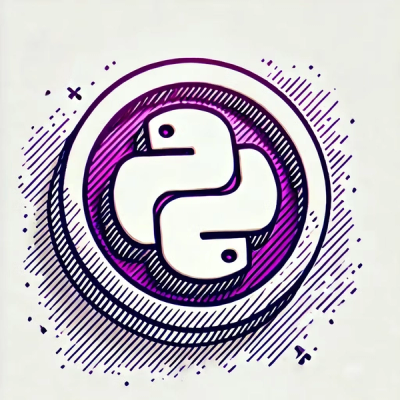
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
p-iteration
Advanced tools
Supply Chain Security
Vulnerability
Quality
Maintenance
License
The p-iteration npm package provides utility functions for working with asynchronous iterables in a more convenient and readable manner. It allows you to perform operations like map, forEach, filter, and reduce on promises and async functions, making it easier to handle asynchronous control flow.
map
The `map` function allows you to apply an asynchronous function to each element in an array and returns a new array with the results. This is useful for transforming data asynchronously.
const pIteration = require('p-iteration');
async function asyncMapExample() {
const numbers = [1, 2, 3, 4, 5];
const results = await pIteration.map(numbers, async (num) => {
return num * 2;
});
console.log(results); // [2, 4, 6, 8, 10]
}
asyncMapExample();
forEach
The `forEach` function allows you to perform an asynchronous operation on each element in an array without returning a new array. This is useful for side effects like logging or updating a database.
const pIteration = require('p-iteration');
async function asyncForEachExample() {
const numbers = [1, 2, 3, 4, 5];
await pIteration.forEach(numbers, async (num) => {
console.log(num);
});
}
asyncForEachExample();
filter
The `filter` function allows you to apply an asynchronous predicate function to each element in an array and returns a new array with only the elements that pass the predicate. This is useful for filtering data asynchronously.
const pIteration = require('p-iteration');
async function asyncFilterExample() {
const numbers = [1, 2, 3, 4, 5];
const results = await pIteration.filter(numbers, async (num) => {
return num % 2 === 0;
});
console.log(results); // [2, 4]
}
asyncFilterExample();
reduce
The `reduce` function allows you to apply an asynchronous reducer function to each element in an array, accumulating the results into a single value. This is useful for aggregating data asynchronously.
const pIteration = require('p-iteration');
async function asyncReduceExample() {
const numbers = [1, 2, 3, 4, 5];
const result = await pIteration.reduce(numbers, async (acc, num) => {
return acc + num;
}, 0);
console.log(result); // 15
}
asyncReduceExample();
Bluebird is a fully-featured promise library that provides a wide range of utilities for working with promises, including methods for mapping, filtering, and reducing collections of promises. It is more feature-rich and has better performance optimizations compared to p-iteration.
The async library provides a collection of functions for working with asynchronous JavaScript, including utilities for parallel and sequential execution of async functions. It offers similar functionalities to p-iteration but with a broader range of control flow utilities.
p-map is a smaller, focused library that provides a method for mapping over promises with concurrency control. It is similar to p-iteration's map function but does not include other utilities like forEach, filter, or reduce.
Make array iteration easy when using async/await and promises
Promise
, making them awaitable and thenable$ npm install --save p-iteration
Smooth asynchronous iteration using async/await
:
const { map } = require('p-iteration');
// map passing an async function as callback
function getUsers (userIds) {
return map(userIds, async userId => {
const response = await fetch(`/api/users/${userId}`);
return response.json();
});
}
// map passing a non-async function as callback
async function getRawResponses (userIds) {
const responses = await map(userIds, userId => fetch(`/api/users/${userId}`));
// ... do some stuff
return responses;
}
// ...
const { filter } = require('p-iteration');
async function getFilteredUsers (userIds, name) {
const filteredUsers = await filter(userIds, async userId => {
const response = await fetch(`/api/users/${userId}`);
const user = await response.json();
return user.name === name;
});
// ... do some stuff
return filteredUsers;
}
// ...
All methods return a Promise so they can just be used outside an async function just with plain Promises:
const { map } = require('p-iteration');
map([123, 125, 156], (userId) => fetch(`/api/users/${userId}`))
.then((result) => {
// ...
})
.catch((error) => {
// ...
});
If there is a Promise in the array, it will be unwrapped before calling the callback:
const { forEach } = require('p-iteration');
const fetchJSON = require('nonexistent-module');
function logUsers () {
const users = [
fetchJSON('/api/users/125'), // returns a Promise
{ userId: 123, name: 'Jolyne', age: 19 },
{ userId: 156, name: 'Caesar', age: 20 }
];
return forEach(users, (user) => {
console.log(user);
});
}
const { find } = require('p-iteration');
const fetchJSON = require('nonexistent-module');
function findUser (name) {
const users = [
fetchJSON('/api/users/125'), // returns a Promise
{ userId: 123, name: 'Jolyne', age: 19 },
{ userId: 156, name: 'Caesar', age: 20 }
];
return find(users, (user) => user.name === name);
}
The callback will be invoked as soon as the Promise is unwrapped:
const { forEach } = require('p-iteration');
// function that returns a Promise resolved after 'ms' passed
const delay = (ms) => new Promise(resolve => setTimeout(() => resolve(ms), ms));
// 100, 200, 300 and 500 will be logged in this order
async function logNumbers () {
const numbers = [
delay(500),
delay(200),
delay(300),
100
];
await forEach(numbers, (number) => {
console.log(number);
});
}
The methods are implementations of the ES5 Array iteration methods we all know with the same syntax, but all return a Promise
. Also, with the exception of reduce()
, all methods callbacks are run concurrently. There is a series version of each method, called: ${methodName}Series
, series methods use the same API that their respective concurrent ones.
There is a link to the original reference of each method in the docs of this module:
Extending native objects is discouraged and I don't recommend it, but in case you know what you are doing, you can extend Array.prototype
to use the above methods as instance methods. They have been renamed as async${MethodName}
, so the original ones are not overwritten.
const { instanceMethods } = require('p-iteration');
Object.assign(Array.prototype, instanceMethods);
async function example () {
const foo = await [1, 2, 3].asyncMap((id) => fetch(`/api/example/${id}`));
}
MIT © Antonio V
FAQs
Make array iteration easy when using async/await and Promises
The npm package p-iteration receives a total of 394,988 weekly downloads. As such, p-iteration popularity was classified as popular.
We found that p-iteration demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.