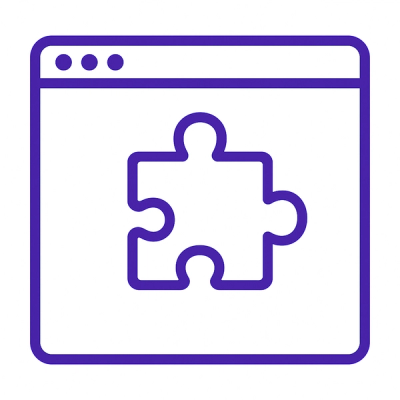
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
An RFC-3986 compliant and zero-dependencies Node.js module to parse URIs, punycode, punydecode, test URIs, URLs, Sitemap URLS, domains, IPs but also encode and decode URIs, URLs and Sitemap URLs
An RFC-3986 compliant and zero-dependencies Node.js module to parse URIs, punycode, punydecode, test URIs, URLs, Sitemap URLs, domains, IPs but also encode and decode URIs, URLs and Sitemap URLs.
Few libraries exist in the Node.js sphere providing helpers to deal with URIs and to be fully RFC-3986 compliant without any dependency.
Amongst being RFC-3986 compliant and having no dependency, node-uri aims to support Sitemap URLs for SEO purposes, fix url.parse
that automatically punycodes a host and cannot parse URIs other than URLs, fix encodeURI
and encodeURIComponent
native functions that relies on an old standard prior to RFC-3986 and fix decodeURI
that does not support IDNs and cannot properly work with encodeURI
since the function is based on an outdated standard.
The main features of this project are:
2838 assertions ensure parsing, encoding, decoding, checking URIs, URLs, IPs, domains are working as expected. This does not make this library a 100% reliable source. So if you find any errors, please feel free to report, contribute and help fixing any issues.
To make sure we properly understand the difference between an URI and an URL, these two are not exactly the same thing. An URI is an identifier of a specific resource. Like a page, a book, or a document. An URL is a special type of identifier that also tells you how to access it, such as HTTPs, FTP, etc. If the protocol (https, ftp, etc.) is either present or implied for a domain, you should call it an URL even though it’s also an URI.
npm install node-uri
npm i -S node-uri
Node.js >= Dubnium (10.22.1) could be required for some testing modules.
ESLint with Airbnb base rules. See Airbnb JavaScript Style Guide.
npm run lint
Mocha and Chai.
npm test
const uri = require('node-uri');
// uri is an object of functions
const {
punycode,
punydecode,
parseURI,
recomposeURI,
isDomainLabel,
isDomain,
isIP,
isIPv4,
isIPv6,
checkURI,
checkHttpURL,
checkHttpsURL,
checkHttpSitemapURL,
checkHttpsSitemapURL,
checkWebURL,
checkSitemapURL,
encodeURIComponentString,
encodeURIString,
encodeWebURL,
encodeSitemapURL,
decodeURIComponentString,
decodeURIString,
decodeWebURL,
decodeSitemapURL,
} = require('node-uri');
node-uri module exports an object of functions. You'll find the complete list of functions below.
uri
<Object> with the following functions.Returns the Punycode ASCII serialization of the domain. If domain is an invalid domain, the empty string is returned.
Note:
url.domainToASCII
does not support IPv6 only IPv4;url.domainToASCII
throws if no domain is provided or returns null
, undefined
, nan
for null
, undefined
or NaN
values which is not what to be expected.domain
<String>Examples:
punycode(); // ''
punycode('a.b.c.d.e.fg'); // 'a.b.c.d.e.fg'
punycode('xn--iñvalid.com'); // ''
punycode('中文.com'); // 'xn--fiq228c.com'
punycode('xn--fiq228c.com'); // 'xn--fiq228c.com'
punycode('2001:db8:85a3:8d3:1319:8a2e:370:7348'); // '2001:db8:85a3:8d3:1319:8a2e:370:7348'
punycode('127.0.0.1'); // '127.0.0.1'
punycode(undefined|null|NaN); // ''
Returns the Unicode serialization of the domain. If domain is an invalid domain, the empty string is returned.
Note:
url.domainToUnicode
does not support IPv6 only IPv4;url.domainToUnicode
throws if no domain is provided or returns null
, undefined
, nan
for null
, undefined
or NaN
values which is not what to be expected.domain
<String>Examples:
punydecode(); // ''
punydecode('a.b.c.d.e.fg'); // 'a.b.c.d.e.fg'
punydecode('xn--iñvalid.com'); // ''
punydecode('xn--fiq228c.com'); // '中文.com'
punydecode('中文.com'); // '中文.com'
punydecode('2001:db8:85a3:8d3:1319:8a2e:370:7348'); // '2001:db8:85a3:8d3:1319:8a2e:370:7348'
punydecode('127.0.0.1'); // '127.0.0.1'
punydecode(undefined|null|NaN); // ''
Parse a string to get URI components.
