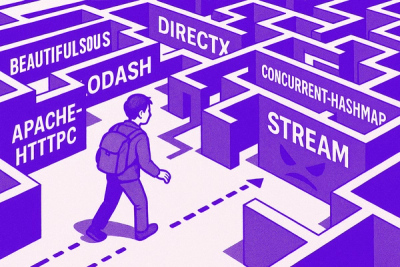
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
OneEntry Headless CMS SDK is an SDK that provides an easy way to interact with the OneEntry Headless CMS API.
Visit the official AsyncModules website at https://oneentry.cloud to learn more about the AsyncModules Headless CMS.
To get started with AsyncModules, sign up for an account at https://account.oneentry.cloud/authentication/register.
To install the AsyncModules Headless CMS SDK in your project, run the following command:
npm install oneentry
To use the AsyncModules Headless CMS SDK in your project, import the defineOneEntry function:
import { defineOneEntry } from 'oneentry'
const {
Admins,
AttributesSets,
AuthProvider,
Blocks,
Events,
Forms,
FormData,
FileUploading,
GeneralTypes,
IntegrationCollections,
Locales,
Menus,
Orders,
Pages,
Products,
ProductStatuses,
System,
Templates,
TemplatePreviews,
Users,
WS
} = defineOneEntry('your-url');
Or
const api = defineOneEntry('your-url');
The second parameter of the constructor takes the 'config'. It contains the following values:
'token' - Set the token key if your project secure "Security API Token". If you are using certificate protection, do not pass this variable. You can read more about the security of your project here.
'langCode' - Set the "langCode" to set the default language. By specifying this parameter once, you don't have to pass the langCode to the methods ONEENTRY API. If you have not passed the default language, it will be set "en_US".
'traficLimit' - Some methods use more than one request to the CMS so that the data you receive is complete and easy to work with. Pass the value "true" for this parameter to save traffic and decide for yourself what data you need. The default value "false".
'auth' - An object with authorization settings. By default, the SDK is configured to work with tokens inside the user's session and does not require any additional work from you. At the same time, the SDK does not store the session state between sessions. If you are satisfied with such settings, do not pass the variable 'auth' at all.
The 'auth' contains the following settings:
'refreshToken' - The user's refresh token. Transfer it here from the repository to restore the user's session during initialization.
'saveFunction' - A function that works with the update refresh token. If you want to store the token between sessions, for example in local storage, pass a function here that does this. The function must accept a parameter to which the string with the token will be passed.
'customAuth' - If you want to configure authorization and work with tokens yourself, set this flag to true. If you want to use the sdk settings, set it to false or do not transfer it at all.
An example of a configuration with token protection and automatic authentication that stores state between sessions
const tokenFunction = (token) => {
localStorage.setItem('refreshToken', token)
}
const api = defineOneEntry('https://my-project.oneentry.cloud', {
token:'my-token',
langCode:'en_US',
auth: {
refreshToken: localStorage.getItem('refreshToken'),
saveFunction: tokenFunction
}
})
An example of a configuration that is protected with a certificate allows you to configure the authorization system yourself and saves data on requests.
const api = defineOneEntry('https://my-project.oneentry.cloud', {
langCode:'en_US',
traficLimit: true,
auth: {
customAuth: true,
refreshToken: localStorage.getItem('refreshToken')
}
})
If you have chosen to configure tokens yourself, you can pass the token to the method as follows. The intermediate method allows you to pass an access token to the request. Then call the required method. This method (setAccessToken) should not be called if the method does not require user authorization.
const user = api.Users.setAccessToken('my.access.token').getUser()
If you chose token protection to ensure connection security, just pass your token to the function as an optional parameter.
You can get a token as follows
You can also connect a tls certificate to protect your project. In this case, do not pass the "token" at all. When using the certificate, set up a proxy in your project. Pass an empty string as an url parameter. Learn more about security
const saveTokenFromLocalStorage = (token) => {
localStorage.setItem('refreshToken', token)
}
const api = defineOneEntry('your-url', {
token: 'my-token',
langCode:'my-langCode',
auth: {
customAuth: false,
userToken: 'rerfesh.token',
saveFunction: saveTokenFromLocalStorage
}
});
If you want to escape errors inside the sc, leave the "errors" property by default. In this case, you will receive either the entity data or the error object. You need to do a type check. for example, by checking the statusCode property with ".hasOwnProperty"
However, if you want to use the construction "try {} catch(e) {}", set the property "isShell" to the value "false". In this case, you need to handle the error using "try {} catch(e) {}".
Also, you can pass custom functions that will be called inside the sdk with the appropriate error code. These functions receive an error object as an argument. You can process it yourself.
const api = defineOneEntry('your-url', {
token: 'my-token',
langCode:'my-langCode',
errors: {
isShell: false,
customErrors: {
400: (error) => console.error(error.message),
404: (error) => console.error(error.message),
500: (error) => console.error(error.message)
}
}
});
Now you can use the following links to jump to specific entries:
The "Administrators" module is designed for creating and managing administrators. An administrator is a user who can add, update, and edit information on the website or in the mobile application.
const { Admins } = defineOneEntry('your-url');
Method accept the body as a parameter for filtering. If you don't want to set up filtering/sorting, pass an empty array or don't pass anything.
Parameters:
const body = [
{
"attributeMarker": "num",
"conditionMarker": "mth",
"conditionValue": 1
},
{
"attributeMarker": "num",
"conditionMarker": "lth",
"conditionValue": 3
}
]
Schema: (body)
attributeMarker: string
text identifier attribute
example: priceconditionMarker: string
text identifier condition, possible values: 'in' - contains, 'nin' - does not contain, 'eq' - equal, 'neq' - not equal, 'mth' - more than, 'lth' - less than, 'exs' - exists, 'nexs' - does not exist, 'pat' - pattern, for example -, where '' any character, 'same' - same value as the selected attribute*
example: in
Enum:
[ in, nin, eq, neq, mth, lth, exs, nexs, pat, same ]conditionValue: number
condition value
example: 1
Getting all objects of user-admins
const value = await Admins.getAdminsInfo();
Schema
body: array
array of filter objects FilterAdminsDto with search conditions
example: []langCode: string
language code
example: en_USoffset: number
parameter for pagination, default 0
example: 0limit number
parameter for pagination, default 30
example: 30
Example return:
[
{
"id": 1764,
"identifier": "admin1",
"attributeSetId": 7,
"isSync": false,
"attributeValues": {
"marker": {
"value": "",
"type": "string"
}
},
"position": 192
}
]
Schema
id: number
object identifier
example: 1764identifier: string
textual identifier for the record field
example: admin1
default: admin1attributeSetId: number
Attribute set identifier
example: 7isSync boolean
Page indexing flag (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of user attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }position: number
Position number (for sorting)
example: 192
Get admins with lang en_US
const result = await Admins.getAdminsInfo([], 'en_US', 0, 30);
Get admins with lang ru_RU
const result = await Admins.getAdminsInfo([], 'ru_RU', 0, 30);
Get admins with filter by attributeMarker
const body = [
{
attributeMarker: 'string_id1',
conditionMarker: 'in',
conditionValue: 1,
},
]
const result = await Admins.getAdminsInfo(body, 'en_US', 0, 30);
Attributes are an entity that allows you to configure the structure of your application. With them, you can achieve maximum flexibility and eliminate hardcoding.
Attribute sets are similar to a collection of properties, the values of which you can manage through the admin panel. They differ by the type of binding to various entities. You can read more about the types in the section "Types".
An attribute set forms the data structure that can then be used within your project. For example, for a product, you can define that its structure should contain a name, image, price, and text description. At the same time, the structure of a blog post may contain text with a title and a set of images.
Some attributes may be similar in structure and used in different entities, so you can reuse attribute sets for different entities.
Structure Each attribute set contains a customizable collection of attributes that store the content of your project. You can manage these attributes from the management system. This is convenient, as you no longer need to edit the application code to change your project. Just move all content components that can be changed into attributes.
Markers Markers cannot contain spaces and cannot start with a digit. If you attempt to enter invalid values in the marker field, the system will ignore the input.
Name To make it easier for you to navigate, each attribute has a required name parameter, which is a non-unique string. Name the attributes in a way that makes it easy for you to work with them.
Marker As an identifier, each attribute has a required parameter - "marker", which is a unique string. The marker is also used as a key to access the attributes in your project code. Keep this in mind when setting the attribute marker to avoid syntax conflicts.
Data Type Choose the data type for each attribute field that it will store.
Data types can be of the following types:
String: Simple text, for example, "Hello, world!". Text: Longer text, often formatted, for example, an article or a letter. Text with Header: Text with a header that can be used to denote a topic or category. Integer: An integer, for example, 5, 100, -2. Float: A data type for floating-point numbers that can have a decimal part, for example, 3.14, 1.5, -0.25. Real: The same as Float, but with higher precision. Date and Time: A combination of date and time, for example, 2023-10-27 10:00:00. Date: A date, for example, 2023-10-27. Time: A time, for example, 10:00:00. File: Any file on your computer, for example, a document, image, music. Image: An image, for example, a photograph, drawing. Group of Images: A collection of images, for example, a photo album. Radio Button: A selection button from which only one option can be chosen. List: A list of items, for example, a shopping list. Entity: An entity representing an object from the real world, for example, a person, place, organization. The content filling interface will correspond to the selected data type for each attribute field.
Opportunities You can add validators for attributes. This mechanism is discussed in more detail in the section "Validators".
Attributes If one attribute set is used by several entities at once, you need to be careful when changing the attributes of that set. For example, if you delete one of the attributes, that attribute will be removed everywhere it is used along with the content. If you add a new attribute to the set, that attribute will also be added everywhere the attribute set is used.
const { AttributesSets } = defineOneEntry('your-url');
Getting all attribute set objects
const value = await AttributesSets.getAttributes()
Schema
langCode: string
language code
example: en_USoffset: number
parameter offset of record selection, default - 0
example: 0limit: number
parameter limiting the selection of records, default - 30
example: 30typeId: any
identifier of the attribute set type
example: nullsortBy: string
sorting key
example: id
This method return all attribute sets objects and total.
Example return:
{
"total": 100,
"items": [
{
"id": 1764,
"updatedDate": "2025-01-31T21:53:39.276Z",
"version": 10,
"identifier": "my_id",
"title": "Set for pages",
"schema": {
"attribute1": {
"id": 1,
"type": "string",
"isPrice": false,
"original": true,
"identifier": "string",
"localizeInfos": {
"en_US": {
"title": "String"
}
}
}
},
"isVisible": true,
"type": {
"id": 5,
"type": "forProducts"
},
"position": 1
}
]
}
Schema
total: number
Total number of found records
example: 100items: ContentPositionAttributesSetDto
ContentPositionAttributesSetDtoid: number
Object identifier
example: 1764updatedDate: string($date-time)
Object modification date
example: ''version: number
Object modification version number
example: 10identifier: string
Text identifier for record field
example: 'my-id'title: string
Attribute set name
example: Set for pagesschema: Record<string, string>
Schema JSON description (attributes used by the set) of the attribute set
example: OrderedMap { "attribute1": OrderedMap { "id": 1, "type": "string", "isPrice": false, "original": true, "identifier": "string", "localizeInfos": OrderedMap { "en_US": OrderedMap { "title": "String" } } } }title: string
Attribute set name
example: Set for pagesisVisible: boolean
Visibility flag of the settype: object
Object of set typeposition: number
Position number
example: 1
Getting all attributes with data from the attribute set
const value = await AttributesSets.getAttributesByMarker('my-marker')
Schema
marker:* string
text identifier (marker) of the attribute set
example: 'form'langCode: string
language code
example: en_US
Example return:
[
{
"type": "list",
"marker": "list1",
"position": 192,
"validators": {
"requiredValidator": {
"strict": true
},
"defaultValueValidator": {
"fieldDefaultValue": 11
}
},
"localizeInfos": {
"title": "My attribute"
},
"listTitles": [
{
"title": "red",
"value": 1,
"position": 1,
"extended": {
"value": null,
"type": null
}
},
{
"title": "yellow",
"value": 2,
"position": 2,
"extended": {
"value": null,
"type": null
}
}
],
"settings": {},
"additionalFields": [
{
"type": "string",
"value": "Your Name",
"marker": "placeholder"
}
]
}
]
Schema
type: string
attribute type
example: listmarker: string
textual identifier of the attribute (marker)
Enum: [ string, text, textWithHeader, integer, real, float, dateTime, date, time, file, image, groupOfImages, radioButton, list, button ]
example: list1position: number
position number for sorting
example: 192validators: Record<string, any>
set of validators for validation
example: OrderedMap { "requiredValidator": OrderedMap { "strict": true }, "defaultValueValidator": OrderedMap { "fieldDefaultValue": 11 } }localizeInfos: Record<string, any>
localization data for the set (name)
example: OrderedMap { "title": "My attribute" }listTitles Record<string, any>
array of values (with extended data) for attributes of type list and radioButton
example: List [ OrderedMap { "title": "red", "value": 1, "position": 1, "extended": OrderedMap { "value": null, "type": null } }, OrderedMap { "title": "yellow", "value": 2, "position": 2, "extended": OrderedMap { "value": null, "type": null } } ]settings: Record<string, any>
additional attribute settings (optional)
example: OrderedMap {}additionalFields: Record<string, AttributeInSetDto>
example: List [ OrderedMap { "type": "string", "value": "Your Name", "marker": "placeholder" } ]
example: OrderedMap {}
Getting one attribute with data from the attribute set
const value = await AttributesSets.getSingleAttributeByMarkerSet('list1', 'list1')
Schema
setMarker:* number
text identifier (marker) of the attribute set
example: 'form'attributeMarker:* string
text identifier (marker) of the attribute in the set
example: 'list1'langCode: string
language code
example: en_US
Example return:
{
"type": "list",
"marker": "list1",
"position": 192,
"validators": {
"requiredValidator": {
"strict": true
},
"defaultValueValidator": {
"fieldDefaultValue": 11
}
},
"localizeInfos": {
"title": "My attribute"
},
"listTitles": [
{
"title": "red",
"value": 1,
"position": 1,
"extended": {
"value": null,
"type": null
}
},
{
"title": "yellow",
"value": 2,
"position": 2,
"extended": {
"value": null,
"type": null
}
}
]
}
Schema
type: string
attribute type
example: listmarker: string
textual identifier of the attribute (marker)
example: list1position: number
position number for sorting
example: 192validators: Record<string, any>
set of validators for validation
example: OrderedMap { "requiredValidator": OrderedMap { "strict": true }, "defaultValueValidator": OrderedMap { "fieldDefaultValue": 11 } }localizeInfos: Record<string, any>
localization data for the set (name)
example: OrderedMap { "title": "My attribute" }listTitles Record<string, any>
array of values (with extended data) for list and radioButton attributes
example: List [ OrderedMap { "title": "red", "value": 1, "position": 1, "extendedValue": null, "extendedValueType": null }, OrderedMap { "title": "yellow", "value": 2, "position": 2, "extendedValue": null, "extendedValueType": null } ]
Getting a single object of attributes set by marker
const value = await AttributesSets.getAttributeSetByMarker('my-marker')
Schema
marker:* string
text identifier (marker) of the attribute set
example: 'form'langCode: string
language code
example: en_US
Example return:
{
"id": 1764,
"updatedDate": "2025-01-31T22:25:11.952Z",
"version": 10,
"identifier": "my-id",
"title": "Set for pages",
"schema": {
"attribute1": {
"id": 1,
"type": "string",
"isPrice": false,
"original": true,
"identifier": "string",
"localizeInfos": {
"en_US": {
"title": "String"
}
}
}
},
"isVisible": true,
"type": {
"id": 5,
"type": "forProducts"
},
"position": 1
}
Schema
id: number
Object identifier
example: 1764updatedDate: string($date-time)
Object modification date
example:version: number
Object modification version number
example: 10identifier:* string
Text identifier for record field
example: 'my-id'title:* string
Attribute set name
example: 'Set for pages'schema:* Record<string, string>
Schema JSON description (attributes used by the set) of the attribute set
example: OrderedMap { "attribute1": OrderedMap { "id": 1, "type": "string", "isPrice": false, "original": true, "identifier": "string", "localizeInfos": OrderedMap { "en_US": OrderedMap { "title": "String" } } } }isVisible:* boolean
Visibility flag of the settype:* object
Object of set typeposition:* number
Position number
example: 1
The User Auth Provider in OneEntry Headless CMS facilitates user authentication and registration through various methods, such as email. By using the AuthProvider object, developers can handle user registration, including sending activation codes when necessary. The system supports diverse data types for form attributes, allowing comprehensive user data management. Key functionalities include user authentication, token management (access and refresh tokens), password changes, and logout. Additionally, it provides methods to manage authentication providers and retrieve specific details by marker or language code, ensuring secure and efficient user management across applications.
const { AuthProvider } = defineOneEntry('your-url');
User registration (❗️For provider with user activation, activation code is sent through corresponding user notification method)
Schema
marker:* string
The text identifier of the authorization provider
example: emailbody:* ISignUpData
Request body
example: { "formIdentifier": "reg", "authData": [ { "marker": "login", "value": "example@oneentry.cloud" }, { "marker": "password", "value": "12345" } ], "formData": [ { "marker": "last_name", "type": "string", "value": "Name" } ], "notificationData": { "email": "example@oneentry.cloud", "phonePush": ["+99999999999"], "phoneSMS": "+99999999999" } }langCode: string
Language code
example: en_US
Method accept the body as a parameter.
