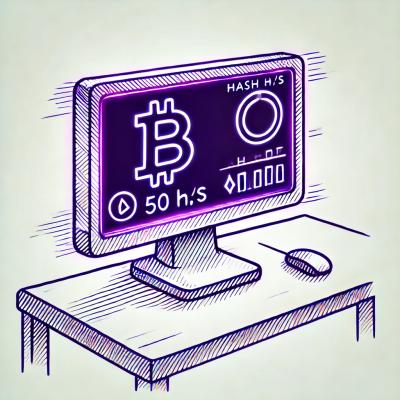
Security News
Research
Supply Chain Attack on Rspack npm Packages Injects Cryptojacking Malware
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Settle promises concurrently and get their fulfillment value or rejection reason
The p-settle npm package is used to settle multiple promises and get their results regardless of whether they were fulfilled or rejected. It returns an array of objects with the state and value/reason of each promise.
Settling multiple promises
This feature allows you to settle multiple promises and get their results regardless of whether they were fulfilled or rejected. The output is an array of objects with the status and value/reason of each promise.
const pSettle = require('p-settle');
const promises = [
Promise.resolve('Success'),
Promise.reject(new Error('Failure')),
Promise.resolve('Another success')
];
pSettle(promises).then(results => {
console.log(results);
/*
Output:
[
{ status: 'fulfilled', value: 'Success' },
{ status: 'rejected', reason: Error: Failure },
{ status: 'fulfilled', value: 'Another success' }
]
*/
});
The promise-settle package provides similar functionality to p-settle by settling multiple promises and returning their results. It also returns an array of objects with the state and value/reason of each promise. However, p-settle is more actively maintained and has a more modern API.
The settle-promise package is another alternative that settles multiple promises and returns their results. It is less popular and less actively maintained compared to p-settle, but it offers similar functionality.
The promise.allsettled package is a polyfill for the Promise.allSettled method, which is now a standard part of JavaScript. It provides the same functionality as p-settle but is built into the language itself, making it a more native solution.
Settle promises concurrently and get their fulfillment value or rejection reason
npm install p-settle
import fs from 'node:fs/promises';
import pSettle from 'p-settle';
const files = [
'a.txt',
'b.txt' // Doesn't exist
].map(fileName => fs.readFile(fileName, 'utf8'));
console.log(await pSettle(files));
/*
[
{
status: 'fulfilled',
value: '🦄',
isFulfilled: true,
isRejected: false,
},
{
status: 'rejected',
reason: [Error: ENOENT: no such file or directory, open 'b.txt'],
isFulfilled: false,
isRejected: true,
}
]
*/
Returns a Promise<object[]>
that is fulfilled when all promises from the array
argument are settled.
The objects in the array have the following properties:
status
('fulfilled'
or 'rejected'
, depending on how the promise resolved)value
or reason
(Depending on whether the promise fulfilled or rejected)isFulfilled
isRejected
Type: Array<ValueType | PromiseLike<ValueType> | ((...args: any[]) => PromiseLike<ValueType>)>
The array can contain a mix of any value, promise, and async function. Promises are awaited. Async functions are executed and awaited. The concurrency
option only works for elements that are async functions.
Type: object
Type: number
(Integer)
Default: Infinity
Minimum: 1
The number of concurrently pending promises.
Note: This only limits concurrency for elements that are async functions, not promises.
This is a type guard for TypeScript users.
This is useful since await pSettle(promiseArray)
always returns a PromiseResult[]
. This function can be used to determine whether PromiseResult
is PromiseFulfilledResult
or PromiseRejectedResult
.
This is a type guard for TypeScript users.
This is useful since await pSettle(promiseArray)
always returns a PromiseResult[]
. This function can be used to determine whether PromiseResult
is PromiseRejectedResult
or PromiseFulfilledResult
.
FAQs
Settle promises concurrently and get their fulfillment value or rejection reason
The npm package p-settle receives a total of 419,958 weekly downloads. As such, p-settle popularity was classified as popular.
We found that p-settle demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.
Security News
Sonar’s acquisition of Tidelift highlights a growing industry shift toward sustainable open source funding, addressing maintainer burnout and critical software dependencies.