Support:
Note:
Generic syntax:
Example URIs:
Based on:
uri
<String>scheme
<String> The URI scheme. Default: null
authority
<String> The URI authority with the Punycode ASCII serialization of the domain. Default: null
authorityPunydecoded
<String> The URI authority with the Unicode serialization of the domain. Default: null
userinfo
<String> The URI userinfo. Default: null
host
<String> The URI authority's host with the Punycode ASCII serialization of the domain. Default: null
hostPunydecoded
<String> The URI authority's host with the Unicode serialization of the domain. Default: null
port
<Number> || <String> The URI authority's port. A string if not able to be parsed in an integer. Default: null
path
<String> The URI path. Default: null
pathqf
<String> The URI path, query and fragment. Default: null
query
<String> The URI query. Default: null
fragment
<String> The URI fragment. Default: null
href
<String> The URI recomposed. See recomposeURI. Default: null
Examples:
parseURI();
// {
// scheme: null,
// authority: null,
// authorityPunydecoded: null,
// userinfo: null,
// host: null,
// hostPunydecoded: null,
// port: null,
// path: null,
// pathqf: null,
// query: null,
// fragment: null,
// href: null,
// }
parseURI('foo://user:pass@xn--fiq228c.com:8042/over/there?name=ferret#nose');
// {
// scheme: 'foo',
// authority: 'user:pass@xn--fiq228c.com:8042',
// authorityPunydecoded: 'user:pass@中文.com:8042',
// userinfo: 'user:pass',
// host: 'xn--fiq228c.com',
// hostPunydecoded: '中文.com',
// port: 8042,
// path: '/over/there',
// pathqf: '/over/there?name=ferret#nose',
// query: 'name=ferret',
// fragment: 'nose',
// href: 'foo://user:pass@xn--fiq228c.com:8042/over/there?name=ferret#nose',
// }
parseURI('foo://user:pass@中文.com:80g42/over/there?name=ferret#nose');
// {
// scheme: 'foo',
// authority: 'user:pass@xn--fiq228c.com:80g42',
// authorityPunydecoded: 'user:pass@中文.com:80g42',
// userinfo: 'user:pass',
// host: 'xn--fiq228c.com',
// hostPunydecoded: '中文.com',
// port: '80g42',
// path: '/over/there',
// pathqf: '/over/there?name=ferret#nose',
// query: 'name=ferret',
// fragment: 'nose',
// href: 'foo://user:pass@xn--fiq228c.com:80g42/over/there?name=ferret#nose',
// }
parseURI('urn:isbn:0-486-27557-4');
// {
// scheme: 'urn',
// authority: null,
// authorityPunydecoded: null,
// userinfo: null,
// host: null,
// hostPunydecoded: null,
// port: null,
// path: 'isbn:0-486-27557-4',
// pathqf: 'isbn:0-486-27557-4',
// query: null,
// fragment: null
// href: 'urn:isbn:0-486-27557-4',
// }
parseURI('http://user:pass@[fe80::7:8%eth0]:8080');
// {
// scheme: 'http',
// authority: 'user:pass@[fe80::7:8%eth0]:8080',
// authorityPunydecoded: 'user:pass@[fe80::7:8%eth0]:8080',
// userinfo: 'user:pass',
// host: 'fe80::7:8%eth0',
// hostPunydecoded: 'fe80::7:8%eth0',
// port: 8080,
// path: '',
// pathqf: '',
// query: null,
// fragment: null,
// href: 'http://user:pass@[fe80::7:8%eth0]:8080/'
// }
Recompose an URI from its components with basic URI checking.
The empty string is returned if unable to recompose the URI.
Rules:
/
;//
;Support:
Note:
/
is added to any URI with a host and an empty path.Generic syntax:
Based on:
components
<Object>:
scheme
* <String> The URI scheme.userinfo
<String> The URI userinfo.host
<String> The URI authority's host.port
<Number> The URI authority's port.path
* <String> The URI path.query
<String> The URI query.fragment
<String> The URI fragment.Examples:
recomposeURI(); // ''
recomposeURI({
scheme: null,
userinfo: 'user:pass',
host: 'example.com',
port: 8080,
path: null,
query: 'a=b',
fragment: 'anchor',
}); // ''
recomposeURI({
scheme: 'foo',
userinfo: null,
host: null,
port: null,
path: '',
query: null,
fragment: null,
}); // 'foo:'
recomposeURI({
scheme: 'foo',
userinfo: 'user:pass',
host: 'bar.com',
port: 8080,
path: '/over/there',
query: 'a=b',
fragment: 'anchor',
}); // 'foo://user:pass@bar.com:8080/over/there?a=b#anchor'
recomposeURI({
scheme: 'foo',
userinfo: 'user:pass',
host: 'fe80::7:8%eth0',
port: '8080',
path: '/over/there',
query: 'a=b',
fragment: 'anchor',
}); // 'foo://user:pass@[fe80::7:8%eth0]:8080/over/there?a=b#anchor'
recomposeURI({
scheme: 'foo',
userinfo: '',
host: 'fe80::7:8%eth0',
port: '55g55',
path: '/over/there',
query: '',
fragment: '',
}); // 'foo://[fe80::7:8%eth0]/over/there'
Test a label is a valid domain label according to RFC-1034.
"Note that while upper and lower case letters are allowed in domain names, no significance is attached to the case. That is, two names with the same spelling but different case are to be treated as if identical."
By convention uppercased domain label will be considered invalid.