Examples for body parameter with different types data:
Example with attributes of simple types formData "string", "integer", "float".
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "last_name",
"type": "string",
"value": "Fyodor Ivanov"
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attributes of types "date", "dateTime", "time"
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "birthday",
"type": "date",
"value": {
"fullDate": "2024-05-07T21:02:00.000Z",
"formattedValue": "08-05-2024 00:02",
"formatString": "DD-MM-YYYY HH:mm"
}
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attribute of type "text"
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "about",
"type": "text",
"value": {
"htmlValue": "<p>This is me</p>",
"plainValue": "",
"params": {
"isEditorDisabled": false,
"isImageCompressed": true
}
}
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attribute type "textWithHeader"
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "about",
"type": "textWithHeader",
"value": {
"header": "Header",
"htmlValue": "<p>This is me</p>",
"plainValue": "",
"params": {
"isEditorDisabled": false,
"isImageCompressed": true
}
}
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attributes type "image" and "groupOfImages"
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "avatar",
"type": "image",
"value": [
{
"filename": "files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"downloadLink": "http://my-site.zone/cloud-static/files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"size": 392585,
"previewLink": "",
"params": {
"isImageCompressed": true
}
}
]
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attribute type "file"
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "picture",
"type": "file",
"value": [
{
"filename": "files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"downloadLink": "http://my-site.zone/cloud-static/files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"size": 392585
}
]
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attributes type "radioButton" and "list"
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "selector",
"type": "list",
"value": [
{
"title": "red",
"value": "1",
"extended": {
"value": "red",
"type": "string"
}
}
]
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with attribute type "entity" (nested list)
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "entity-selector",
"type": "entity",
"value": [
{
"id": "1",
"title": "red",
"value": "1",
"parentId": "null"
}
]
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [],
"phoneSMS": "+79991234567"
}
}
Example with one push identifier
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "selector",
"type": "list",
"value": [
{
"title": "red",
"value": "1",
"extended": {
"value": "red",
"type": "string"
}
}
]
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [
"7DD987F846400079F4B03C058365A4869047B4A0."
],
"phoneSMS": "+79991234567"
}
}
Example with multiple push identifiers
{
"formIdentifier": "reg",
"langCode": "en_US",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": {
"en_US": [
{
"marker": "selector",
"type": "list",
"value": [
{
"title": "red",
"value": "1",
"extended": {
"value": "red",
"type": "string"
}
}
]
}
]
},
"notificationData": {
"email": "test@test.zone",
"phonePush": [
"7DD987F846400079F4B03C058365A4869047B4A0",
"7DD987F846400079F4B03C058365A4869047B4A0",
"7DD987F846400079F4B03C058365A4869047B4A0."
],
"phoneSMS": "+79991234567"
}
}
const body = {
"formIdentifier": "reg",
"authData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "password",
"value": "12345"
}
],
"formData": [
{
"marker": "last_name",
"type": "string",
"value": "Username"
}
],
"notificationData": {
"email": "test@test.com",
"phonePush": [],
"phoneSMS": "+99999999999"
}
}
const value = await AuthProvider.signUp('email', body)
Schema
formIdentifier: string
textual identifier of the authorization provider's form example: reg_formformData:
form data attached to the authorization providerauthData:
authorization data taken from the form attached to the authorization provider
example: List [ OrderedMap { "marker": "login", "value": "test" }, OrderedMap { "marker": "password", "value": "12345" } ]notificationData:
user notification dataattributeSetId: number
identifier for the used attribute set
example: 7formData: FormDataLangType
Data submitted by the form
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "value": "Name" } ] }notificationData: UserNotificationDataType
data for notifying the user
example: OrderedMap { "email": "test@test.zone", "phonePush": "", "phoneSMS": "+79991234567" }systemCode: string
system code for performing official actions (password reset, activation)
example: OrderedMap { "value": "90BDCX", "expiredDate": "2024-05-07T21:02:00.000Z" }formIdentifier: string
the text identifier of the authorization provider's form
example: reg_formauthData: FormAuthDataType
authorization data taken from the form linked to the authorization provider
example: List [ OrderedMap { "marker": "login", "value": "test" }, OrderedMap { "marker": "password", "value": "12345" } ]authProviderId: number
ID of the authorization provider
example: 1
This method will register a new user. Returns the registered user's object.
Example return:
{
"id": 1764,
"updatedDate": "2024-05-23T12:43:00.169Z",
"version": 10,
"identifier": "catalog",
"isActive": false,
"authProviderId": 1,
"formData": [
{
"marker": "login",
"value": "test"
},
{
"marker": "f-name",
"value": "Second name"
}
],
"notificationData": {
"email": "test@test.com",
"phonePush": ["+999999999"],
"phoneSMS": "+9999999999"
},
"systemCode": {
"value": "90BDCX",
"expiredDate": "2024-05-07T21:02:00.000Z"
}
}
Schema
id: number
object identifier example: 1764updatedDate: string
object modification dateversion: number
object version number
example: 10identifier: string
textual identifier for the field record
example: catalog
default: markerattributeSetId: number
identifier for the used attribute set
example: 7formData: FormDataLangType
Data submitted by the form
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "value": "Name" } ] }notificationData: UserNotificationDataType
data for notifying the user
example: OrderedMap { "email": "test@test.zone", "phonePush": "", "phoneSMS": "+79991234567" }systemCode: string
system code for performing official actions (password reset, activation)
example: OrderedMap { "value": "90BDCX", "expiredDate": "2024-05-07T21:02:00.000Z" }formIdentifier: string
the text identifier of the authorization provider's form
example: reg_formauthData: FormAuthDataType
authorization data taken from the form linked to the authorization provider
example: List [ OrderedMap { "marker": "login", "value": "test" }, OrderedMap { "marker": "password", "value": "12345" } ]authProviderId: number
ID of the authorization provider
example: 1
Getting the code to activate the user
const value = await AuthProvider.generateCode('email', 'example@oneentry.cloud', 'auth')
Schema
marker:* array
The text identifier of the authorization provider
example: emailuserIdentifier:* string
The text identifier of the user's object (user login)
example: example@oneentry.cloudeventIdentifier:* string
Text identifier of the event object for which the code is generated
example: auth
This method receives a code to activate the user. Code is returned through the corresponding user notification method
Checking the user activation code
const value = await AuthProvider.checkCode('email', 'example@oneentry.cloud', 'auth', 'WTGC9E')
Schema
marker:* string
The text identifier of the authorization provider.
example: emailuserIdentifier:* string
The text identifier of the user's object (user login)
example: example@oneentry.cloudeventIdentifier:* string
Text identifier of the event object for which the code is generated
example: authcode:* string
Service code
example: WTGC9E
This method checks the user's code. Returns true (if the code is correct) or false (if it is incorrect).
Example return:
true
User activation
const value = await AuthProvider.activateUser('email', 'example@oneentry.cloud', 'WTGC9E')
Schema
marker:* string
Textual identifier of the authentication provider
example: emailuserIdentifier:* string
The text identifier of the user's object (user login)
example: example@oneentry.cloudcode:* string
Service code
example: WTGC9E
This method activates the user by code. If successful, it will return true.
Example return:
true
User authentication
const data = {
authData: [
{
marker: "login",
value: "example@oneentry.cloud"
},
{
marker: "password",
value: "12345"
}
]
}
const value = await AuthProvider.auth('email', data)
Schema
marker: string
The text identifier of the authorization provider
example: emaildata: IAuthPostBody
Array of objects contains auth information
example: { authData: [{marker: "login",value: "test"},{marker: "password",value: "12345"}]}
Schema
authData: string
Authorization data taken from the form attached to the authorization provider example: List [ OrderedMap { "marker": "login", "value": "test" }, OrderedMap { "marker": "password", "value": "12345" } ]
This method performs user authorization. Returns an object with a set of tokens.
Example return:
{
"userIdentifier": "example@oneentry.cloud",
"authProviderIdentifier": "email",
"accessToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MywiYXV0aFByb3ZpZGVySWRlbnRpZmllciI6ImVtYWlsIiwidXNlcklkZW50aWZpZXIiOiJ0ZXN0QHRlc3QucnUiLCJ1c2VyQWdlbnQiOiJQb3N0bWFuUnVudGltZS83LjM3LjMiLCJpYXQiOjE3MTQ1NTc2NzAsImV4cCI6MTcxNDU2MTI3MH0.vm74Ha-S37462CAF3QiDpO9b0OhlJFNDMKq4eEyoaB8",
"refreshToken": "1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9"
}
Schema
userIdentifier: string
user identifier example: example@oneentry.cloudauthProviderIdentifier: string
auth provider identifier
example: emailaccessToken: string
access token
example:eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MywiYXV0aFByb3ZpZGVySWRlbnRpZmllciI6ImVtYWlsIiwidXNlcklkZW50aWZpZXIiOiJ0ZXN0QHRlc3QucnUiLCJ1c2VyQWdlbnQiOiJQb3N0bWFuUnVudGltZS83LjM3LjMiLCJpYXQiOjE3MTQ1NTc2NzAsImV4cCI6MTcxNDU2MTI3MH0.vm74Ha-S37462CAF3QiDpO9b0OhlJFNDMKq4eEyoaB8refreshToken: string
refresh token
example: 1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9
Update user tokens
const value = await AuthProvider.refresh('email', '1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9')
Schema
marker:* string
The text identifier of the authorization provider. Example - email
example: emailtoken:* string
Refresh token
example: 1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9
This method updates the user's token. Returns an object with a set of tokens.
Example return:
{
"userIdentifier": "example@oneentry.cloud",
"authProviderIdentifier": "email",
"accessToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MywiYXV0aFByb3ZpZGVySWRlbnRpZmllciI6ImVtYWlsIiwidXNlcklkZW50aWZpZXIiOiJ0ZXN0QHRlc3QucnUiLCJ1c2VyQWdlbnQiOiJQb3N0bWFuUnVudGltZS83LjM3LjMiLCJpYXQiOjE3MTQ1NTc2NzAsImV4cCI6MTcxNDU2MTI3MH0.vm74Ha-S37462CAF3QiDpO9b0OhlJFNDMKq4eEyoaB8",
"refreshToken": "1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9"
}
Schema
userIdentifier: string
user identifier example: example@oneentry.cloudauthProviderIdentifier: string
auth provider identifier
example: emailaccessToken: string
access token
example:eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MywiYXV0aFByb3ZpZGVySWRlbnRpZmllciI6ImVtYWlsIiwidXNlcklkZW50aWZpZXIiOiJ0ZXN0QHRlc3QucnUiLCJ1c2VyQWdlbnQiOiJQb3N0bWFuUnVudGltZS83LjM3LjMiLCJpYXQiOjE3MTQ1NTc2NzAsImV4cCI6MTcxNDU2MTI3MH0.vm74Ha-S37462CAF3QiDpO9b0OhlJFNDMKq4eEyoaB8refreshToken: string
refresh token
example: 1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9
User account logout 🔐 This method requires authorization.
const value = await AuthProvider.logout('email', '1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9')
Schema
marker:* string
The text identifier of the authorization provider
example: emailtoken:* string
Refresh token
example: 1714557670334-cb85112d-618d-4b2a-bad5-137b19c135b9
This method performs a user logout. If successful, it will return true. This method requires user authorization.
Example return:
true
User password change (only for activated account tariffs with Activation feature enabled)
const value = await AuthProvider.changePassword('email', 'example@oneentry.cloud', 1, 'EW32RF', 654321, 654321)
Schema
marker:* string
The text identifier of the authorization provider.
example: emailuserIdentifier:* string
The text identifier of the user's object (user login)
example: example@oneentry.cloudtype:* string
Operation type (1 - for changing password, 2 - for recovery)
example: 1code:* string
Service code
example: EW32RFnewPassword:* string
New password
example: 654321repeatPassword: string
Optional variable contains repeat new password for validation
example: 654321
This method changes the password of an authorized user. If successful, it will return true.
Example return:
true
Get all auth providers objects
const value = await AuthProvider.getAuthProviders()
Schema
langCode: string
language code
example: en_USoffset: number
parameter for pagination, default 0
example: 0limit: number
parameter for pagination, default 30
example: 30
This method gets all the objects of the authorization providers.
Example return:
[
{
"id": 1,
"localizeInfos": {
"title": "email"
},
"config": {
"deleteNoneActiveUsersAfterDays": 2,
"systemCodeTlsSec": 120,
"systemCodeLength": 8,
"systemCodeOnlyNumbers": null
},
"version": 0,
"identifier": "email",
"type": "email",
"formIdentifier": "reg",
"isActive": true,
"isCheckCode": false
}
]
Schema
id: number
object identifier example: 1764localizeInfos: CommonLocalizeInfos
block name with localization
example: OrderedMap { "en_US": OrderedMap { "title": "My block" } }version: number
object version number
example: 10identifier: string
textual identifier for the field record
example: catalog
default: markerisActive: boolean
Flag of usage
example: falseisCheckCode: boolean
a sign of user activation via a code
example: falsetype: string
type of providere
example: emailformIdentifier: string
the marker of the form used by the provider (may be null)
example: email
Get one auth provider object by marker
const value = await AuthProvider.getMarker('email')
Schema
marker:* string
The text identifier of the authorization provider
example: emaillangCode: string
language code
example: en_US
Example return:
[
{
"id": 1,
"localizeInfos": {
"title": "email"
},
"version": 0,
"identifier": "email",
"type": "email",
"formIdentifier": "reg",
"isActive": true,
"isCheckCode": false,
"config": {
"deleteNoneActiveUsersAfterDays": 2,
"systemCodeLength": 8,
"systemCodeOnlyNumbers": null,
"systemCodeTlsSec": 120
}
}
]
Schema
id: number
object identifier example: 1764localizeInfos: CommonLocalizeInfos
block name with localization
example: OrderedMap { "en_US": OrderedMap { "title": "My block" } }version: number
object version number
example: 10identifier: string
textual identifier for the field record
example: catalog
default: markerisActive: boolean
Flag of usage
example: falseisCheckCode: boolean
a sign of user activation via a code
example: falsetype: string
type of providere
example: emailformIdentifier: string
the marker of the form used by the provider (may be null)
example: email
The "Blocks" module is designed to create structures that contain sets of attributes. The Headless CMS OneEntry provides users with very flexible tools, and you can use the "Blocks" module as you see fit for your specific case. The main concept of the "Blocks" module is to create reusable entities (blocks) that contain sets of attributes and additional content.
For example, you can create a block - "footer" and use it on all pages of your project.
const { Blocks } = defineOneEntry('your-url');
Getting all block objects
const value = await Blocks.getBlocks('forTextBlock')
Schema
type:* BlockType
Available values: forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, forOrder, service
example: forTextBlocklangCode: string
Language code
example: en_USoffset: number
Parameter for pagination. Default 0
example: 0limit: number
Parameter for pagination. Default 30
example: 30
This method return array of all blocks object and total.
Example return:
{
"total": 100,
"items": [
{
"id": 1,
"localizeInfos": {
"title": "Block"
},
"version": 0,
"position": 1,
"identifier": "block",
"type": "forTextBlock",
"templateIdentifier": null,
"isVisible": true,
"attributeValues": {}
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier example: 1764attributeSetId: number
identifier for the used attribute set
example: 7localizeInfos: CommonLocalizeInfos
block name with localization
example: OrderedMap { "en_US": OrderedMap { "title": "My block" } }customSettings: BlockCustomSettings
custom settings for different block types
example: OrderedMap { "sliderDelay": 0, "sliderDelayType": "", "productQuantity": 4, "productSortType": "By_ID", "productSortOrder": "Descending", "productCountElementsPerRow": 10, "similarProductRules": List [ OrderedMap { "property": "Descending", "includes": "", "keywords": "", "strict": "" } ] }version: number
object version number
example: 10identifier: string
textual identifier for the field record
example: catalog
default: markerposition: number
position number (for sorting)
example: 192attributeValues: Record<string, string>
array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }type: string
block type
example: forNewsPagetemplateIdentifier: string
template marker used by the block (can be null)
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]
example: null
Getting a single block object by marker
const value = await Blocks.getBlockByMarker('my-marker')
Schema
marker:* string
Marker of Block
example: my-markerlangCode: string
Language code
example: en_USoffset: number
Parameter for pagination. Default 0
example: 0limit: number
Parameter for pagination. Default 30
example: 30
This method return one blocks object by marker.
