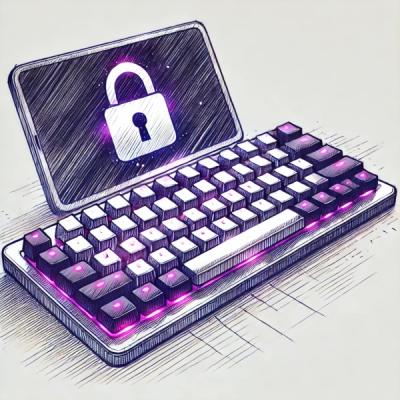
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
node-vk-bot-api
Advanced tools
Readme
🤖 VK bot framework for Node.js, based on Bots Long Poll API.
$ npm i node-vk-bot-api@2 -S # bots longpoll api
$ npm i node-vk-bot-api@1 -S # user longpoll api
const VkBot = require('node-vk-bot-api')
const bot = new VkBot({
token: process.env.TOKEN,
group_id: process.env.GROUP_ID
})
bot.command('/start', (ctx) => {
ctx.reply('Hello!')
})
bot.startPolling()
There's a few simple examples.
Create bot.
const bot = new VkBot({
token: process.env.TOKEN,
group_id: process.env.GROUP_ID
})
Add simple middleware.
bot.use((ctx, next) => {
ctx.message.timestamp = new Date().getTime()
next()
})
Add middlewares with triggers for message_new
event.
bot.command('start', (ctx) => {
ctx.reply('Hello!')
})
Add middlewares with triggers for selected events.
bot.event('message_edit', (ctx) => {
ctx.reply('Your message was editted')
})
Add reserved middlewares without triggers.
bot.on((ctx) => {
ctx.reply('No commands for you.')
})
Send message to user.
// Simple usage
bot.sendMessage(145003487, 'Hello!', 'photo1_1')
// Advanced usage
bot.sendMessage(145003487, {
message: 'Hello!',
lat: 59.939095,
lng: 30.315868
})
Start polling with given timeout (25 by default).
bot.startPolling()
Helper method for reply to the current user.
bot.command('start', (ctx) => {
ctx.reply('Hello!')
})
Add keyboard in message.
const VkBot = require('node-vk-bot-api')
const Markup = require('node-vk-bot-api/lib/markup')
const bot = new VkBot({
token: process.env.TOKEN,
group_id: process.env.GROUP_ID,
})
bot.command('/sport', (ctx) => {
ctx.reply('Select your sport', null, Markup
.keyboard([
'Football',
'Basketball',
])
.oneTime())
})
bot.command('/mood', (ctx) => {
ctx.reply('How are you doing?', null, Markup
.keyboard([
[
Markup.button('Normally', 'primary'),
],
[
Markup.button('Fine', 'positive'),
Markup.button('Bad', 'negative'),
],
]))
})
Store anything for current user in local (or redis) memory.
const VkBot = require('node-vk-bot-api')
const Session = require('node-vk-bot-api/lib/session')
const bot = new VkBot({
token: process.env.TOKEN,
group_id: process.env.GROUP_ID,
})
const session = new Session()
bot.use(session.middleware())
bot.on((ctx) => {
ctx.session.counter = ctx.session.counter || 0
ctx.session.counter++
ctx.reply(`You wrote ${ctx.session.counter} messages.`)
})
bot.startPolling()
key
: Context property name (default: session
)getSessionKey
: Getter for session keygetSessionKey(ctx)
const getSessionKey = (ctx) => {
return `${ctx.message.from_id}:${ctx.message.from_id}`
}
MIT.
FAQs
Unknown package
We found that node-vk-bot-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.