Rules:
Based on:
label
<String>Examples:
isDomainLabel('a'); // true
isDomainLabel('1a3'); // true
isDomainLabel('1-3'); // true
isDomainLabel('1-y'); // true
isDomainLabel(); // false
isDomainLabel('a'.repeat(64)); // false
isDomainLabel('A'); // false
isDomainLabel('-a'); // false
isDomainLabel('a-'); // false
isDomainLabel('-a'); // false
isDomainLabel('la--bel'); // false
isDomainLabel(undefined|null|NaN); // false
Test a name is a valid domain according to RFC-1034.
Supports Fully-Qualified Domain Name (FQDN) and Internationalized Domain Name (IDN).
Rules:
xn--
for IDNs if the ASCII serialization is a valid Punycode and has valid characters.Based on:
name
<String>Examples:
isDomain('a.b'); // true
isDomain('a.b.'); // true
isDomain('中文.com'); // true
isDomain('xn--fiq228c.com'); // true
isDomain('www.中文.com'); // true
isDomain(`${'a'.repeat(63)}.${'b'.repeat(63)}.${'c'.repeat(63)}.${'d'.repeat(63)}`); // true
isDomain(); // false
isDomain('a'); // false
isDomain('a.a'); // false
isDomain('a.b.a'); // false
isDomain('a.b.a'); // false
isDomain('中文.xn--fiq228c.com'); // false
isDomain('www.xn--hf.com'); // false
isDomain(`${'a'.repeat(63)}.${'b'.repeat(63)}.${'c'.repeat(63)}.${'d'.repeat(63)}.`); // false
isDomain('xn--\'-6xd.com') // false even though xn--'-6xd is a valid Punycode for ॐ but has an invalid character
Test a string is a valid IP.
Supports IPv4 and IPv6.
ip
<String>Examples:
isIP('23.71.254.72'); // true
isIP('1:2:3:4::6:7:8'); // true
isIP(); // false
isIP('100..100.100.100.'); // false
isIP('3ffe:b00::1::a'); // false
Test a string is a valid IPv4.
ip
<String>Examples:
isIPv4('8.8.8.8'); // true
isIPv4('1:2::8'); // false
isIPv4(); // false
Test a string is a valid IPv6.
ip
<String>Examples:
isIPv6('2001:0000:1234:0000:0000:C1C0:ABCD:0876'); // true
isIPv6('212.58.241.131'); // false
isIPv6(); // false
Check an URI is valid according to RFC-3986.
Rules:
/
;//
;Generic syntax:
Based on:
uri
<String>scheme
<String> The URI scheme.authority
<String> The URI authority with the Punycode ASCII serialization of the domain. Default: null
authorityPunydecoded
<String> The URI authority with the Unicode serialization of the domain. Default: null
userinfo
<String> The URI userinfo. Default: null
host
<String> The URI authority's host with the Punycode ASCII serialization of the domain. Default: null
hostPunydecoded
<String> The URI authority's host with the Unicode serialization of the domain. Default: null
port
<Number> || <String> The URI authority's port. A string if not able to be parsed in an integer. Default: null
path
<String> The URI path.pathqf
<String> The URI path, query and fragment.query
<String> The URI query. Default: null
fragment
<String> The URI fragment. Default: null
href
<String> The URI recomposed. Default: null
valid
<Boolean> Whether the URI is valid. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME_CHAR
URI_INVALID_USERINFO_CHAR
URI_INVALID_PORT
URI_INVALID_PATH_CHAR
URI_INVALID_QUERY_CHAR
URI_INVALID_FRAGMENT_CHAR
URI_INVALID_PERCENT_ENCODING
Examples:
checkURI(); // throws URIError with code URI_INVALID_TYPE
checkURI('://example.com'); // throws URIError with code URI_MISSING_SCHEME
checkURI('foo:////bar'); // throws URIError with code URI_INVALID_PATH
checkURI('foo://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
checkURI('fôo:bar'); // throws URIError with code URI_INVALID_SCHEME_CHAR
checkURI('foo://üser:pass@bar.com'); // throws URIError with code URI_INVALID_USERINFO_CHAR
checkURI('foo://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
checkURI('foo://bar.com/°'); // throws URIError with code URI_INVALID_PATH_CHAR
checkURI('foo://bar.com/over/there?quêry=5'); // throws URIError with code URI_INVALID_QUERY_CHAR
checkURI('foo://bar.com/over/there?query=5#anch#r'); // throws URIError with code URI_INVALID_FRAGMENT_CHAR
checkURI('http://www.bar.baz/foo%2') // throws URIError with code URI_INVALID_PERCENT_ENCODING
checkURI('foo://user:pass@xn--fiq228c.com:8042/over/there?name=ferret#nose');
// {
// scheme: 'foo',
// authority: 'user:pass@xn--fiq228c.com:8042',
// authorityPunydecoded: 'user:pass@中文.com:8042',
// userinfo: 'user:pass',
// host: 'xn--fiq228c.com',
// hostPunydecoded: '中文.com',
// port: 8042,
// path: '/over/there',
// pathqf: '/over/there?name=ferret#nose',
// query: 'name=ferret',
// fragment: 'nose',
// href: 'foo://user:pass@xn--fiq228c.com:8042/over/there?name=ferret#nose',
// valid: true
// }
Check an URI is a valid HTTP URL.