Example return:
{
"id": 1764,
"localizeInfos": {
"en_US": {
"title": "My block"
}
},
"customSettings": {
"sliderDelay": 0,
"sliderDelayType": "",
"productConfig": {
"quantity": 2,
"sortType": "By_ID",
"sortOrder": "DESC",
"countElementsPerRow": 10
},
"similarProductRules": [
{
"property": "Descending",
"includes": "",
"keywords": "",
"strict": ""
}
],
"condition": {
"name": "title"
}
},
"version": 10,
"identifier": "catalog",
"position": 192,
"productPageUrls": [
"23-laminat-floorwood-maxima"
],
"isVisible": true,
"attributeValues": {
"en_US": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
}
},
"type": "forNewsPage",
"templateIdentifier": null,
"attributeSetIdentifier": "my-attributes-sets"
}
Schema
id: number
object identifier
example: 1764localizeInfos: CommonLocalizeInfos
block name considering localization
example: OrderedMap { "en_US": OrderedMap { "title": "My block" } }customSettings: BlockCustomSettings
individual settings for different types of blocks
BlockCustomSettings example: OrderedMap { "sliderDelay": 0, "sliderDelayType": "", "productConfig": OrderedMap { "quantity": 2, "sortType": "By_ID", "sortOrder": "DESC", "countElementsPerRow": 10 }, "similarProductRules": List [ OrderedMap { "property": "Descending", "includes": "", "keywords": "", "strict": "" } ], "condition": OrderedMap { "name": "title" } }version: number
version number of the object change
example: 10identifier: string
textual identifier for the recording field
example: catalog
default: markerposition: number
position number (for sorting)
example: 192productPageUrls: any
array of unique parts of the URL page (after the last "/") - categories from where products can be taken (optional)
example: List [ "23-laminat-floorwood-maxima" ]isVisible: boolean
visibility (availability) indicator of the block
example: trueattributeValues: Record<string, string>
Array of attribute values from the index (type, value, array of additional fields for the attribute)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string", "position": 1, "isProductPreview": false, "isIcon": false, "attributeFields": OrderedMap { "marker": OrderedMap { "type": "string", "value": "test" } } } } }type: string
block type
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, forOrder, service ]attributeSetId: number
identifier for the used attribute set
example: 7position: number
position number (for sorting)
example: 192templateIdentifier: string
Template marker used by the block (can be null)
example: null
Speedy search for limited display of block objects
const value = await Blocks.searchBlock('my-marker')
Schema
marker:* string
Block identifier
example: my-markerlangCode: string
Language code
example: en_US
Quick search for block objects with limited output.
Example return:
[
{
"id": 1,
"name": "my block",
"identifier": "my-block"
}
]
The "Events" module allows you to create and edit events, through which you can interact with the users of your system. In this module, you select an event that the system will respond to and send notifications to the users of your system. For example, you have statuses for catalog items "In stock" and "Out of stock," and you want to send users a notification about the status change from "Out of stock" to "In stock"
const { Events } = defineOneEntry('your-url');
In the Headless CMS OneEntry, there are three ways to interact with the users of your system through the "Events" module:
Push Notification
Email
Socket IO
Returns all subscriptions to products 🔐 This method requires authorization.
const value = await Events.getAllSubscriptions()
Schema
offset: number
Pagination parameter, default is 0
example: 0limit: number
Pagination parameter, default is 30
example: 30
This method return all subscriptions to product.
Example return:
{
"total": 100,
"items": [
{
"eventMarker": "string",
"productId": 0
}
]
}
Schema
total: number
Total number of records foundeventMarker: string
Event markerproductId number
Product identifier
Subscribe to an event on a product 🔐 This method requires authorization.
const value = await Events.subscribeByMarker('test_event', 1, 1)
Schema
marker:* string
Event marker
example: test_eventproductId:* string
Product id
example: 14langCode: string
Language code
example: en_USthreshold: number
Threshold value for comparing numerical value
example: 0
This method subscribes to the product event. Returns nothing if the subscription was successful. This method requires user authorization.
Unsubscribe from events on a product 🔐 This method requires authorization.
const value = await Events.unsubscribeByMarker('test_event', 1, 1)
Schema
marker:* string
Event marker
example: test_eventproductId:* string
Product id
example: 14langCode: string
Language code
example: en_USthreshold: number
Threshold value for comparing numerical value
example: 0
This method unsubscribes to the product event. Returns nothing if the unsubscription was successful.
FileUploading in OneEntry Headless CMS provides methods for managing files in cloud storage. The upload method allows uploading files with optional parameters such as type, entity, ID, and image optimization options. It returns information about the uploaded file, including its name and download link. The delete method removes a file from storage by its name and specified parameters. The getFile method searches for and returns a file object based on provided parameters such as ID, type, entity, and filename.
const { FileUploading } = defineOneEntry('your-url');
File upload
const query = {
type:"page",
entity:"editor",
id:3787,
width:0,
height:0,
compress:true,
}
const value = await FileUploading.upload(data, query)
Schema
data:* File
File objects. Get data as File from your unput as e.target.files[0]
example:fileQuery: IUploadingQuery
Optional set query parameters.
example:fileQuery.type: string
Type, determines the folder name in the storage
example: pagefileQuery.entity: string
Entity name from which the file is uploaded, determines the folder name in the storage
example: editorfileQuery.id number
Identifier of the object from which the file is uploaded, determines the folder name in the storage
example: 3787fileQuery.width number
Optional width parameter.
example: 0fileQuery.height number
Optional height parameter
example: 0fileQuery.compress boolean
Optional flag of optimization (compression) for images
example: truefileQuery.template string
preview template identifier
example: 1
This method uploads a file to a cloud file storage. Pass to the date the value obtained from input type "file".
Data is file object (or array), learn more - File Object
Example return:
[
{
"filename": "string",
"downloadLink": "string",
"size": 0
}
]
Schema
filename: string
filename with relative pathdownloadLink: string
link for downloading the filesize number
size of the file in bytes
File deletion
const query = {
type:"page",
entity:"editor",
id:3787
}
const value = await FileUploading.delete("file.png", query)
Schema
filename: string
File name.
example: file.pngfileQuery: IUploadingQuery
Optional set query parameters
example:fileQuery.type: string
Type, determines the folder name in the storage
example: pagefileQuery.entity: string
Entity name from which the file is uploaded, determines the folder name in the storage
example: editorfileQuery.id number
Identifier of the object from which the file is uploaded, determines the folder name in the storage
example: 3787fileQuery.template string
preview template identifier
example: 1
This void method delete a file from the cloud file storage.
File search
const value = await FileUploading.getFile(123, 'page', 'editor', 'file.png')
Schema
id: number
Object identifier, from which the file is uploaded, determines the folder name in the storage
example: 123type: string
Type, determines the folder name in the storage
example: pageentity: string
entity name, from which the file is uploaded, determines the folder name in the storage
example: editorfilename: string
Filename
example: file.pngtemplate: string
preview template identifier
example: 1
This method return file object by parameters.
Example return:
{
"file": "string"
}
The "Forms" module is a user-friendly tool for creating and managing various forms, such as contact forms, surveys, and registration sheets. With an intuitive interface, users can add form elements, customize layouts, and apply themes. The module supports organizing and categorizing forms, setting access permissions, and tracking responses in real-time. Automation features include notifications upon form submissions and integration with applications.
const { Forms } = defineOneEntry('your-url');
Get all form objects
const value = await Forms.getAllForms()
Schema
langCode string
Language code. Default "en_US"
example: en_USoffset number
Parameter for pagination. Default 0
example: 0limit number
Parameter for pagination. Default 30
example: 30
This method retrieves all form objects from the API. It returns a Promise that resolves to an array of FormEntity objects.
Example return:
[
{
"id": 1764,
"attributeSetId": 0,
"processingType": "email",
"localizeInfos": {
"title": "My Form",
"titleForSite": "",
"successMessage": "",
"unsuccessMessage": "",
"urlAddress": "",
"database": "0",
"script": "0"
},
"processingData": "Unknown Type: ProcessingData",
"version": 10,
"type": "data",
"identifier": "catalog",
"position": 192,
"attributes": [
{
"type": "list",
"marker": "l1",
"position": 2,
"settings": {},
"listTitles": [
{
"title": "red",
"value": 1,
"position": 1,
"extendedValue": null,
"extendedValueType": null
},
{
"title": "yellow",
"value": 2,
"position": 2,
"extendedValue": null,
"extendedValueType": null
}
],
"validators": {},
"localizeInfos": {
"title": "l1"
}
}
]
}
]
Schema
id: number
object identifier
example: 1764attributeSetId: number
identifier of the attribute set usedprocessingType: string
form processing type
example: emaillocalizeInfos: FormLocalizeInfos
form name with localization
Enum: [ db, email, script ] example: OrderedMap { "en_US": OrderedMap { "title": "My Form", "titleForSite": "", "successMessage": "", "unsuccessMessage": "", "urlAddress": "", "database": "0", "script": "0" } }processingData: ProcessingData
form dataversion: number
object version number
example: 10identifier: string
textual identifier for the record field
example: catalog
default: markerposition: number
position number (for sorting)
example: 192position: string
Form type
example: 'data'attributes:
array of attribute values from the used attribute set for displaying the form (taking into account the specified language)
example: List [ OrderedMap { "type": "list", "marker": "l1", "position": 2, "listTitles": List [ OrderedMap { "title": "red", "value": 1, "position": 1, "extendedValue": null, "extendedValueType": null }, OrderedMap { "title": "yellow", "value": 2, "position": 2, "extendedValue": null, "extendedValueType": null } ], "validators": OrderedMap {}, "localizeInfos": OrderedMap { "title": "l1" } } ]
Getting one form object by marker
const value = await Forms.getFormByMarker('my-form')
Schema
marker:* string
Marker of form
example: my-formlangCode: string
Language code. Default "en_US"
example: en_US
This method retrieves a single form object based on its textual identifier (marker) from the API. It returns a Promise that resolves to a FormEntity object.
Example return:
{
"id": 1764,
"attributeSetId": 0,
"processingType": "email",
"localizeInfos": {
"title": "My Form",
"titleForSite": "",
"successMessage": "",
"unsuccessMessage": "",
"urlAddress": "",
"database": "0",
"script": "0"
},
"processingData": "Unknown Type: ProcessingData",
"version": 10,
"type": "data",
"identifier": "catalog",
"position": 192,
"attributes": [
{
"type": "list",
"marker": "l1",
"position": 2,
"settings": {},
"listTitles": [
{
"title": "red",
"value": 1,
"position": 1,
"extendedValue": null,
"extendedValueType": null
},
{
"title": "yellow",
"value": 2,
"position": 2,
"extendedValue": null,
"extendedValueType": null
}
],
"validators": {},
"localizeInfos": {
"title": "l1"
}
}
]
}
Schema
id: number
object identifier
example: 1764attributeSetId: number
identifier of the attribute set usedprocessingType: string
form processing type
example: emaillocalizeInfos: FormLocalizeInfos
form name with localization
Enum: [ db, email, script ] example: OrderedMap { "en_US": OrderedMap { "title": "My Form", "titleForSite": "", "successMessage": "", "unsuccessMessage": "", "urlAddress": "", "database": "0", "script": "0" } }processingData: ProcessingData
form dataversion: number
object version number
example: 10identifier: string
textual identifier for the record field
example: catalog
default: markerposition: number
position number (for sorting)
example: 192position: string
Form type
example: 'data'attributes:
array of attribute values from the used attribute set for displaying the form (taking into account the specified language)
example: List [ OrderedMap { "type": "list", "marker": "l1", "position": 2, "listTitles": List [ OrderedMap { "title": "red", "value": 1, "position": 1, "extendedValue": null, "extendedValueType": null }, OrderedMap { "title": "yellow", "value": 2, "position": 2, "extendedValue": null, "extendedValueType": null } ], "validators": OrderedMap {}, "localizeInfos": OrderedMap { "title": "l1" } } ]
The FormData module in OneEntry Headless CMS allows for managing and interacting with form data. By using the defineOneEntry function, you can create a FormData object to handle various operations. The module supports different data types such as strings, numbers, dates, text, images, files, lists, and nested entities. It provides methods like getFormsData, postFormsData, and getFormsDataByMarker for retrieving, creating, and searching form data. Each method interacts with the API to perform these tasks, returning structured JSON objects with detailed information about the form data, including identifiers, timestamps, and language-specific data.
const { FormData } = defineOneEntry('your-url');
Methods with a post request accept as the request body an object with the form data field, which corresponds to the type of information being sent. The following are examples of form data objects for different data types.
Example with a simple type attribute "string", "number", "float"
{
"marker": "last_name",
"type": "string",
"value": "Username"
}
Example with a simple type attribute "date", "dateTime", "time"
{
"marker": "birthday",
"type": "date",
"value": {
"fullDate": "2024-05-07T21:02:00.000Z",
"formattedValue": "08-05-2024 00:02",
"formatString": "DD-MM-YYYY HH:mm"
}
}
Example with a simple type attribute "text"
{
"marker": "about",
"type": "text",
"value": {
"htmlValue": "<p>Hello world</p>",
"plainValue": "",
"params": {
"isEditorDisabled": false,
"isImageCompressed": true
}
}
}
Example with a simple type attribute "textWithHeader"
{
"marker": "about",
"type": "textWithHeader",
"value": {
"header": "Headline",
"htmlValue": "<p>Hello World</p>",
"plainValue": "",
"params": {
"isEditorDisabled": false,
"isImageCompressed": true
}
}
}
Example with a simple type attribute "image" or "groupOfImages"
{
"marker": "avatar",
"type": "image",
"value": [
{
"filename": "files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"downloadLink": "http://my-site.com/cloud-static/files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"size": 392585,
"previewLink": "",
"params": {
"isImageCompressed": true
}
}
]
}
Example with a simple type attribute "files"
{
"marker": "picture",
"type": "file",
"value": [
{
"filename": "files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"downloadLink": "http://my-site.com/cloud-static/files/project/page/10/image/Screenshot-from-2024-05-02-15-23-14.png",
"size": 392585
}
]
}
Example with a simple type attribute "radioButton" or "list"
{
"marker": "selector",
"type": "list",
"value": [
{
"title": "red",
"value": "1",
"extended": {
"value": "red",
"type": "string"
}
}
]
}
Example with attribute type "entity" (nested list)
{
"formIdentifier": "reg",
"formData": {
"en_US": [
{
"marker": "entity-marker",
"type": "entity",
"value": [25, 32, 24]
}
]
}
}
Value - numerical identifiers for pages and string identifiers for products. Identifiers for products should include the prefix 'p-', for example, 'p-1-', 'p-2-', etc. p-[parentId]-[productId]
Example with attribute type "timeInterval"
{
"formIdentifier": "reg",
"formData": {
"en_US": [
{
"marker": "interval",
"type": "timeInterval",
"value": [
[
"2025-02-11T16:00:00:000Z",
"2025-02-13T16:00:00:000Z",
]
]
}
]
}
}
value — array of interval arrays in ISO 8601 format. for example 2025-02-11T16:00:00:000Z
2025 — year; 02 — month; 11 — day of the month; T — separator between date and time; 16:00:00 — time in hours:minutes:seconds format; 000Z — milliseconds and time zone indication. Z means that the time is specified in UTC format.
Searching for all form data
const value = await FormData.getFormsData()
Schema
langCode: string
Language code. Default "en_US"
example:offset: number
Parameter for pagination. Default 0
example: 0limit: number
Parameter for pagination. Default 30
example: 30
This method creates form data objects by sending a request to the API. It accepts an array of objects of type IFormsPost as the request body to provide the necessary form data. It returns a Promise that resolves to the created CreateFormDataDto objects.
