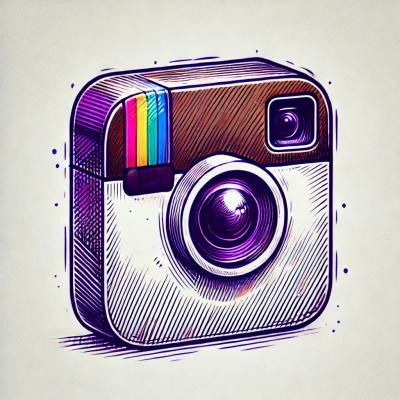
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
CSV parser for node.js.
Install with npm:
$ npm install parse-csv --save
Based on mr-data-converter by @shancarter. Copyright (c) 2011 Shan Carter.
var csv = require('parse-csv');
var str = [
'id,fruit,vegetable',
'1,apple,carrot',
'2,orange,corn',
'3,banana,potato'
].join('\n');
var obj = csv.toJSON(str, {headers: {included: true}});
console.log(obj);
Parse a string of CSV to a datagrid and render it using the specified renderer. The .json
renderer is used by default.
Params
method
{String}: The name of the renderer method to use, or a string of CSV. If CSV, the .json
method will be used.str
{String|Object}: String of CSV or options.options
{Object}returns
{String}Example
var str = `
id,fruit,vegetable
1,apple,carrot
2,orange,corn
3,banana,potato`;
var res = csv(str, {headers: {included: true}});
console.log(res);
// results in:
// [{"id":"1","fruit":"apple","vegetable":"carrot"},
// {"id":"2","fruit":"orange","vegetable":"corn"},
// {"id":"3","fruit":"banana","vegetable":"potato"}]
Parse a string of CSV to a datagrid, format it using one of the .json*
renderer methods, then parse it back to JSON.
Params
method
{String}: The name of the renderer method to use, or a string of CSV. If CSV, the .json
method will be used.str
{String|Object}: String of CSV or options.options
{Object}returns
{String}Example
var str = `
id,fruit,vegetable
1,apple,carrot
2,orange,corn
3,banana,potato`;
var res = csv.toJSON('jsonDict', str, {headers: {included: true}});
console.log(res);
// results in:
// { '1': { fruit: 'apple', vegetable: 'carrot' },
// '2': { fruit: 'orange', vegetable: 'corn' },
// '3': { fruit: 'banana', vegetable: 'potato' } }
Create a new Parser
with the given options
.
Params
options
{Options}Example
var csv = require('parse-csv');
var parser = new csv.Parser();
Parse CSV or tab-delimited string into a data-grid formatted JavaScript object.
Params
str
{String}options
{Object}returns
{Object}Example
var parser = new Parser();
var str = `
id,fruit,vegetable
1,apple,carrot
2,orange,corn
3,banana,potato`;
var datagrid = parser.parse(str);
// results in:
// { data:
// [ [ '1', 'apple', 'carrot' ],
// [ '2', 'orange', 'corn' ],
// [ '3', 'banana', 'potato' ] ],
// header:
// { names: [ 'id', 'fruit', 'vegetable' ],
// types: [ '-1': 'string' ] } }
Create a new Renderer
with the given options
.
Params
options
{Object}Example
var csv = require('parse-csv');
var renderer = new csv.Renderer();
The following render methods are available when the renderer is used directly. Or specify the renderer on options.renderer
when using the main export function.
.as
: Actionscript.asp
: ASP/VBScript.html
: HTML.json
: JSON - Properties.jsonArrayCols
: JSON - Column Arrays.jsonArrayRows
: JSON - Row Arrays.jsonDict
: JSON - Dictionary.mysql
: MySQL.php
: PHP.python
: Python - Dict.ruby
: Ruby.xmlProperties
: XML - Properties.xml
: XML - Nodes.xmlIllustrator
: XML - IllustratorExample
To render CSV as HTML:
var csv = require('parse-csv');
var renderer = new csv.Renderer();
var str = `
id,fruit,vegetable
1,apple,carrot
2,orange,corn
3,banana,potato`;
var html = renderer.html(str, {headers: {included: true}});
console.log(html);
Results in:
<table>
<thead>
<tr>
<th class="id-cell">id</th>
<th class="fruit-cell">fruit</th>
<th class="vegetable-cell">vegetable</th>
</tr>
</thead>
<tbody>
<tr class="firstRow">
<td class="id-cell">1</td>
<td class="fruit-cell">apple</td>
<td class="vegetable-cell">carrot</td>
</tr>
<tr>
<td class="id-cell">2</td>
<td class="fruit-cell">orange</td>
<td class="vegetable-cell">corn</td>
</tr>
<tr class="lastRow">
<td class="id-cell">3</td>
<td class="fruit-cell">banana</td>
<td class="vegetable-cell">potato</td>
</tr>
</tbody>
</table>
Available parser options and the actual defaults used.
{
headers: {
included: false,
downcase: true,
upcase: true
},
delimiter: 'tab',
decimalSign: 'comma'
}
Available renderer options and the actual defaults used.
{
headers: {
included: true,
downcase: true,
upcase: true
},
delimiter: 'tab',
decimalSign: 'comma',
outputDataType: 'json',
columnDelimiter: "\t",
rowDelimiter: '\n',
inputHeader: {},
outputHeader: {},
dataSelect: {},
outputText: '',
newline: '\n',
indent: ' ',
commentLine: '//',
commentLineEnd: '',
tableName: 'converter',
useUnderscores: true,
includeWhiteSpace: true,
useTabsForIndent: false
}
You might also be interested in these projects:
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Generate readme and API documentation with verb:
$ npm install verb && npm run docs
Or, if verb is installed globally:
$ verb
Install dev dependencies:
$ npm install -d && npm test
Jon Schlinkert
Copyright © 2016, Jon Schlinkert. Released under the MIT license.
This file was generated by verb, v0.9.0, on May 09, 2016.
FAQs
CSV parser for node.js.
The npm package parse-csv receives a total of 102 weekly downloads. As such, parse-csv popularity was classified as not popular.
We found that parse-csv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.