Rules:
http
or HTTP
;Based on:
uri
<String>scheme
<String> The URL scheme.authority
<String> The URL authority with the Punycode ASCII serialization of the domain. Default: null
authorityPunydecoded
<String> The URL authority with the Unicode serialization of the domain. Default: null
userinfo
<String> The URL userinfo. Default: null
host
<String> The URL authority's host with the Punycode ASCII serialization of the domain. Default: null
hostPunydecoded
<String> The URL authority's host with the Unicode serialization of the domain. Default: null
port
<Number> || <String> The URL authority's port. A string if not able to be parsed in an integer. Default: null
path
<String> The URL path.pathqf
<String> The URI path, query and fragment.query
<String> The URL query. Default: null
fragment
<String> The URL fragment. Default: null
href
<String> The URL recomposed. Default: null
valid
<Boolean> Whether the URL is valid. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_USERINFO_CHAR
URI_INVALID_PORT
URI_INVALID_PATH_CHAR
URI_INVALID_QUERY_CHAR
URI_INVALID_FRAGMENT_CHAR
URI_INVALID_PERCENT_ENCODING
URI_INVALID_SCHEME
URI_MISSING_AUTHORITY
URI_MAX_LENGTH_URL
Examples:
checkHttpURL(); // throws URIError with code URI_INVALID_TYPE
checkHttpURL('://example.com'); // throws URIError with code URI_MISSING_SCHEME
checkHttpURL('http:////bar'); // throws URIError with code URI_INVALID_PATH
checkHttpURL('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
checkHttpURL('http://üser:pass@bar.com'); // throws URIError with code URI_INVALID_USERINFO_CHAR
checkHttpURL('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
checkHttpURL('http://bar.com/°'); // throws URIError with code URI_INVALID_PATH_CHAR
checkHttpURL('http://bar.com/over/there?quêry=5'); // throws URIError with code URI_INVALID_QUERY_CHAR
checkHttpURL('http://bar.com/over/there?query=5#anch#r'); // throws URIError with code URI_INVALID_FRAGMENT_CHAR
checkHttpURL('http://www.bar.baz/foo%2') // throws URIError with code URI_INVALID_PERCENT_ENCODING
checkHttpURL('httê://bar.com:8080'); // throws URIError with code URI_INVALID_SCHEME
checkHttpURL('http:isbn:0-486-27557-4'); // throws URIError with code URI_MISSING_AUTHORITY
checkHttpURL(`http://example.com/${'path'.repeat(2040)}`); // throws URIError with code URI_MAX_LENGTH_URL
checkHttpURL('http://user:pass@xn--fiq228c.com:8042/over/there?name=ferret#nose');
// {
// scheme: 'http',
// authority: 'user:pass@xn--fiq228c.com:8042',
// authorityPunydecoded: 'user:pass@中文.com:8042',
// userinfo: 'user:pass',
// host: 'xn--fiq228c.com',
// hostPunydecoded: '中文.com',
// port: 8042,
// path: '/over/there',
// pathqf: '/over/there?name=ferret#nose',
// query: 'name=ferret',
// fragment: 'nose',
// href: 'http://user:pass@xn--fiq228c.com:8042/over/there?name=ferret#nose',
// valid: true
// }
Check an URI is a valid HTTPS URL. Same behavior than checkHttpURL except scheme must be https
or HTTPS
.
Check an URI is a valid HTTP URL to be used in an XML sitemap file.
For text sitemap please refer to checkHttpURL as there is no need to escape entities but URL must be in lowercase.
