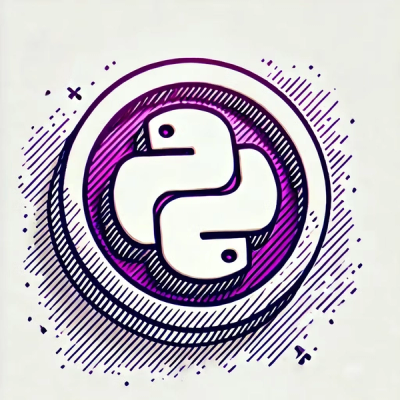
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
The p-try npm package is used to start a promise chain and execute a function asynchronously. It is a simple utility that allows you to attempt to execute a function and return a Promise that resolves with the function's result or rejects if the function throws an error.
Asynchronous function execution
This feature allows you to execute a function asynchronously and handle the result or error using promise chaining.
const pTry = require('p-try');
pTry(() => {
return 'result';
}).then(result => {
console.log(result); // 'result'
}).catch(error => {
console.error(error);
});
Bluebird is a full-featured promise library with a focus on innovative features and performance. It includes utilities for converting callback-based APIs to promises, advanced error handling, and concurrency helpers. Compared to p-try, Bluebird is more feature-rich and can be used for a wider range of promise-related tasks.
Q is one of the earliest promise libraries for JavaScript. It provides a robust set of features for creating and managing promises. While Q is more comprehensive than p-try, it is also larger in size and may be more complex for simple use cases where p-try would suffice.
ES6-Promise is a polyfill for the ES6 Promise specification. It provides the basic functionality needed to work with promises in environments that do not support them natively. Unlike p-try, which is a utility for starting promise chains, ES6-Promise is focused on implementing the standard Promise API.
Start a promise chain
npm install p-try
import pTry from 'p-try';
try {
const value = await pTry(() => {
return synchronousFunctionThatMightThrow();
});
console.log(value);
} catch (error) {
console.error(error);
}
Returns a Promise
resolved with the value of calling fn(...arguments)
. If the function throws an error, the returned Promise
will be rejected with that error.
Support for passing arguments on to the fn
is provided in order to be able to avoid creating unnecessary closures. You probably don't need this optimization unless you're pushing a lot of functions.
The function to run to start the promise chain.
Arguments to pass to fn
.
FAQs
`Start a promise chain
The npm package p-try receives a total of 53,139,358 weekly downloads. As such, p-try popularity was classified as popular.
We found that p-try demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.