Example return:
{
"total": 1,
"items": [
{
"id": 1764,
"formIdentifier": "my-form",
"time": "2023-02-12 10:56",
"formData": {
"marker": "name_1",
"value": "Name",
"type": "string"
},
"attributeSetIdentifier": "test-form"
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764formIdentifier: string
Text identifier of the form object (marker)
example: my-formtime: Date
Date and time of form modification
example: 2023-02-12 10:56formData: FormDataLangType
Data submitted by the form
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "value": "Name" } ] }attributeSetIdentifier: string
text identifier (marker) of the used attribute set
example: test-form
Creating an object of form data saving information
const body = {
"formIdentifier": "reg",
"formData": {
"en_US": [
{
"marker": "last_name",
"type": "string",
"value": "Andrey"
}
]
}
}
const value = await FormData.postFormsData(body)
Schema (body)
formIdentifier: string
Text identifier of the form object (marker)
example: my-formformData: FormDataLangType
Data submitted by the form
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "type": "string", "value": "Name" } ] }
This method Returns a created FormDataEntity object. If you want to change the language, just pass it with the second argument
Example return:
{
"id": 1764,
"formIdentifier": "my-form",
"time": "2023-02-12 10:56",
"formData": {
"marker": "name_1",
"value": "Name",
"type": "string"
}
}
Schema
id: number
object identifier
example: 1764formIdentifier: string
Text identifier of the form object (marker)
example: my-formtime: Date
Date and time of form modification
example: 2023-02-12 10:56formData: FormDataLangType
Data submitted by the form
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "value": "Name" } ] }
Searching for form data by text identifier (marker)
const value = await FormData.getFormsDataByMarker('my-marker')
Schema
marker:* string
Marker of form
example: my-markerlangCode: string
Language code. Default "en_US"
example: en_USoffset: number
Parameter for pagination. Default 0"
example: 0limit: number
Parameter for pagination. Default 30"
example: 30
This method retrieves a specific form data object by its marker from the API. It accepts a marker parameter as the marker of the form data. It returns a Promise that resolves to an array of objects of type FormDataEntity.
Example return:
{
"total": 10,
"items": [
{
"id": 1764,
"formIdentifier": "my-form",
"time": "2023-02-12 10:56",
"formData": {
"marker": "name_1",
"value": "Name",
"type": "string"
},
"attributeSetIdentifier": "test-form"
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764formIdentifier: string
Text identifier of the form object (marker)
example: my-formtime: Date
Date and time of form modification
example: 2023-02-12 10:56formData: FormDataLangType
Data submitted by the form
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "value": "Name" } ] }attributeSetIdentifier: string
text identifier (marker) of the used attribute set
example: test-form
The GeneralTypes
module in OneEntry Headless CMS is designed to manage and retrieve general types used within the system. By using the defineOneEntry
function, you can create a GeneralTypes
object to interact with these types. The primary method available is getAllTypes
, which retrieves all objects of type GeneralTypeEntity
from the API. This method returns a Promise that resolves to an array of GeneralTypeEntity
objects, each containing an id
and a type
. The types cover various purposes, such as page layouts, forms, product blocks, and more, providing flexibility for different content management needs.
const { GeneralTypes } = defineOneEntry('your-url');
Getting all types
const value = await GeneralTypes.getAllTypes()
This method retrieves all objects of type GeneralTypeEntity from the API. It returns a Promise that resolves to an array of GeneralTypeEntity objects.
Example return:
[
{
"id": 1,
"type": "forNewsPage"
}
]
Schema
id: number
object identifier
example: 1764type: string
type value
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]
Integration Collections is a module for storing various data that can be used for integrating different services and systems. To integrate third-party services, it is necessary to create and configure collections.
const { IntegrationCollections } = defineOneEntry('your-url');
Getting all collections
const result = await IntegrationCollections.getICollections();
Schema
langCode: string
Language code
example: en_USuserQuery: object
Optional set query parametersuserQuery.limit: number
Optional parameter for pagination, default is 0
example: 0userQuery.offset: number
Optional parameter for pagination, default is 30
example: 30
Get all collections.
Example return:
[
{
"id": 1764,
"localizeInfos": {
"en_US": {
"title": "Collection 1"
}
},
"identifier": "collection",
"formId": 1,
"attributeSetId": "1",
"selectedAttributeMarkers": "marker1, marker2"
}
]
Schema
id: number
object identifier
example: 1764localizeInfos: CommonLocalizeInfos
Name considering localization
example: OrderedMap { "en_US": OrderedMap { "title": "Collection 1" } }identifier: string
Text identifier for record field
example: 1
default: markerformId: number
Identifier for the form used by the order storage
default:attributeSetId: string
Identifier of the set of attributes used by the form attached to the collection
example: 1
default:selectedAttributeMarkers: string
Text identifiers of form object attributes for display in the data table
example: marker1, marker2
default:
Get collection by id.
const result = await IntegrationCollections.getICollectionById(1);
Schema
id:* number
Collection id
example: 10langCode: string
Language code
example: en_US
Example return:
{
"id": 1764,
"localizeInfos": {
"en_US": {
"title": "Collection 1"
}
},
"identifier": "collection",
"formId": 1,
"attributeSetId": "1",
"selectedAttributeMarkers": "marker1, marker2"
}
Schema
id: number
object identifier
example: 1764localizeInfos: CommonLocalizeInfos
Name considering localization
example: OrderedMap { "en_US": OrderedMap { "title": "Collection 1" } }identifier: string
Text identifier for record field
example: collection
default: markerformId: number
Identifier for the form used by the order storage
example: 1
default:attributeSetId: string
Identifier of the set of attributes used by the form attached to the collection
example: 1
default:selectedAttributeMarkers: string
Text identifiers of form object attributes for display in the data table
example: marker1, marker2
Get all records belonging to the collection by collection id.
const result = await IntegrationCollections.getICollectionRowsById(1);
Schema
id:* number
Collection id
example: 10langCode: string
Language code
example: en_USuserQuery: any
Optional set query parametersuserQuery.entityType: any
Entity type
example: 1userQuery.entityId: any
Entity identifier
example: ordersuserQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30
Example return:
{
"total": 100,
"items": [
{
"id": 1764,
"formData": [
{
"marker": "naimenovanie_1",
"type": "string",
"value": "Name"
}
],
"attributeSetIdentifier": "collection_form",
"createdDate": "2025-03-02T16:17:23.970Z",
"updatedDate": "2025-03-02T16:17:23.970Z",
"entityType": "orders",
"entityId": 1
}
]
}
Schema
total: number
Total number of found records
example: 100items: ICollection
CollectionDtoid: number
Object identifier
example: 1764formData:* FormDataType
data submitted by the form attached to the collection
example: List [ OrderedMap { "marker": "naimenovanie_1", "type": "string", "value": "Name" } ]attributeSetIdentifier: string
text identifier of the attribute set used
example: collection_formcreatedDate: string($date-time)
date of record creation in the collection
example: 2025-03-02T16:17:23.970ZupdatedDate: string($date-time)
date of record update in the collection
example: 2025-03-02T16:17:23.970ZentityType: string
record type
example: ordersentityId: number
record identifier
example: 1
Check for the existence of a text identifier (marker)
const result = await IntegrationCollections.validateICollectionMarker('test_collection');
Schema
marker:* string
Collection marker
example: test_collection
Example return:
{
"valid": true
}
Schema
valid: boolean
Valid marker
example:
Getting all records from the collection.
const result = await IntegrationCollections.getICollectionRowsByMarker('test_collection');
Schema
marker:* string
Collection text identifier
example: test_collectionlangCode: string
Language code
example: en_US
Example return:
{
"items": [
{
"id": 2,
"entityId": null,
"entityType": null,
"createdDate": "2025-01-30T01:28:19.906Z",
"updatedDate": "2025-01-30T01:28:19.906Z",
"formData": [{ "marker": "c_marker", "type": "string", "value": "Value" }],
"attributeSetIdentifier": null,
},
],
"total": 1,
}
Schema
total: number
Total number of found records
example: 100items: ICollectionRow[]
Object identifier
example: 2
Getting one record from the collection.
const result = await IntegrationCollections.getICollectionRowByMarkerAndId('test_collection', 1);
Schema
marker:* string
Collection text identifier
example: test_collectionid:* number
Collection record identifier
example: 1langCode: string
Language code
example: en_US
Example return:
{
"id": 1764,
"createdDate": "2025-01-26T17:17:23.013Z",
"updatedDate": "2025-01-26T17:17:23.013Z",
"collectionId": 1,
"langCode": "en_US",
"formData": {
"en_US": [
{
"marker": "marker_1",
"type": "string",
"value": "Title"
}
]
}
}
Schema
id: number
Object identifier
example: 1764createdDate: string($date-time)
Date of record creation in the collectionupdatedDate: string($date-time)
Date of object modificationcollectionId: number
Object identifier of the collection
example: 1langCode: string
Language code in which the record in the collection was created
example: en_USformData: FormDataLangType
Data submitted by the form attached to the collection
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "type": "string", "value": "Title" } ] }
Create a record in the collection
const body = {
"formIdentifier": "collection-form",
"formData": {
"en_US": [
{
"marker": "name_1",
"type": "string",
"value": "Value"
}
]
}
}
const result = await IntegrationCollections.createICollectionRow('test_collection', body);
Schema
marker:* string
Collection text identifier
example: collection1body:* ICollectionFormObject
Object for creating a record
example: { "formIdentifier": "collection-form", "formData": { "en_US": [ { "marker": "collection_marker", "type": "string", "value": "Collection marker" } ] } }langCode: string
Language code
example: en_US
Example return:
{
"formIdentifier": "collection-form",
"formData": {
"en_US": [
{
"marker": "name_1",
"type": "string",
"value": "Value"
}
]
}
}
Schema
formIdentifier: string
Text identifier of the form object attached to the order storage
example: collection_formformData: FormDataLangType
Data submitted by the form attached to the collection
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "type": "string", "value": "Name" } ] }
Edit a record in the collection
const body = {
"formIdentifier": "collection-form",
"formData": {
"en_US": [
{
"marker": "collection_marker",
"type": "string",
"value": "Collection marker"
}
]
}
}
const result = await IntegrationCollections.updateICollectionRow('test_collection', body);
Schema
marker: string
text identifier of the collection
example: test_collectionid: number
row id
example: 10body: object
Object UpdateCollectionRowDto for updating a record in the collection
example: { "formIdentifier": "collection-form", "formData": { "en_US": [ { "marker": "collection_marker", "type": "string", "value": "Collection marker" } ] } }langCode: string
language code
example: en_US
Example return:
{
"formIdentifier": "collection_form",
"formData": {
"en_US": [
{
"marker": "marker_1",
"type": "string",
"value": "Name"
}
]
}
}
Schema
formIdentifier: string
Text identifier of the form object attached to the order storage
example: collection_formformData: FormDataLangType
Data submitted by the form attached to the collection
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "marker_1", "type": "string", "value": "Name" } ] }
Deletion of collection record object
const result = await IntegrationCollections.deleteICollectionRowByMarkerAndId('test_collection', 1);
Schema
marker:* string
text identifier of the collection
example: test_collectionid: number
record identifier in the collection
example: 12
Returns true (in case of successful deletion) or false (in case of unsuccessful deletion) (permission "collections.row.delete" required for access)
Thanks to the support of multiple languages in Oneentry Headless CMS, you can flexibly customize the interface of your dashboard and conveniently manage languages in your project. In this section, you can learn about the principles of working with languages.
const { Locales } = defineOneEntry('your-url');
Searching for all active objects of language localizations (available for use)
const value = await Locales.getLocales()
This method retrieves all active language localization objects from the API. It returns a Promise that resolves to an array of LocaleEntity objects.
Example return:
[
{
"id": 1764,
"shortCode": "en",
"code": "en_US",
"name": "Bengali",
"nativeName": "বাংলা",
"isActive": false,
"image": "🇦🇨",
"position": 1
}
]
Schema
id: number
object identifier
example: 1764shortCode: string
language code (short)
example: encode: string
language code with country code
example: en_USname string
Language name (in English)
example: BengalinativeName string
Language name (in native language)
example: বাংলাisActive: boolean
Flag of usage
example: falseimage: string
Graphic image of the language (under development)
example: 🇦🇨position: { description:position number }
The menu is a key element of the structure and navigation of your site. With the menu, you provide visitors with a convenient way to navigate through different sections of your application and find the information they need.
In OneEntry Headless CMS, you can create the menu structure directly from your personal account. This allows you to change the set of pages included in the menu, their order, and titles without modifying the source code of your application.
Additionally, if necessary, you can create nested levels in the menu, allowing for a tree-like structure with sections and subsections.
All of this enables you to work conveniently and flexibly with the navigation of your application directly from your personal account.
At the core of the menu are pages. The menu structure is created from pages.
You can define:
Which pages will be included in the menu In what order they will be presented to the user What title will be given to the menu item leading to a specific page The nesting of menu items (Tree structure) Some of these concepts were discussed in the pages section, and some page settings affect the menu structure you can create from these pages.
const { Menus } = defineOneEntry('your-url')
Getting pages included in the menu by marker
const value = await Menus.getMenusByMarker('my-marker')
Schema
marker:* string
Menu marker
example: my-markerlangCode: string
Language code
example: en_US
This method retrieves a single menu object based on its marker (marker) from the API. It returns a Promise that resolves to a single menu object as a ContentMenuDto object with included pages.
Example return:
{
"id": 1764,
"identifier": "catalog",
"localizeInfos": {
"title": "Main Menu"
},
"pages": [
{
"id": 11,
"pageUrl": "122",
"localizeInfos": {
"title": "12",
"content": "",
"menuTitle": "12"
},
"position": 0,
"parentId": null
}
]
}
Schema
id: number
object identifier
example: 1764identifier: string
textual identifier for a record field
example: cataloglocalizeInfos Record<string, any>
json object description of the menu item with the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Main Menu" } }pages:
data of the pages included in the menu
example: List [ OrderedMap { "id": 11, "pageUrl": "122", "localizeInfos": OrderedMap { "en_US": OrderedMap { "title": "12", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "12" } }, "position": 0, "parentId": null } ]
The ability to make and pay for goods or services is an integral part of the E-commerce application. In this section, you can familiarize yourself with the principles of working with orders.
const { Orders } = defineOneEntry('your-url');
Getting all order storage objects 🔐 This method requires authorization.
const value = await Orders.getAllOrdersStorage()
Schema
langCode: number
Optional language field
example: en_USoffset: number
Optional parameter for pagination, default is 0
example: 0limit: number
Optional parameter for pagination, default is 30
example: 30
This method getting all the order storage objects. The method will add the default language to the request body. If you want to change the language, just pass it with the second argument
Example return:
[
{
"id": 1764,
"localizeInfos": {
"title": "Order 1"
},
"identifier": "catalog",
"generalTypeId": 4,
"formIdentifier": "catalog-form",
"paymentAccountIdentifiers": [
{
"identifier": "p1"
}
]
}
]
Schema
id: number
object identifier
example: 1764localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }identifier string
textual identifier for the record field
example: cataloggeneralTypeId number
type identifier
example: 4formIdentifier string
textual identifier for the form used by the order storage
example: catalog-formpaymentAccountIdentifiers Array<{identifier:string}
array of textual identifiers of payment accounts used by the order storage
example: [{ "identifier": "p1" }]
Getting all orders from the order storage object created by the user 🔐 This method requires authorization.
const value = await Orders.getAllOrdersByMarker('my-order')
Schema
marker:* string
Textual identifier of the order storage object
example: my-orderlangCode: string
Optional language field
example: en_USoffset: number
Offset parameter. Default 0
example: 0limit: number
Limit parameter. Default 30
example: 30
This method getting all order storage object by marker. The method will add the default language to the request body. If you want to change the language, just pass it with the second argument
Example return:
{
"total": 100,
"items": [
{
"id": 1764,
"statusIdentifier": "inprogress",
"formIdentifier": "order-form",
"formData": [
{
"marker": "name_1",
"type": "string",
"value": "Name"
}
],
"products": [
{
"id": 1,
"title": "Laminate Floorwood Maxima, 9811 Oak Mistral",
"sku": null,
"price": "1.00",
"quantity": 10,
"previewImage": [
{
"filename": "files/project/page/36/image/20240322_77c83b02-4c82-4bea-80eb-3763c469b00e.jpg",
"downloadLink": "http://my-site.zone/files/project/page/36/image/20240322_77c83b02-4c82-4bea-80eb-3763c469b00e.jpg",
"size": 296391,
"previewLink": ""
}
]
}
],
"totalSum": "12.00",
"currency": "USD",
"createdDate": "2023-01-01 12:12",
"paymentAccountIdentifier": "payment-1",
"paymentAccountLocalizeInfos": {"title": "Account 1"
},
"isHistory": true
}
]
}
Schema
total: number
total number of found records
example: 100statusIdentifier: string
text identifier of the order status
example: inprogressformIdentifier: string
text identifier of the form status
example: order-formformData FormDataType
data submitted by the form linked to the order store
example: [{ "marker": "name_1", "value": "Name" } ]products Record<string, string | any>[]
array of products added to ordertotalSum string
total order amount
example: 12.00currency string
currency used to pay for the order
example: USDcreatedDate string
date when the order was created
example: 2023-01-01 12:12price number
price of the product per unit (at the time of ordering)
example: 20.00paymentAccountIdentifier string
textual identifier for the order payment
example: payment-1paymentAccountLocalizeInfos CommonLocalizeInfos
payment account name considering localization
example: { "title": "Account 1" }isHistory boolean
indicates that the order has been saved in the order history
example: true
Get one order storage object by marker 🔐 This method requires authorization.
const value = await Orders.getOrderByMarker('my-order')
Schema
marker:* string
The text identifier of the order storage object
example: my-orderlangCode: string
Optional language field
example: en_US
This method retrieves one order storage object by marker.