Rules:
http
;Valid URI characters to be escaped or percent-encoded in a sitemap URL:
Character | Value | Escape Code |
---|---|---|
Ampersand | & | & |
Single Quote | ' | ' |
Asterisk | * | %2A |
Based on:
uri
<String>scheme
<String> The URL scheme.authority
<String> The URL authority with the Punycode ASCII serialization of the domain. Default: null
authorityPunydecoded
<String> The URL authority with the Unicode serialization of the domain. Default: null
userinfo
<String> The URL userinfo. Default: null
host
<String> The URL authority's host with the Punycode ASCII serialization of the domain. Default: null
hostPunydecoded
<String> The URL authority's host with the Unicode serialization of the domain. Default: null
port
<Number> || <String> The URL authority's port. A string if not able to be parsed in an integer. Default: null
path
<String> The URL path.pathqf
<String> The URI path, query and fragment.query
<String> The URL query. Default: null
fragment
<String> The URL fragment. Default: null
href
<String> The URL recomposed. Default: null
valid
<Boolean> Whether the URL is valid. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_USERINFO_CHAR
URI_INVALID_PORT
URI_INVALID_CHAR
URI_INVALID_PATH_CHAR
URI_INVALID_QUERY_CHAR
URI_INVALID_FRAGMENT_CHAR
URI_INVALID_PERCENT_ENCODING
URI_INVALID_SITEMAP_ENCODING
URI_INVALID_SCHEME
URI_MISSING_AUTHORITY
URI_MAX_LENGTH_URL
Examples:
checkHttpSitemapURL(); // throws URIError with code URI_INVALID_TYPE
checkHttpSitemapURL('://example.com'); // throws URIError with code URI_MISSING_SCHEME
checkHttpSitemapURL('http:////bar'); // throws URIError with code URI_INVALID_PATH
checkHttpSitemapURL('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
checkHttpSitemapURL('http://*ser:pass@bar.com'); // throws URIError with code URI_INVALID_USERINFO_CHAR
checkHttpSitemapURL('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
checkHttpSitemapURL('hTtp://bar.com/Path'); // throws URIError with code URI_INVALID_CHAR
checkHttpSitemapURL('http://bAr.com/Path'); // throws URIError with code URI_INVALID_CHAR
checkHttpSitemapURL('http://bar.com/Path'); // throws URIError with code URI_INVALID_CHAR
checkHttpSitemapURL('http://bar.com/path\''); // throws URIError with code URI_INVALID_PATH_CHAR
checkHttpSitemapURL('http://bar.com/over/there?a=5&b=9'); // throws URIError with code URI_INVALID_QUERY_CHAR
checkHttpSitemapURL('http://bar.com/over/there?a=5#anch*r'); // throws URIError with code URI_INVALID_FRAGMENT_CHAR
checkHttpSitemapURL('http://www.bar.baz/foo%2') // throws URIError with code URI_INVALID_PERCENT_ENCODING
checkHttpSitemapURL('http://www.bar.baz/foo?a=5&am;b=9') // throws URIError with code URI_INVALID_SITEMAP_ENCODING
checkHttpSitemapURL('hêtp://bar.com:8080'); // throws URIError with code URI_INVALID_SCHEME
checkHttpSitemapURL('http:isbn:0-486-27557-4'); // throws URIError with code URI_MISSING_AUTHORITY
checkHttpSitemapURL(`http://example.com/${'path'.repeat(2040)}`); // throws URIError with code URI_MAX_LENGTH_URL
checkHttpSitemapURL('http://user:pass@xn--fiq228c.com:8042/over/there?name=ferret&catch=rabbits#nose');
// {
// scheme: 'http',
// authority: 'user:pass@xn--fiq228c.com:8042',
// authorityPunydecoded: 'user:pass@中文.com:8042',
// userinfo: 'user:pass',
// host: 'xn--fiq228c.com',
// hostPunydecoded: '中文.com',
// port: 8042,
// path: '/over/there',
// pathqf: '/over/there?name=ferret&catch=rabbits#nose',
// query: 'name=ferret&catch=rabbits',
// fragment: 'nose',
// href: 'http://user:pass@xn--fiq228c.com:8042/over/there?name=ferret&catch=rabbits#nose',
// valid: true
// }
Check an URI is a valid HTTPS URL to be used in an XML sitemap file. Same behavior than checkHttpSitemapURL except scheme must be https
.
Check an URI is a valid HTTP or HTTPS URL. Same behavior than checkHttpURL except scheme can be http
/HTTP
or https
/HTTPS
.
Check an URI is a valid HTTP or HTTPS URL to be used in an XML sitemap file. Same behavior than checkHttpSitemapURL except scheme can be http
or https
.
Encode an URI component according to RFC-3986.
Support:
Note:
userinfo
, path
, query
and fragment
components can be encoded with specific rules for each type regarding valid characters (RFC-3986);scheme
and authority
(host and port) can never have escaped or percent-encoded characters;Generic syntax:
Based on:
component
<String>options
<Object>:
type
<String> The component type. If no type is provided native function encodeURIComponent will be used to encode each character. Default: none
One of:
userinfo
path
query
fragment
lowercase
<Boolean> Whether the component should be returned in lowercase. Default: false
sitemap
<Boolean> Whether to escape Sitemap's special characters. See checkHttpSitemapURL.Examples:
encodeURIComponentString(); // ''
encodeURIComponentString(''); // ''
encodeURIComponentString('cômpön€nt'); // 'c%C3%B4mp%C3%B6n%E2%82%ACnt'
encodeURIComponentString('AbC'); // 'AbC'
encodeURIComponentString('AbC', { lowercase: true }); // 'abc'
encodeURIComponentString('*'); // '*'
encodeURIComponentString('*', { sitemap: true }); // '%2A'
// it is highly recommended to use a component type
encodeURIComponentString('A#/?@[]&\'*'); // 'A%23%2F%3F%40%5B%5D%26\'*' (native function, outdated standard)
encodeURIComponentString('A#/?@[]&\'*', { type: 'userinfo' }); // 'A%23%2F%3F%40%5B%5D&\'*' (RFC-3986 characters in userinfo)
encodeURIComponentString('A#/?@[]&\'*', { type: 'path' }); // 'A%23/%3F@%5B%5D&\'*'
encodeURIComponentString('A#/?@[]&\'*', { type: 'query' }); // 'A%23/?@%5B%5D&\'*'
encodeURIComponentString('A#/?@[]&\'*', { type: 'fragment' }); // 'A%23/?@%5B%5D&\'*'
encodeURIComponentString('A#/?@[]&\'*', { type: 'fragment', sitemap: true }); // 'a%23/?@%5B%5D&'%2A'
Encode an URI string according to RFC-3986 with basic checking.
