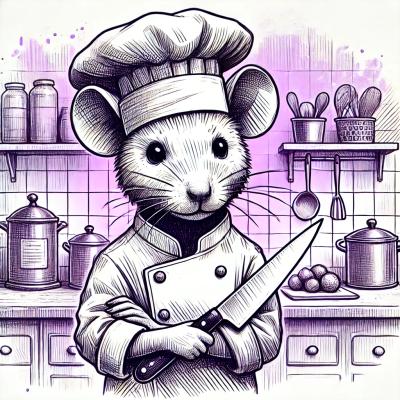
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The 'pinkie' npm package is a lightweight promise implementation that follows the Promises/A+ specification. It is primarily used for handling asynchronous operations in environments where native promises are not available or when a minimalistic promise library is preferred.
Promise creation and resolution
This code demonstrates how to create a new promise using pinkie and resolve it with a value. The 'then' method is used to handle the resolved value.
const pinkie = require('pinkie');
const promise = new pinkie((resolve, reject) => {
resolve('Success!');
});
promise.then(result => console.log(result));
Promise rejection and error handling
This example shows how to create a promise that gets rejected with an error and how to handle the error using the 'catch' method.
const pinkie = require('pinkie');
const promise = new pinkie((resolve, reject) => {
reject(new Error('Failed!'));
});
promise.catch(error => console.error(error.message));
Bluebird is a full-featured promise library with a focus on innovative features and performance. It includes utilities for concurrency, which are not present in pinkie, making it more suitable for complex applications.
Q is another popular promise library that supports more advanced features like promise chaining and combination, deferreds, and progress notifications, which go beyond the basic functionality provided by pinkie.
Itty bitty little widdle twinkie pinkie ES2015 Promise implementation
There are tons of Promise implementations out there, but all of them focus on browser compatibility and are often bloated with functionality.
This module is an exact Promise specification polyfill (like native-promise-only), but in Node.js land (it should be browserify-able though).
$ npm install --save pinkie
var fs = require('fs');
var Promise = require('pinkie');
new Promise(function (resolve, reject) {
fs.readFile('foo.json', 'utf8', function (err, data) {
if (err) {
reject(err);
return;
}
resolve(data);
});
});
//=> Promise
pinkie
exports bare ES2015 Promise implementation and polyfills Node.js rejection events. In case you forgot:
Returns new instance of Promise
.
Required
Type: function
Function with two arguments resolve
and reject
. The first argument fulfills the promise, the second argument rejects it.
Returns a promise that resolves when all of the promises in the promises
Array argument have resolved.
Returns a promise that resolves or rejects as soon as one of the promises in the promises
Array resolves or rejects, with the value or reason from that promise.
Returns a Promise object that is rejected with the given reason
.
Returns a Promise object that is resolved with the given value
. If the value
is a thenable (i.e. has a then method), the returned promise will "follow" that thenable, adopting its eventual state; otherwise the returned promise will be fulfilled with the value
.
MIT © Vsevolod Strukchinsky
FAQs
Itty bitty little widdle twinkie pinkie ES2015 Promise implementation
The npm package pinkie receives a total of 5,384,799 weekly downloads. As such, pinkie popularity was classified as popular.
We found that pinkie demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.