Example return:
{
"id": 2,
"localizeInfos": {
"title": "My order"
},
"position": 1,
"identifier": "my_order",
"formIdentifier": "orderForm",
"generalTypeId": 21,
"paymentAccountIdentifiers": [
{
"identifier": "cash"
}
]
}
Schema
id: number
object identifier
example: 1764localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }identifier string
textual identifier for the record field
example: cataloggeneralTypeId number
type identifier
example: 4formIdentifier string
textual identifier for the form used by the order storage
example: catalog-formpaymentAccountIdentifiers Array<{identifier:string}
array of textual identifiers of payment accounts used by the order storage
example: [{ "identifier": "p1" }]
Getting one order by marker and id from the order storage object created by the user 🔐 This method requires authorization.
const value = await Orders.getOrderByMarkerAndId('my-order', 1764)
Schema
marker:* string
The text identifier of the order storage object
example: my-orderid:* number
ID of the order object
example: 1764langCode: string
Optional language field
example: en_US
This method retrieves one order storage object by marker and id.
Example return:
{
"id": 1764,
"statusIdentifier": "inprogress",
"formIdentifier": "order-form",
"formData": [
{
"marker": "name_1",
"type": "string",
"value": "Name"
}
],
"products": [
{
"id": 1,
"title": "Floorwood Maxima Laminate, 9811 Oak Mistral",
"sku": null,
"price": "1.00",
"quantity": 10,
"previewImage": [
{
"filename": "files/project/page/36/image/20240322_77c83b02-4c82-4bea-80eb-3763c469b00e.jpg",
"downloadLink": "http://my-site.zone/files/project/page/36/image/20240322_77c83b02-4c82-4bea-80eb-3763c469b00e.jpg",
"size": 296391,
"previewLink": ""
}
]
}
],
"totalSum": "12.00",
"currency": "USD",
"createdDate": "2023-01-01 12:12",
"paymentAccountIdentifier": "payment-1",
"paymentAccountLocalizeInfos": {
"en_US": {
"title": "Account 1"
}
},
"isHistory": true
}
Schema
id: number
object identifier
example: 1764localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }identifier string
textual identifier for the record field
example: cataloggeneralTypeId number
type identifier
example: 4formIdentifier string
textual identifier for the form used by the order storage
example: catalog-formpaymentAccountIdentifiers Array<{identifier:string}
array of textual identifiers of payment accounts used by the order storage
example: [{ "identifier": "p1" }]
Creating an order in the order storage 🔐 This method requires authorization.
const body = {
"formIdentifier": "orderForm",
"paymentAccountIdentifier": "cash",
"formData": {
"marker": "order_name",
"value": "Ivan",
"type": "string"
},
"products": [
{
"productId": 2,
"quantity": 2
}
]
}
const value = await Orders.createOrder('my-order', body)
Schema
marker:* string
Textual identifier of the order storage object
example: my-orderbody:* IOrderData
Object for creating an order
example: {}langCode: String
Optional language field
example: en_US
Schema (body)
formIdentifier: string
text identifier of the form object linked to the order storage
example: barspaymentAccountIdentifier: string
text identifier of the payment object linked to the order storage
example: payment1statusIdentifier: string
text identifier of the order status object (if not specified, the default status will be assigned)
example: inprogressformData: FormDataType
data submitted by the form linked to the order store
example: [{ "marker": "name_1", "value": "Name" } ]products Record<string, string | any>[]
array of products added to orderproductId: number
product identifier
example: 12quantity: number
quantity of the product
example: 1
This method retrieves one order storage object by marker. The method will add the default language to the request body. If you want to change the language, just pass it with the second argument
Example return:
{
"formIdentifier": "bars",
"paymentAccountIdentifier": "payment1",
"statusIdentifier": "inprogress",
"formData": [
{
"marker": "marker_1",
"type": "string",
"value": "Name"
}
],
"products": [
{
"productId": 1,
"quantity": 2
},
{
"productId": 2,
"quantity": 3
}
],
"createdDate": "2024-06-21T09:42:54.848Z",
"currency": "USD",
"totalSum": 345
}
Schema
statusIdentifier: string
text identifier of the order status
example: inprogressformIdentifier: string
text identifier of the form status
example: order-formpaymentAccountIdentifier string
text identifier of the order payment
example: payment-1formData FormDataType
data submitted by the form linked to the order store
example: [{ "marker": "name_1", "value": "Name" } ]products Record<string, string | any>[]
array of products added to ordertotalSum string
total order amount
example: 12.00currency string
currency used to pay for the order
example: USDcreatedDate string
date when the order was created
example: 2023-01-01 12:12
Order modification in the order storage 🔐 This method requires authorization.
const body = {
"formIdentifier": "orderForm",
"paymentAccountIdentifier": "cash",
"formData": {
"marker": "order_name",
"value": "Ivan",
"type": "string"
},
"products": [
{
"productId": 2,
"quantity": 2
}
]
}
const value = await Orders.updateOrderByMarkerAndId('my-order', 1, body)
Schema
marker:* string
The text identifier of the order storage object
example: my-orderid:* number
ID of the order object
example: 1data:* IOrderData
Object for updating an order
example: {}langCode: string
Optional language field
example: en_US
Schema (body)
formIdentifier: string
text identifier of the form object linked to the order storage
example: barspaymentAccountIdentifier: string
text identifier of the payment object linked to the order storage
example: payment1statusIdentifier string
text identifier of the order status object (if not specified, the default status will be assigned)
example: inprogressformData FormDataType
data submitted by the form linked to the order store
example: [{ "marker": "name_1", "value": "Name" } ]products Record<string, string | any>[]
array of products added to orderproductId number
product identifier
example: 12.00quantity number
quantity of the product
example: 1
This method update one order storage object by marker. The method will add the default language to the request body. If you want to change the language, just pass it with the second argument
Example return:
{
"formIdentifier": "bars",
"paymentAccountIdentifier": "payment1",
"statusIdentifier": "inprogress",
"formData": [
{
"marker": "marker_1",
"type": "string",
"value": "Name"
}
],
"products": [
{
"productId": 1,
"quantity": 2
},
{
"productId": 2,
"quantity": 3
}
],
"createdDate": "2024-06-21T09:42:54.848Z",
"currency": "USD",
"totalSum": 345
}
Schema
statusIdentifier: string
text identifier of the order status
example: inprogressformIdentifier: string
text identifier of the form status
example: order-formpaymentAccountIdentifier string
text identifier of the order payment
example: payment-1formData FormDataType
data submitted by the form linked to the order store
example: [{ "marker": "name_1", "value": "Name" } ]products Record<string, string | any>[]
array of products added to ordertotalSum string
total order amount
example: 12.00currency string
currency used to pay for the order
example: USDcreatedDate string
date when the order was created
example: 2023-01-01 12:12
The Pages module in the Headless CMS OneEntry interface allows you to manage pages if it is a web application, or screens if it is a mobile application.
const { Pages } = defineOneEntry('your-url');
Getting all top-level page objects (parentId = null)
const value = await Pages.getRootPages()
Schema
langCode: string
lang code
example: en_US
This method retrieves all top-level page objects from the API. It returns a Promise that resolves to an array of top-level page objects or an empty array [] if there is no data.
Example return:
[
{
"id": 1,
"config": {},
"depth": 0,
"parentId": null,
"pageUrl": "blog",
"attributeSetIdentifier": "page",
"localizeInfos": {
"title": "Blog",
"menuTitle": "Blog",
"htmlContent": "",
"plainContent": ""
},
"position": 1,
"isVisible": true,
"products": 0,
"childrenCount": 1,
"type": "forUsualPage",
"templateIdentifier": "template",
"isSync": true,
"attributeValues": {
"text": {
"type": "string",
"value": "some text",
"position": 0
}
}
}
]
Schema
id: number
object identifier
example: 1764parentId number
parent page identifier, if null, it is a top-level page
example: nullconfig Record<string, number>
output settings for catalog pages
example: OrderedMap { "rowsPerPage": 1, "productsPerRow": 1 }pageUrl string
unique page URLdepth number
page nesting depth relative to parentId
example: 3localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueproducts number
Number of products linked to the page
example: 0attributeSetId: number
attribute set identifier
example: 7forms
Array of FormEntity object identifier values linked to the page (optional)blocks
Array of BlockEntity object identifier values linked to the page (optional)isSync: boolean
indicator of page indexing (true or false)
example: falsetemplateIdentifier: string
Custom identifier of the linked template
example: my-templateattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }position: number
position number for sorting (optional)
example: 192type: string
Page type
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]childrenCount: number
number of nested pages
example: 0
Getting all page objects with product information as an array
const value = await Pages.getPages();
Schema
langCode: string
lang code
example: en_US
This method retrieves all created pages as an array from the API. It returns a Promise that resolves to an array of ContentIndexedPageDto objects or an empty array [] if there is no data.
Example return:
[
{
"id": 2,
"config": {},
"depth": 0,
"parentId": null,
"pageUrl": "catalog",
"attributeSetIdentifier": "page",
"localizeInfos": {
"title": "Catalog",
"menuTitle": "Catalog",
"htmlContent": "",
"plainContent": ""
},
"position": 3,
"isVisible": true,
"products": 3,
"childrenCount": 0,
"type": "forCatalogPages",
"templateIdentifier": "template",
"isSync": true,
"attributeValues": {
"text": {
"type": "string",
"value": "catalog text",
"position": 0
}
}
}
]
Schema
id: number
object identifier
example: 1764parentId number
parent page identifier, if null, it is a top-level page
example: nullconfig Record<string, number>
output settings for catalog pages
example: OrderedMap { "rowsPerPage": 1, "productsPerRow": 1 }pageUrl string
unique page URLdepth number
page nesting depth relative to parentId
example: 3localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueproducts number
Number of products linked to the page
example: 0attributeSetId: number
attribute set identifier
example: 7forms
Array of FormEntity object identifier values linked to the page (optional)blocks
Array of BlockEntity object identifier values linked to the page (optional)isSync: boolean
indicator of page indexing (true or false)
example: falsetemplateIdentifier: string
Custom identifier of the linked template
example: my-templateattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }position: number
position number for sorting (optional)
example: 192type: string
Page type
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]childrenCount: number
number of nested pages
example: 0
Getting a single page object with information about forms, blocks, menus attached to the page
const value = await Pages.getPageById(1);
Schema
id:*
Page object identifier
example: 1langCode:
lang code
example: en_US
This method retrieves a single page object based on its identifier (id) from the API. It returns a Promise that resolves to the page object, with the specific DTO depending on the type of page being returned.
Example return:
{
"id": 1,
"parentId": null,
"pageUrl": "blog",
"depth": 0,
"localizeInfos": {
"title": "Blog",
"menuTitle": "Blog",
"htmlContent": "",
"plainContent": ""
},
"isVisible": true,
"forms": [],
"blocks": [],
"type": "forUsualPage",
"templateIdentifier": "template",
"attributeValues": {
"text": {
"type": "string",
"value": "some text",
"position": 0
}
},
"isSync": true
}
Schema
id: number
object identifier
example: 1764parentId number
parent page identifier, if null, it is a top-level page
example: nullpageUrl string
unique page URLdepth number
page nesting depth relative to parentId
example: 3localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192type string
page type:
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7forms
Array of FormEntity object identifier values linked to the page (optional)blocks
Array of BlockEntity object identifier values linked to the page (optional)isSync: boolean
indicator of page indexing (true or false)
example: falseproducts number
number of products associated with the page
example: 0
Getting a single page object with information about forms, blocks, menus attached to the page by URL
const value = await Pages.getPageByUrl('shop');
Schema
url:* string
Page URL
example: shoplangCode: string
lang code
example: en_US
This method retrieves a single page object based on its URL (url) from the API. It returns a Promise that resolves to the page object, with the specific DTO depending on the type of page being returned.
Example return:
{
"id": 3,
"parentId": 1,
"pageUrl": "blog1",
"depth": 1,
"localizeInfos": {
"title": "Blog 1",
"menuTitle": "Blog 1",
"htmlContent": "",
"plainContent": ""
},
"isVisible": true,
"forms": [],
"blocks": [],
"type": "forUsualPage",
"templateIdentifier": null,
"attributeValues": {},
"isSync": false
}
Schema
id: number
object identifier
example: 1764parentId number
parent page identifier, if null, it is a top-level page
example: nullpageUrl string
unique page URLdepth number
page nesting depth relative to parentId
example: 3localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192type string
page type:
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7forms
Array of FormEntity object identifier values linked to the page (optional)blocks
Array of BlockEntity object identifier values linked to the page (optional)isSync: boolean
indicator of page indexing (true or false)
example: falseproducts number
number of products associated with the page
example: 0
Getting child pages with product information as an array
const value = await Pages.getChildPagesByParentUrl('shop');
Schema
url:* string
Parent page URL
example: shoplangCode: string
Required parameter lang code
example: en_US
Getting child pages with information about products in the form of an array. Returns all created pages as an array of AdminIndexedPageDto objects or an empty array [] (if there is no data) for the selected parent.
Example return:
[
{
"id": 3,
"config": {},
"depth": 1,
"parentId": 1,
"pageUrl": "blog1",
"attributeSetIdentifier": null,
"localizeInfos": {
"title": "Blog 1",
"menuTitle": "Blog 1",
"htmlContent": "",
"plainContent": ""
},
"position": 1,
"isVisible": true,
"products": 0,
"childrenCount": 0,
"type": "forUsualPage",
"templateIdentifier": null,
"isSync": false,
"attributeValues": {}
}
]
Schema
id: number
object identifier
example: 1764parentId number
parent page identifier, if null, it is a top-level page
example: nullconfig Record<string, number>
output settings for catalog pages
example: OrderedMap { "rowsPerPage": 1, "productsPerRow": 1 }pageUrl string
unique page URLdepth number
page nesting depth relative to parentId
example: 3localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueproducts number
Number of products linked to the page
example: 0attributeSetId: number
attribute set identifier
example: 7forms
Array of FormEntity object identifier values linked to the page (optional)blocks
Array of BlockEntity object identifier values linked to the page (optional)isSync: boolean
indicator of page indexing (true or false)
example: falsetemplateIdentifier: string
Custom identifier of the linked template
example: my-templateattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }position: number
position number for sorting (optional)
example: 192type: string
Page type
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]childrenCount: number
number of nested pages
example: 0
Receiving objects ContentPageBlockDto for a related block by URL
const value = await Pages.getFormsByPageUrl('shop');
Schema
url:* string
Page URL
example: shoplangCode: string
lang code
example: en_US
Get PositionBlock objects for a related form by url. Returns an array of PositionBlock objects.
Example return:
[
{
"id": 2,
"localizeInfos": {
"title": "test"
},
"attributeSetIdentifier": "block",
"version": 0,
"position": 2,
"identifier": "test_identifier",
"type": "forTextBlock",
"templateIdentifier": null,
"isVisible": true,
"isSync": false,
"attributeValues": {},
"countElementsPerRow": 0
}
]
Schema
id: number
object identifier
example: 1764version number
object version number
example: 10identifier: string
text identifier for the record field
example: catalogattributeSetId: number
attribute set identifier
example: 7localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }customSettings: BlockCustomSettings
custom settings for different types of blocks
example: OrderedMap { "sliderDelay": 0, "sliderDelayType": "", "productQuantity": 4, "productSortType": "By_ID", "productSortOrder": "Descending", "productCountElementsPerRow": 10, "similarProductRules": List [ OrderedMap { "property": "Descending", "includes": "", "keywords": "", "strict": "" } ] }isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }position: number
position number for sorting (optional)
example: 192type: string
Page type
example: forNewsPage
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]templateIdentifier: string
marker of the template used by the block (can be null)
example: null
Receiving objects ContentPageFormDto for a related form by URL
const value = await Pages.getFormsByPageUrl('shop')
Schema
url:* string
Page URL
example: shoplangCode: string
lang code
example: en_US
Get PositionForm objects for a related form by url. Returns an array of PositionForm objects.