Checked:
Support:
Note:
userinfo
, path
, query
and fragment
can be percent-encoded;encodeURI
encodes string according to RFC-2396 which is outdated;encodeURI
also encodes scheme and host that cannot have
percend-encoded characters;[]
to represent IPv6 host;Generic syntax:
Based on:
uri
<String>options
<Object>:
lowercase
<Boolean> Whether the uri should be returned in lowercase. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME_CHAR
URI_INVALID_PORT
Examples:
encodeURIString(); // throws URIError with code URI_INVALID_TYPE
encodeURIString('://example.com'); // throws URIError with code URI_MISSING_SCHEME
encodeURIString('http:////bar'); // throws URIError with code URI_INVALID_PATH
encodeURIString('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
encodeURIString('hôtp:bar'); // throws URIError with code URI_INVALID_SCHEME_CHAR
encodeURIString('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
encodeURIString('HTTPS://WWW.中文.COM./Over/There?a=B&b=c#Anchor'); // 'https://www.xn--fiq228c.com./Over/There?a=B&b=c#Anchor'
encodeURIString('HTTPS://WWW.中文.COM./Over/There?a=B&b=c#Anchor', { lowercase: true }); // 'https://www.xn--fiq228c.com./over/there?a=b&b=c#anchor'
encodeURIString('foo://usër:pâss@bar.baz:8080/Ovër There?ù=B&b=c#Anchôr'); // 'foo://us%C3%ABr:p%C3%A2ss@bar.baz:8080/Ov%C3%ABr%20There?%C3%B9=B&b=c#Anch%C3%B4r'
Encode an URI string with basic checking based on RFC-3986 standard applied to HTTP and HTTPS URLs.
Uses a fixed encodeURI function to be RFC-3986 compliant.
Checked:
http
/HTTP
or https
/HTTPS
;Support:
Note:
userinfo
, path
, query
and fragment
can be percent-encoded;encodeURI
encodes string according to RFC-2396 which is outdated;encodeURI
also encodes scheme and host that cannot have
percend-encoded characters;[]
to represent IPv6 host;Generic syntax:
Based on:
uri
<String>options
<Object>:
lowercase
<Boolean> Whether the uri should be returned in lowercase. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME
URI_INVALID_PORT
URI_MISSING_AUTHORITY
URI_MAX_LENGTH_URL
Examples:
encodeWebURL(); // throws URIError with code URI_INVALID_TYPE
encodeWebURL('://example.com'); // throws URIError with code URI_MISSING_SCHEME
encodeWebURL('http:////bar'); // throws URIError with code URI_INVALID_PATH
encodeWebURL('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
encodeWebURL('ftp://bar.baz'); // throws URIError with code URI_INVALID_SCHEME
encodeWebURL('hôtp://bar.baz'); // throws URIError with code URI_INVALID_SCHEME
encodeWebURL('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
encodeWebURL('http:isbn:0-486-27557-4'); // throws URIError with code URI_MISSING_AUTHORITY
encodeWebURL(`http://example.com/${'path'.repeat(2040)}`); // throws URIError with code URI_MAX_LENGTH_URL
encodeWebURL('HTTPS://WWW.中文.COM./Over/There?a=B&b=c#Anchor'); // 'https://www.xn--fiq228c.com./Over/There?a=B&b=c#Anchor'
encodeWebURL('HTTPS://WWW.中文.COM./Over/There?a=B&b=c#Anchor', { lowercase: true }); // 'https://www.xn--fiq228c.com./over/there?a=b&b=c#anchor'
encodeWebURL('http://usër:pâss@bar.baz:8080/Ovër There?ù=B&b=c#Anchôr'); // 'http://us%C3%ABr:p%C3%A2ss@bar.baz:8080/Ov%C3%ABr%20There?%C3%B9=B&b=c#Anch%C3%B4r'
Encode an URI string with basic checking based on RFC-3986 standard applied to HTTP and HTTPS URLs and sitemap requirements regarding special characters to escape.
Uses a fixed encodeURI function to be RFC-3986 compliant.
Checked:
http
/HTTP
or https
/HTTPS
;Support:
Note:
userinfo
, path
, query
and fragment
can be percent-encoded;encodeURI
encodes string according to RFC-2396 which is outdated;encodeURI
also encodes scheme and host that cannot have
percend-encoded characters;[]
to represent IPv6 host;Generic syntax:
Based on:
uri
<String>URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME
URI_INVALID_PORT
URI_MISSING_AUTHORITY
URI_MAX_LENGTH_URL
Examples:
encodeSitemapURL(); // throws URIError with code URI_INVALID_TYPE
encodeSitemapURL('://example.com'); // throws URIError with code URI_MISSING_SCHEME
encodeSitemapURL('http:////bar'); // throws URIError with code URI_INVALID_PATH
encodeSitemapURL('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
encodeSitemapURL('ftp://bar.baz'); // throws URIError with code URI_INVALID_SCHEME
encodeSitemapURL('hôtp://bar.baz'); // throws URIError with code URI_INVALID_SCHEME
encodeSitemapURL('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
encodeSitemapURL('http:isbn:0-486-27557-4'); // throws URIError with code URI_MISSING_AUTHORITY
encodeSitemapURL(`http://example.com/${'path'.repeat(2040)}`); // throws URIError with code URI_MAX_LENGTH_URL
encodeSitemapURL('http://user:p\'âss@bar.baz/it\'s *ver/there?a=b&b=c#anch*r'); // 'http://user:p'%C3%A2ss@bar.baz/it's%20%2Aver/there?a=b&b=c#anch%2Ar'
Decode an URI component string.
