, isMainThread} = require('node-threads-pool');
if(!isMainThread) {
const options = {};
new Thread(_data => {
const {template, data} = _data;
options.data = data;
return pug.renderFile(template, options);
});
} else {
const tp = new Eve(__filename, os.cpus().length);
module.exports = async (template, data) => {
return await tp.run({
template, data
});
};
}
npm run eve
Node-Threads-Pool is licensed under the MIT License. (see LICENSE)
FAQs
Node-Threads-Pool is a tool for creating thread pools in Node.js, making it easy to implement efficient parallel computing. With Node-Threads-Pool, you can easily create and manage multiple threads, allocate tasks to worker threads in the thread pool, and
The npm package node-threads-pool receives a total of 190 weekly downloads. As such, node-threads-pool popularity was classified as not popular.
We found that node-threads-pool demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.