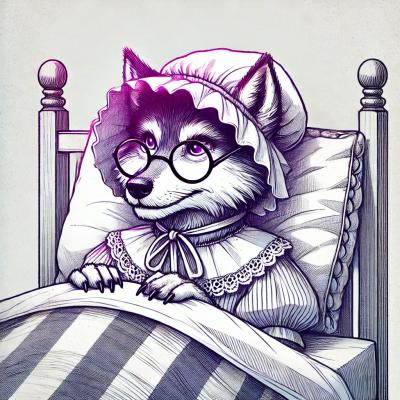
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
oro-postgresql
Advanced tools
Class OroPostgresql is a wrapper of npm-postgres to use it async/await
Class OroPostgres is a wrapper of npm-postgres to use it async/await.
npm-postgres is a psql API Wrapper for node.js.
npm install oro-postgresql
Example:
const { OPsql } = require( 'oro-postgresql' );
const settings = {
host: 'localhost',
port: '5432',
database: 'custom-database',
user: 'custom-user',
password: 'custom-password',
}
const server = new OPsql( { settings } );
const poolOpen = await server.poolOpen();
if( ! poolOpen.status ) { return poolOpen; }
const result = server.query( "SELECT * FROM table" );
const poolClose = await server.poolClose();
if( ! poolClose.status ) { return poolClose; }
console.log( result ); // resultArray
const { OPsql } = require( 'oro-postgres' );
const settings = {
host: 'localhost',
database: '',
user: 'root',
password: ''
}
const server = new OPsql( { settings } );
When it opens pool, the connection to database is created to execute queries.
const poolOpen = await server.poolOpen();
console.log( poolOpen ); // { status: true|false }
To close the opened pool.
const poolOpen = await server.poolOpen();
console.log( poolOpen ); // { status: true|false }
If you want to use the library pg
, you can get the class.
const psql = server.getClient();
When pool is open, you can get the npm-psql conn object.
const db = server.getDB();
Get settings info (without the password).
const info = server.getInfo();
Get the status object. If status is false, show the error message.
status
is only true
when pool is opened and it's enabled to call a query.
const statusObj = server.getStatus();
console.log( statusObj ); // { status: true|false }
Another way to simplify getting the status is directly with using the property server.status
.
console.log( server.status ); // true|false
const statusObj = server.getStatus();
console.log( statusObj ); // { status: true/false }
Get all resultArray
of the queries are saved in a heap.
Note: By default, you get a deep copy of each resultArray
to avoid modify data,
but if you need a better performance and you understand what are you doing, you can get the resultArray
as shallow copy.
const allResults = server.getAllQueries();
console.log( allResults ); // [ resultArray, ... ]
Get the last resultArray
of the queries, with the param offset
you can get the preceding queries.
Note: By default, you get a deep copy of the resultArray
to avoid modify data,
but if you need a better performance and you understand what are you doing, you can get the resultArray
as shallow copy.
const lastResult = server.getLastQuery();
console.log( lastResult ); // resultArray
Get the first resultArray
of the queries, with the param offset
you can get the following queries.
Note: By default, you get a deep copy of each resultArray
to avoid modify data,
but if you need a better performance and you understand what are you doing, you can get the resultArray
as shallow copy.
const firstResult = server.getFirstQuery();
console.log( firstResult ); // resultArray
Get the total number of rows that are affected in the last query.
const count = server.getAffectedRows();
console.log( count ); // integer
Sanitize the value to avoid code injections.
const valNumber = server.sanitize( 20 );
console.log( valNumber ); // `20`
const valString = server.sanitize( "chacho" );
console.log( valString ); // `'chacho'`
const valInjection = server.sanitize( "' OR 1 = 1" );
console.log( valInjection ); // `'\' OR 1 = 1'`
Note: It could be called as static too.
If you just need to call only one query, this function calls poolOpen, query, poolClose
respectively.
You can choose the format that return the query.
By default the returned object is resultArray
. This object extends from Array
and it has extra params.
{
status = true || false,
count = 0, // affected row
statement = 'QUERY';
columns = []; // table columns data
error?: { msg: 'error reason', ... } // only when status is false
}
"SELECT * FROM table"
.default
,bool
,count
,value
,values
,valuesById
,array
,arrayById
,rowStrict
,row
.row|array
, it maps each column-value, not the whole object.default
, return object resultArray.const resultArray = server.query( "SELECT * FROM table" );
// [
// 0: { ... },
// 1: { ... }
// status: true,
// count: 2,
// statement: "SELECT * FROM table",
// columns: [ ... ]
// ]
bool
, if the query has affected rows it returned true
.const result = server.query( "UPDATE table SET value WHERE condition", 'bool' );
// true
count
, return number of affected rows.const count = server.query( "SELECT * FROM table", 'count' );
// 2
value
, return the first column value.const value = server.query( "SELECT column FROM table", 'value' );
// column-value
const value2 = server.query( "SELECT * FROM table", 'value', 'column2' );
// column2-value
values
, return array
of column values.const values = server.query( "SELECT column FROM table", 'values' );
// [ column-value, ... ]
const values = server.query( "SELECT * FROM table", 'values', 'column2' );
// [ column2-value, ... ]
valuesById
, return object
of values with key as second column-value.const valuesById = server.query( "SELECT * FROM table", 'valuesById', 'column', 'column2' );
// { "column2-value": column-value, ... }
array
, return array
of object-row.const arr = server.query( "SELECT * FROM table", 'array' );
// [ row, ... ]
arrayById
, return object
of object-row with key as column-value.const arr = server.query( "SELECT * FROM table", 'arrayById', 'column' );
// { "column-value": { row }, ... }
row
, return object
row.const arr = server.query( "SELECT * FROM table", 'row' );
// { row }
rowStrict
, return object
row without columns with falsy values.const arr = server.query( "SELECT * FROM table", 'rowStrict' );
// { row }
If you want to run npm run test
, you can create your own ./test/config.json
(you can copypaste it from ./test/config-default.json
).
{
"host": "localhost",
"port": "5432",
"database": null,
"user": "postgres",
"password": ""
}
ADVISE: When run the testing, it's created and removed the database test_oropsql
,
so if config.user
has not permission to create database, you should create the database test_oropsql
manually.
On the other hand, if in your psql already exist test_oropsql
and it's required for you, avoid to run test
.
NOTE: If testing is not working because of I18n issue, change lc_messages
to english English_United States.1252
in $psqlDir\postgresql.conf
.
StackOverflow.
FAQs
Class OroPostgresql is a wrapper of npm-postgres to use it async/await
We found that oro-postgresql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.