Native function decodeURIComponent
could throw and to be consistent with encodeURIComponentString the empty string is returned if unable to decode.
Support:
Based on:
component
<String>options
<Object>:
lowercase
<Boolean> Whether the component should be returned in lowercase. Default: false
sitemap
<Boolean> Whether to decode Sitemap's escape codes. See checkHttpSitemapURL.Examples:
decodeURIComponentString(); // ''
decodeURIComponentString(''); // ''
decodeURIComponentString('AbC'); // 'AbC'
decodeURIComponentString('AbC', { lowercase: true }); // 'abc'
decodeURIComponentString('%2A'); // '*'
decodeURIComponentString(''&%2A', { sitemap: true }); // '\'&*'
decodeURIComponentString('SITE&maP', { sitemap: true, lowercase: true }); // 'site&map'
Decode an URI string according to RFC-3986 with basic checking.
Checked:
Support:
Note:
userinfo
, path
, query
or fragment
component cannot be decoded, it will be ignored;decodeURI
does not support IDNs and cannot properly work with encodeURI
since the function is based on an outdated standard;Based on:
uri
<String>options
<Object>:
lowercase
<Boolean> Whether the uri should be returned in lowercase. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME_CHAR
URI_INVALID_PORT
Examples:
decodeURIString(); // throws URIError with code URI_INVALID_TYPE
decodeURIString('://example.com'); // throws URIError with code URI_MISSING_SCHEME
decodeURIString('http:////bar'); // throws URIError with code URI_INVALID_PATH
decodeURIString('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
decodeURIString('hôtp:bar'); // throws URIError with code URI_INVALID_SCHEME_CHAR
decodeURIString('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
decodeURIString('http://user%:pass@xn--fiq228c.com/%?query=%E0%A5%90#anch#or'); // 'http://中文.com/?query=ॐ'
decodeURIString('HTTPS://WWW.xn--fiq228c.COM./Over/There?a=B&b=c#Anchor'); // 'https://www.中文.com./Over/There?a=B&b=c#Anchor'
decodeURIString('HTTPS://WWW.xn--fiq228c.COM./Over/There?a=B&b=c#Anchor', { lowercase: true }); // 'https://www.中文.com./over/there?a=b&b=c#anchor'
decodeURIString('foo://us%C3%ABr:p%C3%A2ss@bar.baz:8080/Ov%C3%ABr%20There?%C3%B9=B&b=c#Anch%C3%B4r'); // 'foo://usër:pâss@bar.baz:8080/Ovër There?ù=B&b=c#Anchôr'
Decode an URI string with basic checking based on RFC-3986 standard applied to HTTP and HTTPS URLs.
Uses a fixed decodeURI function to be RFC-3986 compliant.
Checked:
http
/HTTP
or https
/HTTPS
;Support:
Note:
userinfo
, path
, query
or fragment
component cannot be decoded, it will be ignored;decodeURI
does not support IDNs and cannot properly work with encodeURI
since the function is based on an outdated standard;Based on:
uri
<String>options
<Object>:
lowercase
<Boolean> Whether the uri should be returned in lowercase. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME
URI_INVALID_PORT
URI_MISSING_AUTHORITY
URI_MAX_LENGTH_URL
Examples:
decodeWebURL(); // throws URIError with code URI_INVALID_TYPE
decodeWebURL('://example.com'); // throws URIError with code URI_MISSING_SCHEME
decodeWebURL('http:////bar'); // throws URIError with code URI_INVALID_PATH
decodeWebURL('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
decodeWebURL('ftp://bar.com'); // throws URIError with code URI_INVALID_SCHEME
decodeWebURL('hôtp://bar.com'); // throws URIError with code URI_INVALID_SCHEME
decodeWebURL('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
decodeWebURL('http:isbn:0-486-27557-4'); // throws URIError with code URI_MISSING_AUTHORITY
decodeWebURL(`http://example.com/${'path'.repeat(2040)}`); // throws URIError with code URI_MAX_LENGTH_URL
decodeWebURL('http://user%:pass@xn--fiq228c.com/%?query=%E0%A5%90#anch#or'); // 'http://中文.com/?query=ॐ'
decodeWebURL('HTTPS://WWW.xn--fiq228c.COM./Over/There?a=B&b=c#Anchor'); // 'https://www.中文.com./Over/There?a=B&b=c#Anchor'
decodeWebURL('HTTPS://WWW.xn--fiq228c.COM./Over/There?a=B&b=c#Anchor', { lowercase: true }); // 'https://www.中文.com./over/there?a=b&b=c#anchor'
decodeWebURL('http://us%C3%ABr:p%C3%A2ss@bar.baz:8080/Ov%C3%ABr%20There?%C3%B9=B&b=c#Anch%C3%B4r'); // 'http://usër:pâss@bar.baz:8080/Ovër There?ù=B&b=c#Anchôr'
Decode an URI string with basic checking based on RFC-3986 standard applied to HTTP and HTTPS URLs and sitemap requirements regarding escape codes to decode.