Example return:
[
{
"id": 1764,
"version": 10,
"identifier": "catalog",
"attributeSetId": 0,
"processingType": "email",
"localizeInfos": {
"title": "My form",
"titleForSite": "",
"successMessage": "",
"unsuccessMessage": "",
"urlAddress": "",
"database": "0",
"script": "0"
},
"processingData": {},
"position": 0,
"attributes": [
{
"type": "list",
"marker": "list_marker",
"position": 2,
"listTitles": [
{
"title": "red",
"value": 1,
"position": 1,
"extendedValue": null,
"extendedValueType": null
},
{
"title": "yellow",
"value": 2,
"position": 2,
"extendedValue": null,
"extendedValueType": null
}
],
"validators": {},
"localizeInfos": {
"title": "l1"
}
}
]
}
]
Schema
id: number
object identifier
example: 1764version number
object version number
example: 10identifier: string
text identifier for the record field
example: catalogattributeSetId: number
attribute set identifier
example: 7processingType: string
form processing type
example: email
Enum: [ db, email, script ]localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }processingData:
form dataattributes: Record<string, string>
array of attribute values from the used attribute set for displaying the form (taking into account the specified language)
example: List [ OrderedMap { "type": "list", "marker": "l1", "position": 2, "listTitles": List [ OrderedMap { "title": "red", "value": 1, "position": 1, "extendedValue": null, "extendedValueType": null }, OrderedMap { "title": "yellow", "value": 2, "position": 2, "extendedValue": null, "extendedValueType": null } ], "validators": OrderedMap {}, "localizeInfos": OrderedMap { "title": "l1" } } ]position: number
position number for sorting (optional)
example: 192
Getting settings for the page
const value = await Pages.getConfigPageByUrl('shop')
Schema
url:* string
Page URL
example: shop
This method retrieves the settings for a specific page based on its URL (url). It returns a Promise that resolves to a ConfigPageDto object with page display settings.
Example return:
{
"rowsPerPage": 10,
"productsPerRow": 10
}
Schema
rowsPerPage: number
Number of rows per page
example: 10productsPerRow number
Number of products per row
example: 10
Quick search for page objects with limited output
const value = await Pages.searchPage('cup')
Schema
name:* string
Text for searching page objects (search is performed on the title field of the localizeInfos object with the language taken into account)
example: cuplangCode: string
lang code
example: en_US
This method performs a quick search for page objects based on a text query (name). It returns a Promise that resolves to a ContentIndexedPageDto objects or an empty array [].
Example return:
[
{
"id": 2,
"parentId": null,
"pageUrl": "catalog",
"depth": 0,
"localizeInfos": {
"title": "Catalog",
"menuTitle": "Catalog",
"htmlContent": "",
"plainContent": ""
},
"isVisible": true,
"forms": [],
"blocks": [
"test",
"product_block"
],
"type": "forCatalogPages",
"templateIdentifier": "template",
"attributeValues": {
"text": {
"type": "string",
"value": "catalog text",
"position": 0
}
},
"products": 3,
"isSync": true
}
]
An important part of the E-commerce application is working with payments. In this section, you can learn about the principles of working with payments.
const { Payments } = defineOneEntry('your-url');
Get list of payment sessions 🔐 This method requires authorization.
const value = await Payments.getSessions()
Schema
offset: number
Optional parameter for pagination, default is 0
example: 0limit: number
Optional parameter for pagination, default is 30
example: 30
This method get list of a payment session. It returns a Promise that resolves to a payment session object.
Example return:
{
"total": 100,
"items": [
{
"id": 1764,
"updatedDate": "2024-06-21T09:51:57.785Z",
"type": "session",
"status": "completed",
"orderId": 1,
"paymentAccountId": 1,
"paymentUrl": "https://checkout.stripe.com/c/pay/cs_test_a1iOHnSWAmeN3SN5IgYtPv8Fzv48vGUmKxFuhxD0FOjkOaTAlgiwNY9OYW#fid2BXKsdWBEZmZqcGtxJz8nZGZmcVo0VTZjazFUb2Z8YEBRYkxHJyknZHVsTmB8Jz8ndW5acWB2cVowNEpKcW43TVVBa1NSMU5ST3JfY3VcRGlRSUR8cVx0XFxOXG9Cbn1oM1V0QUExR0RRRnJwV0FCYlNcXUtGdGtzcndgcmJxQVNkQnxvcDBTY0ZpUjZCd319UTU1ME5rXDJIVjYnKSdjd2poVmB3c2B3Jz9xd3BgKSdpZHxqcHFRfHVgJz8ndmxrYmlgWmxxYGgnKSdga2RnaWBVaWRmYG1qaWFgd3YnP3F3cGB4JSUl",
"clientSecret": "pi_3MtwBwLkdIwHu7ix28a3tqPa_secret_YrKJUKribcBjcG8HVhfZluoGH"
}
]
}
Schema
id: number
object identifier
example: 1764updatedDate: string
object modification dateversion: number
object modification version number
example: 10identifier: string
text identifier for the record field
example: catalogtype: string
type may be 'session' or 'intent'
example: sessionlineItems: array
list of itemsorderId: number
order identifier
example: 1paymentAccountId: number
payment account object identifier
example: 1status: string
payment status
example: completedsessionId: string
Payment ID from an external provider
example: 9BE88048TU058770MpaymentUrl: string
payment link
example: https://www.sandbox.paypal.com/checkoutnow?token=9BE88048TU058770MsuccessUrl: string
redirect link after successful payment
example: https://example.com/successcancelUrl string
redirect link after payment cancellation
example: https://example.com/cancelintent string
example: { "amount": 1, "currency": "usd" }intentId number
intent object identifier
example: 1clientSecret string
client secret key
example: pi_3Oyz2kQWzXG1R23w144qG7o4_secret_OeScuCwTpHmyOM1atbm7pWJw2
Get one payment session object by its identifier 🔐 This method requires authorization.
const value = await Payments.getSessionById(1764)
Schema
id:* number
Identifier of the retrieved payment session object
example: 12
This method get a single payment session object by its identifier. It returns a Promise that resolves to a payment session object.
Example return:
{
"id": 1764,
"updatedDate": "2024-06-21T09:51:57.785Z",
"type": "session",
"status": "completed",
"orderId": 1,
"paymentAccountId": 1,
"paymentUrl": "https://checkout.stripe.com/c/pay/cs...",
"clientSecret": "pi_3MtwBwLkdIwHu7ix28a3tqPa_secret..."
}
Schema
id: number
object identifier
example: 1764updatedDate: string
object modification dateversion: number
object modification version number
example: 10identifier: string
text identifier for the record field
example: catalogtype: string
type may be 'session' or 'intent'
example: sessionlineItems: array
list of itemsorderId: number
order identifier
example: 1paymentAccountId: number
payment account object identifier
example: 1status: string
payment status
example: completedsessionId: string
Payment ID from an external provider
example: 9BE88048TU058770MpaymentUrl: string
payment link
example: https://www.sandbox.paypal.com/checkoutnow?token=9BE88048TU058770MsuccessUrl: string
redirect link after successful payment
example: https://example.com/successcancelUrl: string
redirect link after payment cancellation
example: https://example.com/cancelintent: string
example: { "amount": 1, "currency": "usd" }intentId: number
intent object identifier
example: 1clientSecret: string
client secret key
example: pi_3Oyz2kQWz...
Get one payment session object by order identifier 🔐 This method requires authorization.
const value = await Payments.getSessionByOrderId(1764)
Schema
id:* number
Identifier of the retrieved payment session object
example: 1764
This method...
Example return:
{
"id": 1764,
"createdDate": "2025-03-02T21:56:53.600Z",
"updatedDate": "2025-03-02T21:56:53.600Z",
"type": "session",
"status": "completed",
"orderId": 1,
"paymentAccountId": 1,
"paymentUrl": "https://checkout.stripe.com/c/pay/cs_...",
"clientSecret": "pi_3MtwBwLkdIwHu7ix28a3tq..."
}
Schema
id: number
object identifier
example: 1764createdDate: string($date-time)
Object creation dateupdatedDate: string($date-time)
Object modification datetype:* string
Session type Enum: [ session, intent ]
example: sessionstatus:* string
Payment status Enum: [ waiting, completed, canceled, expired ]
example: completedorderId:* number
Order ID
example: 1paymentAccountId:* number
Payment account ID
example: 1paymentUrl:* string
Payment link
example: https://checkout.stripe.com/c/pay/cs_...clientSecret: string
Secret key
example: pi_3MtwBwLkdIwHu7ix28a3tqPa_secret...
This method Update payment session 🔐 This method requires authorization.
const body = {
"status": "completed",
"paymentUrl": "https://www.paypal.com/checkoutnow?token=6TC49050A66458205"
};
const value = await Payments.updateSessionById(10, body)
Schema (body)
status: string
payment status Enum: [ waiting, completed, canceled, expired ]
example: completedpaymentUrl:* string
payment link
example: https://www.paypal.com/checkoutnow?token=6TC49050A66458205
Example return:
{
"id": 1764,
"createdDate": "2025-03-02T22:11:25.315Z",
"updatedDate": "2025-03-02T22:11:25.315Z",
"type": "session",
"status": "completed",
"orderId": 1,
"paymentAccountId": 1,
"paymentUrl": "https://checkout.stripe.com/c/pay/cs_test_a1iOHnSWAmeN3SN5IgYtPv8Fzv48vGUmKxFuhxD0FOjkOaTAlgiwNY9OYW#fid2BXKsdWBEZmZqcGtxJz8nZGZmcVo0VTZjazFUb2Z8YEBRYkxHJyknZHVsTmB8Jz8ndW5acWB2cVowNEpKcW43TVVBa1NSMU5ST3JfY3VcRGlRSUR8cVx0XFxOXG9Cbn1oM1V0QUExR0RRRnJwV0FCYlNcXUtGdGtzcndgcmJxQVNkQnxvcDBTY0ZpUjZCd319UTU1ME5rXDJIVjYnKSdjd2poVmB3c2B3Jz9xd3BgKSdpZHxqcHFRfHVgJz8ndmxrYmlgWmxxYGgnKSdga2RnaWBVaWRmYG1qaWFgd3YnP3F3cGB4JSUl",
"clientSecret": "pi_3MtwBwLkdIwHu7ix28a3tqPa_secret_YrKJUKribcBjcG8HVhfZluoGH"
}
Schema
id:* number
Object ID
example: 1764createdDate: string($date-time)
Object creation dateupdatedDate: string($date-time)
Object modification datetype:* string
Session type Enum: [ session, intent ]
example: sessionstatus:* string
Payment status Enum: [ waiting, completed, canceled, expired ]
example: completedorderId:* number
Order ID
example: 1paymentAccountId:* number
Payment account ID
example: 1paymentUrl:* string
Payment link
example: https://checkout.stripe.com/c/pay/cs_...clientSecret: string
Secret key
example: pi_3MtwBwLkdIwHu7i...
Create payment session 🔐 This method requires authorization.
const value = await Payments.createSession(1, 'session')
Schema
orderId:* number
Order identifier
example: 1type:* 'session' | 'intent'
Session type
example: sessionautomaticTaxEnabled: boolean
Automatic calculation of the tax rate
example: false
This method creation of a payment session. It returns a Promise that resolves to a payment session object.
Example return:
{
"id": 1764,
"updatedDate": "2024-06-21T09:53:28.898Z",
"version": 10,
"identifier": "my-id",
"paymentUrl": "https://paymewntlink.com"
}
Schema
id: number
object identifier
example: 1764updatedDate: string
object modification dateversion: number
object modification version number
example: 10identifier: string
text identifier for the record field
example: catalogtype: string
type may be 'session' or 'intent'
example: sessionlineItems: array
list of itemsorderId: number
order identifier
example: 1paymentAccountId: number
payment account object identifier
example: 1status: string
payment status
example: completedsessionId: string
Payment ID from an external provider
example: 9BE88048TU058770MpaymentUrl: string
payment link
example: https://www.sandbox.paypal.com/checkoutnow?token=9BE88048TU058770MsuccessUrl: string
redirect link after successful payment
example: https://example.com/successcancelUrl: string
redirect link after payment cancellation
example: https://example.com/cancelintent: string
example: { "amount": 1, "currency": "usd" }intentId: number
intent object identifier
example: 1clientSecret: string
client secret key
example: pi_3Oyz2kQWzXG1R23w144qG7o4_secret_OeScuCwTpHmyOM1atbm7pWJw2
Get payment settings 🔐 This method requires authorization.
const value = await Payments.getConnected()
This method get payment settings. It returns a Promise that resolves to a payment connection object.
Example return:
{
"stripeAccountId": "acct_1OtRiIHTHOaLRCAa",
"stripePublishableKey": "pk_51OOvk2HPDnVW5KWJwZfiYAlTLAytYqYYKYjGkxm6PqDD4BATCwuRDGgVYXNCqnvwrewgtDVaGyju5VfClW3GrxxT005KnY7MS3"
}
Schema
stripeAccountId: string
Identifier of connected Stripe account
example: acct_1OtRiIHTHOaLRCAastripePublishableKey: string
Stripe Connect public key
example: pk_51OOvk2HPDnVW5KWJwZfiYAlTLAytYqYYKYjGkxm6PqDD4BATCwuRDGgVYXNCqnvwrewgtDVaGyju5VfClW3GrxxT005KnY7MS3paypalAccountId: string
Identifier of connected Paypal account
example: 4Q0BANTF5BE7N
Get all payment accounts as array
const value = await Payments.getAccounts()
This method get payment account as an array. It returns a Promise that resolves to a payment account object.
Example return:
[
{
"id": 2,
"localizeInfos": {
"title": "Stripe"
},
"identifier": "stripe",
"type": "stripe",
"isVisible": true
}
]
Schema
id: number
object identifier
example: 1764updatedDate: string
object modification dateversion: number
object modification version number
example: 10identifier: string
text identifier for the recording field
example: cataloglocalizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" }type: string
type may be 'stripe', 'paypal' or 'custom'
example: stripeisVisible: boolean
visibility indicator of the payment account
example: true
Get one payment account object by its identifier 🔐 This method requires authorization.
const value = await Payments.getAccountById(1764)
Schema
id:* number
Identifier of the retrieved payment account object
example: 1764
This method get a single payment account object by its identifier. It returns a Promise that resolves to a payment account object.
Example return:
{
"id": 2,
"localizeInfos": {
"title": "Stripe"
},
"identifier": "stripe",
"type": "stripe",
"isVisible": true
}
Schema
id: number
object identifier
example: 1764updatedDate: string
object modification dateversion: number
object modification version number
example: 10identifier: string
text identifier for the recording field
example: cataloglocalizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" }type: string
type may be 'stripe', 'paypal' or 'custom'
example: stripeisVisible: boolean
visibility indicator of the payment account
example: true
Webhook for Stripe
const value = await Payments.webhookStripe()
This method use webhook for Stripe. Returns true (in case of successful execution) or false (in case of unsuccessful execution).
