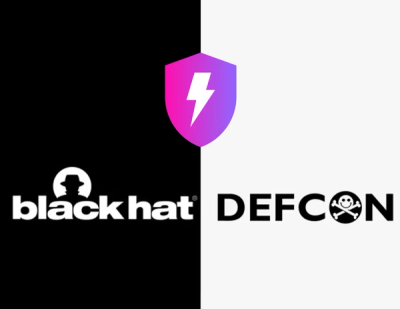
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
parse-bmfont-binary
Advanced tools
The parse-bmfont-binary npm package is used to parse binary BMFont files. BMFont is a bitmap font format that is commonly used in game development and other graphical applications. This package allows you to read and interpret the binary data from BMFont files, making it easier to use bitmap fonts in your projects.
Parsing Binary BMFont Files
This feature allows you to read and parse binary BMFont files. The code sample demonstrates how to read a BMFont file from the filesystem and parse it using the parse-bmfont-binary package.
const fs = require('fs');
const parseBMFont = require('parse-bmfont-binary');
fs.readFile('path/to/font.fnt', (err, data) => {
if (err) throw err;
const font = parseBMFont(data);
console.log(font);
});
The parse-bmfont-ascii package is used to parse ASCII BMFont files. While parse-bmfont-binary is designed for binary BMFont files, parse-bmfont-ascii handles the ASCII format. Both packages serve similar purposes but are tailored to different file formats.
Fontkit is a comprehensive font library that supports various font formats, including TrueType, OpenType, WOFF, and more. While parse-bmfont-binary is specialized for BMFont files, fontkit offers broader functionality for handling different types of font files.
Opentype.js is a JavaScript parser and writer for OpenType and TrueType fonts. It provides extensive functionality for working with these font formats, including parsing, editing, and rendering. Unlike parse-bmfont-binary, which focuses on BMFont files, opentype.js is geared towards OpenType and TrueType fonts.
Encodes a BMFont from a binary Buffer into JSON, as per the BMFont Spec. Can be used in Node or the browser (e.g. with browserify).
var parse = require('parse-bmfont-binary')
fs.readFile('fonts/Lato.bin', function(err, data) {
if (err) throw err
var font = parse(data)
//do something with your font
console.log(font.info.face)
console.log(font.info.size)
console.log(font.common.lineHeight)
console.log(font.chars)
console.log(font.kernings)
})
See text-modules for related modules.
font = parse(buffer)
Reads a binary BMFont Buffer and returns a new JSON representation of that font. See here for details on the return format.
MIT, see LICENSE.md for details.
FAQs
reads a BMFont binary in a Buffer into a JSON object
The npm package parse-bmfont-binary receives a total of 1,379,797 weekly downloads. As such, parse-bmfont-binary popularity was classified as popular.
We found that parse-bmfont-binary demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.