Uses a fixed decodeURI function to be RFC-3986 compliant.
Checked:
http
/HTTP
or https
/HTTPS
;Support:
Note:
userinfo
, path
, query
or fragment
component cannot be decoded, it will be ignored;decodeURI
does not support IDNs and cannot properly work with encodeURI
since the function is based on an outdated standard;Based on:
uri
<String>options
<Object>:
lowercase
<Boolean> Whether the uri should be returned in lowercase. Default: false
URI_INVALID_TYPE
URI_MISSING_SCHEME
URI_EMPTY_SCHEME
URI_MISSING_PATH
URI_INVALID_PATH
URI_INVALID_HOST
URI_INVALID_SCHEME
URI_INVALID_PORT
URI_MISSING_AUTHORITY
URI_MAX_LENGTH_URL
Examples:
decodeSitemapURL(); // throws URIError with code URI_INVALID_TYPE
decodeSitemapURL('://example.com'); // throws URIError with code URI_MISSING_SCHEME
decodeSitemapURL('http:////bar'); // throws URIError with code URI_INVALID_PATH
decodeSitemapURL('http://xn--iñvalid.com'); // throws URIError with code URI_INVALID_HOST
decodeSitemapURL('ftp://bar.com'); // throws URIError with code URI_INVALID_SCHEME
decodeSitemapURL('hôtp://bar.com'); // throws URIError with code URI_INVALID_SCHEME
decodeSitemapURL('http://bar.com:80g80'); // throws URIError with code URI_INVALID_PORT
decodeSitemapURL('http:isbn:0-486-27557-4'); // throws URIError with code URI_MISSING_AUTHORITY
decodeSitemapURL(`http://example.com/${'path'.repeat(2040)}`); // throws URIError with code URI_MAX_LENGTH_URL
decodeSitemapURL('http://user%:pass@xn--fiq228c.com/%?query=%E0%A5%90#anch#or'); // 'http://中文.com/?query=ॐ'
decodeSitemapURL('HTTP://bar.BAZ/IT'S%20OVER%2Athere%2A?a=b&c=d'); // 'http://bar.baz/IT\'S OVER*there*?a=b&c=d'
decodeSitemapURL('http://bar.baz/IT'S%20OVER%2Athere%2A?A=b&c=D', { lowercase: true }); // 'http://bar.baz/it\'s over*there*?a=b&c=d'
Errors emitted by node-uri are native URIError with an additional code property:
{
name,
code,
message,
stack,
}
name | code | description | module |
---|---|---|---|
URIError | |||
URI_INVALID_TYPE | URI variable type is not valid | src/checkers | |
URI_MISSING_SCHEME | URI scheme is missing | src/checkers | |
URI_EMPTY_SCHEME | URI scheme is empty | src/checkers | |
URI_INVALID_SCHEME | URI scheme is not valid | src/checkers src/decoders src/encoders | |
URI_INVALID_SCHEME_CHAR | URI scheme contains an invalid character | src/checkers src/decoders src/encoders | |
URI_MISSING_PATH | URI path is missing | src/checkers | |
URI_INVALID_PATH | URI path is not valid based on RFC-3986 | src/checkers | |
URI_MISSING_AUTHORITY | URI authority is missing | src/checkers src/decoders src/encoders | |
URI_INVALID_HOST | URI host is not valid IP or domain | src/checkers | |
URI_INVALID_PORT | URI port is not a number | src/checkers src/decoders src/encoders | |
URI_INVALID_CHAR | URI contains an invalid character | src/checkers | |
URI_INVALID_USERINFO_CHAR | URI userinfo contains an invalid character | src/checkers | |
URI_INVALID_PATH_CHAR | URI path contains an invalid character | src/checkers | |
URI_INVALID_QUERY_CHAR | URI query contains an invalid character | src/checkers | |
URI_INVALID_FRAGMENT_CHAR | URI fragment contains an invalid character | src/checkers | |
URI_INVALID_PERCENT_ENCODING | A percent-encoding character is not valid | src/checkers | |
URI_INVALID_SITEMAP_ENCODING | URI contains an invalid sitemap escape code | src/checkers | |
URI_MAX_LENGTH_URL | Maximum URL allowed length of 2048 characters has been reached | src/checkers |
This project has a Code of Conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
Please have a look at our TODO for any work in progress.
Please take also a moment to read our Contributing Guidelines if you haven't yet done so.
Please see our Support page if you have any questions or for any help needed.
For any security concerns or issues, please visit our Security Policy page.
MIT.
1.1.2 - delivery @06/06/2023
FAQs
An RFC-3986 compliant and zero-dependencies Node.js module to parse URIs, punycode, punydecode, test URIs, URLs, Sitemap URLS, domains, IPs but also encode and decode URIs, URLs and Sitemap URLs
We found that node-uri demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.