Products is a separate module in the Headless CMS OneEntry interface that allows you to create a product catalog or a set of multimedia elements, such as a gallery.
const { Products } = defineOneEntry('your-url');
This module accepts a set of user parameters called userQuery. If the parameters are not passed to the method, the default value will be applied. Some methods accept the body as a parameter for filtering. If you don't want to set up sorting, pass an empty array or don't pass anything. Parameters:
const userQuery = {
offset: 0,
limit: 30,
sortOrder: 'DESC',
sortKey: 'id'
}
Schema
offset: number
pagination parameter, default 0
example: 0limit: number
pagination parameter, default 30
example: 30sortKey: string
field for sorting (default not set - sorting by position, possible values: id, title, date, price, position)
Available values : id, position, title, date, pricesortOrder: string
sorting order DESC | ASC (default DESC)
example: "DESC"
"conditionMarker" by which values are filtered (not set by default), possible values:
'in' - Contains,
'nin' - Does not contain,
'eq' - Equal,
'neq' - Not equal,
'mth' - Greater than,
'lth' - Less than,
'exs' - Exists,
'nexs' - Does not exist
Search all product objects with pagination and filter
const body = [
{
"attributeMarker": "price",
"conditionMarker": "mth",
"statusMarker": "waiting",
"conditionValue": 1,
"pageUrls": [
"23-laminat-floorwood-maxima"
],
"isNested": false,
"title": ""
},
{
"attributeMarker": "price",
"conditionMarker": "lth",
"conditionValue": 3,
"pageUrls": [
"23-laminat-floorwood-maxima"
],
"isNested": false,
"title": ""
}
]
const value = await Products.getProducts(body)
Schema
body:* IFilterParams[]
Request body. Default [].
example: []langCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parameters.userQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: iduserQuery.statusId: number
Optional parameter - search by status id
example: 1userQuery.statusMarker: string
Optional identifier of the product page status
example: waitinguserQuery.conditionValue: string
Optional value that is being searched
example: 3userQuery.conditionMarker: string
Optional identifier of the filter condition by which values are filtered
example: mthuserQuery.attributeMarker: string
Optional text identifier of the indexed attribute by which values are filtered
example: price
Schema (body)
attributeMarker: string
textual identifier of the attribute
example: priceconditionMarker: string
textual identifier of the condition
example: instatusMarker: string
textual identifier of the product page status (default not set)
example: status_1conditionValue: number
condition value
example: 1pageUrls: Array
unique part of the page URL (after the last "/")title: string
example: Iphone 17 Pro
product nameisNested: boolean
search indicator for all nested categories (pageUrls)
example: false
This method searches for all products objects with pagination that do not have a category, based on the provided query parameters (userQuery). It returns a Promise that resolves to an array of items, where each item is a ContentIndexedProductDto object.
Example return:
{
"total": 100,
"items": [
{
"id": 4,
"localizeInfos": {
"title": "Cosmo"
},
"statusIdentifier": null,
"statusLocalizeInfos": {},
"attributeSetIdentifier": "products",
"position": 1,
"templateIdentifier": null,
"isPositionLocked": false,
"shortDescTemplateIdentifier": null,
"price": 150,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": 150,
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Cosmo",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
Search all product objects with pagination that do not have a category
const value = await Products.getProductsEmptyPage('en_US', userQuery)
Schema
langCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parameters.userQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.statusMarker: string
Optional identifier of the product page status
example: waitinguserQuery.conditionValue: string
Optional value that is being searched
example: 1userQuery.conditionMarker: string
Optional identifier of the filter condition by which values are filtered
example: lthuserQuery.attributeMarker: string
Optional text identifier of the indexed attribute by which values are filtered
example: priceuserQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: id
This method searches for product page objects with pagination that do not have a category, based on the provided query parameters (userQuery). It returns a Promise that resolves to an array of items, where each item is a ContentIndexedProductDto object.
Example return:
{
"total": 100,
"itema": [
{
"id": 4,
"localizeInfos": {
"title": "Cosmo"
},
"statusIdentifier": null,
"statusLocalizeInfos": {},
"attributeSetIdentifier": "products",
"position": 1,
"templateIdentifier": null,
"isPositionLocked": false,
"shortDescTemplateIdentifier": null,
"price": 150,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": 150,
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Cosmo",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
Search all product objects with pagination for selected category
const body = [
{
"attributeMarker": "price",
"conditionMarker": "mth",
"statusMarker": "waiting",
"conditionValue": 1,
"pageUrls": [
"23-laminat-floorwood-maxima"
],
"isNested": false,
"title": ""
},
{
"attributeMarker": "price",
"conditionMarker": "lth",
"conditionValue": 3,
"pageUrls": [
"23-laminat-floorwood-maxima"
],
"isNested": false,
"title": ""
}
];
const value = await Products.getProductsByPageId(1764, body);
Schema
id:* number
Page id
example: 1body: IFilterParams[]
Request body
example: []langCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parameters.
example: {}userQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.statusMarker: string
Optional identifier of the product page status
example: waitinguserQuery.conditionValue: string
Optional value that is being searched
example: 1userQuery.conditionMarker: string
Optional identifier of the filter condition by which values are filtered
example: mthuserQuery.attributeMarker: string
Optional text identifier of the indexed attribute by which values are filtered
example: priceuserQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: id
Schema (body)
attributeMarker: string
textual identifier of the attribute
example: priceconditionMarker: string
textual identifier of the condition
example: instatusMarker: string
textual identifier of the product page status (default not set)
example: status_1conditionValue: number
condition value
example: 1pageUrls: Array
unique part of the page URL (after the last "/")title: string
example: Iphone 17 Pro
product nameisNested: boolean
search indicator for all nested categories (pageUrls)
example: false
This method searches for all products objects with pagination for the selected category, based on the provided query parameters (userQuery). It returns a Promise that resolves to an array of items, where each item is a ContentIndexedProductDto object.
Example return:
{
"total": 100,
"items": [
{
"id": 2,
"localizeInfos": {
"title": "Box"
},
"statusIdentifier": "sold",
"statusLocalizeInfos": {
"title": "Sold"
},
"attributeSetIdentifier": "products",
"position": 3,
"templateIdentifier": null,
"isPositionLocked": false,
"shortDescTemplateIdentifier": null,
"price": 148,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": 148,
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Box text",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "$",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true,
"isPositionLocked": false
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
Search information about products and prices for selected category
const value = await Products.getProductsPriceByPageUrl('catalog')
Schema
url:* string
Page url
example: cataloglangCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parametersuserQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.statusMarker: string
Optional identifier of the product page status
example: waitinguserQuery.conditionValue: string
Optional value that is being searched
example: 1userQuery.conditionMarker: string
Optional identifier of the filter condition by which values are filtered
example: mthuserQuery.attributeMarker: string
Optional text identifier of the indexed attribute by which values are filtered
example: priceuserQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: id
This method searches for information about products and prices for the selected category, based on the provided query parameters (userQuery). It returns a Promise that resolves to an array of items, where each item is a ContentIndexedProductDto object.
Example return:
{
"total": 100,
"items": [
{
"id": 1764,
"price": 0
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764price: number
price value of the product page taken from the index
example: 0
Search for all product objects with pagination for the selected category (by its URL)
const body = [
{
"attributeMarker": "price",
"conditionMarker": "mth",
"statusMarker": "waiting",
"conditionValue": 1,
"pageUrls": [
"23-laminat-floorwood-maxima"
],
"isNested": false,
"title": ""
},
{
"attributeMarker": "price",
"conditionMarker": "lth",
"conditionValue": 3,
"pageUrls": [
"23-laminat-floorwood-maxima"
],
"isNested": false,
"title": ""
}
]
const value = await Products.getProductsByPageUrl('catalog', body)
Schema
url:* string
Page url
example: catalogbody:* IFilterParams[]
Request body
example: []langCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parameters.userQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.statusMarker: string
Optional identifier of the product page status
example: waitinguserQuery.conditionValue: string
Optional value that is being searched
example: 1userQuery.conditionMarker: string
Optional identifier of the filter condition by which values are filtered
example: mthuserQuery.attributeMarker: string
Optional text identifier of the indexed attribute by which values are filtered
example: priceuserQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: id
Schema (body)
attributeMarker: string
textual identifier of the attribute
example: priceconditionMarker: string
textual identifier of the condition
example: instatusMarker: string
textual identifier of the product page status (default not set)
example: status_1conditionValue: number
condition value
example: 1pageUrls: Array
unique part of the page URL (after the last "/")title: string
example: Iphone 17 Pro
product nameisNested: boolean
search indicator for all nested categories (pageUrls)
example: false
This method searches for all products objects with pagination for the selected category, based on the provided query parameters (userQuery). It returns a Promise that resolves to an array of items, where each item is a ContentIndexedProductDto object.
Example return:
{
"total": 100,
"items": [
{
"id": 2,
"localizeInfos": {
"title": "Box"
},
"statusIdentifier": "sold",
"statusLocalizeInfos": {
"title": "Sold"
},
"attributeSetIdentifier": "products",
"position": 3,
"templateIdentifier": null,
"shortDescTemplateIdentifier": null,
"price": 148,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": 148,
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Box text",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "$",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true,
"isPositionLocked": false
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
Search for all related product objects by page id
const value = await Products.getRelatedProductsById(1)
Schema
id:* number
Product page identifier for which to find relationship
example:langCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parameters
example: []userQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: id
This method retrieves all related product page objects for a specific product based on its identifier (id) from the API. It accepts an optional userQuery parameter for additional query parameters such as offset, limit, sortOrder, and sortKey. It returns a Promise that resolves to an array of ContentIndexedProductDto objects.
Example return:
{
"total": 100,
"items": [
{
"id": 2,
"localizeInfos": {
"title": "Box"
},
"statusIdentifier": "sold",
"statusLocalizeInfos": {
"title": "Sold"
},
"attributeSetIdentifier": "products",
"position": 3,
"templateIdentifier": null,
"isPositionLocked": false,
"shortDescTemplateIdentifier": null,
"price": 148,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": 148,
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Box text",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "$",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true,
"isPositionLocked": false
}
]
}
Schema
total: number
total number of found records
example: 100id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
Getting multiple products by its ids
const value = await Products.getProductsByIds('1, 5, 8', 'en_US')
Schema
ids:* string
Product page identifiers for which to find relationships
example: 1,3,5,15langCode: string
Language code parameter. Default "en_US"
example: en_USuserQuery: IProductsQuery
Optional set query parameters
example: []userQuery.offset: number
Optional parameter for pagination, default is 0
example: 0userQuery.limit: number
Optional parameter for pagination, default is 30
example: 30userQuery.sortOrder: string
Optional sorting order DESC | ASC
example: DESCuserQuery.sortKey: string
Optional field to sort by (id, title, date, price, position, status)
example: id
This method retrieves a products objects based on its identifiers (ids) from the API. It returns a Promise that resolves to a IProductsEntity objects for the product.
Example return:
[
{
"id": 1764,
"localizeInfos": {
"en_US": {
"title": "Product"
}
},
"isVisible": true,
"isSync": true,
"price": 0,
"additional": {
"prices": {
"min": 0,
"max": 100
}
},
"blocks": [
null
],
"sku": "0-123",
"productPages": [
{
"id": 8997,
"pageId": 1176,
"productId": 8872
}
],
"statusLocalizeInfos": {
"en_US": {
"title": "Product"
}
},
"templateIdentifier": "my-template",
"shortDescTemplateIdentifier": "my-template-short",
"attributeValues": {
"en_US": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
}
},
"attributeSetIdentifier": "my-set",
"statusIdentifier": "my-status",
"position": 1
}
]
Schema
id: number
object identifier
example: 1764localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueisSync: boolean
indicator of page indexing (true or false)
example: falseprice: number
price value of the product page taken from the index
example: 0additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }blocks: array
product blocks
example: ['product_block']sku: string
product SKU value taken from the index
example: 1productPages: array
ProductPageEntity objects linked to the product page (optional)
example: List [ OrderedMap { "id": 8997, "pageId": 1176, "productId": 8872 } ]statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }templateIdentifier: string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-shortattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }attributeSetIdentifier: string
textual identifier of the attribute set used
example: 'my-set'statusIdentifier: string
textual identifier of the product status
example: 'my-status'position: number
position number (for sorting)
example: 1
Get one product object by id
const value = await Products.getProductById(1)
Schema
id:* Product id
- Product id*
example: 1langCode:
Language code parameter. Default "en_US"
example: en_US
This method retrieves a single product object based on its identifier (id) from the API. It returns a Promise that resolves to a ContentIndexedProductDto object for the product.
Example return:
{
"id": 3,
"localizeInfos": {
"title": "Product"
},
"statusIdentifier": null,
"statusLocalizeInfos": {},
"attributeSetIdentifier": "products",
"position": 1,
"templateIdentifier": null,
"shortDescTemplateIdentifier": null,
"price": 120,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": "120",
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Prod",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "$",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true,
"productPages": {
"id": 3,
"pageId": 2,
"productId": 3,
"positionId": 215
},
"blocks": [
"product_block",
"another"
]
}
Schema
id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
Get ContentPageBlockDto objects by product identifier
const value = await Products.getProductBlockById(1764)
Schema
id:* number
Product id
example: 1764
This method Getting a product block object by product id.
Example return:
[
{
"id": 3,
"attributeSetIdentifier": null,
"localizeInfos": {
"title": "Product block"
},
"version": 0,
"position": 1,
"identifier": "product_block",
"type": "forProductBlock",
"customSettings": {
"productConfig": {
"quantity": "1",
"countElementsPerRow": "1"
},
"similarProductRules": [],
"condition": {
"name": "cost",
"costTo": 130,
"costFrom": 0
},
"sliderDelay": null,
"sliderDelayType": null
},
"templateIdentifier": null,
"isVisible": true,
"isSync": false,
"attributeValues": {}
}
]
Schema
id: number
object identifier example: 1764attributeSetId: number
identifier for the used attribute set
example: 7localizeInfos: CommonLocalizeInfos
block name with localization
example: OrderedMap { "en_US": OrderedMap { "title": "My block" } }customSettings: BlockCustomSettings
custom settings for different block types
example: OrderedMap { "sliderDelay": 0, "sliderDelayType": "", "productQuantity": 4, "productSortType": "By_ID", "productSortOrder": "Descending", "productCountElementsPerRow": 10, "similarProductRules": List [ OrderedMap { "property": "Descending", "includes": "", "keywords": "", "strict": "" } ] }version: number
object version number
example: 10identifier: string
textual identifier for the field record
example: catalog
default: markerposition: number
position number (for sorting)
example: 192attributeValues: Record<string, string>
array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }type: string
block type
example: forNewsPagetemplateIdentifier: string
template marker used by the block (can be null)
Enum: [ forCatalogProducts, forBasketPage, forErrorPage, forCatalogPages, forProductPreview, forProductPage, forSimilarProductBlock, forStatisticProductBlock, forProductBlock, forForm, forFormField, forNewsPage, forNewsBlock, forNewsPreview, forOneNewsPage, forUsualPage, forTextBlock, forSlider, service ]
example: null
Quick search for page objects with limited output
const value = await Products.searchProduct('cup')
Schema
name:* string
Text to search product page objects (search is based on the title field of the localizeInfos object with language consideration)
example: cuplangCode: string
Language code. Default "en_US"
example: en_US
This method performs a quick search for product page objects based on a text query name. The search is performed on the title field of the localizeInfos object, taking the specified lang language code into consideration. It returns a Promise that resolves to an array of ContentIndexedProductDto objects.
Example return:
[
{
"id": 4,
"localizeInfos": {
"title": "Cosmo"
},
"statusIdentifier": null,
"statusLocalizeInfos": {},
"attributeSetIdentifier": "products",
"position": 1,
"templateIdentifier": null,
"isPositionLocked": false,
"shortDescTemplateIdentifier": null,
"price": 150,
"additional": {
"prices": {
"min": 120,
"max": 150
}
},
"sku": null,
"isSync": true,
"attributeValues": {
"price": {
"type": "integer",
"value": "150",
"position": 1,
"isProductPreview": false
},
"product-name": {
"type": "string",
"value": "Cosmo",
"position": 0,
"isProductPreview": false
},
"currency_products": {
"type": "string",
"value": "",
"position": 2,
"isProductPreview": false
}
},
"isVisible": true,
"productPages": {
"id": 6,
"pageId": 2,
"productId": 4,
"positionId": 229
},
"blocks": "product_block"
}
]
Schema
id: number
object identifier
example: 1764additional: Record<string, any>
additional value from the index
example: OrderedMap { "prices": OrderedMap { "min": 0, "max": 100 } }statusLocalizeInfos: CommonLocalizeInfos
json description of the item status object, taking into account the language
example: { "title": "Product" }localizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }isVisible: boolean
Page visibility flag
example: trueposition: number
position number (for sorting)
example: 192templateIdentifier: string
custom identifier of the associated template
example: my-templateattributeSetId: number
attribute set identifier
example: 7blocks: array
product blocks
example: ['product_block']isSync: boolean
indicator of page indexing (true or false)
example: falseattributeValues: Record<string, string>
Array of attribute values from the index (presented as a pair of custom attribute identifier: attribute value)
example: OrderedMap { "en_US": OrderedMap { "marker": OrderedMap { "value": "", "type": "string" } } }statusId: number
status identifiers of the product page (can be null)
example: 1sku: string
product SKU value taken from the index
example: 1relatedIds: array
identifiers of related product pages
example: List [ 1, 2, 3 ]price: number
price value of the product page taken from the index
example: 0templateIdentifier string
custom identifier of the associated template
example: my-templateshortDescTemplateIdentifier string
custom identifier of the associated template for short description
example: my-template-short
To create additional filtering conditions for catalog items in Headless CMS OneEntry, the functionality of Product Statuses has been added. In this tab, you can create a status that will serve as an additional filter alongside the existing conditions from attributes.
const { ProductStatuses } = defineOneEntry('your-url');
Search for all product status objects
const value = await ProductStatuses.getProductStatuses()
Schema
langCode: string
language code
example: en_US
This method searches for all product status objects from the API. It returns a Promise that resolves to an array of product status objects.
Example return:
[
{
"id": 1764,
"version": 10,
"position": 2,
"identifier": "catalog",
"localizeInfos": {
"title": "Status 1"
}
}
]
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloglocalizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }
Search for a single product status object by identifier
const value = await ProductStatuses.getProductStatusesById(1)
Schema
id:* number
Status id
example: 1langCode: string
language code
example: en_US
This method searches for a product status object based on its identifier (id) from the API. It returns a Promise that resolves to a product status object.
Example return:
{
"id": 1764,
"version": 10,
"position": 2,
"identifier": "catalog",
"localizeInfos": {
"title": "Status 1"
}
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloglocalizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }
Search for a product status object by its textual identifier (marker)
const value = await ProductStatuses.getProductsByStatusMarker('my-marker')
Schema
marker:* string
Product marker
example: my-markerlangCode: string
language code
example: en_US
This method searches for a product status object based on its textual identifier (marker) from the API. It returns a Promise that resolves to a product status object.
Example return:
{
"id": 1764,
"version": 10,
"position": 2,
"identifier": "catalog",
"localizeInfos": {
"title": "Status 1"
}
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloglocalizeInfos: Record<string, any>
json description of the main page data object taking into account the language "en_US" (for example)
example: OrderedMap { "en_US": OrderedMap { "title": "Catalog", "plainContent": "Content for catalog", "htmlContent": "Content for catalog", "menuTitle": "Catalog" } }
Check the existence of a textual identifier (marker)
const value = await ProductStatuses.validateMarker('marker')
Schema
marker:* string
Product marker
example: marker
This method checks the existence of a textual identifier (marker). It takes a marker parameter as input, representing the product marker to validate. It returns a Promise that resolves to true if the textual identifier (marker) exists or false if it doesn't.
Example return:
true
The System module in OneEntry Headless CMS provides methods for testing error page redirections, allowing developers to ensure that their error handling mechanisms are functioning correctly. By using the defineOneEntry function, you can create a System object to access these functionalities. The module offers two primary methods: test404 and test500. Both methods simulate scenarios where the user is redirected to an error page, specifically 404 (Not Found) and 500 (Internal Server Error) pages, respectively. These tools are essential for verifying that the system's error pages are correctly implemented and displayed to users when needed.
const { System } = defineOneEntry('your-url');
const value = await System.test404()
This method allows you to redirect to the error page.
const value = await System.test500()
This method allows you to redirect to the error page.
Templates help configure the display of the content part of your application. Some templates affect the images stored in the CMS system, while others influence the appearance of other entities, such as blocks, pages, products, etc. In this section, you can familiarize yourself with the principles of working with templates.
const { Templates } = defineOneEntry('your-url');
Getting all template objects of a specific type
const value = await Templates.getAllTemplates()
Schema
langCode: string
Optional parameter language code
example: en_US
This method retrieves all template objects grouped by types from the API. It returns a Promise that resolves to an object GroupedTemplatesObject, which contains an array of template objects.
Example return:
{
"forTextBlock": [
{
"id": 1,
"version": 0,
"identifier": "for-block",
"title": "For blocks",
"generalTypeName": "forTextBlock",
"generalTypeId": 18,
"position": 1,
"attributeValues": {
"en_US": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
}
},
"attributeIdentifier": "my-set"
}
]
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloggeneralTypeId: number
type identifier
example: 4title: string
template name
example: page templateposition object
position number
example: 0generalTypeName string
example: forProductPreview
general type name
Getting all template objects, grouped by types
const value = await Templates.getTemplateByType('forCatalogProducts')
Schema
type:* Types
Product marker
example: forCatalogProductslangCode: string
Optional parameter language code
example: en_US
This method retrieves a single template object based on its identifier (id) from the API. It returns a Promise that resolves to a template object.
Example return:
[
{
"id": 1764,
"generalTypeId": 4,
"generalTypeName": "forCatalogProducts",
"title": "Page template",
"identifier": "marker",
"position": 1,
"version": 10,
"attributeValues": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
},
"attributeSetIdentifier": "my-set"
}
]
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloggeneralTypeId: number
type identifier
example: 4title: string
template name
example: page templateposition object
position number
example: 0generalTypeName string
example: forProductPreview
general type name
Get one template object by id.
const value = await Templates.getTemplateById(1)
Schema
id:* number
Template id
example: 1langCode: string
Optional parameter language code
example: en_US
This method retrieves a single template object based on its identifier (id) from the API. It returns a Promise that resolves to a template object.
Example return:
{
"id": 1764,
"generalTypeId": 4,
"generalTypeName": "forProductPreview",
"title": "Page template",
"identifier": "marker",
"position": 1,
"version": 10,
"attributeValues": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
},
"attributeSetIdentifier": "my-set"
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloggeneralTypeId: number
type identifier
example: 4title: string
template name
example: page templateposition object
position number
example: 0generalTypeName string
example: forProductPreview
general type name
Getting one template object by marker
const value = await Templates.getTemplateByMarker('my-marker')
Schema
marker:* number
Template marker
example: my-markerlangCode: string
Optional parameter language code
example: en_US
This method retrieves a single template object based on its identifier (marker) from the API. It returns a Promise that resolves to a template object.
Example return:
{
"id": 1764,
"generalTypeId": 4,
"generalTypeName": "forProductPreview",
"title": "Page template",
"identifier": "marker",
"position": 1,
"version": 10,
"attributeValues": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
},
"attributeSetIdentifier": "my-set"
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: cataloggeneralTypeId: number
type identifier
example: 4title: string
template name
example: page templateposition object
position number
example: 0generalTypeName string
example: forProductPreview
general type name
Preview templates allow you to configure the image parameters used in attributes. By applying a template to an attribute of type "Image" or "Image Group," you will get images standardized according to the specifications in the preview template. This is convenient if the design of your project is sensitive to image sizes. By configuring the preview template, you can change the image parameters that the end user will see.
const { TemplatePreviews } = defineOneEntry('your-url');
Getting all template objects
const value = await TemplatePreviews.getTemplatePreviews()
Schema
langCode: string
Optional parameter language code
example: en_US
This method retrieves all template objects from the API. It returns a Promise that resolves to an array of TemplatePreviewsEntity template objects.
Example return:
[
{
"id": 1,
"version": 0,
"identifier": "preview-templates",
"attributeValues": {
"en_US": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
}
},
"attributeSetIdentifier": "my-set",
"proportion": {
"vertical": {
"width": "2",
"height": "3",
"alignmentType": "leftTop",
"marker": "v"
},
"horizontal": {
"width": "234",
"height": "324",
"alignmentType": "middleBottom",
"marker": "h"
},
"square": {
"side": "3",
"alignmentType": "middleBottom",
"marker": "s"
}
},
"title": "Preview Templates",
"position": 1
}
]
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: catalogproportion ITemplateProportionType
template proportion parameters
example: OrderedMap { "horizontal": OrderedMap { "height": 200, "weight": 10, "marker": "horizontal", "title": "Horizontal", "alignmentType": "left" }, "vertical": OrderedMap { "height": 10, "weight": 200, "marker": "vertical", "title": "Vertical", "alignmentType": "left" }, "square": OrderedMap { "marker": "square", "title": "Square", "slide": 3, "alignmentType": "center" } }title: string
template name
example: page templateposition object
position number
example: 0positionId: number
position object identifier
example: 12
Getting one template object by id
const value = await TemplatePreviews.getTemplatePreviewById(1764)
Schema
id:* number
Product marker
example: 1764langCode: string
Optional parameter language code
example: en_US
This method retrieves a single template object based on its identifier (id) from the API. It returns a Promise that resolves to a TemplatePreviewsEntity object.
Example return:
{
"id": 1,
"version": 0,
"identifier": "preview-templates",
"attributeValues": {
"en_US": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
}
},
"attributeSetIdentifier": "my-set",
"proportion": {
"vertical": {
"width": "2",
"height": "3",
"alignmentType": "leftTop",
"marker": "v"
},
"horizontal": {
"width": "234",
"height": "324",
"alignmentType": "middleBottom",
"marker": "h"
},
"square": {
"side": "3",
"alignmentType": "middleBottom",
"marker": "s"
}
},
"title": "Preview Templates",
"position": 1
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: catalogproportion ITemplateProportionType
template proportion parameters
example: OrderedMap { "horizontal": OrderedMap { "height": 200, "weight": 10, "marker": "horizontal", "title": "Horizontal", "alignmentType": "left" }, "vertical": OrderedMap { "height": 10, "weight": 200, "marker": "vertical", "title": "Vertical", "alignmentType": "left" }, "square": OrderedMap { "marker": "square", "title": "Square", "slide": 3, "alignmentType": "center" } }title: string
template name
example: page templateposition object
position number
example: 0positionId: number
position object identifier
example: 12
Getting one template object by marker
const value = await TemplatePreviews.getTemplatePreviewByMarker('my-marker')
Schema
marker:* string
Product marker
example: my-markerlangCode: string
Optional parameter language code
example: en_US
This method retrieves a single template object based on its textual identifier (marker) from the API. It returns a Promise that resolves to a TemplatePreviewsEntity object.
Example return:
{
"id": 1,
"version": 0,
"identifier": "preview-templates",
"attributeValues": {
"marker": {
"value": "",
"type": "string",
"position": 1,
"isProductPreview": false,
"isIcon": false,
"attributeFields": {
"marker": {
"type": "string",
"value": "test"
}
}
}
},
"attributeSetIdentifier": "my-set",
"proportion": {
"vertical": {
"width": "2",
"height": "3",
"alignmentType": "leftTop",
"marker": "v"
},
"horizontal": {
"width": "234",
"height": "324",
"alignmentType": "middleBottom",
"marker": "h"
},
"square": {
"side": "3",
"alignmentType": "middleBottom",
"marker": "s"
}
},
"title": "Preview Templates",
"position": 1
}
Schema
id: number
object identifier
example: 1764version number
object's version number of modification
example: 10identifier: string
textual identifier for a field in the record
example: catalogproportion ITemplateProportionType
template proportion parameters
example: OrderedMap { "horizontal": OrderedMap { "height": 200, "weight": 10, "marker": "horizontal", "title": "Horizontal", "alignmentType": "left" }, "vertical": OrderedMap { "height": 10, "weight": 200, "marker": "vertical", "title": "Vertical", "alignmentType": "left" }, "square": OrderedMap { "marker": "square", "title": "Square", "slide": 3, "alignmentType": "center" } }title: string
template name
example: page templatepositionId object
position number
example: 0positionId: number
position object identifier
example: 12
In Headless CMS OneEntry, there are the necessary tools for working with registered users. In this section, you can familiarize yourself with the principles of working with users.
You can store the data necessary for your application to work in a state object. When changing the user, add the necessary data to the state. When the user's data is subsequently received, it will contain a state object.
An example in which we add information to the user about how many orders he has made. Add a field "orderCount" with the value to the state object.
const data = {
"formIdentifier": "reg",
"authData": [
{
"marker": "password",
"value": "12345"
}
],
"formData": [
{
"marker": "last_name",
"type": "string",
"value": "Username"
}
],
"notificationData": {
"email": "example@oneentry.cloud",
"phonePush": ["+99999999999"],
"phoneSMS": "+99999999999"
},
"state": {
"orderCount": 1
}
}
const value = await Users.updateUser(data)
When the user's data is received, it will contain information about the number of orders
const value = await Users.getUser()
console.log(value.state.orderCount) // 1
const { Users } = defineOneEntry('your-url');
Getting data of an authorized user 🔐 This method requires authorization.
const value = await Users.getUser()
Schema
langCode: string
lang code
example: en_US
This method sends a request to get the data of an authorized user. Returns the authorized user's data object.
Example return:
{
"id": 1764,
"identifier": "admin1",
"formIdentifier": "regForm",
"authProviderIdentifier": "email",
"groups": [
"group_1"
],
"state": {
"orderCount": 1
}
}
Schema
id: number
object identifier
example: 1764identifier: string
textual identifier for a field in the record
example: catalogformIdentifier string
the text identifier of the form linked to the authorization provider
example: , regFormauthProviderIdentifier: string
the text ID of the authorization provider
example: emailgroups string[]
An array of values for the text identifiers of the groups that the user belongs to
example: List [ "group_1" ]
Updating a single user object 🔐 This method requires authorization.
const body = {
"formIdentifier": "reg",
"authData": [
{
"marker": "password",
"value": "12345"
}
],
"formData": [
{
"marker": "last_name",
"type": "string",
"value": "Username"
}
],
"notificationData": {
"email": "example@oneentry.cloud",
"phonePush": ["+99999999999"],
"phoneSMS": "+99999999999"
},
"state": {
"orderCount": 1
}
}
const value = await Users.updateUser(body)
Schema
body:* object
Request body
example: {}langCode: string
Optional language field
example: en_US
Schema (body)
formIdentifier string
the text identifier of the form linked to the authorization provider
example: , regFormformData: FormDataLangType
the data of the form linked to the authorization provider
example: OrderedMap { "en_US": List [ OrderedMap { "marker": "first-name", "value": "First name" }, OrderedMap { "marker": "last-name", "value": "Second name" } ] }authData FormDataType
Authorization data taken from the form linked to the authorization provider (used only to change the password)
example: List [ OrderedMap { "marker": "password", "value": "12345" } ]notificationData UserNotificationDataType
Data for notifying the user
example: OrderedMap { "email": "example@oneentry.cloud", "phonePush": "", "phoneSMS": "+9999999999" }
This method updates the authorized user's data object. Returns true (in case of successful update) or false (in case of unsuccessful update).
Example return:
true
Deleting a single user object 🔐 This method requires authorization.
const value = await Users.deleteUser(body)
Schema
body:* object
Request body
example: []langCode: string
Optional language field
example: en_US
Adds FCM token for sending Push notifications 🔐 This method requires authorization.
const value = await Users.addFCMToken('my-token')
Schema
token:* string
Cloud messaging token
example: xxxx-xxxxx-xxxxx
This method adds FCM token for sending Push notifications. Return true in case of successful token update.
Example return:
true
Deletes FCM token 🔐 This method requires authorization.
const value = await Users.deleteFCMToken('my-token')
Schema
token:* string
Cloud messaging token
example: xxxx-xxxxx-xxxxx
This method delete FCM token for sending Push notifications. Return true in case of successful token deletion.
Example return:
true
You can subscribe to events via the WebSocket to receive notifications
const { WS } = defineOneEntry('your-url');
This method creates and return an instance of an object Socket.io with a connection. This method requires mandatory user authorization.
const socket = await WS.connect()
socket.on('my_event', callback)
When the attributes change (if the user is subscribed to the corresponding event and the Websocket option is active in the event) The "attributes" field always contains event attributes (the "events" field), and depending on the selected type of event for the catalog or form, the fields with the attributes "product" contain product attributes, and "user" contains fields from the user form, respectively. For a product, there is an additional field "title" (product name as a string), and for registration and code submission forms, there are additional fields "code" and "email."
The attribute value is stored in the format key - marker, value - {type, identifier, value}.
Example:
{
"message": "Message",
"attributes": {
"company": {
"identifier": "company",
"type": "string",
"value": "OneEntry"
}
}
}
Schema
message string
Text message
example: Messageattributes: Record<string, any>
Contains event attributes
example: { { "company": { "identifier": "company", "type": "string", "value": "OneEntry" } }}
Product Example:
{
"product": {
"id": 10,
"info": {
"title": "Pink Planet",
},
"status": {
"identifier": "available",
"info": {
"title": "ADD TO CART"
}
},
"attributes": {
"currency": {
"identifier": "currency",
"type": "string",
"value": "USD"
}
}
}
}
User Example:
{
"user": {
"notificationData": {},
"attributes": {}
},
"order": {
"id": 1,
"attributes": {}
}
}
Order Example:
{
"user": {
"notificationData": {},
"attributes": {}
},
"order": {
"id": 1,
"attributes": {}
}
}
Form Example:
{
"email": "string",
"formData": {}
}
FAQs
OneEntry NPM package
The npm package oneentry receives a total of 0 weekly downloads. As such, oneentry popularity was classified as not popular.
We found that oneentry demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.